-
Notifications
You must be signed in to change notification settings - Fork 72
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
48 changed files
with
2,009 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
name: Publish wiki | ||
on: | ||
push: | ||
branches: [ main ] | ||
paths: | ||
- wiki/** | ||
- .github/workflows/publish-wiki.yml | ||
|
||
concurrency: | ||
group: publish-wiki | ||
cancel-in-progress: true | ||
|
||
permissions: | ||
contents: write | ||
|
||
jobs: | ||
publish-wiki: | ||
runs-on: ubuntu-latest | ||
steps: | ||
- uses: actions/checkout@v3 | ||
- uses: Andrew-Chen-Wang/github-wiki-action@v4 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
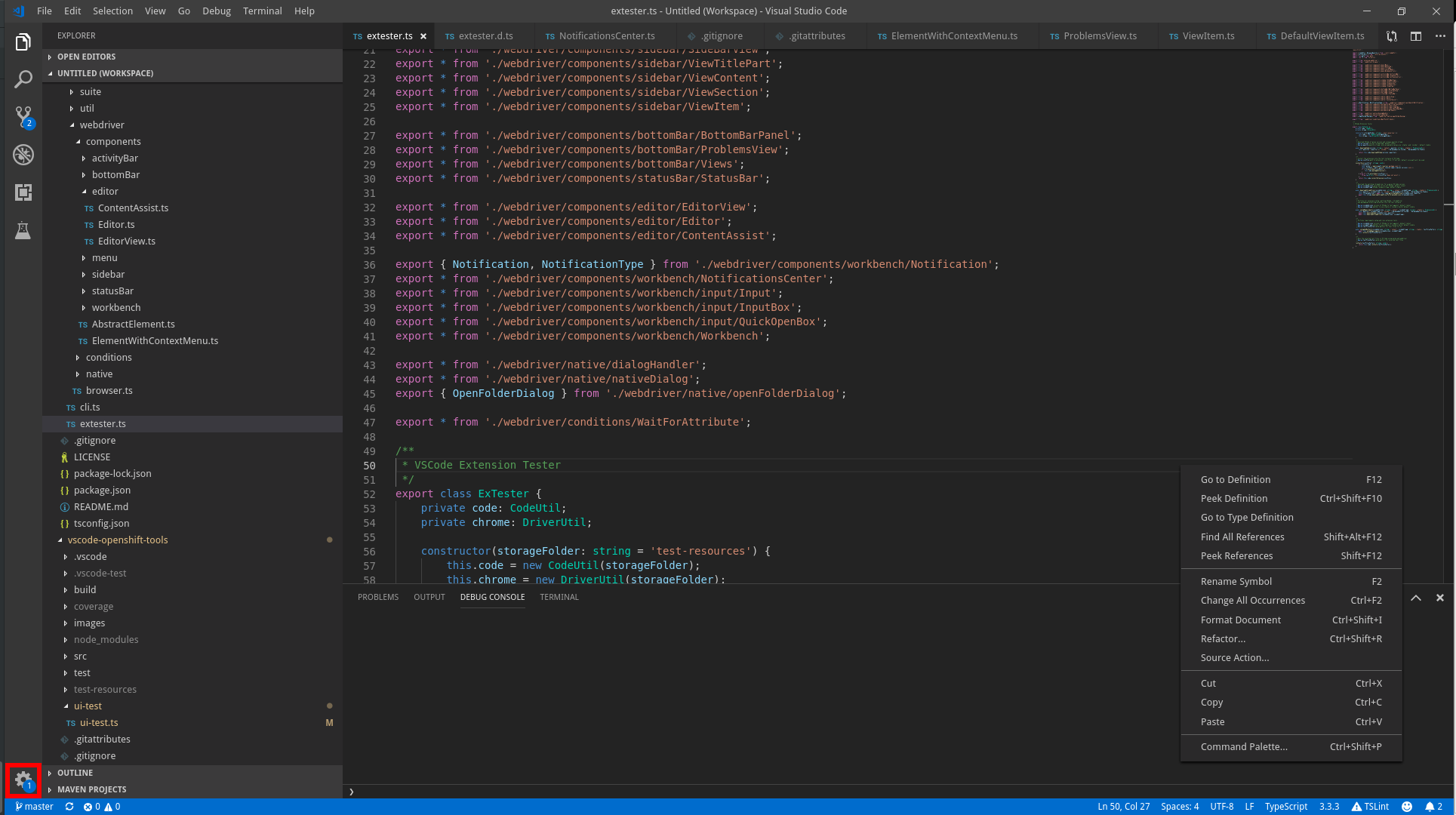 | ||
|
||
#### Look up the ActionsControl by title | ||
Import and find the control through activity bar | ||
```typescript | ||
import { ActivityBar, ActionsControl } from 'vscode-extension-tester'; | ||
... | ||
// get actions control for 'Manage' | ||
const control: ActionsControl = new ActivityBar().getGlobalAction('Manage'); | ||
``` | ||
|
||
#### Open action menu | ||
Click the action control to open its context menu | ||
```typescript | ||
const menu = await control.openActionMenu(); | ||
``` | ||
|
||
#### Get title | ||
Get the control's title | ||
```typescript | ||
const title = control.getTitle(); | ||
``` | ||
|
||
