-
Notifications
You must be signed in to change notification settings - Fork 0
Architecture
- We will use React for the front-end of our web app, with a MERN (MongoDB, Express.JS, React.JS, Node.JS) stack to support mongoDB. Express.JS and Node.JS will be used for the server backend of our web app.
- We will all use npm.
- We will all do full-stack development, but each section of our app will have "leaders" who are responsible for checking on the status of these areas: front-end, back-end, databases, deployment. Rahul and Alfred will lead front-end. Sai will lead databases. Jack will lead deployment. Hobbs will lead back-end.
- No images needed
-
To deploy our bookstore web application we plan on using DigitalOcean. Embedded into DigitalOcean comes the option of using the mongoDB database which will be used for storing user information and the book inventory. Integrated with this, we plan to use the Amazon API for book browsing and potential book purchases. The stored user information and book inventory will follow JSON formatting. https://www.digitalocean.com/community/tutorials/how-to-deploy-a-react-application-to-digitalocean-app-platform
-
In using DigitalOcean as the method to deploy our bookstore web application, we will be using Docker containers for development. Using these containers for our web app are essential because they are small, executable packages which are easy to use for development and running the application. The up side to Docker is that it is faster, scalable, and easy to use compared to virtual-machines. https://www.docker.com/
-
We will use a traditional web app model because the DigitalOcean hosting platform will easily handle the small load of server operations for our app.
http://bookstore.app/create-account
Redirects to home
http://bookstore.app/terms-of-service
5. REST API
get/ : gets information on spa
account/post : creates account
storefront/get: gets book inventory
storefront/subscribe/post: adds email to subscription list
storefront/#book id#/get: gets information on specific book
community/get : get forums
community/post : create forum
community/put : update forum
community/delete : delete forum
community/#forum id#/get/ : get posts under forum
community/#forum id#/post : create post under forum
community/#forum id#/delete : delete a post under forum
community/#forum id#/#post id#/get : get comments under forum post
community/#forum id#/#post id#/post : add comment
community/#forum id#/#post id#/put : update a comment
community/#forum id#/#post id#/delete: delete a comment
- View
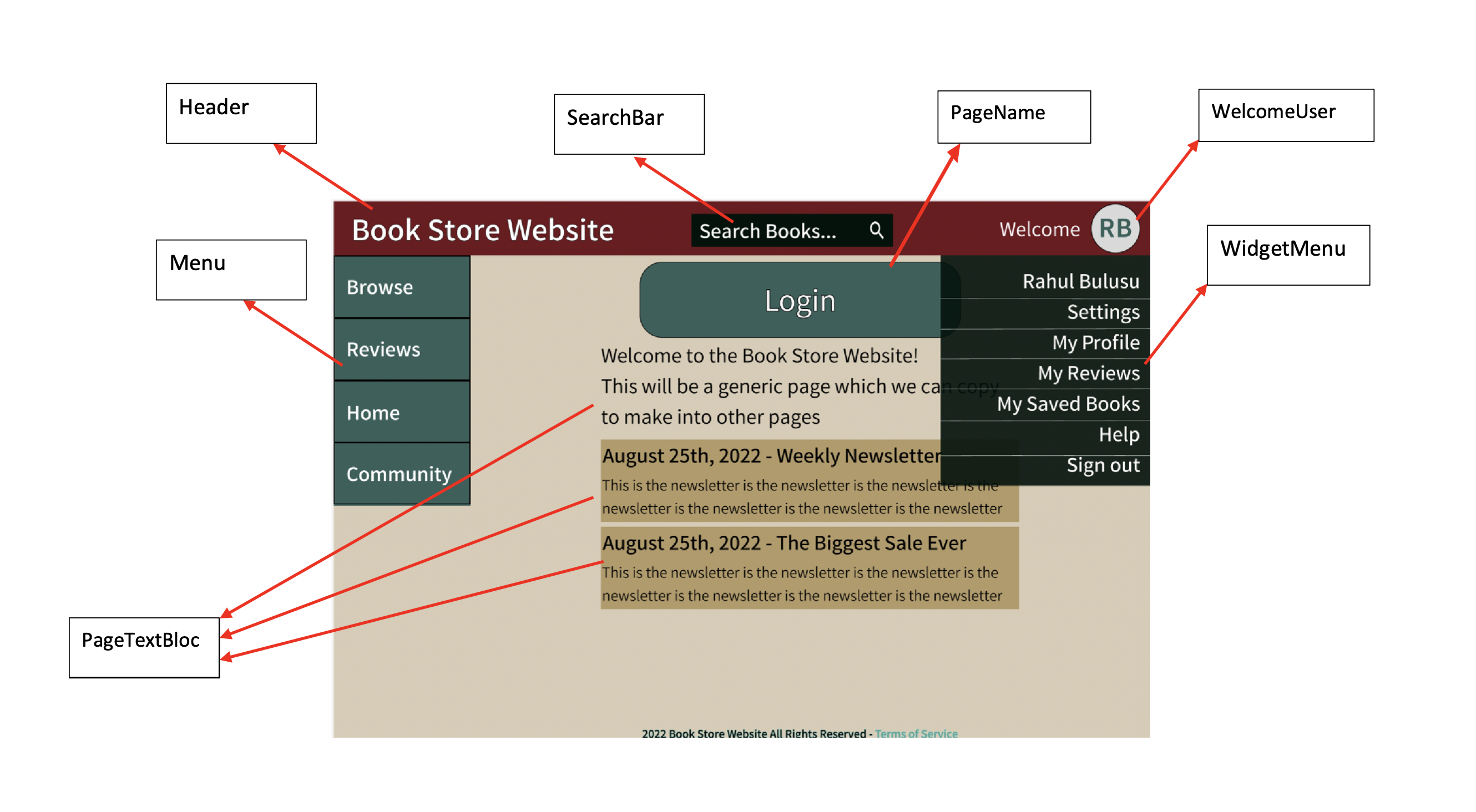
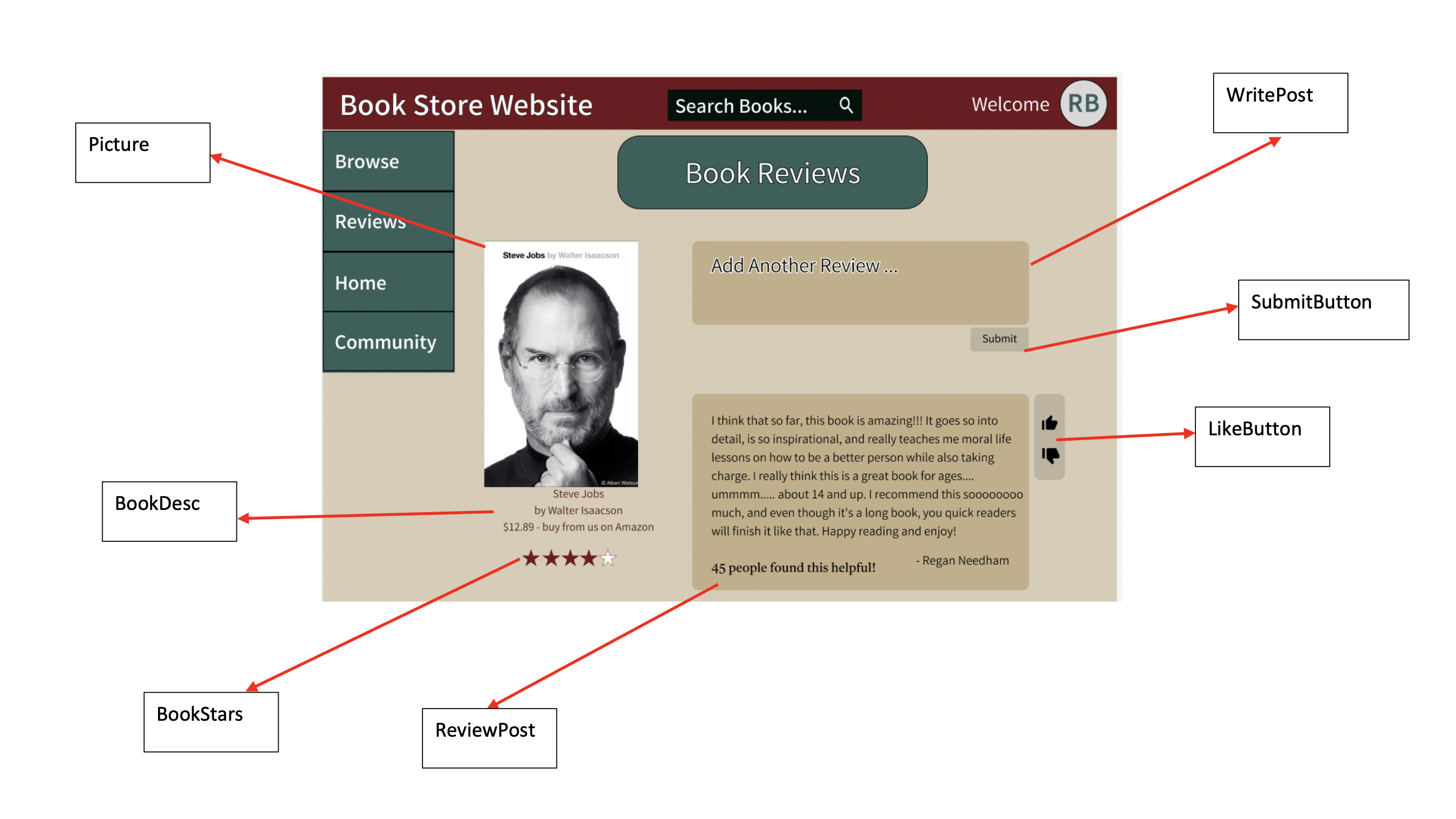
- Header: where it contains the name of the bookstore website, search book, and the welcome of the user.
- SearchBar: it searches for book, like a quick search.
- PageName: name of the page that the user is currently on.
- WelcomeUser: shows the initial of the user's name where they can click on it to access the widget menu.
- WidgetMenu: where the user can access their account setting, my profile, my review, my saved books, help, or sign out.
- PageTextBloc: a description of what they are seeing on the current page.
- Menu: a quick access to some popular pages throughout the website.
