-
Notifications
You must be signed in to change notification settings - Fork 1
Architecture
- Frameworks: Next.js and React
- Language: Typescript
-
Libraries:
- Next-Auth
- Axios
- APIs: Spotify Web API
- Package Manager: Yarn
-
What each person will work on:
- Dalton: configuration of infrastructure/build tools, Next setup, API routes
- Kevin: database development, Frontend UX/UI
- Mason: development/maintenance of database, development of the various pages, interfacing with the Spotify Web API
- Sophie: Frontend, database setup, cloud service setup
To deploy the application, we’ll be using Vercel. Vercel has a GitHub integration that allows for continuous deployment, so any commit pushed to the main branch will auto-trigger a deployment. We will use branch protection rules + PR reviews to ensure only high-quality code is deployed.
It’s a mix - React is SPA but Next.js adds traditional routing features.
The primary feed is the core feature of the app. In a shift from our original designs, we’ve decided to merge both the “feed” and “forums” concept into one cohesive page. An optional ID parameter will allow for permalinking to an individual post, as well as allowing the comments/reactions to be displayed.
Going to /profile
will redirect to the current user’s profile; any other profile will open via the username.
- /profile (redirect to current user’s profile)
- /profile/[id]
No arguments are needed for the settings page.
Search will be handled in two ways: a search query followed by a single optional scope (artist, song, playlist, or user). If no scope is provided, the query will be applied to all possible categories.
-
/search
- /[search-query]/[scope]
Query parameters for following methods
- GET - Retrieves resources
- POST - Creates resources
- PUT - Changes and/or replaces resources or collections
- DELETE - Deletes resources
- Spotify ID - Base-62 identifier that finds artist, track, album, playlist, etc
- Spotify user ID/URL - Unique string to identify Spotify User