#### Open context menu | ||
Left click on the control to open the context menu (in this case has the same effect as openActionMenu) | ||
```typescript | ||
const menu = await control.openContextMenu(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
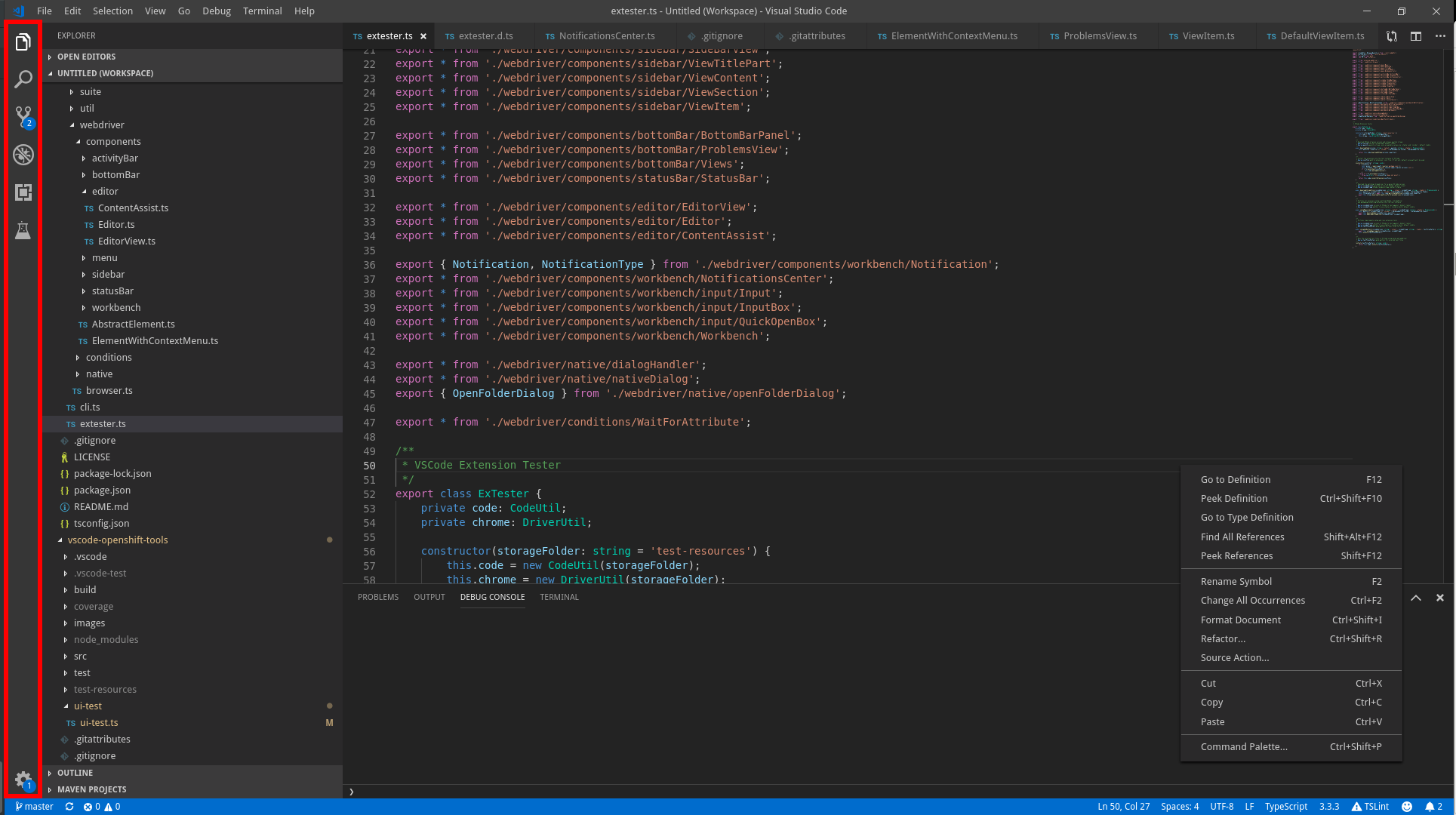 | ||
|
||
#### Look up the Activity Bar | ||
Import and find the activity bar | ||
```typescript | ||
import { ActivityBar } from 'vscode-extension-tester'; | ||
... | ||
const activityBar = new ActivityBar(); | ||
``` | ||
|
||
#### Get view controls | ||
Get handles for all view controls/buttons that operate the view containers | ||
```typescript | ||
const controls = await activityBar.getViewControls(); | ||
``` | ||
|
||
#### Get view control by title | ||
Find a view control/button in the activity bar by its title | ||
```typescript | ||
// get Explorer view control | ||
const controls = await activityBar.getViewControl('Explorer'); | ||
``` | ||
|
||
#### Get global actions | ||
Get handles for all global actions buttons on the bottom of the action bar | ||
```typescript | ||
const actions = await activityBar.getGlobalActions(); | ||
``` | ||
|
||
#### Get global action by title | ||
Find global actions button by title | ||
```typescript | ||
const actions = await activityBar.getGlobalAction('Manage'); | ||
``` | ||
|
||
#### Open context menu | ||
Left click on the activity bar to open the context menu | ||
