diff --git a/docs/company/index-company.mdx b/docs/company/index-company.mdx
index bab60a4..66bd2a8 100644
--- a/docs/company/index-company.mdx
+++ b/docs/company/index-company.mdx
@@ -5,14 +5,14 @@ sidebar_label: Overview
slug: /company
---
-import RedisCard from '@theme/RedisCard';
+import ThreeTenCard from '@theme/ThreeTenCard';
Find documentation, sample code and tools to develop with your favorite language.
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
diff --git a/docs/games/guacamole/nodejs-nginx-redis/docker_nginx.png b/docs/games/guacamole/nodejs-nginx-redis/docker_nginx.png
deleted file mode 100644
index ba4d86d..0000000
Binary files a/docs/games/guacamole/nodejs-nginx-redis/docker_nginx.png and /dev/null differ
diff --git a/docs/games/guacamole/nodejs-nginx-redis/index-nodejs-nginx-redis.mdx b/docs/games/guacamole/nodejs-nginx-redis/index-nodejs-nginx-redis.mdx
deleted file mode 100644
index 61b34db..0000000
--- a/docs/games/guacamole/nodejs-nginx-redis/index-nodejs-nginx-redis.mdx
+++ /dev/null
@@ -1,336 +0,0 @@
----
-id: index-nodejs-nginx-redis
-title: How to build and run a Node.js application using Nginx, Docker and Redis
-sidebar_label: Node.js, Nginx, Redis and Docker
-slug: /create/docker/nodejs-nginx-redis
-authors: [ajeet]
----
-
-Thanks to [Node.js](https://nodejs.dev/) - Millions of frontend developers that write JavaScript for the browser are now able to write the server-side code in addition to the client-side code without the need to learn a completely different language. Node.js is a free, open-sourced, cross-platform JavaScript run-time environment. It is capable to handle thousands of concurrent connections with a single server without introducing the burden of managing thread concurrency, which could be a significant source of bugs.
-
-
-
-In this quickstart guide, you will see how to build a Node.js application (visitor counter) using Nginx, Redis and Docker.
-
-### What do you need?
-
-- **Node.js**: An open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine and executes JavaScript code outside a web browser.
-- **Nginx**: An open source software for web serving, reverse proxying, caching, load balancing, media streaming, and more.
-- **Docker**: a containerization platform for developing, shipping, and running applications.
-- **Docker Compose**: A tool for defining and running multi-container Docker applications.
-
-### Project structure
-
-```
-.
-├── docker-compose.yml
-├── redis
-├── nginx
-│ ├── Dockerfile
-│ └── nginx.conf
-├── web1
-│ ├── Dockerfile
-│ ├── package.json
-│ └── server.js
-└── web2
- ├── Dockerfile
- ├── package.json
- └── server.js
-
-```
-
-### Prerequisite:
-
-– Install Docker Desktop
-
-Visit https://docs.docker.com/desktop/mac/install/ to setup Docker Desktop for Mac or Windows on your local system.
-
-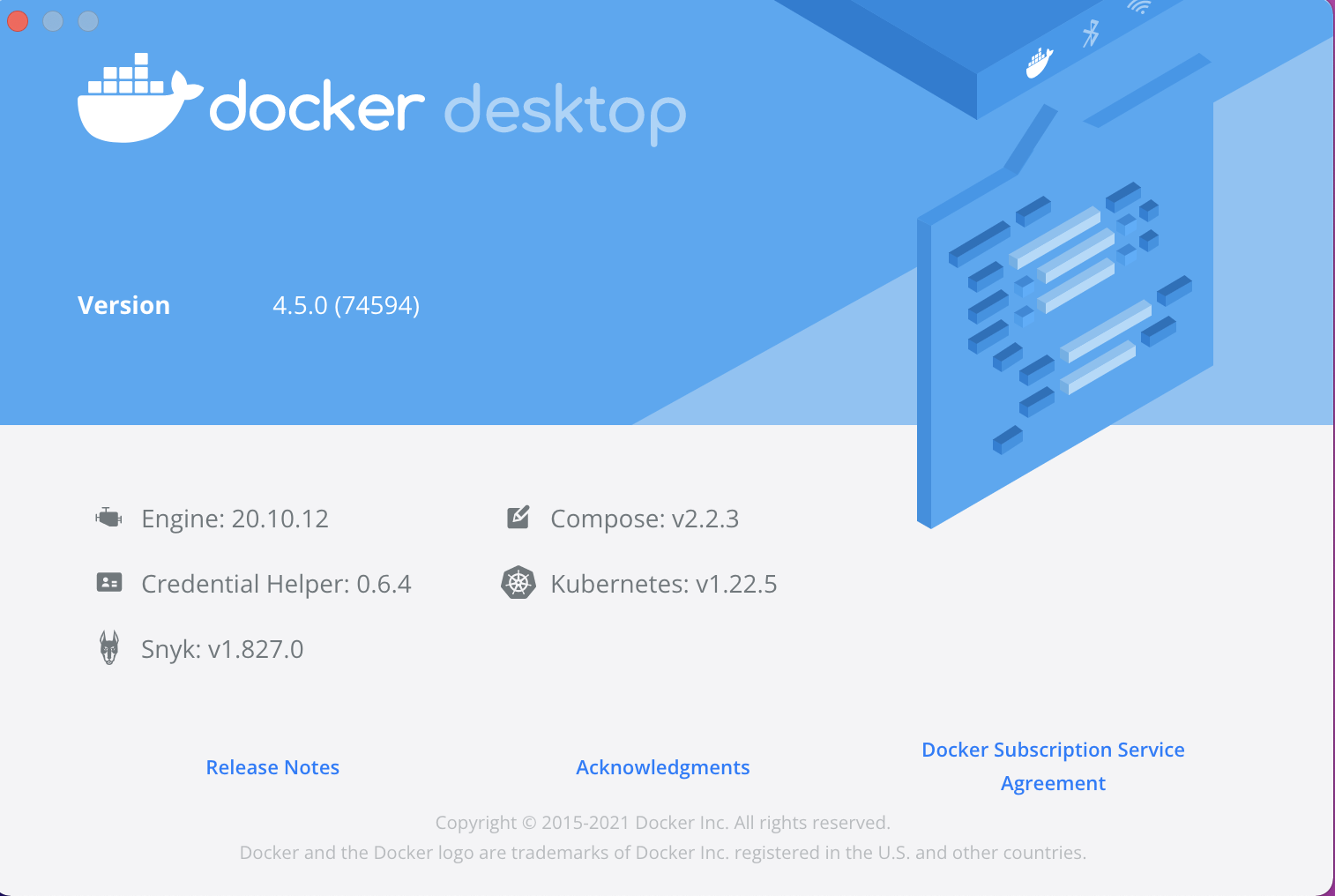
-
-:::info
-
-Docker Desktop comes with Docker compose installed by default, hence you don't need to install it separately.
-
-:::
-
-### Step 1. Create a Docker compose file
-
-Create an empty file with the below content and save it by name - "docker-compose.yml"
-
-```
-version: '3.9'
-services:
- redis:
- image: 'redis:alpine'
- ports:
- - '6379:6379'
- web1:
- restart: on-failure
- build: ./web1
- ports:
- - '81:5000'
- web2:
- restart: on-failure
- build: ./web2
- ports:
- - '82:5000'
- nginx:
- build: ./nginx
- ports:
- - '80:80'
- depends_on:
- - web1
- - web2
-```
-
-The compose file defines an application with four services `redis`, `web1`, `web2` and `nginx`.
-When deploying the application, docker-compose maps port 80 of the web service container to port 80 of the host as specified in the file.
-
-:::info
-
-By default, Redis runs on port 6379. Make sure you don't run another instance of Redis on your system or port 6379 on the host is not being used by another container, otherwise the port should be changed.
-:::
-
-### Step 2. Create an nginx directory and add the below files:
-
-#### File: nginx/nginx.conf
-
-```
-upstream loadbalancer {
- server web1:5000;
- server web2:5000;
-}
-
-server {
- listen 80;
- server_name localhost;
- location / {
- proxy_pass http://loadbalancer;
- }
-}
-```
-
-#### File: Dockerfile
-
-```
-FROM nginx
-RUN rm /etc/nginx/conf.d/default.conf
-COPY nginx.conf /etc/nginx/conf.d/default.conf
-```
-
-### Step 3. Create a web directory and add the below files:
-
-#### File: web/Dockerfile
-
-```
-FROM node:alpine
-
-WORKDIR /usr/src/app
-
-COPY ./package.json ./
-RUN npm install
-COPY ./server.js ./
-
-CMD ["npm","start"]
-```
-
-#### File: web/package.json
-
-```
-
- "name": "web",
- "version": "1.0.0",
- "description": "Running Node.js and Express.js on Docker",
- "main": "server.js",
- "scripts": {
- "start": "node server.js"
- },
- "dependencies": {
- "express": "^4.17.2",
- "redis": "3.1.2"
- },
- "author": "",
- "license": "MIT"
-}
-```
-
-#### File: web/server.js
-
-```
-const express = require('express');
-const redis = require('redis');
-const app = express();
-const redisClient = redis.createClient({
- host: 'redis',
- port: 6379
-});
-
-app.get('/', function(req, res) {
- redisClient.get('numVisits', function(err, numVisits) {
- numVisitsToDisplay = parseInt(numVisits) + 1;
- if (isNaN(numVisitsToDisplay)) {
- numVisitsToDisplay = 1;
- }
- res.send('Number of visits is: ' + numVisitsToDisplay);
- numVisits++;
- redisClient.set('numVisits', numVisits);
- });
-});
-
-app.listen(5000, function() {
- console.log('Web application is listening on port 5000');
-});
-```
-
-### Step 4. Creating a web1 directory and add the below files:
-
-#### File: Dockerfile
-
-```
-FROM node:alpine
-
-WORKDIR /usr/src/app
-
-COPY ./package*.json ./
-RUN npm install
-COPY ./server.js ./
-
-CMD ["npm","start"]
-
-```
-
-#### File: server.js
-
-```
-const express = require('express');
-const redis = require('redis');
-const app = express();
-const redisClient = redis.createClient({
- host: 'redis',
- port: 6379
-});
-
-
-app.get('/', function(req, res) {
- redisClient.get('numVisits', function(err, numVisits) {
- numVisitsToDisplay = parseInt(numVisits) + 1;
- if (isNaN(numVisitsToDisplay)) {
- numVisitsToDisplay = 1;
- }
- res.send('web1: Total number of visits is: ' + numVisitsToDisplay);
- numVisits++;
- redisClient.set('numVisits', numVisits);
- });
-});
-
-app.listen(5000, function() {
- console.log('Web app is listening on port 5000');
-});
-```
-
-#### File: package.json
-
-```
-{
- "name": "web1",
- "version": "1.0.0",
- "description": "Running Node.js and Express.js on Docker",
- "main": "server.js",
- "scripts": {
- "start": "node server.js"
- },
- "dependencies": {
- "express": "^4.17.2",
- "redis": "3.1.2"
- },
- "author": "",
- "license": "MIT"
-}
-```
-
-### Step 5. Deploy the application
-
-Let us deploy the full-fledged app using docker-compose
-
-```
-$ docker-compose up -d
-```
-
-```
-Creating nginx-nodejs-redis_redis_1 ... done
-Creating nginx-nodejs-redis_web1_1 ... done
-Creating nginx-nodejs-redis_web2_1 ... done
-Creating nginx-nodejs-redis_nginx_1 ... done
-```
-
-#### Expected result
-
-Listing containers must show three containers running and the port mapping as below:
-
-```
-docker-compose ps
- Name Command State Ports
-------------------------------------------------------------------------------------------
-nginx-nodejs-redis_nginx_1 /docker-entrypoint.sh ngin Up 0.0.0.0:80->80/tcp
- ...
-nginx-nodejs-redis_redis_1 docker-entrypoint.sh redis Up 0.0.0.0:6379->6379/tcp
- ...
-nginx-nodejs-redis_web1_1 docker-entrypoint.sh npm Up 0.0.0.0:81->5000/tcp
- start
-nginx-nodejs-redis_web2_1 docker-entrypoint.sh npm Up 0.0.0.0:82->5000/tcp
- start
-```
-
-### Step 6. Testing the app
-
-After the application starts, navigate to `http://localhost:80` in your web browser or run:
-
-```
-curl localhost:80
-curl localhost:80
-web1: Total number of visits is: 1
-```
-
-```
-curl localhost:80
-web1: Total number of visits is: 2
-```
-
-```
-$ curl localhost:80
-web2: Total number of visits is: 3
-```
-
-```
-$ curl localhost:80
-web2: Total number of visits is: 4
-```
-
-### Step 7. Monitoring Redis keys
-
-If you want to monitor the Redis keys, you can use monitor command. Install redis-client in your Mac system using `brew install redis` and then directly connect to Redis container by issuing the below command:
-
-```
-% redis-cli
-127.0.0.1:6379> monitor
-OK
-1646485507.290868 [0 172.24.0.2:34330] "get" "numVisits"
-1646485507.309070 [0 172.24.0.2:34330] "set" "numVisits" "5"
-1646485509.228084 [0 172.24.0.2:34330] "get" "numVisits"
-1646485509.241762 [0 172.24.0.2:34330] "set" "numVisits" "6"
-1646485509.619369 [0 172.24.0.4:52082] "get" "numVisits"
-1646485509.629739 [0 172.24.0.4:52082] "set" "numVisits" "7"
-1646485509.990926 [0 172.24.0.2:34330] "get" "numVisits"
-1646485509.999947 [0 172.24.0.2:34330] "set" "numVisits" "8"
-1646485510.270934 [0 172.24.0.4:52082] "get" "numVisits"
-1646485510.286785 [0 172.24.0.4:52082] "set" "numVisits" "9"
-1646485510.469613 [0 172.24.0.2:34330] "get" "numVisits"
-1646485510.480849 [0 172.24.0.2:34330] "set" "numVisits" "10"
-1646485510.622615 [0 172.24.0.4:52082] "get" "numVisits"
-1646485510.632720 [0 172.24.0.4:52082] "set" "numVisits" "11"
-```
-
-### Further References
-
-- [ Complete Source Code](https://github.com/ajeetraina/awesome-compose/tree/master/nginx-nodejs-redis)
-- [How to Deploy and Run Redis in a Docker container](https://developer.redis.com/create/docker/)
diff --git a/docs/games/images/activate.png b/docs/games/images/activate.png
deleted file mode 100644
index b871b07..0000000
Binary files a/docs/games/images/activate.png and /dev/null differ
diff --git a/docs/games/images/aws.png b/docs/games/images/aws.png
deleted file mode 100644
index 5d49974..0000000
Binary files a/docs/games/images/aws.png and /dev/null differ
diff --git a/docs/games/images/choosemodule.png b/docs/games/images/choosemodule.png
deleted file mode 100644
index ba5165d..0000000
Binary files a/docs/games/images/choosemodule.png and /dev/null differ
diff --git a/docs/games/images/createdatabase.png b/docs/games/images/createdatabase.png
deleted file mode 100644
index a4415e9..0000000
Binary files a/docs/games/images/createdatabase.png and /dev/null differ
diff --git a/docs/games/images/plan.png b/docs/games/images/plan.png
deleted file mode 100644
index d481c75..0000000
Binary files a/docs/games/images/plan.png and /dev/null differ
diff --git a/docs/games/images/region.png b/docs/games/images/region.png
deleted file mode 100644
index ddbe75e..0000000
Binary files a/docs/games/images/region.png and /dev/null differ
diff --git a/docs/games/images/subscription.png b/docs/games/images/subscription.png
deleted file mode 100644
index b4a6134..0000000
Binary files a/docs/games/images/subscription.png and /dev/null differ
diff --git a/docs/games/index-games.mdx b/docs/games/index-games.mdx
index a21b12d..cb52feb 100644
--- a/docs/games/index-games.mdx
+++ b/docs/games/index-games.mdx
@@ -5,63 +5,19 @@ sidebar_label: Overview - All Quick Starts
slug: /games
---
-import RedisCard from '@theme/RedisCard';
+import ThreeTenCard from '@theme/ThreeTenCard';
The following quick starts shows various ways of how to get started and create a new Redis database:
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
diff --git a/docs/games/spider/analytics-using-aws/analytics3.png b/docs/games/spider/analytics-using-aws/analytics3.png
deleted file mode 100644
index 9d48729..0000000
Binary files a/docs/games/spider/analytics-using-aws/analytics3.png and /dev/null differ
diff --git a/docs/games/spider/analytics-using-aws/analytics4.png b/docs/games/spider/analytics-using-aws/analytics4.png
deleted file mode 100644
index cf3dbb1..0000000
Binary files a/docs/games/spider/analytics-using-aws/analytics4.png and /dev/null differ
diff --git a/docs/games/spider/analytics-using-aws/analytics_dashboard.png b/docs/games/spider/analytics-using-aws/analytics_dashboard.png
deleted file mode 100644
index 76774c0..0000000
Binary files a/docs/games/spider/analytics-using-aws/analytics_dashboard.png and /dev/null differ
diff --git a/docs/games/spider/analytics-using-aws/analytics_traffic.png b/docs/games/spider/analytics-using-aws/analytics_traffic.png
deleted file mode 100644
index e1fc2ca..0000000
Binary files a/docs/games/spider/analytics-using-aws/analytics_traffic.png and /dev/null differ
diff --git a/docs/games/spider/analytics-using-aws/index-analytics-using-aws.mdx b/docs/games/spider/analytics-using-aws/index-analytics-using-aws.mdx
deleted file mode 100644
index 619721e..0000000
--- a/docs/games/spider/analytics-using-aws/index-analytics-using-aws.mdx
+++ /dev/null
@@ -1,437 +0,0 @@
----
-id: index-analytics-using-aws
-title: How to Build and Deploy Your Own Analytics Dashboard using NodeJS and Redis on the AWS Platform
-sidebar_label: Building an Analytics Dashboard using NodeJS, Redis and AWS
-slug: /create/aws/analytics-using-aws
-authors: [ajeet]
----
-
-
-An interactive analytics dashboard serves several purposes. They allow you to share data and provide you with all those vital information to make game-changing decisions at a faster pace. Building a real-time dynamic dashboard using a traditional relational database might require a complex set of queries. By using a NoSQL database like Redis, you can build a powerful interactive and dynamic dashboard with a small number of Redis commands.