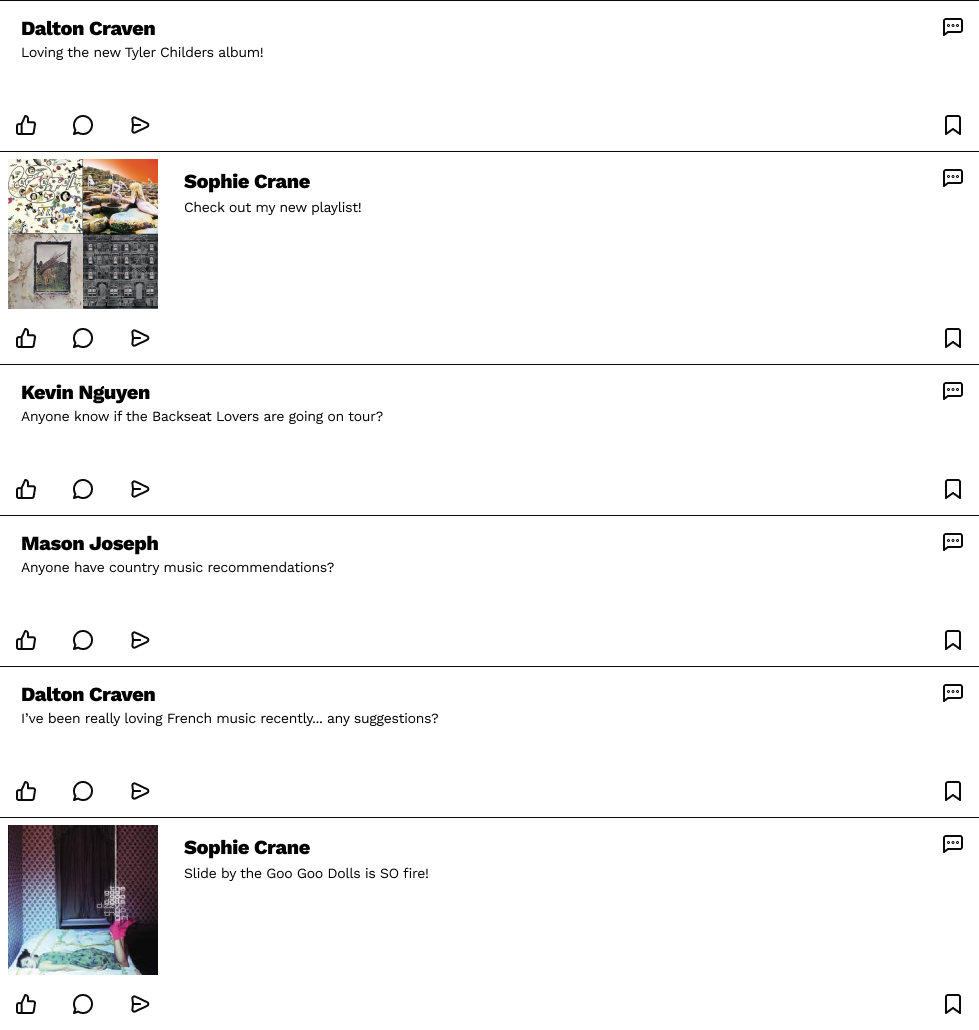

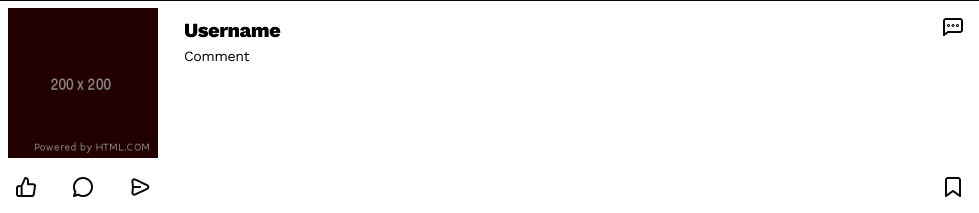

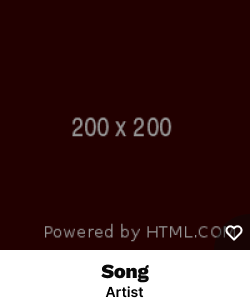



Posts:
- id: int that is used to distinguish each post
- type: string that distinguishes between 'feed' and 'forum' posts
- username: string that identifies the user who owns the post
- body: string that contains the content of the post
- likes: int that indicates how many likes a post has received
- comments: list of comments posted by the owner of the post or other users
- picture: image that contains the picture associated with the post, should the user choose to include a picture
Users:
- id: int that is used to distinguish each user
- username: string that displays in the app to distinguish between users on the front end of the app
- profilePicture: image that displays the user’s profile picture in the ‘Profile’ page of the app
- pinnedSongs: list of songs that contains the songs a user has pinned
- playlists: list of playlists which contains all the user’s public playlists.
- followedArtists: list of artist id’s that the user is following
- friends: list of user id’s that the user is following
- showListeningActivity: boolean that allows the user to choose whether to make their listening activity public to their friends
- language: string that allows the user to select a language preference in the app
- volume: int that controls the maximum playback volume
- autoplay: boolean that allows the user to choose whether music will play automatically at the end of a previous song
- privateAccount: boolean that allows the user to choose whether to make their profile public or private
- posts: list of post id’s that belong to the user
- notifications: list of notification id’s that belong to the user
Comments:
- id: int that is used to distinguish each comment
- username: string that represents the user who posted the comment
- body: string that contains the content of the comment
- time: smalldatetime that provides a timestamp of the comment
Notifications:
- id: int that is used to distinguish each notification
- title: string that gives a brief indication of the notification’s content
- time: smalldatetime that gives the timestamp of the notification
- body: string that gives a description of the notification
Artists:
- id: int that is used to distinguish each artist
- name: string that indicates the name of the artist
- albums: list of album id’s for album released by the artist
- songs: list of song id’s for songs released by the artist
Albums:
- id: int that is used to distinguish each album
- title: string that indicates the title of the album
- songs: list of song id’s for songs included on the album
- releaseDate: date that indicates the date the album was released
Songs:
- id: int that is used to distinguish each song
- title: string that indicates the title of the song
- releaseDate: date that indicates the date the song was released
Playlists:
- id: int that is used to distinguish each playlist
- title: string that indicates the title of the playlist
- owner: user id that indicates the owner of the playlist
- songs: list of song id's for songs included in the playlist
Create Post:
- INSERT INTO Posts (id, type, username, body, likes, picture)
Delete Post:
- DELETE FROM Posts
- WHERE id = x;
Create Comment:
- INSERT INTO Comments (id, username, body, title)
Search for Artist:
- SELECT name
- FROM Artists
- WHERE name = ‘xyz’;
Search for Album:
- SELECT title
- FROM Albums -WHERE title = ‘xyz’;
Search for Song:
- SELECT title
- FROM Songs
- WHERE title = ‘xyz’;
Search for Playlist:
- SELECT title
- FROM Playlists
- WHERE title = ‘xyz’;
Change Settings:
-
UPDATE Settings
-
SET showListeningActivity = !showListeningActivity;
-
UPDATE Settings
-
SET Volume = x;
-
UPDATE Settings
-
SET Language = ‘English’;