```typescript | ||
const menu = await activityBar.openContextMenu(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
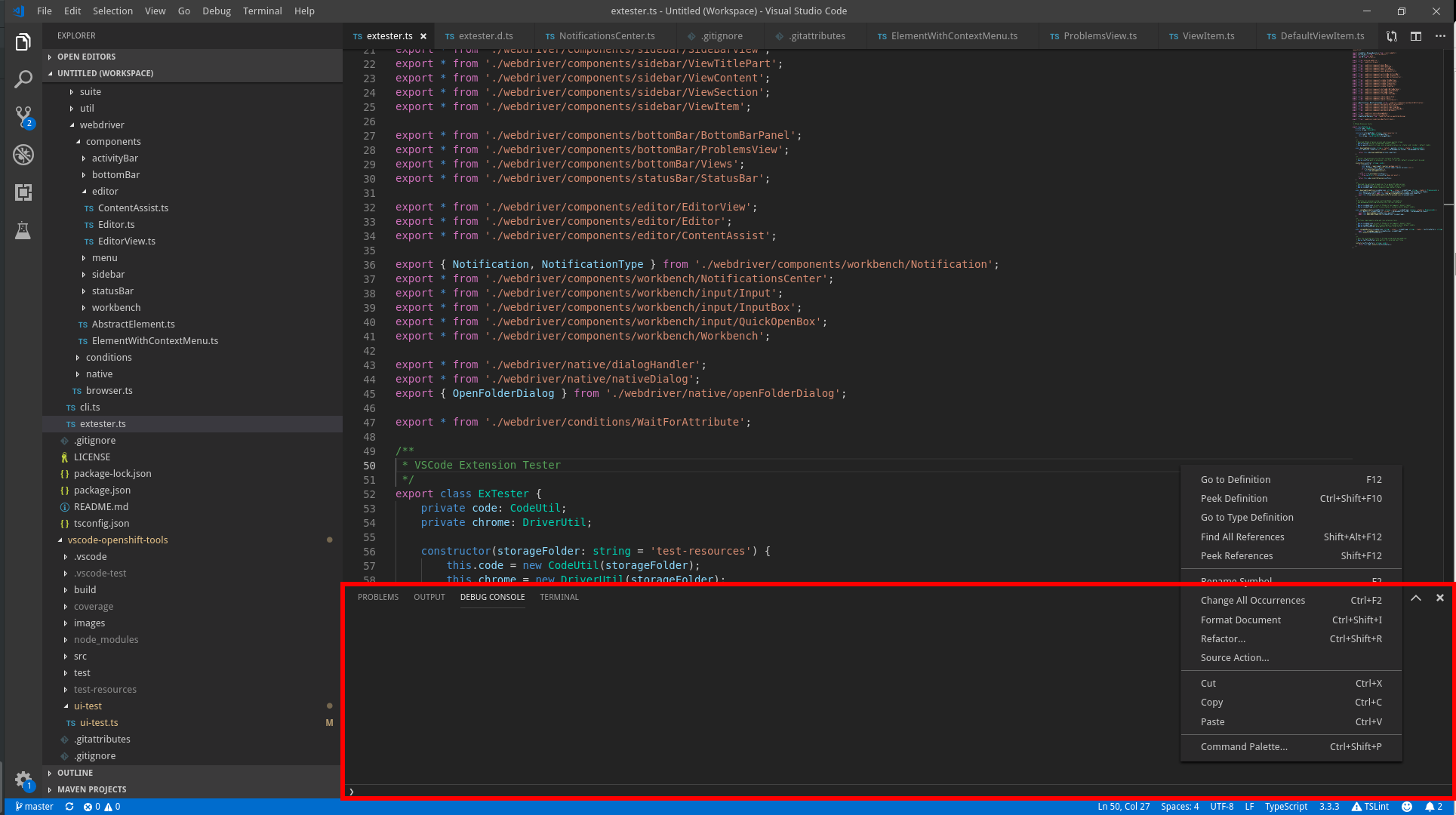 | ||
|
||
#### Lookup | ||
```typescript | ||
import { BottomBarPanel } from 'vscode-extension-tester'; | ||
... | ||
const bottomBar = new BottomBarPanel(); | ||
``` | ||
|
||
#### Open/Close the panel | ||
```typescript | ||
// open | ||
await bottomBar.toggle(true); | ||
// close | ||
await bottomBar.toggle(false); | ||
``` | ||
|
||
#### Maximize/Restore the panel | ||
```typescript | ||
await bottomBar.maximize(); | ||
await bottomBar.restore(); | ||
``` | ||
|
||
#### Open specific view in the bottom panel | ||
```typescript | ||
const problemsView = await bottomBar.openProblemsView(); | ||
const outputView = await bottomBar.openOutputView(); | ||
const debugConsoleView = await bottomBar.openDebugConsoleView(); | ||
const terminalView = await bottomBar.openTerminalView(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
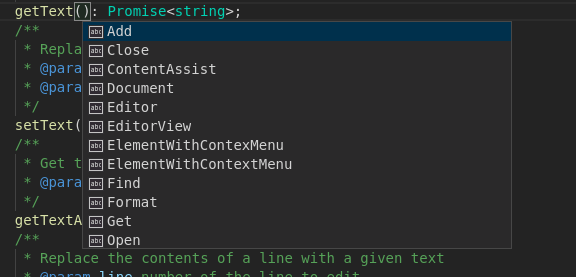 | ||
|
||
#### Open/Lookup | ||
```typescript | ||
import { TextEditor, ContentAssist } from 'vscode-extension-tester'; | ||
... | ||
const contentAssist = await new TextEditor().toggleContentAssist(true); | ||
``` | ||
|
||
#### Get Items | ||
```typescript | ||
// find if an item with given label is present | ||
