-
Notifications
You must be signed in to change notification settings - Fork 88
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
dengwen49
committed
Oct 14, 2019
1 parent
469f5c1
commit 7520eb0
Showing
7 changed files
with
778 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,143 @@ | ||
|
||
|
||
|
||
#### [中文版文档](https://github.com/AwesomeDevin/vue-waterfall2/blob/master/CHINESE-README.md) | ||
# vue-waterfall2 | ||
1. auto adaption for width and height | ||
2. High degree of customization | ||
3. support lazy load(attribute with `lazy-src`) | ||
|
||
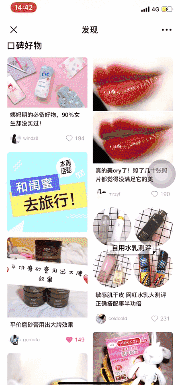 | ||
|
||
|
||
If you have some questions,welcome to describe issues、suggestions;Thank you for your Star ! | ||
[Welcome to my blog !!!](https://github.com/AwesomeDevin/blog) | ||
|
||
|
||
## Demo | ||
[Common Demo](http://47.105.188.15:3001/) | ||
[Lazyload Demo](http://47.105.188.15:3001/#/list) | ||
[Code Demo](https://codesandbox.io/embed/vue-template-99ps6) | ||
|
||
|
||
|
||
|
||
|
||
[GITHUB](https://github.com/Rise-Devin/vue-waterfall2) | ||
``` | ||
npm i | ||
npm run dev | ||
``` | ||
|
||
## Installation | ||
``` | ||
npm i vue-waterfall2@latest --save | ||
``` | ||
|
||
## <waterfall> Props | ||
Name | Default | Type | Desc | ||
-------- | -------- | -------- | -------- | ||
height | null | Number | height of container | ||
col | 2 | Number | The number of column | ||
width | null | Number | The width of each column | ||
gutterWidth | 10 | Number | The value of margin | ||
data | [] | Array | data | ||
isTransition | true | Boolean | load image with transition | ||
lazyDistance | 300 | Number | The distance of image lazy loading | ||
loadDistance | 300 | Number | The distance of loadmore | ||
|
||
## Lazy Load | ||
For images that need to be loaded lazily, the 'lazy-src' attribute needs to be used | ||
```html | ||
<waterfall :col='col' :data="data" > | ||
<template> | ||
<img v-if="item.img" :lazy-src="item.img" alt="load error" /> | ||
</template> | ||
</waterfall> | ||
``` | ||
|
||
## <waterfall> Events | ||
Name | Data | Desc | ||
-------- | --- | -------- | ||
loadmore | - | Scroll to the bottom to trigger on PC / pull up to trigger on Mobile | ||
scroll | obj | Scroll to trigger and get the infomation of scroll | ||
finish | - | finish render | ||
|
||
## $waterfall API | ||
``` | ||
this.$waterfall.forceUpdate() //forceUpdate | ||
``` | ||
|
||
## Usage | ||
Notes: | ||
1. attribute `gutterWidth` needs to be used together with `width` to be effective, otherwise it will be adaptive width (when using `rem` to layout, calculate the width after adaptation before passing the value). | ||
2. Use the parent component of 'waterfall' if there is a problem with the style, remove CSS `scoped` and try it | ||
##### main.js | ||
```javascript | ||
import waterfall from 'vue-waterfall2' | ||
Vue.use(waterfall) | ||
``` | ||
##### app.vue | ||
```javascript | ||
<template> | ||
<div class="container-water-fall"> | ||
<div><button @click="loadmore">loadmore</button> <button @click="mix">mix</button> <button @click="switchCol('5')">5列</button> <button @click="switchCol('8')">8列</button> <button @click="switchCol('10')">10列</button> </div> | ||
|
||
<waterfall :col='col' :width="itemWidth" :gutterWidth="gutterWidth" :data="data" @loadmore="loadmore" @scroll="scroll" > | ||
<template > | ||
<div class="cell-item" v-for="(item,index) in data"> | ||
<div class="item-body"> | ||
<div class="item-desc">{{item.title}}</div> | ||
<div class="item-footer"> | ||
<div class="avatar" :style="{backgroundImage : `url(${item.avatar})` }"></div> | ||
<div class="name">{{item.user}}</div> | ||
<div class="like" :class="item.liked?'active':''" > | ||
<i ></i> | ||
<div class="like-total">{{item.liked}}</div> | ||
</div> | ||
</div> | ||
</div> | ||
</div> | ||
</template> | ||
</waterfall> | ||
|
||
</div> | ||
</template> | ||
|
||
|
||
/* | ||
notes: | ||
1. attribute `gutterWidth` needs to be used together with `width` to be effective, otherwise it will be adaptive width (when using `rem` to layout, calculate the width after adaptation before passing the value). | ||
2. Use the parent component of 'waterfall' if there is a problem with the style, remove CSS 'scoped' and try it | ||
*/ | ||
|
||
import Vue from 'vue' | ||
export default{ | ||
data(){ | ||
return{ | ||
data:[], | ||
col:5, | ||
} | ||
}, | ||
computed:{ | ||
itemWidth(){ | ||
return (138*0.5*(document.documentElement.clientWidth/375)) #rem to layout, Calculate the value of width | ||
}, | ||
gutterWidth(){ | ||
return (9*0.5*(document.documentElement.clientWidth/375)) #rem to layout, Calculate the value of margin | ||