-
-Let’s take a look at how this was achieved.
-
-- What will you build?
-- What will you need?
-- Getting started
-- How does it work?
-- How data is stored
-- Navigating the application
-
-### What will you build?
-
-You’ll build an analytics dashboard app that uses Redis Bitmap written in NodeJS (JavaScript) and then deploy it to AWS.
-
-Ready to get started? Ok, let’s dive straight in.
-
-### What will you need?
-
-- [NodeJS](https://developer.redis.com/develop/node): used as an open-source, cross-platform, backend JavaScript runtime environment that executes Javascript code outside a web browser.
-- [Redis Cloud](https://redis.com/try-free): used as a real-time database, cache, and message broker.
-- [NPM](https://www.npmjs.com/): used as a package manager. It allows you to build node apps.
-
-### Getting Started
-
-### Prepare the environment
-
-- Install Node - v12.19.0
-- Install NPM - v6.14.8
-
-### Step 1. Sign up for a Free Redis Enterprise Cloud Account
-
-[Follow this tutorial](https://developer.redis.com/create/aws/redis-on-aws) to sign up for a free Redis Enterprise Cloud account.
-
-
-
-Choose AWS as a Cloud vendor while creating your new subscription. At the end of the database creation process, you will get a Redis Enterprise CLoud database endpoint and password. You can save it for later use.
-
-
-
-### Step 2. Clone the repository
-
-```bash
- git clone https://github.com/redis-developer/basic-analytics-dashboard-redis-bitmaps-nodejs
-```
-
-### Step 3. Set up a backend environment
-
-First we will be setting up environment variables
-
-Go to /server folder (cd ./server) and then execute the below command:
-
-```bash
- cp .env.example .env
-```
-
-Open .env file and add Redis Enterprise Cloud Database Endpoint URL, port and password as shown below:
-
-```
-
-PORT=3000
-
-# Host and a port. Can be with `redis://` or without.
-# Host and a port encoded in redis uri take precedence over other environment variable.
-# preferable
-REDIS_ENDPOINT_URI=redis://redis-XXXX.c212.ap-south-1-1.ec2.cloud.redislabs.com:15564
-
-# Or you can set it here (ie. for docker development)
-REDIS_HOST=redis-XXXX.c212.ap-south-1-1.ec2.cloud.redislabs.com
-REDIS_PORT=XXXX
-
-# You can set password here
-REDIS_PASSWORD=reXXX
-
-COMPOSE_PROJECT_NAME=redis-analytics-bitmaps
-```
-
-### Step 4. Install dependencies
-
-```bash
- npm install
-```
-
-### Step 5. Run the backend
-
-```bash
- npm run dev
-```
-
-### Step 6. Set up the frontend environment
-
-Go to the `client` folder (`cd ./client`) and then:
-
-```bash
- cp .env.example .env
-```
-
-Add the exact URL path and port number of your choice for VUE_APP_API_URL parameter as shown below:
-
-```
-VUE_APP_API_URL=http://localhost:3000
-```
-
-### Step 7. Install dependencies
-
-```bash
- npm install
-```
-
-### Step 8. Run the frontend
-
-```bash
- npm run serve
-```
-
-
-
-### How does it work?
-
-#### How the data is stored:
-
-The event data is stored in various keys and data types which is discussed below:
-
-##### For each of time spans:
-
-- year: like 2021
-- month: like 2021-03 (means March of 2021)
-- day: like 2021-03-03 (means 3rd March of 2021)
-- weekOfMonth: like 2021-03/4 (means 4th week of March 2021)
-- anytime
-
-##### For each of scopes:
-
-- source
-- action
-- source + action
-- action + page
-- userId + action
-- global
-
-##### For each of data types/types:
-
-- count (Integer stored as String)
-- bitmap
-- set
-
-It generates keys with the following naming convention:
-
-```bash
- rab:{type}[:custom:{customName}][:user:{userId}][:source:{source}][:action:{action}][:page:{page}]:timeSpan:{timeSpan}
-```
-
-where values in [] are optional.
-
-For each generated key like `rab:count:*`, data is stored like: `INCR {key}`
-
-##### Example:
-
-```bash
- INCR rab:count:action:addToCart:timeSpan:2015-12/3
-```
-
-For each generated key like: `rab:set:*`, data is stored like:
-
-```bash
- SADD {key} {userId}
-```
-
-##### Example:
-
-```bash
- SADD rab:set:action:addToCart:timeSpan:2015-12/3 8
-```
-
-- For each generated key like `rab:bitmap:*`, data is stored like:
-
- ```bash
- SETBIT {key} {userId} 1
- ```
-
-##### Example:
-
-```bash
- SETBIT rab:bitmap:action:addToCart:timeSpan:2015-12/3 8 1
-```
-
-### Cohort data
-
-- We store users who register and then bought some products (action order matters).
-- For each buy action in December we first check if the user performed register action (register counter must be greater than zero).
-- If so, we set user bit to 1
-
-##### Example
-
-```
- SETBIT rab:bitmap:custom:cohort-buy:timeSpan:{timeSpan} {userId} 1
-```
-
-- Example - User Id 2 bought 2 products on 2015-12-17. It won't be stored.
-- Example - User Id 10 bought 1 product on 2015-12-17 and registered on 2015-12-16.
- So, it will be stored like:
-
-```bash
- SETBIT rab:bitmap:custom:cohort-buy:timeSpan:2015-12 10 1
-```
-
-- We assume that user cannot buy without registering first.
-
-#### Retention data
-
-- Retention means users who bought on two different dates
-- For each buy action we check if user bought more products anytime than bought on particular day (current purchase not included).
-- If so, we add user id to set like:
-
- ```bash
- SADD rab:set:custom:retention-buy:timeSpan:{timeSpan} {userId}
- ```
-
-- Example - User Id 5 bought 3 products on 2015-12-15. His retention won't be stored (products bought on particular day: 2, products bought anytime: 0).
-- Example - User Id 3 bought 1 product on 2015-12-15 and before - 1 product on 2015-12-13. His retention will be stored (products bought on particular day: 0, products bought anytime: 1) like:
-
-```bash
- SADD rab:set:custom:retention-buy:timeSpan:2015-12
-```
-
-### How the data is accessed:
-
-#### Total Traffic:
-
-##### December:
-
-````bash
- BITCOUNT rab:bitmap:custom:global:timeSpan:2015-12```
-````
-
-##### X week of December:
-
-```bash
- BITCOUNT rab:bitmap:custom:global:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:custom:global:timeSpan:2015-12/3
-```
-
-#### Traffic per Page ({page} is one of: homepage, product1, product2, product3):
-
-##### December:
-
-```bash
- BITCOUNT rab:bitmap:action:visit:page:{page}:timeSpan:2015-12
-```
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:action:visit:page:homepage:timeSpan:2015-12
-```
-
-##### X week of December:
-
-```bash
- BITCOUNT rab:bitmap:action:visit:page:{page}:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:action:visit:page:product1:timeSpan:2015-12/2
-```
-
-#### Traffic per Source ({source} is one of: Google, Facebook, email, direct, referral, none):
-
-##### December:
-
-```bash
- BITCOUNT rab:bitmap:source:{source}:timeSpan:2015-12
-```
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:source:referral:timeSpan:2015-12
-```
-
-##### X week of December:
-
-```bash
- BITCOUNT rab:bitmap:source:{source}:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:source:google:timeSpan:2015-12/1
-```
-
-##### Trend traffic ({page} is one of: homepage, product1, product2, product3):
-
-##### December:
-
-From
-
-```bash
- BITCOUNT rab:bitmap:action:visit:{page}:timeSpan:2015-12-01
-```
-
-to
-
-```bash
- BITCOUNT rab:bitmap:action:visit:{page}:timeSpan:2015-12-31
-```
-
-- 1st Week of December: Similar as above, but from 2015-12-01 to 2015-12-07
-- 2nd Week of December: Similar as above, but from 2015-12-08 to 2015-12-14
-- 3rd Week of December: Similar as above, but from 2015-12-15 to 2015-12-21
-- 4th Week of December: Similar as above, but from 2015-12-22 to 2015-12-28
-- 5th Week of December: Similar as above, but from 2015-12-29 to 2015-12-31
-
-##### Example:
-
-```bash
- BITCOUNT rab:bitmap:action:visit:homepage:timeSpan:2015-12-29 => BITCOUNT rab:bitmap:action:visit:homepage:timeSpan:2015-12-30 => BITCOUNT rab:bitmap:action:visit:homepage:timeSpan:2015-12-31
-```
-
-#### Total products bought:
-
-##### December:
-
-```bash
- GET rab:count:action:buy:timeSpan:2015-12
-```
-
-##### X week of December:
-
-```bash
- GET rab:count:action:buy:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- GET rab:count:action:buy:timeSpan:2015-12/1
-```
-
-#### Total products added to cart:
-
-##### December:
-
-```bash
- GET rab:count:action:addToCart:timeSpan:2015-12
-```
-
-##### X week of December:
-
-```bash
- GET rab:count:action:addToCart:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- GET rab:count:action:addToCart:timeSpan:2015-12/1
-```
-
-##### Shares of products bought ({productPage} for product1, product2, product3):
-
-#### December:
-
-```bash
- GET rab:count:action:buy:page:{productPage}:timeSpan:2015-12
-```
-
-##### Example:
-
-```bash
- GET rab:count:action:buy:page:product3:timeSpan:2015-12
-```
-
-##### X week of December:
-
-```bash
- GET rab:count:action:buy:page:{productPage}:timeSpan:2015-12/{X}
-```
-
-##### Example:
-
-```bash
- GET rab:count:action:buy:page:product1:timeSpan:2015-12/2
-```
-
-#### Customer and Cohort Analysis
-
-- People who registered: BITCOUNT rab:bitmap:action:register:timeSpan:2015-12
-- People who register then bought (order matters): BITCOUNT rab:bitmap:custom:cohort-buy:timeSpan:2015-12
-- Dropoff: (People who register then bought / People who register) \* 100 [%]
-- Customers who bought only specified product ({productPage} is one of: product1, product2, product3):
-
- ```bash
- SMEMBERS rab:set:action:buy:page:{productPage}:timeSpan:2015-12
- ```
-
-##### Example:
-
-```bash
- SMEMBERS rab:set:action:buy:page:product2:timeSpan:2015-12
-```
-
-#### Customers who bought Product1 and Product2:
-
-```
- SINTER rab:set:action:buy:page:product1:timeSpan:anytime rab:set:action:buy:page:product2:timeSpan:anytime
-```
-
-#### Customer Retention (customers who bought on the different dates):
-
-```bash
- SMEMBERS rab:set:custom:retention-buy:timeSpan:anytime
-```
-
-### References
-
-- [Project Source Code](https://github.com/redis-developer/basic-analytics-dashboard-redis-bitmaps-nodejs)
-- [Use cases of Bitmaps](https://redis.io/topics/data-types-intro)
-- [How to Build a Slack Bot to Retrieve Lost Files Using AWS S3 and Search](/create/aws/slackbot)
-- [How to Deploy and Manage Redis Database on AWS Using Terraform](/create/aws/terraform)
diff --git a/docs/games/spider/bidding-on-aws/images/architecture.png b/docs/games/spider/bidding-on-aws/images/architecture.png
deleted file mode 100644
index 9070f49..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/architecture.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_10.png b/docs/games/spider/bidding-on-aws/images/image_10.png
deleted file mode 100644
index 8c5ff50..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_10.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_11.png b/docs/games/spider/bidding-on-aws/images/image_11.png
deleted file mode 100644
index b098307..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_11.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_12.png b/docs/games/spider/bidding-on-aws/images/image_12.png
deleted file mode 100644
index feb236b..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_12.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_13.png b/docs/games/spider/bidding-on-aws/images/image_13.png
deleted file mode 100644
index 3790cc2..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_13.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_2.png b/docs/games/spider/bidding-on-aws/images/image_2.png
deleted file mode 100644
index 31bc689..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_2.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_3.png b/docs/games/spider/bidding-on-aws/images/image_3.png
deleted file mode 100644
index 0f3e296..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_3.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_4.png b/docs/games/spider/bidding-on-aws/images/image_4.png
deleted file mode 100644
index 4b20a07..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_4.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_5.png b/docs/games/spider/bidding-on-aws/images/image_5.png
deleted file mode 100644
index 765296b..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_5.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_6.png b/docs/games/spider/bidding-on-aws/images/image_6.png
deleted file mode 100644
index 3790cc2..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_6.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_7.png b/docs/games/spider/bidding-on-aws/images/image_7.png
deleted file mode 100644
index 8c5ff50..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_7.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_8.png b/docs/games/spider/bidding-on-aws/images/image_8.png
deleted file mode 100644
index 765296b..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_8.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_9.png b/docs/games/spider/bidding-on-aws/images/image_9.png
deleted file mode 100644
index 0f3e296..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_9.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/images/image_featured.png b/docs/games/spider/bidding-on-aws/images/image_featured.png
deleted file mode 100644
index d9b3c24..0000000
Binary files a/docs/games/spider/bidding-on-aws/images/image_featured.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/index-bidding-on-aws.mdx b/docs/games/spider/bidding-on-aws/index-bidding-on-aws.mdx
deleted file mode 100644
index 1741c50..0000000
--- a/docs/games/spider/bidding-on-aws/index-bidding-on-aws.mdx
+++ /dev/null
@@ -1,287 +0,0 @@
----
-id: index-bidding-on-aws
-title: How to Build a Real-Time Bidding Platform using NodeJS, AWS Lambda and Redis
-sidebar_label: Building a Real-Time Bidding Platform using NodeJS, AWS Lambda and Redis
-slug: /create/aws/bidding-on-aws
-authors: [ajeet]
----
-
-Digital technology has propelled us forward to an exciting new era and has transformed almost every aspect of life. We’re more interconnected than ever as communication has become instant. Working from home has now become the norm, helping us pivot to a new way of working during the pandemic. And our ability to reduce carbon emissions by attending work-related events online has meant that we’ve taken greater strides to combat global warming. Continuing this trend is [Shamshir Anees and his team](https://github.com/shamshiranees), who have created an application that can host digital auctions. By using Redis, data transmission between components was carried out with maximum efficiency, providing users with real-time bidding updates on the dashboard.
-
-Let’s take a look at how this was achieved. We’d also like to point out that we have a diverse range of exciting applications for you to check out on the [Redis Launchpad](https://launchpad.redis.com).
-
-- What will you build?
-- What will you need?
-- Architecture
-- How does it work?
-- Getting started
-- How data is stored
-- Navigating the application
-
-### What will you build?
-
-You’ll build an application that will allow users to attend and take part in digital auctions. The application will allow users to create an account, put in bids, and even set up their own auction. Below we’ll uncover the required components, their functionality, and how to deploy them within this architecture.
-
-Ready to get started? Ok, let’s dive straight in.
-
-### What will you need?
-
-- [NodeJS](https://developer.redis.com/develop/node): used as an open-source, cross-platform, backend JavaScript runtime environment that executes Javascript code outside a web browser.
-- [Amazon Cognito](https://aws.amazon.com/cognito/): used to securely manage and synchronize app data for users on mobile.
-- [Redis Cloud](https://redis.com/try-free): used as a real-time database, cache, and message broker.
-- [Redis Stack](https://developer.redis.com/quick-start): used to store, update and fetch JSON values from Redis.
-- [Socket.IO](https://socket.io/): used as a library that provides real-time, bi-directional, and event-based communication between the browser and the server.
-- [AWS Lambda](https://aws.amazon.com/lambda/): used as a serverless compute service that runs your code in response events and manages the underlying compute service automatically for you.
-- [Amazon SNS/Amazon SES](https://aws.amazon.com/sns/): a fully managed messaging service for both application-to-application (A2A) and application-to-person (A2P) communication.
-
-### Architecture
-
-
-
-### How does it work?
-
-#### All auctions
-
-NodeJS connects to the Redis Enterprise Cloud database.