const hasItem = await contentAssist.hasItem('Get'); | ||
// get an item by label | ||
const item = await contentAssist.getItem('Get'); | ||
// get all visible items | ||
const items = await contentAssist.getItems(); | ||
``` | ||
|
||
#### Select an Item | ||
```typescript | ||
await contentAssist.getItem('Get').click(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
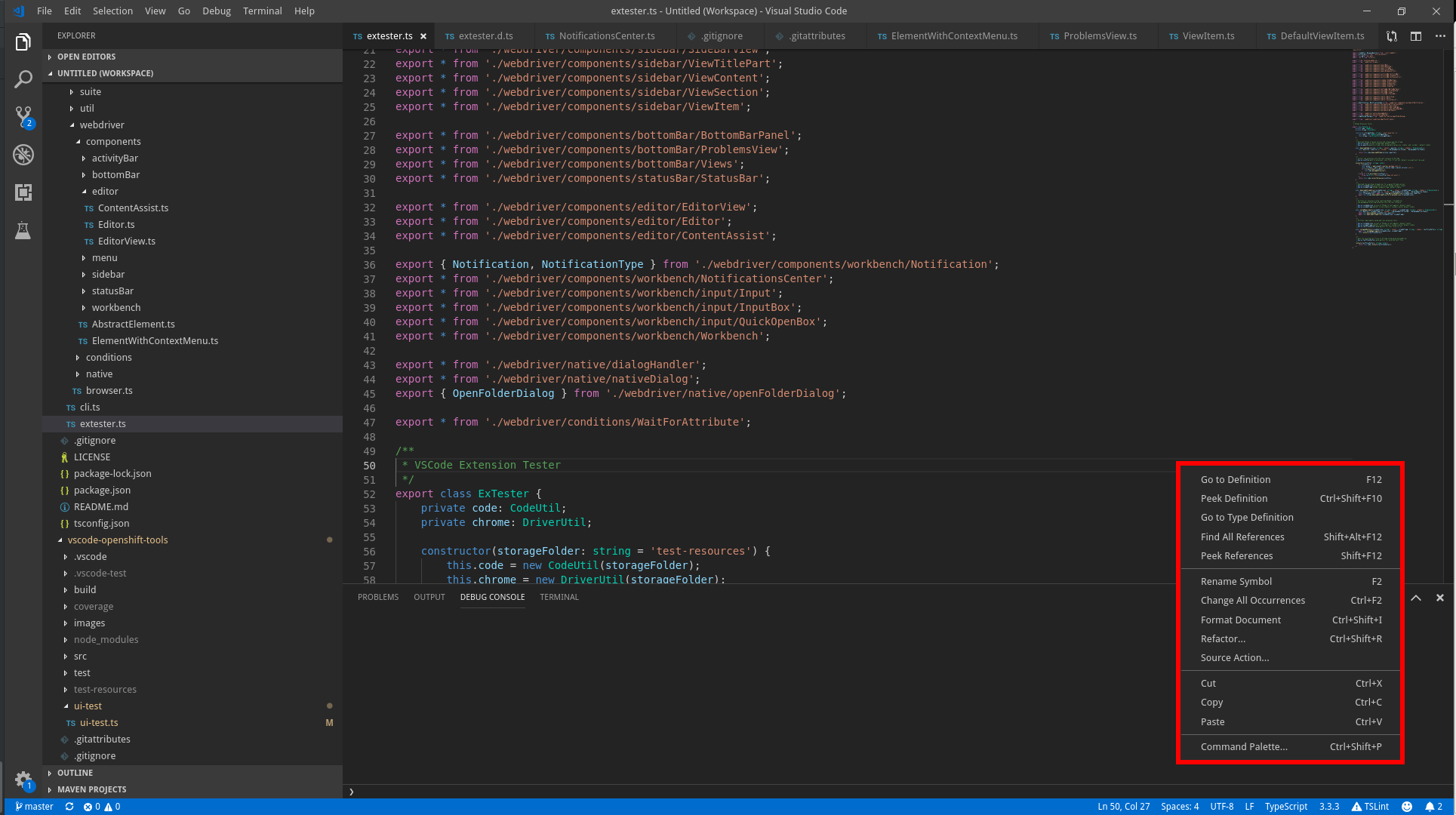 | ||
Page object for any context menu opened by left-clicking an element that has a context menu. Title bar items also produce context menus when clicked. | ||
|
||
#### Open/Lookup | ||
Typically, a context menu is opened by calling ```openContextMenu``` on elements that support it. For example: | ||
```typescript | ||
import { ActivityBar, ContextMenu } from 'vscode-extension-tester'; | ||
... | ||
const menu = await new ActivityBar().openContextMenu(); | ||
``` | ||
|
||
#### Retrieve Items | ||
```typescript | ||
// find if an item with title exists | ||
const exists = await menu.hasItem('Copy'); | ||
// get a handle for an item | ||
const item = await menu.getItem('Copy'); | ||
// get all displayed items | ||
const items = await menu.getItems(); | ||
``` | ||
|
||
#### Select Item | ||
```typescript | ||
// recursively select an item in nested submenus | ||
await menu.select('File', 'Preferences', 'Settings'); | ||
// select an item that has a child submenu | ||