- WritePost: a place where the user can write a review about their book.
- SubmitButton: user can submit their stuff with a click.
- LikeButton: user can like or dislike on what they are seeing.
- ReviewPost: user can see their submitted post or other user's posts about the book.
- BookStars: stars that represents the review of the users' rating about the book.
- BookDesc: a quick description about the book.
- Picture: picture on the page of the website.
- This section contains the example of our database schematic with sets of tables/documents and list of attributes with their types.
This is an example of a collection that contains only the information pretaining to the user login.
- User-ID : UID. It is a unique id to every user.
- Username : String
- Email : String
- Password: String
User-ID | Username | Password | |
---|---|---|---|
62d01d17cdd1b7c8a5f945b9 | {"abrjohn_201"} | {"[email protected]"} | {"Password123"} |
62d01d17cdd1b7c8a5f945ba | {"will_minos"} | {"[email protected]"} | {"NRg05$I8je4k"} |
The table below is an example of a collection that contains information about the saved books, reviews, searched books, and purchased books of a user.
- User-ID : UID. It is a unique id to every user.
- Username : String
- Saved Books : String. This is a set of strings that contain books saved by the user.
- Reviews: String. This is a dictionary that contains all of the reviews each user left at a particular time.
- Searched Books : String. This is a set of strings that contain books searched by the user.
- Purchased Books : String. This is a set of strings that contain books saved by the user.