-
-The frontend then communicates with the NodeJS backend through API calls.
-
-`GET : /api/auctions` fetches all the keys from Auctions Hash.
-
-NodeJS uses the Redis module to work with Redis Enterprise Cloud. The Redis client is then created using the Redis credentials and hmget(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-#### Each auction
-
-`GET : /api/auctions/{auctionId}` fetches each auction item by id.
-
-NodeJS uses the Redis module to work with Redis Cloud. The Redis client is then created using the Redis credentials and hmget(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-All bidding data of an auction item
-
-`GET : /api/bidding/{auctionId}`
-
-NodeJS uses the Redis module to work with Redis Cloud. The Redis client is then created using the Redis credentials and hmget(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-#### Profile settings
-
-`GET : /api/settings`
-
-NodeJS uses the Redis module to work with Redis Cloud. The Redis client is then created using the Redis credentials and hmget(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-#### User info
-
-`GET : /api/users/{email}`
-
-NodeJS uses the Redis module to work with Redis Cloud. The Redis client is then created using the Redis credentials and hmget(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-### Getting started
-
-### Prerequisites
-
-- [NodeJS](https://nodejs.org/en/)
-- [NPM](https://www.npmjs.com/)
-
-### Step 1. Sign up for a Free Redis Enterprise Cloud Account
-
-[Follow this tutorial](https://developer.redis.com/create/aws/redis-on-aws) to sign up for a free Redis Enterprise Cloud account.
-
-
-
-Choose AWS as a Cloud vendor while creating your new subscription. At the end of the database creation process, you will get a Redis Enterprise CLoud database endpoint and password. You can save it for later use.
-
-
-
-### Step 2: Clone the backend GitHub repository
-
-```
-https://github.com/redis-developer/NR-digital-auction-backend
-```
-
-### Step 3. Install the package dependencies
-
-The 'npm install' is a npm cli-command that does the predefined thing i.e install dependencies specified inside package.json
-
-```
-npm install
-```
-
-### Step 4. Setting up environment variables
-
-```
-export REDIS_END_POINT=XXXX
-export REDIS_PASSWORD=XXX
-export REDIS_PORT=XXX
-```
-
-### Step 5. Building the application
-
-```
-npm run build
-```
-
-### Step 6. Starting the application
-
-```
-npm start
-```
-
-### Step 7. Cloning the Frontend GITHUB repository
-
-```
-git clone https://github.com/redis-developer/NR-digital-auction-frontend
-```
-
-### Step 8. Building the application
-
-```
-npm run build
-```
-
-### Step 9. Starting the application
-
-```
-npm start
-```
-
-### Step 10. Accessing the application
-
-
-
-### Step 11. Signing up to the application
-
-
-
-### Step 12. Sign-in
-
-
-
-### Step 13. Accessing the dashboard
-
-
-
-### Step 14. Listing the auction item
-
-
-
-### Step 15. Accessing the bidding page
-
-
-
-### How data is stored
-
-The [Redis Enterprise Cloud](https://redis.com/try-free) database with Redis Stack is what you’ll use to install the data.
-
-### Auctions
-
-- Type - Redis Hash.
-- Used for storing auctions data.
-- UUID generated from the backend (NodeJS) serves as the key
-- JSON data which represents the Auction object and includes the following keys
- - auctionId
- - auctionItemName
- - description
- - lotNo
- - quantity
- - buyersPremium
- - itemUnit
- - minBidAmount
- - bidIncrement
- - startDateTime
- - endDateTime
- - images
- - currentBid
-- NodeJS connects to the Redis Cloud database. The Frontend communicates with the NodeJS backend through API calls.
-- POST : /api/auctions.
-- The request body has JSON data to be inserted into the database.
-- NodeJS uses the Redis module to work with Redis Cloud. The Redis client is created. using the Redis credentials and hmset(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-### Biddings
-
-- Type - Redis Hash
-- Used for storing the bids placed on each auction item
-- NodeJS connects to the Redis Cloud database. The Frontend communicates with the NodeJS backend through API calls.
-- POST : `/api/bidding`
-- The request body has JSON data to be inserted into the database.
-- AuctionId from request body serves as the key
-- JSON data which includes keys:
-
- - currentBid
- - currentBidTime
- - currentBidEndTime, and
- - biddings array (id, auctionId, userId, username, bidAmount, bidTime)
-
-- The bidding array has all of the bids placed for a particular auction item.
-- Based on the current BidEndTime and BidTime, the auction end date is extended based on the Dynamic closing concept.
-- Current dynamic closing logic - If a new bid is placed within the last 5 minutes of the auction end time, the end time is extended by 1 hour.
-- This will be configurable in the SaaS solution.
-- NodeJS uses the Redis module to work with Redis Cloud. The Redis client is created using the Redis credentials and hmset(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-### Profile Settings
-
-- Type - string
-- JSON data which includes keys - serves as a value
-- NodeJS connects to the Redis Cloud database. The frontend communicates with the NodeJS backend through API calls.
-- POST : `/api/settings`
-- The request body has JSON data to be inserted into the database.
-- NodeJS uses the Redis module to work with Redis Cloud. The Redis client is created using the Redis credentials and hmset(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-### Users
-
-- Type - Redis Hash
-- Used for storing user details
-- NodeJS connects to the Redis Cloud database. The Frontend communicates with the NodeJS backend through API calls.
-- POST : `/api/users`
-- The request body has JSON data to be inserted into the database
-- The email id serves as the key
-- The JSON data which includes keys - serves as a value
-- NodeJS uses the Redis module to work with Redis Cloud. The Redis client is created using the Redis credentials and hmset(). This is the equivalent of the HMSET command that’s used to push data to the Redis database.
-
-### Navigating the application
-
-### Creating an account
-
-When you go onto the Digital Auction’s homepage, you’ll come across a range of items that are to be auctioned (see below). Click on the ‘Welcome’ button to create an account.
-
-
-
-You’ll then be taken to the sign-up page. Enter your details and click ‘sign-up.’ Once you’ve completed the sign-up form, you’ll receive a confirmation email to activate your account.
-
-### Placing a bid
-
-Go to the homepage to have access to view all of the items and their auction details. All of the data here is being populated by Redis Stack and Redis Cloud. Scroll through the page and click on the item that you want to place a bid for.
-
-
-
-When you click on an item, you’ll see the details for the bidding process at the top of the page. You’ll also have the option to set a reminder by receiving an email of whenever the bidding process of this item begins.
-
-On the right-hand side of the image, you’ll see the highest bid that’s been placed for this item. Below is a list of previous bids made by different users which are updated in real-time.
-
-Click on the ‘Place Bid’ button to make a bid.
-
-To access the meta-data information or view more images of the item, simply scroll down the page (see below).
-
-
-
-### Viewing your Bidding History
-
-Click on ‘My biddings’ at the top of the navigation bar to view your bidding history (see below).
-
-
-
-### Viewing upcoming auctions
-
-Click on ‘Auctions’ at the top of the navigation bar to view all upcoming auctions.
-
-
-
-### Conclusion: Leveraging Redis and AWS to Empower Auctioneers with Real-time Data
-
-Digital technology has had a ripple effect across all aspects of modern life. The ability to complete important tasks online instead of in-person has revolutionized the way we live, helping us to reduce carbon emissions, save time from traveling and have instant access to reams worth of data that we never had before.
-
-However, the success of such events hinges on a database’s ability to transmit data in real-time. Any blips in transmission would create a disconnect between users and the auction, impeding auctioneers’ reactions to bids. This would only result in frustration, disengagement, and a complete divorce of users from the application.
-
-But thanks to Redis, the components that made up the architecture system became vastly more interconnected so data was able to be sent, processed, and received in real-time. Achieving this paves the way for a smooth bidding process where users can interact with events in real-time without interruptions, ultimately enhancing the functionality of the app.
-
-[NR-Digital-Auction](https://launchpad.redis.com/?id=project%3ANR-digital-auction-frontend) is a fantastic example of how innovations can be brought to life by using Redis. Everyday programmers are experimenting with Redis to build applications that are impacting everyday life from around the world and you can too!
-
-So what can you build with Redis? For more inspiration, you can head over to the [Redis Launchpad](https://launchpad.redis.com/) to access an exciting range of applications. If you're ready to get started building, quickly spin up a free database [Redis Enterprise](https://redis.com/try-free/).
diff --git a/docs/games/spider/bidding-on-aws/matrix200.png b/docs/games/spider/bidding-on-aws/matrix200.png
deleted file mode 100644
index 0465899..0000000
Binary files a/docs/games/spider/bidding-on-aws/matrix200.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/sign3.png b/docs/games/spider/bidding-on-aws/sign3.png
deleted file mode 100644
index 9d48729..0000000
Binary files a/docs/games/spider/bidding-on-aws/sign3.png and /dev/null differ
diff --git a/docs/games/spider/bidding-on-aws/sign4.png b/docs/games/spider/bidding-on-aws/sign4.png
deleted file mode 100644
index cf3dbb1..0000000
Binary files a/docs/games/spider/bidding-on-aws/sign4.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/chatapp2.png b/docs/games/spider/chatapp/chatapp2.png
deleted file mode 100644
index 26d8e55..0000000
Binary files a/docs/games/spider/chatapp/chatapp2.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/chatapp3.png b/docs/games/spider/chatapp/chatapp3.png
deleted file mode 100644
index 9d48729..0000000
Binary files a/docs/games/spider/chatapp/chatapp3.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/chatapp4.png b/docs/games/spider/chatapp/chatapp4.png
deleted file mode 100644
index cf3dbb1..0000000
Binary files a/docs/games/spider/chatapp/chatapp4.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/chatapp_homepage.png b/docs/games/spider/chatapp/chatapp_homepage.png
deleted file mode 100644
index 3967d54..0000000
Binary files a/docs/games/spider/chatapp/chatapp_homepage.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/chatapp_server.png b/docs/games/spider/chatapp/chatapp_server.png
deleted file mode 100644
index b5c1414..0000000
Binary files a/docs/games/spider/chatapp/chatapp_server.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/image_chatapp1.png b/docs/games/spider/chatapp/image_chatapp1.png
deleted file mode 100644
index a995f82..0000000
Binary files a/docs/games/spider/chatapp/image_chatapp1.png and /dev/null differ
diff --git a/docs/games/spider/chatapp/index-chatapp.mdx b/docs/games/spider/chatapp/index-chatapp.mdx
deleted file mode 100644
index 2d5f566..0000000
--- a/docs/games/spider/chatapp/index-chatapp.mdx
+++ /dev/null
@@ -1,257 +0,0 @@
----
-id: index-chatapp
-title: How to Build a Real Time Chat application on Amazon Web Services using Python and Redis
-sidebar_label: Building a Real-Time Chat application on AWS using Python and Redis
-slug: /create/aws/chatapp
-authors: [ajeet]
----
-
-
-Real time chat messaging apps are surging in popularity exponentially. Mobile apps like WhatsApp, Facebook, Telegram, Slack, Discord have become “a part and parcel” of our life. Users are addicted to these live chat mobile app conversations as they bring a personal touch and offer a real-time interaction
-
-
-
-There’s been a rise in the number of social media apps that bring social elements to enable activities like collaboration, messaging, social interaction and commenting. Such activities require a real-time capability to automatically present updated information to users. More and more developers are tapping into the power of Redis as it is extremely fast & its support for a variety of rich data structures such as Lists, Sets, Sorted Sets, Hashes etc. Redis comes along with a Pub/Sub messaging functionality that allows developers to scale the backend by spawning multiple server instances.
-
-- What will you build?
-- What will you need?
-- Getting started
-- How it works?
-- How is the data stored?
-- How is the data accessed?
-
-### 1. What will you build?
-
-In this tutorial, we will see how to build a realtime chat app built with Flask, Socket.IO and Redis Enterprise Cloud running on Amazon Web Services. This example uses pub/sub features combined with web-sockets for implementing the message communication between client and server.
-
-
-
-### 2. What will you need?
-
-- Frontend - React, Socket.IO
-- Backend - Python(Flask), Redis Enterprise Cloud hosted on AWS
-
-### 3. Getting Started
-
-### Step 1. Sign up for a Free Redis Enterprise Cloud Account
-
-[Follow this tutorial](https://developer.redis.com/create/aws/redis-on-aws) to sign up for a free Redis Enterprise Cloud account.
-If you already have an existing account, then all you need are your login credentials to access your subscription.
-
-
-
-Choose AWS as the Cloud vendor while creating your new subscription. While creating a new database, ensure that you set your own password. At the end of the database creation process, you will get a Redis Enterprise Cloud database endpoint and port. Save these, you will need them later.
-
-
-
-:::tip
-You don't need to create an AWS account for setting up your Redis database. Redis Enterprise Cloud on AWS is a fully managed database-as-a-service trusted by thousands of customers for high performance, infinite scalability, true high availability, and best-in-class support.
-:::
-
-### Step 2. Clone the repository
-
-```
-git clone https://github.com/redis-developer/basic-redis-chat-app-demo-python
-```
-
-### Step 3. Installing the required packages
-
-```
-cd client
-yarn install
-```
-
-### Step 4. Starting the frontend
-
-```
-yarn start
-```
-
-```
-You can now view client in the browser.
-
- Local: http://localhost:3000
- On Your Network: http://192.168.1.9:3000
-```
-
-
-
-### Step 5. Installing the required Python modules
-
-```
-cd ..
-pip3 install -r requirements.txt
-```
-
-### Step 6: Running Backend
-
-```
-python3 -m venv venv/
-source venv/bin/activate
-python3 app.py
-```
-
-```
-python3 app.py
- * Restarting with stat
- * Debugger is active!
- * Debugger PIN: 220-696-610
-(8122) wsgi starting up on http://127.0.0.1:5000
-```
-
-
-
-### Step 7. How does it work?
-
-The chat app server works as a basic REST API which involves keeping the session and handling the user state in the chat rooms (besides the WebSocket/real-time part).
-When the server starts, the initialization step occurs. At first, a new Redis connection is established and it's checked whether it's needed to load the demo data.
-
-#### Initialization
-
-For simplicity, a key with total_users value is checked: if it does not exist, we fill the Redis database with initial data. EXISTS total_users (checks if the key exists)
-The demo data initialization is handled in multiple steps:
-
-#### Creating demo users
-
-We create a new user id: INCR total_users. Then we set a user ID lookup key by user name: e.g.
-
-```bash
-SET username:nick user:1
-```
-
-And finally, the rest of the data is written to the hash set:
-
-Example:
-
-```bash
-HSET user:1 username "nick" password "bcrypt_hashed_password".
-```
-
-Additionally, each user is added to the default "General" room.
-For handling rooms for each user, we have a set that holds the room ids. Here's an example command of how to add the room:
-
-```bash
-SADD user:1:rooms "0"
-```
-
-#### Populating private messages between users
-
-First, private rooms are created: if a private room needs to be established, for each user a room id: room:1:2 is generated, where numbers correspond to the user ids in ascending order.
-
-E.g. Create a private room between 2 users:
-
-```bash
-SADD user:1:rooms 1:2 and SADD user:2:rooms 1:2
-```
-
-Then we add messages for each conversation to this room by writing to a sorted set:
-
-```bash
-ZADD room:1:2 1615480369 "{'from': 1, 'date': 1615480369, 'message': 'Hello', 'roomId': '1:2'}"
-```
-
-We are using a stringified JSON to keep the message structure and simplify the implementation details for this demo-app. You may choose to use a Hash or JSON
-
-### Populate the "General" room with messages
-
-Messages are added to the sorted set with id of the "General" room: room:0
-
-#### Pub/sub
-
-After initialization, a pub/sub subscription is created: SUBSCRIBE MESSAGES. At the same time, each server instance will run a listener on a message on this channel to receive real-time updates.
-
-Again, for simplicity, each message is serialized to JSON, which we parse and then handle in the same manner, as WebSocket messages.
-
-Pub/sub allows connecting multiple servers written in different platforms without taking into consideration the implementation detail of each server.
-
-#### Real-time chat and session handling
-
-When a WebSocket connection is established, we can start to listen for events:
-
-- Connection. A new user is connected. At this point, a user ID is captured and saved to the session (which is cached in Redis). Note, that session caching is language/library-specific and it's used here purely for persistence and maintaining the state between server reloads.
-
-A global set with online_users key is used for keeping the online state for each user. So on a new connection, a user ID is written to that set:
-
-```bash
-SADD online_users 1
-```
-
-Here we have added user with id 1 to the set online_users
-
-After that, a message is broadcasted to the clients to notify them that a new user is joined the chat.
-
-- Disconnect. It works similarly to the connection event, except we need to remove the user for online_users set and notify the clients: SREM online_users 1 (makes user with id 1 offline).
-
-- Message. A user sends a message, and it needs to be broadcasted to the other clients. The pub/sub allows us also to broadcast this message to all server instances which are connected to this Redis:
-
-```
- PUBLISH message "{'serverId': 4132, 'type':'message', 'data': {'from': 1, 'date': 1615480369, 'message': 'Hello', 'roomId': '1:2'}}"
-```
-
-Note we send additional data related to the type of the message and the server id. Server id is used to discard the messages by the server instance which sends them since it is connected to the same MESSAGES channel.