const submenu = await menu.select('File', 'Preferences'); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
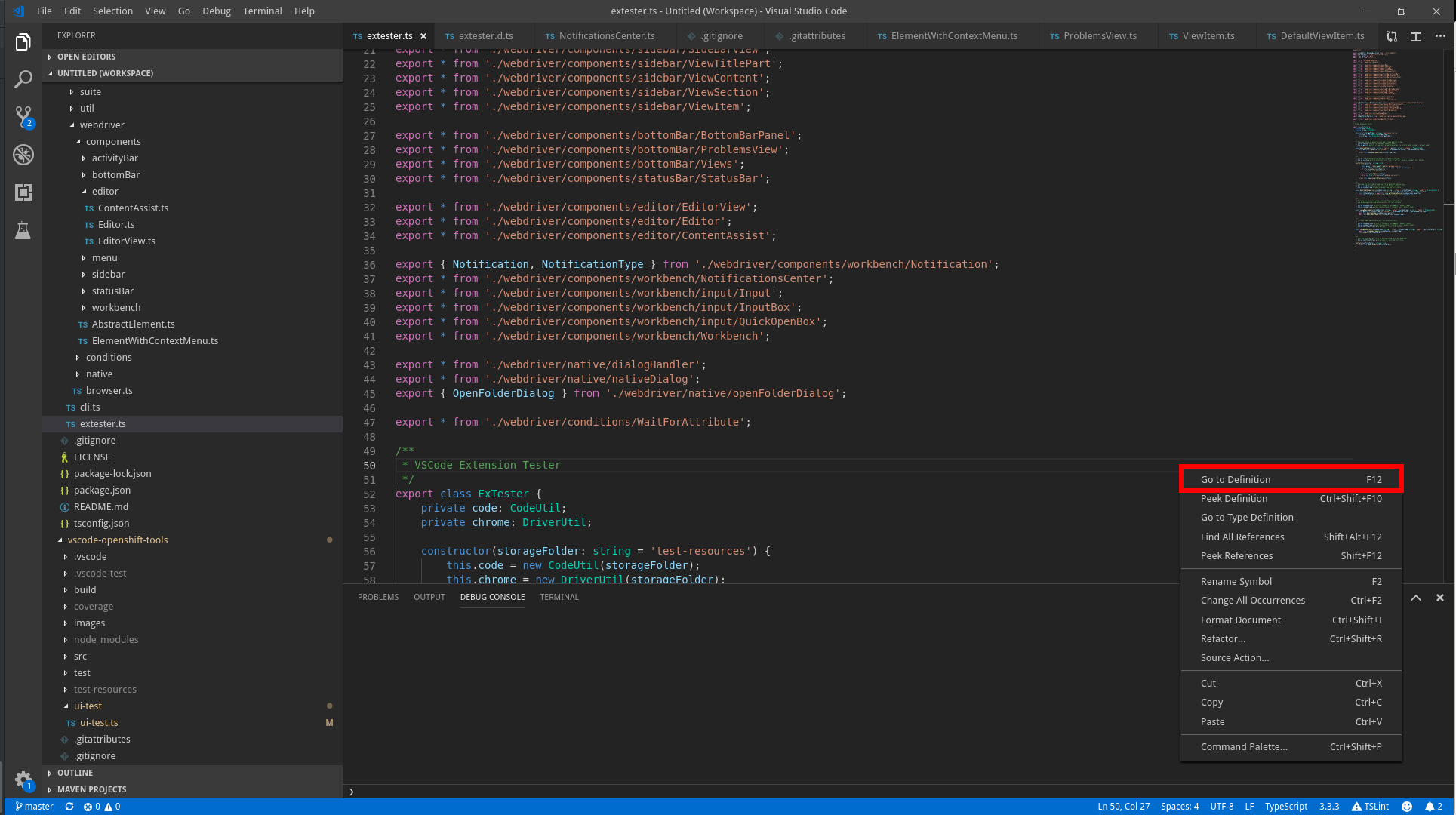 | ||
|
||
#### Lookup | ||
One can retrieve an item from an open context menu, much like follows: | ||
```typescript | ||
import { ActivityBar } from 'vscode-extension-tester'; | ||
... | ||
const menu = await new ActivityBar().openContextMenu(); | ||
const item = await menu.getItem('References'); | ||
``` | ||
|
||
#### Select/Click | ||
```typescript | ||
// if item has no children | ||
await item.select(); | ||
// if there is a submenu under the item | ||
const submenu = await item.select(); | ||
``` | ||
|
||
#### Get Parent Menu | ||
```typescript | ||
const parentMenu = item.getParent(); | ||
``` | ||
|
||
#### Get Label | ||
```typescript | ||
const label = await item.getLabel(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
In case your extension contributes a `CustomEditor`/`CustomTextEditor`. Both are based on a webview, and the page object is a combination of a `Webview` and common editor functionality. | ||
|
||
#### Lookup | ||
```typescript | ||
import { CustomEditor } from 'vscode-extension-tester' | ||
... | ||
// make sure the editor is opened by now | ||
const editor = new CustomEditor(); | ||
``` | ||
|
||
#### Webview | ||
The whole editor is serviced by a [[WebView]], we just need to get a reference to it. | ||