} | ||
}, | ||
methods:{ | ||
scroll(scrollData){ | ||
console.log(scrollData) | ||
}, | ||
switchCol(col){ | ||
this.col = col | ||
console.log(this.col) | ||
}, | ||
loadmore(index){ | ||
this.data = this.data.concat(this.data) | ||
} | ||
} | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
'use strict'; | ||
|
||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
|
||
var _vue = require('vue'); | ||
|
||
var _vue2 = _interopRequireDefault(_vue); | ||
|
||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
|
||
var Bus = new _vue2.default(); | ||
exports.default = Bus; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
'use strict'; | ||
|
||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
|
||
var _vue = require('vue'); | ||
|
||
var _vue2 = _interopRequireDefault(_vue); | ||
|
||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
|
||
var Bus = new _vue2.default(); | ||
exports.default = Bus; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
'use strict'; | ||
|
||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
|
||
var _bus = require('./bus.js'); | ||
|
||
var _bus2 = _interopRequireDefault(_bus); | ||
|
||
var _waterfall = require('./waterfall.vue'); | ||
|
||
var _waterfall2 = _interopRequireDefault(_waterfall); | ||
|
||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
|
||
var Index = { | ||
install: function install(Vue) { | ||
if (this.installed) { | ||
return; | ||
} | ||
this.installed = true; | ||
Vue.component('waterfall', _waterfall2.default); | ||
Vue.prototype.$waterfall = { | ||
resize: function resize() { | ||
_bus2.default.$emit('resize'); | ||
}, | ||
mix: function mix() { | ||
_bus2.default.$emit('mix'); | ||
} | ||
}; | ||
} | ||
}; | ||
exports.default = Index; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
'use strict'; | ||
|
||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
|
||
var _bus = require('./bus.js'); | ||
|
||
var _bus2 = _interopRequireDefault(_bus); | ||
|
||
var _waterfall = require('./waterfall.vue'); | ||
|
||
var _waterfall2 = _interopRequireDefault(_waterfall); | ||
|
||
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; } | ||
|
||
var Index = { | ||
install: function install(Vue) { | ||
if (this.installed) { | ||
return; | ||
} | ||
this.installed = true; | ||
Vue.component('waterfall', _waterfall2.default); | ||
Vue.prototype.$waterfall = { | ||
forceUpdate: function forceUpdate() { | ||
_bus2.default.$emit('forceUpdate'); | ||
}, | ||
mix: function mix() { | ||
_bus2.default.$emit('mix'); | ||
} | ||
}; | ||
} | ||
}; | ||
exports.default = Index; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,66 @@ | ||
{ | ||
"_args": [ | ||
[ | ||
"[email protected]", | ||
"D:\\projects\\act\\proj\\2018\\1112_findv2" | ||
] | ||
], | ||
"_from": "[email protected]", | ||
"_id": "[email protected]", | ||
"_inBundle": false, | ||
"_integrity": "sha512-fMJq9LJwb6/0AciO7IqQNZb+fEkzpkyzOhugT+POndGAEGWpallLw6yDc0ZfKJ/AmStD+gPyvSLv8UY4KBnfEg==", | ||
"_location": "/vue-waterfall2", | ||
"_phantomChildren": {}, | ||
"_requested": { | ||
"type": "range", | ||
"registry": true, | ||
"raw": "vue-waterfall2@^1.9.1", | ||
"name": "vue-waterfall2", | ||
"escapedName": "vue-waterfall2", | ||
"rawSpec": "^1.9.1", | ||
"saveSpec": null, | ||
"fetchSpec": "^1.9.1" | ||
}, | ||
"_requiredBy": [ | ||
"#DEV:/" | ||
], | ||
"_resolved": "https://registry.npm.taobao.org/vue-waterfall2/download/vue-waterfall2-1.9.1.tgz", | ||
"_shasum": "0793f2abead08383d15e072880017858cbdf3223", | ||
"_spec": "vue-waterfall2@^1.9.1", | ||
"_where": "E:\\projects\\work\\vue-waterfall", | ||
"author": { | ||
"name": "[email protected]" | ||
}, | ||
"bugs": { | ||
"url": "https://github.com/Rise-Devin/vue-waterfall2/issues" | ||
}, | ||
"bundleDependencies": false, | ||
"dependencies": { | ||
"babel-runtime": "^6.26.0", | ||
"regenerator-runtime": "^0.13.1" | ||
}, | ||
"deprecated": false, | ||
"description": "vue plugin for waterfall", | ||
"devDependencies": { | ||
"babel-polyfill": "^6.26.0", | ||
"babel-preset-es2015": "^6.24.1", | ||
"vue": "^2.5.2" | ||
}, | ||
"homepage": "https://github.com/Rise-Devin/vue-waterfall2#readme", | ||
"keywords": [ | ||
"waterfall", | ||
"vue" | ||
], | ||
"license": "MIT", | ||
"main": "index.js", | ||
"name": "vue-waterfall2", | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/Rise-Devin/vue-waterfall2.git" | ||
}, | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1" | ||
}, | ||
"version": "1.9.1", | ||
"__npminstall_done": "Mon Oct 14 2019 20:59:33 GMT+0800 (GMT+08:00)" | ||
} |
Oops, something went wrong.