-
-The type field of the serialized JSON corresponds to the real-time method we use for real-time communication (connect/disconnect/message).
-
-The data is method-specific information. In the example above it's related to the new message.
-
-### Step 8. How the data is stored?
-
-Redis is used mainly as a database to keep the user/messages data and for sending messages between connected servers.
-
-The real-time functionality is handled by Socket.IO for server-client messaging. Additionally each server instance subscribes to the MESSAGES channel of pub/sub and dispatches messages once they arrive. Note that, the server transports pub/sub messages with a separate event stream (handled by Server Sent Events), this is due to the need of running pub/sub message loop apart from socket.io signals.
-
-The chat data is stored in various keys and various data types.
-User data is stored in a hash set where each user entry contains the next values:
-
-- username: unique user name;
-- password: hashed password
-
-- Additionally a set of rooms is associated with user
-- Rooms are sorted sets which contains messages where score is the timestamp for each message
-- Each room has a name associated with it
-- Online set is global for all users is used for keeping track on which user is online.
-- User hash set is accessed by key user:{userId}. The data for it stored with HSET key field data. User id is calculated by incrementing the total_users key (INCR total_users)
-
-- Username is stored as a separate key (username:{username}) which returns the userId for quicker access and stored with SET username:{username} {userId}.
-
-- Rooms which user belongs too are stored at user:{userId}:rooms as a set of room ids. A room is added by SADD user:{userId}:rooms {roomId} command.
-
-- Messages are stored at room:{roomId} key in a sorted set (as mentioned above). They are added with ZADD room:{roomId} {timestamp} {message} command. Message is serialized to an app-specific JSON string.
-
-### Step 9. How the data is accessed?
-
-Get User HGETALL user:{id}.
-
-```bash
-HGETALL user:2
-```
-
-where we get data for the user with id: 2.
-
-- Online users: SMEMBERS online_users. This will return ids of users which are online
-
-- Get room ids of a user: SMEMBERS user:{id}:rooms.
- Example:
-
-```
- SMEMBERS user:2:rooms
-```
-
-This will return IDs of rooms for user with ID: 2
-
-- Get list of messages ZREVRANGE room:{roomId} {offset_start} {offset_end}.
- Example:
-
-```
- ZREVRANGE room:1:2 0 50
-```
-
-It will return 50 messages with 0 offsets for the private room between users with IDs 1 and 2.
-
-### Further References
-
-- [Building a realtime chat app demo in .Net](https://github.com/redis-developer/basic-redis-chat-app-demo-dotnet)
-- [Building a realtime chat app demo in Java](https://github.com/redis-developer/basic-redis-chat-app-demo-java)
-- [Building a realtime chat demo in NodeJS](https://github.com/redis-developer/basic-redis-chat-app-demo-nodejs)
-- [Building a realtime chat app demo in Go](https://github.com/redis-developer/basic-redis-chat-demo-go)
-- [Building a Redis chat application demo in Ruby](https://github.com/redis-developer/basic-redis-chat-demo-ruby)
diff --git a/docs/games/spider/chatapp/index-chatapp.mdx_python b/docs/games/spider/chatapp/index-chatapp.mdx_python
deleted file mode 100644
index 309d267..0000000
--- a/docs/games/spider/chatapp/index-chatapp.mdx_python
+++ /dev/null
@@ -1,246 +0,0 @@
----
-id: index-chatapp
-title: How to build a Chat application using Redis
-sidebar_label: How to build a Chat application using Redis
-slug: /howtos/chatapp
----
-
-import Tabs from '@theme/Tabs';
-import TabItem from '@theme/TabItem';
-import useBaseUrl from '@docusaurus/useBaseUrl';
-import RedisCard from '@theme/RedisCard';
-
-
-In this tutorial, we will see how to build a basic chat application built with Flask, Socket.IO and Redis.
-
-
-
-
-
-
-### Step 1. Pre-requisite
-
-- Python 3.6+
-
-### Step 2. Clone the repository
-
-```
-git clone https://github.com/redis-developer/basic-redis-chat-app-demo-python
-```
-
-
-### Step 3. Installing the requred packages
-
-```
-cd client
-yarn install
-```
-
-### Step 4. Starting the frontend
-
-```
-yarn start
-```
-
-```
-You can now view client in the browser.
-
- Local: http://localhost:3000
- On Your Network: http://192.168.1.9:3000
-```
-
-
-
-
-
-
-### Step 4. Installing the required Python modules
-
-
-```
-cd ..
-pip3 install -r requirements.txt
-```
-
-### Step 5: Running Backend
-
-
-
-
-
-```
-python3 -m venv venv/
-source venv/bin/activate
-python3 app.py
-```
-
-```
-python3 app.py
- * Restarting with stat
- * Debugger is active!
- * Debugger PIN: 220-696-610
-(8122) wsgi starting up on http://127.0.0.1:5000
-```
-
-
-
-### How it works?
-
-
-The chat server works as a basic REST API which involves keeping the session and handling the user state in the chat rooms (besides the WebSocket/real-time part).
-When the server starts, the initialization step occurs. At first, a new Redis connection is established and it's checked whether it's needed to load the demo data.
-
-#### Initialization
-
-For simplicity, a key with total_users value is checked: if it does not exist, we fill the Redis database with initial data. EXISTS total_users (checks if the key exists)
-The demo data initialization is handled in multiple steps:
-
-#### Creating of demo users
-
-We create a new user id: INCR total_users. Then we set a user ID lookup key by user name: e.g.
-
-```
-SET username:nick user:1
-```
-
-And finally, the rest of the data is written to the hash set: e.g. HSET user:1 username "nick" password "bcrypt_hashed_password".
-
-Additionally, each user is added to the default "General" room.
-For handling rooms for each user, we have a set that holds the room ids. Here's an example command of how to add the room:
-
-```
-SADD user:1:rooms "0"
-```
-
-Populate private messages between users. At first, private rooms are created: if a private room needs to be established, for each user a room id: room:1:2 is generated, where numbers correspond to the user ids in ascending order.
-
-E.g. Create a private room between 2 users:
-
-```
-SADD user:1:rooms 1:2 and SADD user:2:rooms 1:2
-```
-
-Then we add messages to this room by writing to a sorted set:
-
-```
-ZADD room:1:2 1615480369 "{'from': 1, 'date': 1615480369, 'message': 'Hello', 'roomId': '1:2'}"
-```
-We use a stringified JSON for keeping the message structure and simplify the implementation details for this demo-app.
-
-Populate the "General" room with messages. Messages are added to the sorted set with id of the "General" room: room:0
-
-#### Pub/sub
-
-After initialization, a pub/sub subscription is created: SUBSCRIBE MESSAGES. At the same time, each server instance will run a listener on a message on this channel to receive real-time updates.
-
-Again, for simplicity, each message is serialized to JSON, which we parse and then handle in the same manner, as WebSocket messages.
-
-Pub/sub allows connecting multiple servers written in different platforms without taking into consideration the implementation detail of each server.
-
-#### Real-time chat and session handling
-
-When a WebSocket/real-time server is instantiated, which listens for the next events:
-
-- Connection. A new user is connected. At this point, a user ID is captured and saved to the session (which is cached in Redis). Note, that session caching is language/library-specific and it's used here purely for persistence and maintaining the state between server reloads.
-
-A global set with online_users key is used for keeping the online state for each user. So on a new connection, a user ID is written to that set:
-
-```
-SADD online_users 1
-```
-
-Here we have added user with id 1 to the set online_users
-
-After that, a message is broadcasted to the clients to notify them that a new user is joined the chat.
-
-- Disconnect. It works similarly to the connection event, except we need to remove the user for online_users set and notify the clients: SREM online_users 1 (makes user with id 1 offline).
-
-- Message. A user sends a message, and it needs to be broadcasted to the other clients. The pub/sub allows us also to broadcast this message to all server instances which are connected to this Redis:
-
-```
-PUBLISH message "{'serverId': 4132, 'type':'message', 'data': {'from': 1, 'date': 1615480369, 'message': 'Hello', 'roomId': '1:2'}}"
-```
-
-Note we send additional data related to the type of the message and the server id. Server id is used to discard the messages by the server instance which sends them since it is connected to the same MESSAGES channel.
-
-type field of the serialized JSON corresponds to the real-time method we use for real-time communication (connect/disconnect/message).
-
-data is method-specific information. In the example above it's related to the new message.
-
-### How the data is stored:
-
-Redis is used mainly as a database to keep the user/messages data and for sending messages between connected servers.
-
-The real-time functionality is handled by Socket.IO for server-client messaging. Additionally each server instance subscribes to the MESSAGES channel of pub/sub and dispatches messages once they arrive. Note that, the server transports pub/sub messages with a separate event stream (handled by Server Sent Events), this is due to the need of running pub/sub message loop apart from socket.io signals.
-
-The chat data is stored in various keys and various data types.
-User data is stored in a hash set where each user entry contains the next values:
-- username: unique user name;
-- password: hashed password
-
-- Additionally a set of rooms is associated with user
-- Rooms are sorted sets which contains messages where score is the timestamp for each message
-- Each room has a name associated with it
-- Online set is global for all users is used for keeping track on which user is online.
-- User hash set is accessed by key user:{userId}. The data for it stored with HSET key field data. User id is calculated by incrementing the total_users key (INCR total_users)
-
-- Username is stored as a separate key (username:{username}) which returns the userId for quicker access and stored with SET username:{username} {userId}.
-
-- Rooms which user belongs too are stored at user:{userId}:rooms as a set of room ids. A room is added by SADD user:{userId}:rooms {roomId} command.
-
-- Messages are stored at room:{roomId} key in a sorted set (as mentioned above). They are added with ZADD room:{roomId} {timestamp} {message} command. Message is serialized to an app-specific JSON string.
-
-### How the data is accessed:
-
-Get User HGETALL user:{id}.
-
-```
-HGETALL user:2
-```
-
-where we get data for the user with id: 2.
-
-- Online users: SMEMBERS online_users. This will return ids of users which are online
-
-- Get room ids of a user: SMEMBERS user:{id}:rooms.
-Example:
-
-```
-SMEMBERS user:2:rooms
-```
-
-This will return IDs of rooms for user with ID: 2
-
-- Get list of messages ZREVRANGE room:{roomId} {offset_start} {offset_end}.
-Example:
-
-```
-ZREVRANGE room:1:2 0 50
-```
-It will return 50 messages with 0 offsets for the private room between users with IDs 1 and 2.
-
-
-
-
-
-
-
-
-
-
-
-
-
-## Step 1. Pre-requisite
-
-
-
-
-
-
diff --git a/docs/games/spider/import/index-database-migration-aws-elasticache-redis-enterprise-cloud.mdx b/docs/games/spider/import/index-database-migration-aws-elasticache-redis-enterprise-cloud.mdx
deleted file mode 100644
index 801665d..0000000
--- a/docs/games/spider/import/index-database-migration-aws-elasticache-redis-enterprise-cloud.mdx
+++ /dev/null
@@ -1,140 +0,0 @@
----
-id: index-database-migration-aws-elasticache-redis-enterprise-cloud
-title: How to migrate your database from AWS ElastiCache to Redis without any downtime
-sidebar_label: Online Data Migration from AWS Elasticache to Redis
-slug: /create/aws/import/database-migration-aws-elasticache-redis-enterprise-cloud/
-authors: [ajeet]
----
-
-Most of the database migration tools available today are offline in nature. They are complex and require manual intervention.
-
-If you want to migrate your data from Amazon ElastiCache to Redis Enterprise Cloud, for example, the usual process is to back up your ElastiCache data to an Amazon S3 bucket and then import your data using the Redis Enterprise Cloud UI. This process can require painful downtime and could result in data loss. Other available techniques include creating point-in-time snapshots of the source Redis server and applying the changes to the destination servers to keep both the servers in sync. That might sound like a good approach, but it can be challenging when you have to maintain dozens of scripts to implement the migration strategy.
-
-So we’ve come up with a different approach:
-
-## Introducing RIOT
-
-
-
-RIOT is an open source online migration tool built by Julien Ruaux, a Solution Architect at Redis. RIOT implements client-side replication using a producer/consumer approach. The producer is the combination of the key and value readers that have a connection to ElastiCache. The key reader component identifies keys to be replicated using scan and keyspace notifications. For each key, the value reader component performs a DUMP and handles the resulting key+bytes to the consumer (writer), which performs a RESTORE on the Redis Enterprise connection.
-
-This blog post will show how to perform a seamless online migration of databases from ElastiCache to Redis Enterprise Cloud.
-
-## Prerequisites:
-
-You will require a few resources to use the migration tool:
-
-- A Redis Enterprise Cloud subscription, sign up [here](https://redis.com/try-free/)
-- Amazon ElastiCache (a primary endpoint in the case of a single-master EC and a configuration endpoint in the case of a clustered EC: Refer to Finding Connection Endpoints on the ElastiCache documentation to learn more)
-- An Amazon EC2 instance based on Linux
-
-## Step 1 - Setting up an Amazon EC2 instance
-
-You can either create a new EC2 instance or leverage an existing one. In our example, we will first create an instance on Amazon Web Services (AWS). The most common scenario is to access an ElastiCache cluster from an Amazon EC2 instance in the same Amazon Virtual Private Cloud (Amazon VPC). We have used Ubuntu 16.04 LTS for this setup, but you can choose the Ubuntu or Debian distribution of your choice.
-
-Use SSH to connect to this new EC2 instance from your computer as shown here:
-
-```
-ssh -i “public key”
-```
-
-## Step 2 - Install the redis-cli tool
-
-```
-$ sudo apt update
-# sudo apt install -y redis-tools
-```
-
-## Verify the connectivity with the ElastiCache database
-
-Syntax:
-
-```
-$ redis-cli -h -p 6379
-```
-
-Command:
-
-```
-$ sudo redis-cli -h -p 6379
-```
-
-Ensure that the above command allows you to connect to the remote Redis database successfully.
-
-## Step 3 - Using the RIOT migration tool
-
-Run the commands below to set up the migration tool.
-
-## Prerequisites:
-
-### Install Java
-
-We recommended using OpenJDK 11 or later:
-
-```
-sudo add-apt-repository ppa:openjdk-r/ppa && sudo apt-get update -q && sudo apt install -y openjdk-11-jdk
-```
-
-### Installing RIOT
-
-Unzip the package and make sure the RIOT binaries are in place, as shown here:
-
-```
-wget https://github.com/Redislabs-Solution-Architects/riot/releases/download/v2.0.8/riot-redis-2.0.8.zip
-```
-
-```
-unzip riot-redis-2.0.8.zip
-cd riot-redis-2.0.8/bin/
-```
-
-You can check the version of RIOT by running the command below:
-
-```
-./riot-redis --version
-RIOT version "2.0.8"
-```
-
-```
-bin/riot-redis --help
-Usage: riot-redis [OPTIONS] [COMMAND]
- -q, --quiet Log errors only
- -d, --debug Log in debug mode (includes normal stacktrace)
- -i, --info Set log level to info
- -h, --help Show this help message and exit.
- -V, --version Print version information and exit.
-Redis connection options
- -r, --redis= Redis connection string (default: redis://localhost:6379)
- -c, --cluster Connect to a Redis Cluster
- -m, --metrics Show metrics
- -p, --pool= Max pool connections (default: 8)
-Commands:
- replicate, r Replicate a source Redis database in a target Redis database
- info, i Display INFO command output
- latency, l Calculate latency stats
- ping, p Execute PING command
-```
-
-Once Java and RIOT are installed, we are all set to begin the migration process with the command below, which replicates data directly from the source (ElastiCache) to the target (Redis Enterprise Cloud).
-
-## Step 4 - Migrate the data
-
-Finally, it’s time to replicate the data from ElastiCache to Redis Enterprise Cloud by running the following command:
-
-```
-sudo ./riot-redis -r redis://:6379 replicate -r redis://password@:port --live
-```
-
-ElastiCache can be configured in two ways: clustered and non-clustered. In the chart below, the first row shows what commands you should perform for the non-clustered scenario, while the second row shows the command for the clustered scenario with a specific database namespace:
-
-As you can see, whenever you have a clustered ElastiCache, you need to pass the `–cluster` option before specifying the source ElastiCache endpoint.
-
-## Important notes
-
-- Perform user acceptance testing of the migration before using it in production.
-- Once the migration is complete, ensure that application traffic gets successfully redirected to the Redis Enterprise endpoint.
-- Perform the migration process during a period of low traffic to minimize the chance of data loss.