```typescript | ||
const webview = editor.getWebView(); | ||
``` | ||
|
||
#### Common Functionality | ||
Most editors share this: | ||
```typescript | ||
// check if there are unsaved changes | ||
const dirty = await editor.isDirty(); | ||
// save | ||
await editor.save(); | ||
// open 'save as' prompt | ||
const prompt = await editor.saveAs(); | ||
// get title | ||
const title = await editor.getTitle(); | ||
// get the editor tab | ||
const tab = await editor.getTab(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
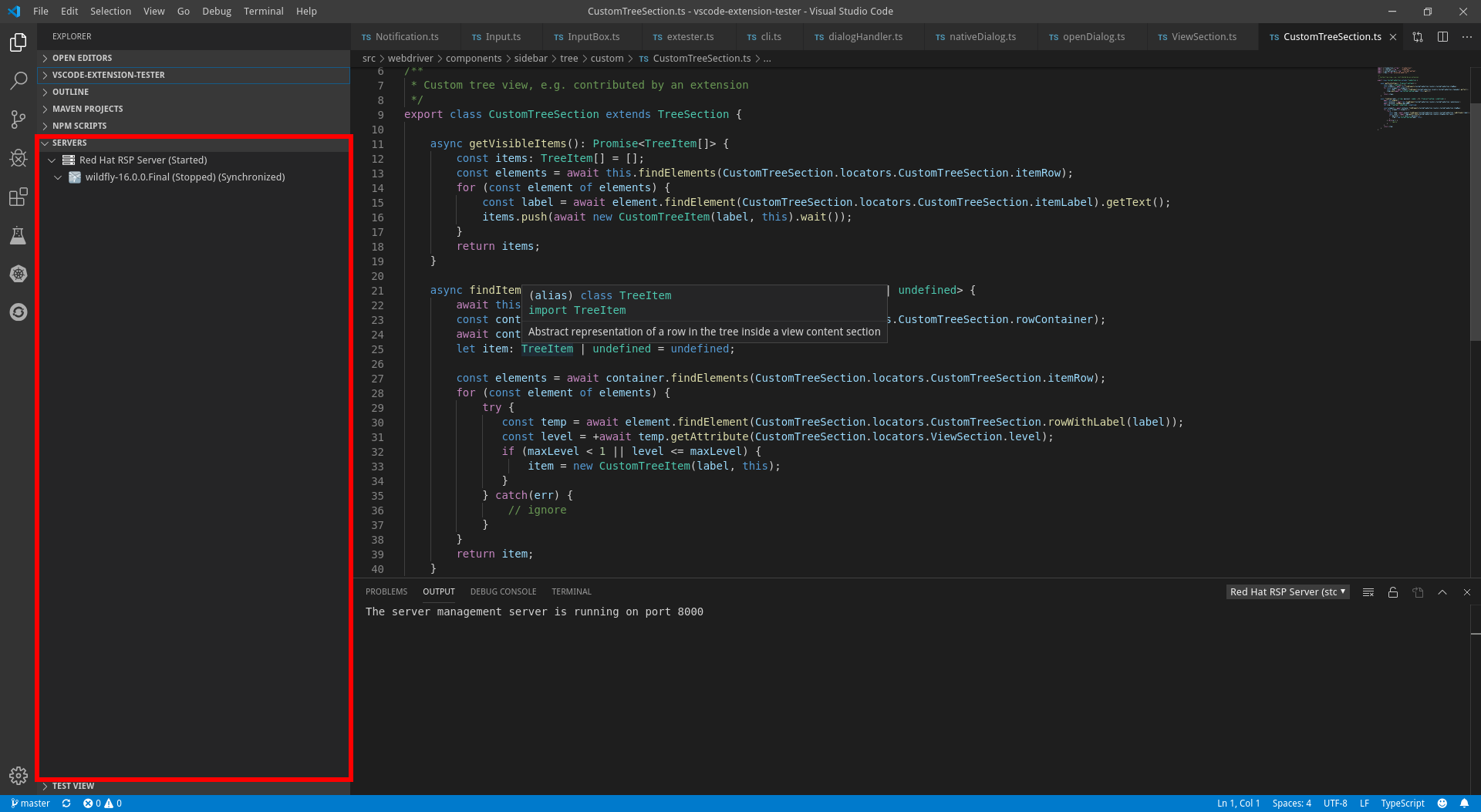 | ||
|
||
The 'custom' tree section, usually contributed by extensions as TreeView. All The behaviour is defined by the general [[ViewSection]] class. | ||
|
||
#### Lookup | ||
```typescript | ||
import { SideBarView, CustomTreeSection } from 'vscode-extension-tester'; | ||
... | ||
// Type is inferred automatically, the type cast here is used to be more explicit | ||
const section = await new SideBarView().getContent().getSection('servers') as CustomTreeSection; | ||
``` | ||
|
||
#### Get Welcome Content | ||
Some sections may provide a welcome content when their tree is empty. | ||
```typescript | ||
// find welcome content, return undefined if not present | ||
const welcome: WelcomeContentSection = await section.findWelcomeContent(); | ||
|
||
// get all the possible buttons and paragraphs in a list | ||