User-ID | Username | Saved Books | Reviews | Searched Books | Purchased Books |
---|---|---|---|---|---|
62d01d17cdd1b7c8a5f945b9 | {"abrjohn_201"} | {"The Great Gatsby", "The Grapes of Wrath", "Ninteen Eighty Four"} | {} | {"The Great Gatsby", "The Grapes of Wrath", "Ninteen Eighty Four"} | {"The Great Gatsby"} |
62d01d17cdd1b7c8a5f945ba | {"will_minos"} | {"Pride and Prejudice", "The Red and the Black"} | {"Moby-Dick":{"Date and time": "10-12-2022 19:52:31", "text": "This was a difficult book to get into but an amazing read nontheless"}} | {"Moby Dick", "Pride and Prejudice", "The Red and the Black", "War and Peace"} | {"Moby Dick", "War and Peace"} |
To manage the books, the table below is an example of a collection the admin has access to manage books. The user can add reviews, but it is managed by the admin.
- Book Name : String. Contains the book name.
- Author : String. Contains the name of the author.
- Publication Date : String. Contains the publication date of the book.
- Quantity : String Contains the number of book currently available for purchase.
- Reviews : String. It is a set of sets of strings that contain reviews and the date and time it was reviewed.
- Thumbs Up : Int. Number of up votes for a book.
- Thumbs Down : Int. Number of down votes for a book.
Book Name | Author | Publication Date | Quantity | Reviews | Thumbs Up | Thumbs Down |
---|---|---|---|---|---|---|
{"The History of Tom Jones, a Foundling"} | {"Henry Fielding"} | {"28 February 1749"} | 12 | {} | 12 | 5 |
{"Pride and Prejudice"} | {"Jane Austen"} | {"28 January 1813"} | 9 | {"Date and time": "04-02-2021 10:15:32", "text": "This book is quite possibly the most insipid novel I have ever read in my life."} | 1 | 2 |
{"The Red and the Black"} | {"Stendhal"} | {"November 1830"} | 1 | {"Date and time": "11-12-2022 19:52:31", "text": "The Red and the Black draws a colorful mosaic about the required hypocrisy to climb the ladder of social status in the France of the July Revolution."} | 4 | 1 |
{"Le Père Goriot"} | {"Honoré de Balzac"} | {"February 1835"} | 1 | {} | 2 | 3 |
{"David Copperfield "} | {"Charles Dickens"} | {"November 1850"} | 3 | {"Date and time": "07-03-2022 12:24:45", "text": ""} | 1 | 0 |
{"Madame Bovary"} | {"Gustave Flaubert"} | {"April 1857"} | 4 | {"Date and time": "10-02-2020 12:34:12", "text": "Nothing is Black and White in the world of Flaubert."} | 9 | 2 |
{"Moby-Dick"} | {"Herman Melville"} | {"October 18, 1851} | 17 | {"Date and time": "10-12-2022 19:52:31", "text": "This was a difficult book to get into but an amazing read nontheless"} | 14 | 6 |
{"Wuthering Heights"} | {"Emily Brontë"} | {"December 1847"} | 5 | {"Date and time": "02-02-2021 02:21:44", "text": ""} | 8 | 5 |
{"War and Peace"} | {"Tolstoy"} | {"1869"} | 2 | {"Date and time": "09-04-2022 12:53:39", "text": "This book is a classic for good reason."} | 2 | 0 |
- Our website is using MongoDB to store our data. MongoDB is an NoSQL database management program. The command to query in MongoDB is different from SQL.
returns the entire user-info collection
returns all of the user info for user named "abrjohn_201". The name can be modified to return info for the desired user in this collection.
db.user-info.find({email: "[email protected]"})
returns all of the user info for user with email "[email protected]". The email can be modified to return info for the desired user in this collection. This can be used in a scenario where if an email already exists a new user cannot create an account with that email.
returns all of the user content for user named "abrjohn_201". The returned info will contain info about the user's saved books, reviews, searched books, and purchased books.
returns all of the users who saved "The Great Gatsby".
returns all of the users who searched for "The Great Gatsby".
returns all of the users who bought "The Great Gatsby".
returns all of the info on "The Great Gatsby". This command can be used to load books to the website depending on the quantity.
returns all of books written by "Jane Austen".
returns all of books that are available for sale.