-
-## Conclusion
-
-If you’re looking for a simple and easy-to-use live migration tool that can help you move data from Amazon ElastiCache to Redis Enterprise Cloud with no downtime, RIOT is a promising option.
diff --git a/docs/games/spider/index-spider.mdx b/docs/games/spider/index-spider.mdx
index bb99196..be86b40 100644
--- a/docs/games/spider/index-spider.mdx
+++ b/docs/games/spider/index-spider.mdx
@@ -5,27 +5,27 @@ sidebar_label: Overview
slug: /games/spider
---
-import RedisCard from '@theme/RedisCard';
+import ThreeTenCard from '@theme/ThreeTenCard';
-The following links provide you with the available options to run apps on AWS using Redis:
+Spider Solitaire Documentation:
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
+
\ No newline at end of file
diff --git a/docs/games/spider/redis-on-aws/README.md b/docs/games/spider/redis-on-aws/README.md
deleted file mode 100644
index 4148b2f..0000000
--- a/docs/games/spider/redis-on-aws/README.md
+++ /dev/null
@@ -1 +0,0 @@
-# images
diff --git a/docs/games/spider/redis-on-aws/Verify_subscription.png b/docs/games/spider/redis-on-aws/Verify_subscription.png
deleted file mode 100644
index e591162..0000000
Binary files a/docs/games/spider/redis-on-aws/Verify_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/activate.png b/docs/games/spider/redis-on-aws/activate.png
deleted file mode 100644
index b871b07..0000000
Binary files a/docs/games/spider/redis-on-aws/activate.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws.png b/docs/games/spider/redis-on-aws/aws.png
deleted file mode 100644
index 5d49974..0000000
Binary files a/docs/games/spider/redis-on-aws/aws.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws1.png b/docs/games/spider/redis-on-aws/aws1.png
deleted file mode 100644
index 2a7cb7c..0000000
Binary files a/docs/games/spider/redis-on-aws/aws1.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws2.png b/docs/games/spider/redis-on-aws/aws2.png
deleted file mode 100644
index a346573..0000000
Binary files a/docs/games/spider/redis-on-aws/aws2.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws3.png b/docs/games/spider/redis-on-aws/aws3.png
deleted file mode 100644
index f2baeab..0000000
Binary files a/docs/games/spider/redis-on-aws/aws3.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws4.png b/docs/games/spider/redis-on-aws/aws4.png
deleted file mode 100644
index aa2899c..0000000
Binary files a/docs/games/spider/redis-on-aws/aws4.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws5.png b/docs/games/spider/redis-on-aws/aws5.png
deleted file mode 100644
index f163131..0000000
Binary files a/docs/games/spider/redis-on-aws/aws5.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/aws6.png b/docs/games/spider/redis-on-aws/aws6.png
deleted file mode 100644
index fb2267b..0000000
Binary files a/docs/games/spider/redis-on-aws/aws6.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/choosemodule.png b/docs/games/spider/redis-on-aws/choosemodule.png
deleted file mode 100644
index ba5165d..0000000
Binary files a/docs/games/spider/redis-on-aws/choosemodule.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/create_database.png b/docs/games/spider/redis-on-aws/create_database.png
deleted file mode 100644
index 6f68abd..0000000
Binary files a/docs/games/spider/redis-on-aws/create_database.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/create_subscription.png b/docs/games/spider/redis-on-aws/create_subscription.png
deleted file mode 100644
index 9ba14b5..0000000
Binary files a/docs/games/spider/redis-on-aws/create_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/createdatabase.png b/docs/games/spider/redis-on-aws/createdatabase.png
deleted file mode 100644
index a4415e9..0000000
Binary files a/docs/games/spider/redis-on-aws/createdatabase.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/deployment.png b/docs/games/spider/redis-on-aws/deployment.png
deleted file mode 100644
index adb4c49..0000000
Binary files a/docs/games/spider/redis-on-aws/deployment.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/final_subscription.png b/docs/games/spider/redis-on-aws/final_subscription.png
deleted file mode 100644
index 333ce58..0000000
Binary files a/docs/games/spider/redis-on-aws/final_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/README.md b/docs/games/spider/redis-on-aws/images/README.md
deleted file mode 100644
index 4148b2f..0000000
--- a/docs/games/spider/redis-on-aws/images/README.md
+++ /dev/null
@@ -1 +0,0 @@
-# images
diff --git a/docs/games/spider/redis-on-aws/images/Verify_subscription.png b/docs/games/spider/redis-on-aws/images/Verify_subscription.png
deleted file mode 100644
index e591162..0000000
Binary files a/docs/games/spider/redis-on-aws/images/Verify_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/activate.png b/docs/games/spider/redis-on-aws/images/activate.png
deleted file mode 100644
index b871b07..0000000
Binary files a/docs/games/spider/redis-on-aws/images/activate.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/aws.png b/docs/games/spider/redis-on-aws/images/aws.png
deleted file mode 100644
index 5d49974..0000000
Binary files a/docs/games/spider/redis-on-aws/images/aws.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/choosemodule.png b/docs/games/spider/redis-on-aws/images/choosemodule.png
deleted file mode 100644
index ba5165d..0000000
Binary files a/docs/games/spider/redis-on-aws/images/choosemodule.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/create_database.png b/docs/games/spider/redis-on-aws/images/create_database.png
deleted file mode 100644
index 6f68abd..0000000
Binary files a/docs/games/spider/redis-on-aws/images/create_database.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/create_subscription.png b/docs/games/spider/redis-on-aws/images/create_subscription.png
deleted file mode 100644
index 347fdd1..0000000
Binary files a/docs/games/spider/redis-on-aws/images/create_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/createdatabase.png b/docs/games/spider/redis-on-aws/images/createdatabase.png
deleted file mode 100644
index a4415e9..0000000
Binary files a/docs/games/spider/redis-on-aws/images/createdatabase.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/deployment.png b/docs/games/spider/redis-on-aws/images/deployment.png
deleted file mode 100644
index adb4c49..0000000
Binary files a/docs/games/spider/redis-on-aws/images/deployment.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/final_subscription.png b/docs/games/spider/redis-on-aws/images/final_subscription.png
deleted file mode 100644
index 333ce58..0000000
Binary files a/docs/games/spider/redis-on-aws/images/final_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/launch_database.png b/docs/games/spider/redis-on-aws/images/launch_database.png
deleted file mode 100644
index 861f20f..0000000
Binary files a/docs/games/spider/redis-on-aws/images/launch_database.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/plan.png b/docs/games/spider/redis-on-aws/images/plan.png
deleted file mode 100644
index d481c75..0000000
Binary files a/docs/games/spider/redis-on-aws/images/plan.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/recloud1.png b/docs/games/spider/redis-on-aws/images/recloud1.png
deleted file mode 100644
index a73de59..0000000
Binary files a/docs/games/spider/redis-on-aws/images/recloud1.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/recloud2.png b/docs/games/spider/redis-on-aws/images/recloud2.png
deleted file mode 100644
index 5cb98fc..0000000
Binary files a/docs/games/spider/redis-on-aws/images/recloud2.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/recloud3.png b/docs/games/spider/redis-on-aws/images/recloud3.png
deleted file mode 100644
index a390a68..0000000
Binary files a/docs/games/spider/redis-on-aws/images/recloud3.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/region.png b/docs/games/spider/redis-on-aws/images/region.png
deleted file mode 100644
index ddbe75e..0000000
Binary files a/docs/games/spider/redis-on-aws/images/region.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/select_cloud.png b/docs/games/spider/redis-on-aws/images/select_cloud.png
deleted file mode 100644
index 2784e45..0000000
Binary files a/docs/games/spider/redis-on-aws/images/select_cloud.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/select_subscription.png b/docs/games/spider/redis-on-aws/images/select_subscription.png
deleted file mode 100644
index 7643333..0000000
Binary files a/docs/games/spider/redis-on-aws/images/select_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/subscription.png b/docs/games/spider/redis-on-aws/images/subscription.png
deleted file mode 100644
index b4a6134..0000000
Binary files a/docs/games/spider/redis-on-aws/images/subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/images/try-free.png b/docs/games/spider/redis-on-aws/images/try-free.png
deleted file mode 100644
index 11915ea..0000000
Binary files a/docs/games/spider/redis-on-aws/images/try-free.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/index-redis-on-aws.mdx b/docs/games/spider/redis-on-aws/index-redis-on-aws.mdx
deleted file mode 100644
index 92b8808..0000000
--- a/docs/games/spider/redis-on-aws/index-redis-on-aws.mdx
+++ /dev/null
@@ -1,81 +0,0 @@
----
-id: index-redis-on-aws
-title: Create Redis database on AWS
-sidebar_label: Redis on AWS
-slug: /create/aws/redis-on-aws
-authors: [ajeet]
----
-
-Redis Enterprise Cloud on AWS is a fully Managed Redis Enterprise as a service. Designed for modern distributed applications, Redis Enterprise Cloud on AWS is known for its high performance, infinite scalability and true high availability.
-
-Follow the below steps to setup Redis Enterprise Cloud hosted over AWS Cloud:
-
-### Step 1. Create free cloud account
-
-Create your free Redis Enterprise Cloud account. Once you click on “Get Started”, you will receive an email with a link to activate your account and complete your signup process.
-
-:::tip
-For a limited time, use **TIGER200** to get **$200** credits on Redis Enterprise Cloud and try all the advanced capabilities!
-
-:tada: [Click here to sign up](https://redis.com/try-free)
-
-:::
-
-
-
-### Step 2. Create Your subscription
-
-Next, you will have to create Redis Enterprise Cloud subscription. In the Redis Enterprise Cloud menu, click "Create your Subscription".
-
-
-
-### Step 3. Select the right Subscription Plan
-
-Select "Fixed Plan" for low throughout application as for now.
-
-
-
-### Step 4. Select cloud vendor
-
-For the cloud provider, select your preferred cloud (for demo purpose)
-
-
-
-### Step 5. Click "Create Subscription"
-
-Finally, click on "Create Subscription" button.
-
-
-
-You can now verify the subscription as shown below:
-
-
-
-### Step 6. Create database
-
-Click "Create Database". Enter database name and your preferred module.
-
-
-
-### Step 7. Launch database
-
-Click "Activate" and wait for few seconds till it gets activated. Once fully activated, you will see the database endpoints as shown below:
-
-
-
-##
-
-
diff --git a/docs/games/spider/redis-on-aws/launch_database.png b/docs/games/spider/redis-on-aws/launch_database.png
deleted file mode 100644
index 861f20f..0000000
Binary files a/docs/games/spider/redis-on-aws/launch_database.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/launchpad.png b/docs/games/spider/redis-on-aws/launchpad.png
deleted file mode 100644
index 66e7a45..0000000
Binary files a/docs/games/spider/redis-on-aws/launchpad.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/plan.png b/docs/games/spider/redis-on-aws/plan.png
deleted file mode 100644
index d481c75..0000000
Binary files a/docs/games/spider/redis-on-aws/plan.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/recloud1.png b/docs/games/spider/redis-on-aws/recloud1.png
deleted file mode 100644
index a73de59..0000000
Binary files a/docs/games/spider/redis-on-aws/recloud1.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/recloud2.png b/docs/games/spider/redis-on-aws/recloud2.png
deleted file mode 100644
index 5cb98fc..0000000
Binary files a/docs/games/spider/redis-on-aws/recloud2.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/recloud3.png b/docs/games/spider/redis-on-aws/recloud3.png
deleted file mode 100644
index a390a68..0000000
Binary files a/docs/games/spider/redis-on-aws/recloud3.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/region.png b/docs/games/spider/redis-on-aws/region.png
deleted file mode 100644
index ddbe75e..0000000
Binary files a/docs/games/spider/redis-on-aws/region.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/select_cloud.png b/docs/games/spider/redis-on-aws/select_cloud.png
deleted file mode 100644
index 2784e45..0000000
Binary files a/docs/games/spider/redis-on-aws/select_cloud.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/select_subscription.png b/docs/games/spider/redis-on-aws/select_subscription.png
deleted file mode 100644
index 5316156..0000000
Binary files a/docs/games/spider/redis-on-aws/select_subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/subscription.png b/docs/games/spider/redis-on-aws/subscription.png
deleted file mode 100644
index b4a6134..0000000
Binary files a/docs/games/spider/redis-on-aws/subscription.png and /dev/null differ
diff --git a/docs/games/spider/redis-on-aws/tryfree.png b/docs/games/spider/redis-on-aws/tryfree.png
deleted file mode 100644
index 1a40ca2..0000000
Binary files a/docs/games/spider/redis-on-aws/tryfree.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image1.png b/docs/games/spider/slackbot/images/image1.png
deleted file mode 100644
index 4ec65f1..0000000
Binary files a/docs/games/spider/slackbot/images/image1.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image10.png b/docs/games/spider/slackbot/images/image10.png
deleted file mode 100644
index 033a7ca..0000000
Binary files a/docs/games/spider/slackbot/images/image10.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image11.png b/docs/games/spider/slackbot/images/image11.png
deleted file mode 100644
index 37f3416..0000000
Binary files a/docs/games/spider/slackbot/images/image11.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image12.png b/docs/games/spider/slackbot/images/image12.png
deleted file mode 100644
index 2ffe89e..0000000
Binary files a/docs/games/spider/slackbot/images/image12.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image13.png b/docs/games/spider/slackbot/images/image13.png
deleted file mode 100644
index 476ebc1..0000000
Binary files a/docs/games/spider/slackbot/images/image13.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image14.png b/docs/games/spider/slackbot/images/image14.png
deleted file mode 100644
index 2dd64dd..0000000
Binary files a/docs/games/spider/slackbot/images/image14.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image15.png b/docs/games/spider/slackbot/images/image15.png
deleted file mode 100644
index 5e13d45..0000000
Binary files a/docs/games/spider/slackbot/images/image15.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image16.png b/docs/games/spider/slackbot/images/image16.png
deleted file mode 100644
index b25b808..0000000
Binary files a/docs/games/spider/slackbot/images/image16.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image17.png b/docs/games/spider/slackbot/images/image17.png
deleted file mode 100644
index b3c686e..0000000
Binary files a/docs/games/spider/slackbot/images/image17.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image18.png b/docs/games/spider/slackbot/images/image18.png
deleted file mode 100644
index bca51c9..0000000
Binary files a/docs/games/spider/slackbot/images/image18.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image19.png b/docs/games/spider/slackbot/images/image19.png
deleted file mode 100644
index 04f135a..0000000
Binary files a/docs/games/spider/slackbot/images/image19.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image2.png b/docs/games/spider/slackbot/images/image2.png
deleted file mode 100644
index 7f68cb4..0000000
Binary files a/docs/games/spider/slackbot/images/image2.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image20.png b/docs/games/spider/slackbot/images/image20.png
deleted file mode 100644
index 03ddb15..0000000
Binary files a/docs/games/spider/slackbot/images/image20.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image21.png b/docs/games/spider/slackbot/images/image21.png
deleted file mode 100644
index 695c01b..0000000
Binary files a/docs/games/spider/slackbot/images/image21.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image22.gif b/docs/games/spider/slackbot/images/image22.gif
deleted file mode 100644
index 6a1c30e..0000000
Binary files a/docs/games/spider/slackbot/images/image22.gif and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image22.png b/docs/games/spider/slackbot/images/image22.png
deleted file mode 100644
index 695c01b..0000000
Binary files a/docs/games/spider/slackbot/images/image22.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image23.png b/docs/games/spider/slackbot/images/image23.png
deleted file mode 100644
index 548649a..0000000
Binary files a/docs/games/spider/slackbot/images/image23.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image24.png b/docs/games/spider/slackbot/images/image24.png
deleted file mode 100644
index bf6fdf0..0000000
Binary files a/docs/games/spider/slackbot/images/image24.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image25.png b/docs/games/spider/slackbot/images/image25.png
deleted file mode 100644
index b6cc828..0000000
Binary files a/docs/games/spider/slackbot/images/image25.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image26.png b/docs/games/spider/slackbot/images/image26.png
deleted file mode 100644
index 08c43a9..0000000
Binary files a/docs/games/spider/slackbot/images/image26.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image27.png b/docs/games/spider/slackbot/images/image27.png
deleted file mode 100644
index 9c86247..0000000
Binary files a/docs/games/spider/slackbot/images/image27.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image28.png b/docs/games/spider/slackbot/images/image28.png
deleted file mode 100644
index 4541398..0000000
Binary files a/docs/games/spider/slackbot/images/image28.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image29.png b/docs/games/spider/slackbot/images/image29.png
deleted file mode 100644
index 31a3c41..0000000
Binary files a/docs/games/spider/slackbot/images/image29.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image3.png b/docs/games/spider/slackbot/images/image3.png
deleted file mode 100644
index 7902c63..0000000
Binary files a/docs/games/spider/slackbot/images/image3.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image30.png b/docs/games/spider/slackbot/images/image30.png
deleted file mode 100644
index 96144f9..0000000
Binary files a/docs/games/spider/slackbot/images/image30.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image31.png b/docs/games/spider/slackbot/images/image31.png
deleted file mode 100644
index 2ef036f..0000000
Binary files a/docs/games/spider/slackbot/images/image31.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image32.png b/docs/games/spider/slackbot/images/image32.png
deleted file mode 100644
index 66aa1d7..0000000
Binary files a/docs/games/spider/slackbot/images/image32.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image35.png b/docs/games/spider/slackbot/images/image35.png
deleted file mode 100644
index 83b1be4..0000000
Binary files a/docs/games/spider/slackbot/images/image35.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image4.png b/docs/games/spider/slackbot/images/image4.png
deleted file mode 100644
index 0237925..0000000
Binary files a/docs/games/spider/slackbot/images/image4.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image5.png b/docs/games/spider/slackbot/images/image5.png
deleted file mode 100644
index 2c40100..0000000
Binary files a/docs/games/spider/slackbot/images/image5.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image6.png b/docs/games/spider/slackbot/images/image6.png
deleted file mode 100644
index fc97464..0000000
Binary files a/docs/games/spider/slackbot/images/image6.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image7.png b/docs/games/spider/slackbot/images/image7.png
deleted file mode 100644
index 9de3655..0000000
Binary files a/docs/games/spider/slackbot/images/image7.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image8.png b/docs/games/spider/slackbot/images/image8.png
deleted file mode 100644
index 8d8f45f..0000000
Binary files a/docs/games/spider/slackbot/images/image8.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/images/image9.png b/docs/games/spider/slackbot/images/image9.png
deleted file mode 100644
index 8947b5d..0000000
Binary files a/docs/games/spider/slackbot/images/image9.png and /dev/null differ
diff --git a/docs/games/spider/slackbot/index-slackbot.mdx b/docs/games/spider/slackbot/index-slackbot.mdx
deleted file mode 100644
index bf26516..0000000
--- a/docs/games/spider/slackbot/index-slackbot.mdx
+++ /dev/null
@@ -1,463 +0,0 @@
----
-id: index-slackbot
-title: How to Build a Slack Bot to Retrieve Lost Files Using AWS S3 and Redis Search and Query Engine
-sidebar_label: Building a Slack Bot using AWS S3 and Redis Search and Query Engine
-slug: /create/aws/slackbot
-authors: [ajeet]
----
-
-
-
-If you work remotely then you’re likely to have come across Slack at some point. And if you use Slack on a daily basis, then you’ll be all too aware of how easy it can be to lose files. Being pinged every day by different employees across different channels makes it difficult to keep track of files.