const contents = await welcome.getContents(); | ||
|
||
// get all buttons | ||
const btns = await welcome.getButtons(); | ||
|
||
// get paragraphs as strings in a list | ||
const text = await welcome.getTextSections(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,28 @@ | ||
Page object needs extending, currently minimal support. | ||
|
||
#### Lookup | ||
```typescript | ||
import { BottomBarPanel, DebugConsoleView } from 'vscode-extension-tester'; | ||
... | ||
const debugView = await new BottomBarPanel().openDebugConsoleView(); | ||
``` | ||
|
||
#### Text Handling | ||
```typescript | ||
// get all text as string | ||
const text = await debugView.getText(); | ||
// clear the text | ||
await debugView.clearText(); | ||
``` | ||
|
||
#### Expressions | ||
```typescript | ||
// type an expression | ||
await debugView.setExpression('expression'); | ||
// evaluate an existing expression | ||
await debugView.evaluateExpression(); | ||
// type and evaluate an expression | ||
await debugView.evaluateExpression('expression'); | ||
// get a handle for content assist | ||
const assist = await debugView.getContentAssist(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
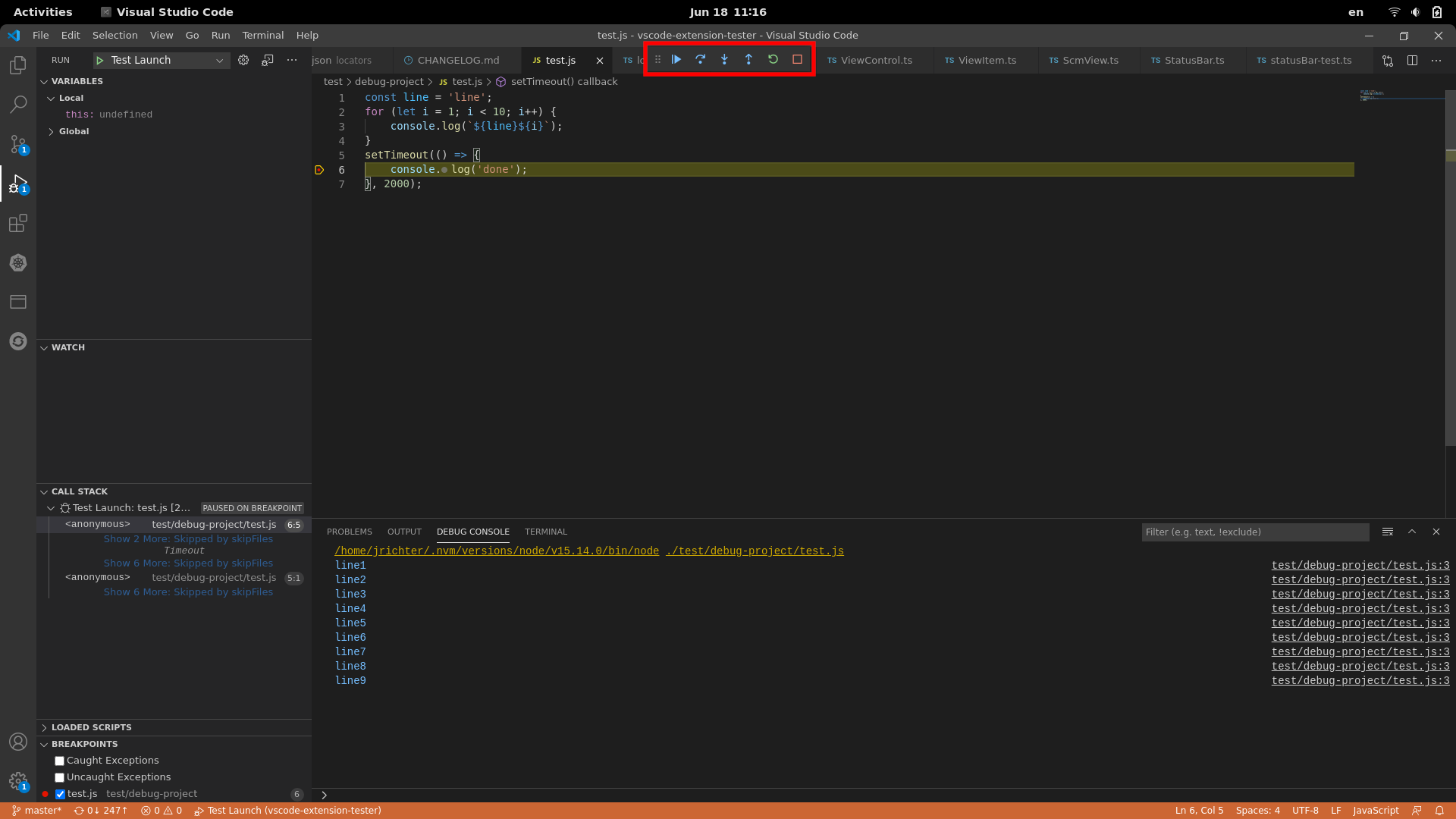 | ||
|
||
#### Lookup | ||
```typescript | ||
// get a handle for existing toolbar (i.e. debug session needs to be in progress) | ||