-
-Eventually, you may be forced to rummage through a cluttered library of documents in search of that one \_crucial \_document that’s needed for a task, report or even a meeting. We’ve all been there and the frustration is just as real as it is irritating...which is why [this Launchpad App](https://launchpad.redis.com/?id=project%3AReeko-Slack-Bot) has created an application to remove this impediment.
-
-It was a tricky application to make, requiring a lot of attention to detail across a number of different components for it to come to fruition. However, the success of this application was possible due to Redis’ ability to extract and process data proficiently.
-
-Thanks to Redis, all of the components functioned harmoniously with one another, creating a fully optimal application that interacts with users in real-time.
-
-Let’s take a look at how this was done. Before we dive in, we’d also like to point out that we have a variety of exciting applications for you to check out in our [Redis Launchpad](https://launchpad.redis.com/).
-
-
-
-1. What will you build?
-2. What will you need?
-3. Architecture overview
-4. Getting started
-5. How it works
-
-### Step 1. What will you build?
-
-You’ll build a special search engine that’s designed to retrieve lost files in Slack. This is especially handy for people who work from home, where documents between channels are easily lost between employees.
-
-Below we’ll go through each step chronologically and highlight what components are required to create this application.
-
-Ready to get started? OK, let’s dive straight in.
-
-### Step 2. What will you need?
-
-- [Slack](https://slack.com/intl/en-gb/): used as an instant messaging app that connects employees with one another.
-- [Slack Block Kit](https://api.slack.com/block-kit): used as a UI framework for Slack apps that offers a balance of control and flexibility when building experiences.
-- [Python](https://www.python.org/): the preferred programming language to connect Redis in the application.
-- [Redis Stack](https://redis.io/docs/stack/): includes a built-in Search and Query feature that provides querying, secondary indexing and full-text search.
-- [S3 bucket](https://aws.amazon.com/es/s3/): used as a public cloud storage resource in Amazon Web Services (AWS).
-- [AWS Textract](https://aws.amazon.com/es/textract/): used as a machine learning service that automatically extracts text.
-- [Nodejs](https://nodejs.org/en/): responsible for image generation.
-
-### Step 3. Architecture
-
-Let’s look at each of the components that creates the Reeko-Slack bot:
-
-
-
-#### 1. file_shared
-
-- When a new file is shared in any public slack channel the file_share event is sent to the Slack Bot app.
-- The file name is added as a suggestion using the [`FT.SUGADD`](https://redis.io/commands/?group=search) command in Redis.
-- All file data is added using the [`JSON.SET`](https://redis.io/commands/?group=json) command.
-- The file is then stored on the S3 bucket as an object with the key as the filename.
-
-#### 2. S3-get
-
-- The [`JSON.GET`](https://redis.io/commands/?group=json) command checks whether the desired file exists.
-- The file will then be retrieved from the S3 bucket if found.
-
-#### 3. S3-search
-
-- The [`FT.SEARCH`](https://redis.io/commands/?group=search) command uses the Redis Search and Query engine to look for documents in the S3 bucket- Users are presented will be prompted with different file name suggestions based on what they’ve typed in the search bar.
-- Once the user chooses one of the file suggestions, it is then downloaded and sent back to Slack.
-
-#### 4. S3-delete
-
-- User types the file name from the command['text'] parameter
-- The file data is deleted from Redis using the [`JSON.DEL`](https://redis.io/commands/?group=json) command and is also removed from Redis's suggestions using the `FT.SUGDEL` command.
-
-#### 5. Summarise-document
-
-- The file name is identified from the command['text'] parameter.
-- It is then retrieved from the S3 bucket through the [JSON.GET](https://redis.io/commands/?group=json) command.
-- Users can either download the pdf or png file locally from the S3 bucket.
-- The text is extracted using [AWS Textract](https://aws.amazon.com/textract/).
-- The extracted text is then summarised using Hugging face transformers summarization pipeline. The text summary is also added back to the `JSON` document using [`JSON.SET`](https://redis.io/commands/?group=json) command.
-- A post request is then sent to the /create-image on the NodeJS backend with the file name and summary text.
-- An image is generated using a base template.
-- The image that is returned is saved to the S3 bucket and sent back to Slack.
-- The image URL is also added to the `JSON` document using [`JSON.SET`](https://redis.io/commands/?group=json) command.
-
-### What is the S3 bucket?
-
-The [S3 bucket](https://aws.amazon.com/s3/) is a simple storage service from Amazon. It allows users to store objects through a web service interface. The product’s value comes from its ability to store, protect and retrieve data from ‘buckets’ at any time from anywhere and on any device.
-
-### Step 4. Getting started
-
-#### Prerequisites
-
-- Python 3.6+
-- ngrok
-- AWS Account
-- Slack
-- Docker
-
-### 1. Run Redis Docker container
-
-This simple container image bundles together the latest stable releases of Redis and select Redis modules from Redis Labs. This image is based on the official image of Redis from Docker. By default, the container starts with Redis' default configuration and all included modules loaded.
-
-```bash
- docker run -d -p 6379:6379 redis/redis-stack
-```
-
-### 2. Setup a Python environment
-
-To test the integration, Python needs to be installed on your computer. You can get a suitable release from [here](https://www.python.org/downloads/). To check your Python version, follow the command below.
-
-```
- # Python 3.6+ required
- git clone https://github.com/redis-developer/Reeko-Slack-Bot
- cd Reeko-Slack-Bot
- python3 -m venv env
- source env/bin/activate
- cd python-backend
- pip3 install -r requirements.txt
-```
-
-### 3. Using ngrok as a local proxy
-
-To develop locally we'll be using ngrok. This will allow you to expose a public endpoint that Slack can use to send your app events. If you haven't already, [install ngrok from their website](https://ngrok.com/download) .
-
-The ngrok exposes local networked services behind NATs and firewalls to the public internet over a secure tunnel. Share local websites, build/test webhook consumers and self-host personal services.
-
-### 4. Setting up an AWS Account
-
-For testing you need a verified aws account. You can get your credentials file at ~/.aws/credentials (C:\Users\USER_NAME.aws\credentials for Windows users) and copy the following lines in the [.env](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/python-backend/.env) file.
-
-Also make sure to add your S3 bucket name in the .env file.
-
-```
- AWS_ACCESS_KEY_ID="YOUR_ACCESS_KEY_ID"
- AWS_SECRET_ACCESS_KEY="YOUR_SECRET_ACCESS_KEY"
- BUCKET_NAME="YOUR_BUCKET_NAME"
-```
-
-### 5. Install Slack on your local system
-
-Slack needs to be installed on your computer. If it hasn’t been installed, you can get it from here for [Windows](https://slack.com/intl/en-in/downloads/windows) or [Mac](https://slack.com/intl/en-in/downloads/mac). If you don’t already have an account you can make one [here](https://slack.com/get-started#/create).
-
-To get started, you'll need to create a new Slack app by clicking on the following link - [https://api.slack.com/apps](https://api.slack.com/apps)
-The foundational framework we’ll be using is Bolt. This will make it easier to build Slack apps with the platform’s latest features.
-
-1. Click on the `Create an App` button
-2. Name the application ‘Reeko’ and choose the development workspace. \
-
-
-
-
-
-3. Requesting scopes - [Scopes](https://api.slack.com/scopes) give your app permission to do things (for example, post messages) in your development workspace. You can select the scopes to add to your app by navigating over to the _OAuth & Permissions_ sidebar.
-4. Add the _Bot Token Scopes_ by clicking on the `Add an OAuth Scope `button.
-
-| OAuth Scope | Description |
-| -------------------- | ------------------------------------------------------------------------------- |
-| channels:history | View messages and other content in public channels that reeko has been added to |
-| channels:join | Join public channels in a workspace |
-| channels:read | View basic information about public channels in a workspace |
-| channels:join | Join public channels in a workspace |
-| chat:write | Send messages as @reeko |
-| chat:write.customize | Send messages as @reeko with a customized username and avatar |
-| chat:write.public | Send messages to channels @reeko isn't a member of |
-| files:read | View files shared in channels and conversations that reeko has been added to |
-| files:write | Upload, edit, and delete files as reeko |
-
-1. Add the _User Token Scopes_ by clicking on the `Add an OAuth Scope `button
-
-| OAuth Scope | Description |
-| ---------------- | -------------------------------------------------------------------------------- |
-| channels:history | View messages and other content in public channels that Reeko has been added to. |
-| files:read | View files shared in channels and conversations that Reeko has been added to. |
-
-1. Install your own app by selecting the `Install App` button at the top of the OAuth & Permissions page, or from the sidebar.
-2. After clicking through one more green `Install App To Workspace` button, you'll be sent through the Slack OAuth UI.
-3. After installation, you'll land back in the _OAuth & Permissions_ page and find a _Bot User OAuth Access Token._ and a _User OAuth Token_. Click on the copy button for each of them. These tokens need to be added to the [.env](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/python-backend/.env) file. (The bot token starts with xoxb whereas the user token is longer and starts with xoxp).
-
-```
-SLACK_USER_TOKEN=xoxp-your-user-token
-SLACK_BOT_TOKEN=xoxb-your-bot-token
-```
-
-
-
-1. As well as the access token, you'll need a signing secret. Your app's signing secret verifies that incoming requests are coming from Slack. Navigate to the _Basic Information_ page from your [app management page](https://api.slack.com/apps). Under App Credentials, copy the value for _Signing Secret_ and add it to the [.env](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/python-backend/env) file.
-
-```
-SLACK_SIGNING_SECRET=your-signing-secret
-```
-
-
-
-1. Make sure you have followed the steps in [Cloning the repo](https://github.com/redis-developer/Reeko-Slack-Bot/tree/master/python-backend#Cloning-the-repo) to start the bolt app. The HTTP server is using a built-in development adapter, which is responsible for handling and parsing incoming events from Slack on port 3000.
-
-```
-python3 app.py
-```
-
-
-
-Open a new terminal and ensure that you've installed [ngrok](https://github.com/redis-developer/Reeko-Slack-Bot/tree/master/python-backend#ngrok). Make sure to tell ngrok to use port 3000 (which Bolt for Python uses by default):
-
-```
-ngrok http 3000
-```
-
-
-
-For local slack development, we'll use your ngrok URL from above, so copy it to your clipboard.
-
-```
-https://your-own-url.ngrok.io
-```
-
-1. Now we’re going to subscribe to events. Your app can listen to all sorts of events that are happening around your workspace - messages being posted, files being shared and more. On your app configuration page, select the _Event Subscriptions_ sidebar. You'll be presented with an input box to enter a Request URL, which is where Slack sends the events your app is subscribed to. Hit the _save_ button.
-
-By default Bolt for Python listens for all incoming requests at the /slack/events route, so for the Request URL you can enter your ngrok URL appended with /slack/events:
-
-```
-https://your-own-url.ngrok.io/slack/events
-```
-
-If the challenge was successful, you’ll get “verified” right next to the Request URL.
-
-
-
-On the same page click on the `Subscribe to bot events` menu that sits at the bottom of the page. Click on the `Add Bot User Event`.
-
-Similarly, click on `Subscribe to events on behalf of the user but` then click on `Add Workspace Event`.
-
-Add the following scopes
-
-| EventName | Description | Required Scope |
-| ---------------- | --------------------------------- | ----------------- |
-| file_share | A file was shared | files:read |
-| message.channels | A message was posted to a channel | channels: history |
-
-
-
-1. Select the _Interactivity & Shortcuts_ sidebar and toggle the switch as on. Again, for the Request URL, enter your ngrok URL appended with /slack/events:
-
-```
-https://your-own-url.ngrok.io/slack/events
-```
-
-
-
-1. Scroll down to the _Select Menus_ section in the Options Load URL and enter your ngork URL appended with /slack/events:
-
-```
-https://your-own-url.ngrok.io/slack/events
-```
-
-
-
-1. Finally we come to the slash commands. Slack's custom slash commands perform a very simple task. First they take whatever text you enter after the command itself (along with some other predefined values). Next, they’ll send it to a URL and accept whatever the script returns. After this, Slack will post it as a Slackbot message to the person who issued the command. We have 5 slash commands to add in the workspace.
-
-Visit the _Slash Commands_ sidebar and click on the `Create New Command` button to head over the Create New Command page. Add the Command, Request URL, Short Description and Usage hint, according to the table provided below.
-
-Click on Save to return to the _Slash Commands._
-
-| Command | Request URL | Short Description | Usage Hint |
-| ------------------- | ------------------------------------------ | ----------------------------------------- | ---------- |
-| /s3-get | https://your-own-url.ngrok.io/slack/events | Get a file from s3 bucket | filename |
-| /s3-search | https://your-own-url.ngrok.io/slack/events | Search for a file in S3 | |
-| /s3-delete | https://your-own-url.ngrok.io/slack/events | Deletes the given file from the s3 bucket | filename |
-| /summarise-document | https://your-own-url.ngrok.io/slack/events | Summarise a document | filename |
-
-
-
-
-
-
-
-
-
-
-
-1. Open the Slack channel and upload a file in any channel. Make sure to note the file name.
-
-### 6. Setting up a NodeJS backend
-
-#### Requirements
-
-- [GraphicsMagick](https://github.com/redis-developer/Reeko-Slack-Bot/tree/master/nodejs-backend#GraphicsMagick)
-- [Nodejs](https://github.com/redis-developer/Reeko-Slack-Bot/tree/master/nodejs-backend#Nodejs)
-
-#### Getting Started:
-
-
-
-#### GraphicsMagick
-
-GraphicsMagick is a highly versatile piece of software used for image processing. To generate images, you need to have GraphicsMagick installed on your machine.
-
-You can find the suitable release from [http://www.graphicsmagick.org/download.html#download-sites.](http://www.graphicsmagick.org/download.html#download-sites)
-
-
-
-#### Nodejs
-
-:::note
-
-Please follow all the steps in [python-backend/README.md](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/python-backend/README.md) first.
-
-:::
-
-Copy the AWS credentials from the [python-backend/.env](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/python-backend/README.md) to the [config.json](https://github.com/redis-developer/Reeko-Slack-Bot/blob/master/nodejs-backend/src/config/config.json) file.
-
-```
-{
- "accessKeyId": "",
- "secretAccessKey": "",
- "region": ""
-}
-```
-
-Install all the packages and run the server.
-
-```
-npm install
-npm start
-```
-
-
-
-### 7: Connecting Slack to S3
-
-This step involves bringing your AWS S3 bucket to your Slack account. Doing this will allow you to upload, download or delete files from your workspace without writing a single line of code.
-
-#### /S3-get filename
-
-The purpose of this command is to retrieve a specified file from the S3 bucket. Once you type in the name of the file in the search bar, Reeko will check whether this document exists. If the document doesn’t exist then it will return as false and nothing will be done.
-
-If the file is found, then the JSON.GET command will capture its name and download it from the S3 bucket. The downloaded file is sent back as a direct message in Slack.
-
-```
-JSON.GET amazonshareholderletterpdf
-```
-
-
-
-#### /s3-delete filename
-
-This command involves deleting files from the S3 bucket. To achieve this you simply need to type in the file name in the search bar and Reeko will pull up the file as demonstrated below.
-
-You’ll have the option to permanently delete the file from the S3 bucket. The file data is deleted from Redis using the JSON.DEL command and is removed from Search suggestions using the `FT.SUGDEL` command. You’ll be informed when the file is deleted.