const bar = await DebugToolbar.create(); | ||
``` | ||
|
||
#### Buttons | ||
```typescript | ||
// continue | ||
await bar.continue(); | ||
// pause | ||
await bar.pause(); | ||
// step over | ||
await bar.stepOver(); | ||
// step into | ||
await bar.stepInto(); | ||
// step out | ||
await bar.stepOut(); | ||
// restart | ||
await bar.restart(); | ||
// stop | ||
await bar.stop(); | ||
``` | ||
|
||
#### Wait for code to pause again | ||
```typescript | ||
await bar.waitForBreakPoint(); | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
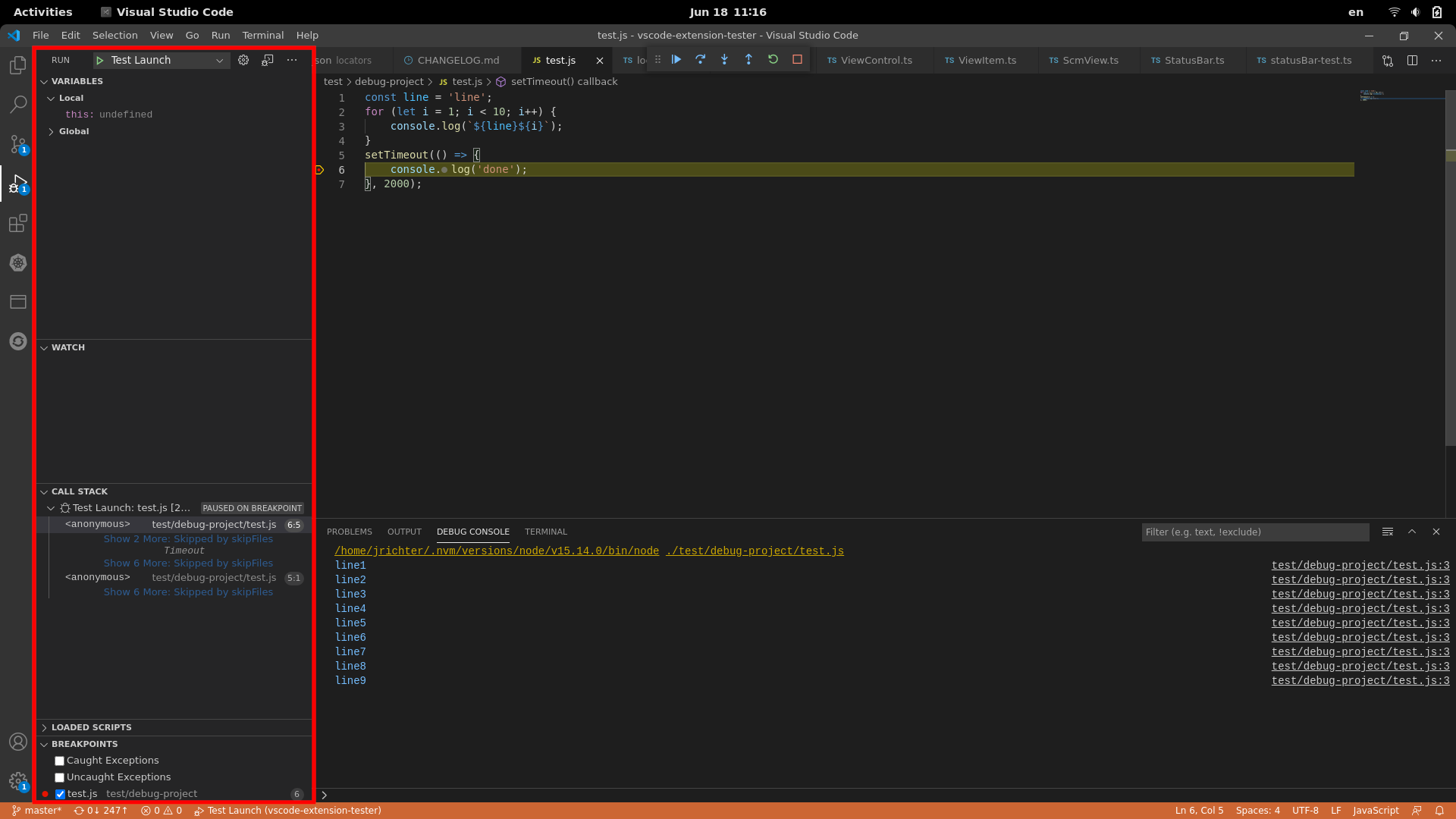 | ||
|
||
#### Lookup | ||
```typescript | ||
// open the view using the icon in the view container | ||
const btn = await new ActivityBar().getViewControl('Run'); | ||
const debugView = (await btn.openView()) as DebugView; | ||
``` | ||
|
||
#### Launch Configurations | ||
```typescript | ||
// get title of current launch configuration | ||
const config = await debugView.getLaunchConfiguration(); | ||
// get titles of all available laynch configurations | ||
const configs = await debugView.getLaunchConfigurations(); | ||
// select launch configuration by title | ||
await debugConfiguration.selectLaunchConfiguration('Test Launch'); | ||
``` | ||
|
||
#### Launch | ||
```typescript | ||
// start selected launch configuration | ||
await debugView.start(); | ||
``` |
Oops, something went wrong.