-
-```
-FT.SUGDEL file-index "amazon-shareholder-letter.pdf"
-
-JSON.DEL amazonshareholderletterpdf
-```
-
-#### Step 8: File searching
-
-Have you ever searched for a file without being entirely sure what it is you’re looking for? You may remember snippets of the content but not enough to manually track down its location. Well due to Search’s autocomplete functionality this will no longer be a problem.
-
-#### /s3-search
-
-This command first opens up a modal inside of Slack with a search bar. You’ll then be suggested different file names will then be suggested depending on whatever the text you’ve written is. The way this works is simple:
-
-Let’s assume the bucket has documents called `abcd.csv`, `abcd.csv` and `abcdef.sv`. If you type `abc` into the search bar, you’ll get these three results as a list from the `FT.SEARCH` command. From here you’ll be able to select the file you’re looking for. Once selected, the file is downloaded and sent back to Slack.
-
-```
-FT.SEARCH file-index "ama"
-```
-
-#### Step 9: Document summarization
-
-In this step, Reeko will extract all of the text from the documents and summarize the content of each one with an image. This will prevent you from having to tediously open each document to get access to important information. Here’s how to do it:
-
-1. Get the file name from the command['text'] parameter.
-2. If the file is found, you can get the file's name by using the `JSON.GET` command.
-
-```
- JSON.GET amazonshareholderletterpdf
-```
-
-3. Download the pdf or png file locally from S3 bucket
-4. Extract the text using AWS Textract.
-5. The extracted text is summarised using the Hugging face transformers summarisation pipeline. The text summary is also added back to the JSON document using `JSON.SET` command.
-
-```
- JSON.SET amazonshareholderletterpdf .summary ' Amazon has grown from having 158 employees to 614. We had just gone public at a split-adjusted stock price of $1. 50 per share. In 1997, we hadnâ\x80\x99t invented prime, marketplace, alexa, or aws. If you want to be successful in business, you have to create more than you consume. Your goal should be to create value for everyone you interact with. Stock prices are not about the past. They are a prediction of future cash flows discounted back to the present.'
-```
-
-6. A post request is then sent to the /create-image on the nodejs backend with the file name and summary text.
-7. An image is generated using a base template.
-8. The image that is returned is saved to the S3 bucket and sent back to Slack.
-9. The image URL is also added to the JSON document using `JSON.SET` command.
-
-```
- JSON.SET amazonshareholderletterpdf .file_path 'https://bucket-1234.s3.amazonaws.com/b8bac45f-7f69-4c28-a26e-9888d9771bed-image.png'
-```
-
-Below we’ve used the [Amazon 2020 shareholder letter](https://s2.q4cdn.com/299287126/files/doc_financials/2021/ar/Amazon-2020-Shareholder-Letter-and-1997-Shareholder-Letter.pdf) as an example of how a document can be summarized using Reeko.
-
-#### 5. How it works
-
-The Slack app is built using Bolt for Python framework. To connect the AWS S3 bucket and AWS Textract, use their respective [boto3](https://github.com/boto/boto3) clients.
-
-Slack is receptive to all events around your workspace such as messages being posted, files being shared, users joining the team, and more. To listen to events, Slack uses the Events API. And to enable custom interactivity, you can use the Block Kit.
-
-Slash commands work in the following way. First they consider the text you enter after the command itself and then send it to a URL. They then accept whatever the script returns and post it as a Slackbot message to the person who issued the command. There’s a set of 4 slash commands that make the slackbot.
-
-In the application there are two Redis Modules:
-
-- [Redis JSON](https://redis.io/docs/stack/json/) - store file information like filename, summary and image url.
-- [Redis Search and Query](https://redis.io/docs/stack/search/) - searches for files in the S3 bucket
-
-The code below is used to initialize Redis in redisearch_connector.py. This is done by creating an index with the name `file_index.`
-
-```
-from redisearch import Client, TextField, AutoCompleter, Suggestion
-
-class RedisSearchConnector():
- def __init__(self):
- self.index_name = 'file_index'
- self.client = Client(self.index_name)
- self.ac = AutoCompleter(self.index_name)
-```
-
-Use the following code to initialise Redis JSON in redisjson_connector.py.
-
-```
-from rejson import Client, Path
-
-class RedisJsonConnector():
- def __init__(self):
- self.rj = Client(decode_responses=True)
-```
-
-And the code below is used to create an index in Redis
-
-```
-FT.CREATE file-index ON HASH SCHEMA file_name TEXT SORTABLE file_id TEXT created TEXT timestamp TEXT mimetype TEXT filetype TEXT user_id TEXT size
-```
-
-### Conclusion: preventing lost files with Redis
-
-The advanced capabilities of Redis allowed this Launchpad App to create an invaluable asset to remote workers - to never lose a file again on Slack. Redis Stack offered a simple yet effective way of transmitting data to and from the S3 bucket with no lags, no pauses and no delays whatsoever. You can discover more about the ins and outs of how this app was made by simply [clicking here](https://launchpad.redis.com/?id=project%3AReeko-Slack-Bot).
-
-Reeko is an innovative application that joins our _exciting_ collection of apps that we currently have on the [Redis Launchpad](https://launchpad.redis.com/). By using Redis, programmers from all over the world are creating breakthrough applications that are having an impact on daily lives… _and you can too_.
-
-So how can you change the world using Redis? For more inspiration, make sure to check out the other applications we have on our [Launchpad](https://launchpad.redis.com/).
-
-
-
-### Who built this application?
-
-#### Sarthak Arora
-
-Being only 20 years old, Sarthak is a young yet highly-advanced programmer who’s already a 2x international Hacong winner.
-
-To discover more about his work and his activity on GitHub, you can [check out his profile here](https://github.com/sarthakarora1208).
-
-### References
-
-- [Create Redis database on AWS](/create/aws/redis-on-aws)
diff --git a/docs/games/spider/terraform/accessmanagement.png b/docs/games/spider/terraform/accessmanagement.png
deleted file mode 100644
index d3ab123..0000000
Binary files a/docs/games/spider/terraform/accessmanagement.png and /dev/null differ
diff --git a/docs/games/spider/terraform/accessmanagement1.png b/docs/games/spider/terraform/accessmanagement1.png
deleted file mode 100644
index d935e06..0000000
Binary files a/docs/games/spider/terraform/accessmanagement1.png and /dev/null differ
diff --git a/docs/games/spider/terraform/index-terraform.mdx b/docs/games/spider/terraform/index-terraform.mdx
deleted file mode 100644
index df0b47e..0000000
--- a/docs/games/spider/terraform/index-terraform.mdx
+++ /dev/null
@@ -1,552 +0,0 @@
----
-id: index-terraform
-title: How to Deploy and Manage Redis Database on AWS Using Terraform
-sidebar_label: Deploy and Manage Redis Database on AWS using Terraform
-slug: /create/aws/terraform
-authors: [ajeet, rahul]
----
-
-
-
-Development teams today are embracing more and more DevOps principles, such as continuous integration and continuous delivery (CI/CD). Therefore, the need to manage infrastructure-as-code (IaC) has become an essential capability for any cloud service. IaC tools allow you to manage infrastructure with configuration files rather than through a graphical user interface. IaC allows you to build, change, and manage your infrastructure in a safe, consistent, and repeatable way by defining resource configurations that you can version, reuse, and share.
-A leading tool in the IaC is HashiCorp Terraform, which supports the major cloud providers and services with its providers and modules cloud infrastructure automation ecosystem for provisioning, compliance, and management of any cloud, infrastructure, and service.
-
-### What is Terraform?
-
-Terraform is an open source IaC software tool that provides a consistent CLI workflow to manage hundreds of cloud services. Terraform codifies cloud APIs into declarative configuration files, which can then be shared amongst team members, treated as code, edited, reviewed, and versioned. It enables you to safely and predictably create, change, and improve infrastructure.
-
-### Capabilities of Terraform
-
-- Terraform is not just a configuration management tool. It also focuses on the higher-level abstraction of the data center and associated services, while allowing you to use configuration management tools on individual systems.
-- It supports multiple cloud providers, such as AWS, GCP, Azure, DigitalOcean, etc.
-- It provides a single unified syntax, instead of requiring operators to use independent and non-interoperable tools for each platform and service.
-- Manages both existing service providers and custom in-house solutions.
-- Terraform is easily portable to any other provider.
-- Provides immutable infrastructure where configuration changes smoothly.
-- Supports client-only architecture, so no need for additional configuration management on a server.
-- Terraform is very flexible, using a plugin-based model to support providers and provisioners, giving it the ability to support almost any service that exposes APIs.
-- It is not intended to give low-level programmatic access to providers, but instead provides a high-level syntax for describing how cloud resources and services should be created, provisioned, and combined.
-- It provides a simple, unified syntax, allowing almost any resource to be managed without learning new tooling.
-
-### The HashiCorp Terraform Redis Enterprise Cloud provider
-
-Redis has developed a Terraform provider for Redis Enterprise Cloud. The HashiCorp Terraform Redis Enterprise Cloud provider allows customers to deploy and manage Redis Enterprise Cloud subscriptions, databases, and network peering easily as code, on any cloud provider. It is a plugin for Terraform that allows Redis Enterprise Cloud Flexible customers to manage the full life cycle of their subscriptions and related Redis databases.
-The Redis Enterprise Cloud provider is used to interact with the resources supported by Redis Enterprise Cloud. The provider needs to be configured with the proper credentials before it can be used. Use the navigation to the left to read about the available provider resources and data sources.
-
-
-
-Before we jump into the implementation, let us take a moment to better understand the Terraform configuration. A Terraform configuration is a complete document in the Terraform language that tells Terraform how to manage a given collection of infrastructure. A configuration can consist of multiple files and directories. Terraform is broken down into three main components:
-
-- Providers
-- Data sources
-- Resources
-
-### Providers
-
-A provider is the first resource that will need to be defined in any project under the Terraform configuration file. The provider gives you access to the API you will be interacting with to create resources. Once the provider has been configured and authenticated, a vast amount of resources are now available to be created. Terraform has more than 100+ cloud providers it serves.
-A provider defines resources and data for a particular infrastructure, such as AWS. As shown below, the terraform block {} contains terraform settings, including the required providers Terraform will use to provision your infrastructure (for example, rediscloud provider).
-
-```
- terraform {
- required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
- }
- }
-```
-
-The provider {} block configures the specific provider. In the below example, it is AWS.
-
-```
- cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
- }
-```
-
-### Resources
-
-Resources are the most important element in the Terraform language. This is where you describe the piece of infrastructure to be created, and this can range from a compute instance to defining specific permissions and much more.
-
-As shown below, the resource {} block is used to define components of your infrastructure. A resource might be a physical or virtual component, such as EC2, or it could be a logical component, such as a Heroku application.
-
-```
- resource "random_password" "passwords" {
- count = 2
- length = 20
- upper = true
- lower = true
- number = true
-}
-```
-
-The resource {} block has two strings before the block: resource types and resource names. The prefix of the type maps to the name of the provider. For example, the resource type “random_password” and the resource name “passwords” form a unique identifier of the resource. Terraform uses this ID to identify the resource.
-
-### Data sources
-
-Data sources allow Terraform to use information defined outside of Terraform, defined by another separate Terraform configuration, or modified by functions. Each provider may offer data sources alongside its set of resource types. A data source is accessed via a special kind of resource known as a data resource, declared using a data block.
-
-```
- data "rediscloud_payment_method" "card" {
- card_type = "Visa"
- last_four_numbers = "XXXX"
- }
-```
-
-A data block requests that Terraform read from a given data source ("rediscloud_payment_method") and export the result under the given local name ("card"). The name is used to refer to this resource from elsewhere in the same Terraform module, but has no significance outside of the scope of a module.
-Within the block body (between { and }) are query constraints defined by the data source. Most arguments in this section depend on the data source, and indeed in this example card_type and last_four_numbers are all arguments defined specifically for the rediscloud_payment_method data source.
-
-### Configure Redis Enterprise Cloud programmatic access
-
-In order to set up authentication with the Redis Enterprise Cloud provider, a programmatic API key must be generated for Redis Enterprise Cloud. The Redis Enterprise Cloud documentation contains the most up-to-date instructions for creating and managing your key(s) and IP access.
-
-:::note
-
-Flexible and Annual Redis Enterprise Cloud subscriptions can leverage a RESTful API that permits operations against a variety of resources, including servers, services, and related infrastructure. The REST API is not supported for Fixed or Free subscriptions.
-
-:::
-
-```
- provider "rediscloud" { } # Example resource configuration
- resource "rediscloud_subscription" "example" { # ... }
-```
-
-### Prerequisites:
-
-- Install Terraform on MacOS.
-- Create a free Redis Enterprise Cloud account.
-- Create your first subscription.
-- Enable API
-
-### Step 1: Install Terraform on MacOS
-
-Use Homebrew to install Terraform on MacOS as shown below:
-
-```
- brew install terraform
-```
-
-### Step 2: Sign up for a free Redis Enterprise Cloud account
-
-[Follow this tutorial](https://developer.redis.com/create/aws/redis-on-aws) to sign up for free Redis Enterprise Cloud account.
-
-
-
-### Step 3: Enable Redis Enterprise Cloud API
-
-If you have a Flexible (or Annual) Redis Enterprise Cloud subscription, you can use a REST API to manage your subscription programmatically. The Redis Cloud REST API is available only to Flexible or Annual subscriptions. It is not supported for Fixed or Free subscriptions.
-
-For security reasons, the Redis Cloud API is disabled by default. To enable the API:
-Sign in to your Redis Cloud subscription as an account owner.
-From the menu, choose Access Management.
-
-When the Access Management screen appears, select the API Keys tab.
-
-
-
-If a Copy button appears to the right of the API account key, the API is enabled. This button copies the account key to the clipboard.
-
-If you see an Enable API button, select it to enable the API and generate your API account key.
-
-To authenticate REST API calls, you need to combine the API account key with an API user key to make API calls.
-
-
-
-### Step 4: Create a main.tf file
-
-It’s time to create an empty “main.tf” file and start adding the provider, resource and data sources as shown below:
-
-```
- terraform {
- required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
- }
- }
-# Provide your credit card details
-data "rediscloud_payment_method" "card" {
-card_type = "Visa"
-last_four_numbers = "XXXX"
-}
-# Generates a random password for the database
-resource "random_password" "passwords" {
-count = 2
-length = 20
-upper = true
-lower = true
-number = true
-special = false
-}
-resource "rediscloud_subscription" "rahul-test-terraform" {
-name = "rahul-test-terraform"
-payment_method_id = data.rediscloud_payment_method.card.id
-memory_storage = "ram"
-cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
-}
-database {
- name = "db-json"
- protocol = "redis"
- memory_limit_in_gb = 1
- replication = true
- data_persistence = "aof-every-1-second"
- module {
- name = "RedisJSON"
- }
- throughput_measurement_by = "operations-per-second"
- throughput_measurement_value = 10000
- password = random_password.passwords[1].result
-}
-}
-```
-
-### Step 5: Create an execution plan
-
-The Terraform plan command creates an execution plan, which lets you preview the changes that Terraform plans to make to your infrastructure. By default, when Terraform creates a plan, it reads the current state of any already existing remote objects to make sure that Terraform state is up to date. It then compares the current configuration to the prior state and then proposes a set of change actions that should make the remote object match the configuration.
-
-```
- % terraform plan
-
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- + create
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # random_password.passwords[1] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # rediscloud_subscription.rahul-test-terraform will be created
- + resource "rediscloud_subscription" "rahul-test-terraform" {
- + id = (known after apply)
- + memory_storage = "ram"
- + name = "rahul-test-terraform"
- + payment_method_id = "XXXX"
- + persistent_storage_encryption = true
-
- + cloud_provider {
- + cloud_account_id = "1"
- + provider = "AWS"
-
- + region {
- + multiple_availability_zones = false
- + networking_deployment_cidr = "10.0.0.0/24"
- + networks = (known after apply)
- + preferred_availability_zones = [
- + "us-east-1a",
- ]
- + region = "us-east-1"
- }
- }
-
- + database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 3 to add, 0 to change, 0 to destroy.
-
-───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────
-
-Note: You didn't use the -out option to save this plan, so Terraform can't guarantee to take exactly these actions if you run "terraform apply" now.
-```
-
-### Step 6: Execute the action
-
-The Terraform apply command executes the actions proposed in a Terraform plan.
-
-```
- terraform apply
-
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- + create
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # random_password.passwords[1] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # rediscloud_subscription.rahul-test-terraform will be created
- + resource "rediscloud_subscription" "rahul-test-terraform" {
- + id = (known after apply)
- + memory_storage = "ram"
- + name = "rahul-test-terraform"
- + payment_method_id = "XXXX"
- + persistent_storage_encryption = true
-
- + cloud_provider {
- + cloud_account_id = "1"
- + provider = "AWS"
-
- + region {
- + multiple_availability_zones = false
- + networking_deployment_cidr = "10.0.0.0/24"
- + networks = (known after apply)
- + preferred_availability_zones = [
- + "us-east-1a",
- ]
- + region = "us-east-1"
- }
- }
-
- + database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 3 to add, 0 to change, 0 to destroy.
-
-Do you want to perform these actions?
- Terraform will perform the actions described above.
- Only 'yes' will be accepted to approve.
-
- Enter a value: yes
-
-random_password.passwords[0]: Creating...
-random_password.passwords[1]: Creating...
-random_password.passwords[1]: Creation complete after 0s [id=none]
-random_password.passwords[0]: Creation complete after 0s [id=none]
-rediscloud_subscription.rahul-test-terraform: Creating...
-rediscloud_subscription.rahul-test-terraform: Still creating... [10s elapsed]
-rediscloud_subscription.rahul-test-terraform: Still creating... [20s elapsed]
-rediscloud_subscription.rahul-test-terraform: Creation complete after 8m32s [id=1649277]
-
-Apply complete! Resources: 3 added, 0 changed, 0 destroyed.
-```
-
-### Step 7: Verify the database
-
-You can now verify the new database created under Subscription named “db-json.”
-
-Deploy a Redis Database with Redis JSON modules on AWS using Terraform:
-
-```
-terraform {
-required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
-}
-}
-# Provide your credit card details
-data "rediscloud_payment_method" "card" {
-card_type = "Visa"
-last_four_numbers = "XXXX"
-}
-# Generates a random password for the database
-resource "random_password" "passwords" {
-count = 2
-length = 20
-upper = true
-lower = true
-number = true
-special = false
-}
-resource "rediscloud_subscription" "rahul-test-terraform" {
-name = "rahul-test-terraform"
-payment_method_id = data.rediscloud_payment_method.card.id
-memory_storage = "ram"
-cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
-}
-database {
- name = "db-json"
- protocol = "redis"
- memory_limit_in_gb = 1
- replication = true
- data_persistence = "aof-every-1-second"
- module {
- name = "RedisJSON"
- }
- throughput_measurement_by = "operations-per-second"
- throughput_measurement_value = 10000
- password = random_password.passwords[1].result
-}
-}
-```
-
-### Step 8: Cleanup
-
-The Terraform destroy command is a convenient way to destroy all remote objects managed by a particular Terraform configuration. While you will typically not want to destroy long-lived objects in a production environment, Terraform is sometimes used to manage ephemeral infrastructure for development purposes, in which case you can use terraform destroy’ to conveniently clean up all of those temporary objects once you are finished with your work.
-
-```
-% terraform destroy
-random_password.passwords[0]: Refreshing state... [id=none]
-random_password.passwords[1]: Refreshing state... [id=none]
-rediscloud_subscription.rahul-test-terraform: Refreshing state... [id=1649277]
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- - destroy
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be destroyed
- - resource "random_password" "passwords" {
- - id = "none" -> null
- - length = 20 -> null
- - lower = true -> null
- - min_lower = 0 -> null
- - min_numeric = 0 -> null
- - min_special = 0 -> null
- - min_upper = 0 -> null
- - number = true -> null
- - result = (sensitive value)
- - special = false -> null
- - upper = true -> null
- }
-
- # random_password.passwords[1] will be destroyed
- - resource "random_password" "passwords" {
- - id = "none" -> null
- - length = 20 -> null
- - lower = true -> null
- - min_lower = 0 -> null
- - min_numeric = 0 -> null
- - min_special = 0 -> null
- - min_upper = 0 -> null
- - number = true -> null
- - result = (sensitive value)
- - special = false -> null
- - upper = true -> null
- }
-
- # rediscloud_subscription.rahul-test-terraform will be destroyed
- - resource "rediscloud_subscription" "rahul-test-terraform" {
- - id = "1649277" -> null
- - memory_storage = "ram" -> null
- - name = "rahul-test-terraform" -> null
- - payment_method_id = "XXXX" -> null
- - persistent_storage_encryption = true -> null
-
- - cloud_provider {
- - cloud_account_id = "1" -> null
- - provider = "AWS" -> null
-
- - region {
- - multiple_availability_zones = false -> null
- - networking_deployment_cidr = "10.0.0.0/24" -> null
- - networks = [
- - {
- - networking_deployment_cidr = "10.0.0.0/24"
- - networking_subnet_id = "subnet-0055e8e3ee3ea796e"
- - networking_vpc_id = ""
- },
- ] -> null
- - preferred_availability_zones = [
- - "us-east-1a",
- ] -> null
- - region = "us-east-1" -> null
- }
- }
-
- - database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 0 to add, 0 to change, 3 to destroy.
-
-Do you really want to destroy all resources?
- Terraform will destroy all your managed infrastructure, as shown above.
- There is no undo. Only 'yes' will be accepted to confirm.
-
- Enter a value: yes
-
-rediscloud_subscription.rahul-test-terraform: Destroying... [id=1649277]
-…
-rediscloud_subscription.rahul-test-terraform: Destruction complete after 1m34s
-random_password.passwords[0]: Destroying... [id=none]
-random_password.passwords[1]: Destroying... [id=none]
-random_password.passwords[0]: Destruction complete after 0s
-random_password.passwords[1]: Destruction complete after 0s
-
-Destroy complete! Resources: 3 destroyed.
-```
-
-### Further References:
-
-- [Provision and Manage Redis Enterprise Cloud Anywhere with HashiCorp Terraform](https://redis.com/blog/provision-manage-redis-enterprise-cloud-hashicorp-terraform/)
-- [The HashiCorp Terraform Redis Enterprise Cloud provider](https://registry.terraform.io/providers/RedisLabs/rediscloud/latest)
diff --git a/docs/games/spider/terraform/newsubscription.png b/docs/games/spider/terraform/newsubscription.png
deleted file mode 100644
index b69d9c5..0000000
Binary files a/docs/games/spider/terraform/newsubscription.png and /dev/null differ
diff --git a/docs/games/spider/terraform/subscription.png b/docs/games/spider/terraform/subscription.png
deleted file mode 100644
index 6016836..0000000
Binary files a/docs/games/spider/terraform/subscription.png and /dev/null differ
diff --git a/docs/games/spider/terraform/subscription2.png b/docs/games/spider/terraform/subscription2.png
deleted file mode 100644
index 9be6010..0000000
Binary files a/docs/games/spider/terraform/subscription2.png and /dev/null differ
diff --git a/docs/games/spider/terraform/terraform_arch.png b/docs/games/spider/terraform/terraform_arch.png
deleted file mode 100644
index 4ac779e..0000000
Binary files a/docs/games/spider/terraform/terraform_arch.png and /dev/null differ
diff --git a/docs/games/spider/terraform/terraform_rediscloud.png b/docs/games/spider/terraform/terraform_rediscloud.png
deleted file mode 100644
index 6699e7a..0000000
Binary files a/docs/games/spider/terraform/terraform_rediscloud.png and /dev/null differ
diff --git a/docs/games/spider/terraform/tryfree.png b/docs/games/spider/terraform/tryfree.png
deleted file mode 100644
index d5188c7..0000000
Binary files a/docs/games/spider/terraform/tryfree.png and /dev/null differ
diff --git a/docs/games/spider/terraform/verifydatabase.png b/docs/games/spider/terraform/verifydatabase.png
deleted file mode 100644
index da78e22..0000000
Binary files a/docs/games/spider/terraform/verifydatabase.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.jpg b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.jpg
deleted file mode 100644
index 4720d9b..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.jpg and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.png
deleted file mode 100644
index b838ccb..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview1.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview1.png
deleted file mode 100644
index 650bab2..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argo_preview1.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_1.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_1.png
deleted file mode 100644
index 4c021a4..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_1.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_10.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_10.png
deleted file mode 100644
index 70a0707..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_10.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_11.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_11.png
deleted file mode 100644
index 453d298..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_11.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_12.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_12.png
deleted file mode 100644
index c4f4957..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_12.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_13.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_13.png
deleted file mode 100644
index 498f68f..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_13.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_14.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_14.png
deleted file mode 100644
index 2c528db..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_14.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_15.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_15.png
deleted file mode 100644
index 15ecc03..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_15.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_16.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_16.png
deleted file mode 100644
index e2d5b24..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_16.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_17.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_17.png
deleted file mode 100644
index 5fc3bb2..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_17.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_18.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_18.png
deleted file mode 100644
index 5fc3bb2..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_18.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_2.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_2.png
deleted file mode 100644
index be0e60b..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_2.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_21.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_21.png
deleted file mode 100644
index 6d1b118..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_21.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_3.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_3.png
deleted file mode 100644
index 6506e00..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_3.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_4.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_4.png
deleted file mode 100644
index d6c5b6e..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_4.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_5.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_5.png
deleted file mode 100644
index 34401e0..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_5.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_6.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_6.png
deleted file mode 100644
index 758ddc4..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_6.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_7.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_7.png
deleted file mode 100644
index 305027b..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_7.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_8.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_8.png
deleted file mode 100644
index c46f8a1..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_8.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_9.png b/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_9.png
deleted file mode 100644
index cf1e28c..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/argocd/images/argocd_9.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/argocd/index-argocd.mdx b/docs/support/_continuous-integration-continuous-deployment/argocd/index-argocd.mdx
deleted file mode 100644
index 3412c7e..0000000
--- a/docs/support/_continuous-integration-continuous-deployment/argocd/index-argocd.mdx
+++ /dev/null
@@ -1,246 +0,0 @@
----
-id: index-argocd
-title: 'Argo CD: What It Is And Why It Should Be Part of Your Redis CI/CD Pipeline'
-sidebar_label: Redis using Argo CD
-slug: /operate/continuous-integration-continuous-deployment/argocd
-authors: [ajeet, talon]
----
-
-
-
-
-
-## What is an Argo CD?
-
-Argo CD is a combination of the two terms “Argo” and “CD,” [Argo](https://argoproj.github.io/) being an open source container-native workflow engine for Kubernetes. It is a [CNCF-hosted project](https://www.cncf.io/blog/2020/04/07/toc-welcomes-argo-into-the-cncf-incubator/) that provides an easy way to combine all three modes of computing—services, workflows, and event-based—all of which are very useful for creating jobs and applications on Kubernetes. It is an engine that makes it easy to specify, schedule, and coordinate the running of complex workflows and applications on Kubernetes. The CD in the name refers to [continuous delivery](https://en.wikipedia.org/wiki/Continuous_delivery), which is an extension of continuous integration (CI) since it automatically deploys all code changes to a testing and/or production environment after the build stage.
-
-
-
-Argo CD follows [GitOps methodology](https://about.gitlab.com/topics/gitops/). GitOps is a CD methodology centered around using [Git as a single source of truth](https://git-scm.com/) for declarative infrastructure and application code. It watches a remote Git repository for new or updated manifest files and synchronizes those changes with the cluster. By managing manifests in Git and syncing them with the cluster, you get all the advantages of a Git-based workflow (version control, pull-request reviews, transparency in collaboration, etc.) and a one-to-one mapping between what is in the Git repo and what is deployed in the cluster.
-
-Argo CD empowers organizations to declaratively build and run cloud native applications and workflows on Kubernetes using GitOps. It is a pull-based, declarative, GitOps continuous delivery tool for Kubernetes with a[ fully loaded UI](https://github.com/argoproj/argo-cd/tree/master/ui). The tool reads your environment configuration from your Git repository and applies it to your Kubernetes namespaces. App definitions, environment, and configurations should be declarative and version controlled. App deployment and life cycle management should be automated, audible, and easy to understand.
-
-Built specifically to make the continuous deployment process to a Kubernetes cluster simpler and more efficient, Argo CD solves multiple challenges, such as the need to set up and install additional tools outside of Jenkins for a complete CI/CD process to Kubernetes, the need to configure access control to Kubernetes in and out (including cloud platforms), the need to have visibility of deployment status once a new app gets pushed to a Kubernetes cluster, and more.
-
-:::note
-
-Argo CD isn’t just deployed inside of Kubernetes but should be looked at as an extension of Kubernetes as it uses existing Kubernetes resources and functionalities like etcd and controllers to store data and monitor real-time updates of application state.
-
-:::
-
-## How does Argo CD work?
-
-
-
-It solves the problems above in the CD process by being a more integral part of the Kubernetes cluster. Instead of pushing changes to the Kubernetes cluster, Argo CD pulls Kubernetes manifest changes and applies them to the cluster. Once you set up Argo CD inside of your Kubernetes cluster, you configure Argo CD to connect and track your Git repo changes.
-
-If any changes are detected, then Argo CD applies those changes automatically to the cluster. Now developers can commit code (for example, Jenkins), which will automatically build a new image, push it to the docker repo, and then finally update the Kubernetes manifest file that will be automatically pulled by Argo CD, ultimately saving manual work, reducing the initial setup configuration, and eliminating security risks. But what about DevOps teams making other changes to the application configuration? Whatever manifest files connected to the Git repo will be tracked and synced by Argo CD and pulled into the Kubernetes cluster, providing a single flexible deployment tool for developers and DevOps.
-
-Argo CD watches the changes in the cluster as well, which becomes important if someone updates the cluster manually. Argo CD will detect the divergence of states between the cluster and Git repo. Argo CD compares desired configuration in the Git repo with the actual state in the Kubernetes cluster and syncs what is defined in the Git repo to the cluster, overriding whatever update was done manually—guaranteeing that the Git repo remains the single source of truth. But, of course, Argo CD is flexible enough to be configured to not automatically override manual updates if a quick update needs to happen directly to the cluster and an alert be sent instead.
-
-## **What are Argo CD’s capabilities?**
-
-- Argo CD is a very simple and efficient way to have declarative and version-controlled application deployments with its automatic monitoring and pulling of manifest changes in the Git repo, but it also has easy rollback and reverts to the previous state, not manually reverting every update in the cluster.
-
-- It also provides automation and traceability via GitOps workflow with a web UI for visualizing Kubernetes resources.
-
-- Argo CD defines Kubernetes manifests in different ways: it supports Kubernetes YAML files, Helm Charts, Kustomize, and other template files that generate Kubernetes manifests.
-
-- Argo CD also has a command-line interface application, a Grafana metrics dashboard, and audit trails for application events and API calls.
-
-- Argo CD has a very simple cluster disaster recovery process whereby pointing a new cluster to the Git repo if a cluster has gone down will automatically recreate the same exact state without any intervention because of its declarative nature.
-
-- Kubernetes cluster access control with Git and Argo CD is simple—you can configure who can commit merge requests and who can actually approve those merge requests, providing a clean way to manage cluster access indirectly via Git. There is also no need to give external access to other tools like Jenkins, keeping cluster credentials safe because Argo CD is already running in the cluster and is the only tool that actually applies changes.
-
-## Prerequisites:
-
-- Install Docker Desktop
-- Enable Kubernetes
-- Install Argo CD
-
-## Getting Started
-
-### Step 1. Install Argo CD
-
-```
-brew install argocd
-```
-
-### Step 2. Create a new namespace
-
-Create a namespace argocd where all Argo CD resources will be installed.
-
-```
-kubectl create namespace argocd
-```
-
-### Step 3. Install Argo CD resources
-
-```
-kubectl apply -n argocd -f https://raw.githubusercontent.com/argoproj/argo-cd/stable/manifests/install.yaml
-
-kubectl get po -n argocd
-NAME READY STATUS RESTARTS AGE
-argocd-application-controller-0 0/1 ContainerCreating 0 3m9s
-argocd-dex-server-65bf5f4fc7-5kjg6 0/1 Init:0/1 0 3m13s
-argocd-redis-d486999b7-929q9 0/1 ContainerCreating 0 3m13s
-argocd-repo-server-8465d84869-rpr9n 0/1 Init:0/1 0 3m12s
-argocd-server-87b47d787-gxwlb 0/1 ContainerCreating 0 3m11s
-```
-
-### Step 4. Ensure that all Pods are up and running
-
-```
-kubectl get po -n argocd
-NAME READY STATUS RESTARTS AGE
-argocd-application-controller-0 1/1 Running 0 5m25s
-argocd-dex-server-65bf5f4fc7-5kjg6 1/1 Running 0 5m29s
-argocd-redis-d486999b7-929q9 1/1 Running 0 5m29s
-argocd-repo-server-8465d84869-rpr9n 1/1 Running 0 5m28s
-argocd-server-87b47d787-gxwlb 1/1 Running 0 5m27s
-```
-
-### Step 5. Configure Port Forwarding for Dashboard Access
-
-```
-kubectl port-forward svc/argocd-server -n argocd 8080:443
-Forwarding from 127.0.0.1:8080 -> 8080
-Forwarding from [::1]:8080 -> 8080
-```
-
-
-
-### Step 6. Log in
-
-```
-kubectl -n argocd get secret argocd-initial-admin-secret -o jsonpath="{.data.password}" | base64 -d; echo
-
-HcD1I0XXXXXQVrq-
-```
-
-
-
-### Step 7. Install Argo CD CLI on Mac using Homebrew
-
-```
-brew install argocd
-```
-
-### Step 8. Access the Argo CD API Server
-
-By default, the Argo CD API server is not exposed with an external IP. To access the API server, choose one of the following techniques to expose the Argo CD API server:
-
-```
-kubectl patch svc argocd-server -n argocd -p '{"spec": {"type": "LoadBalancer"}}'
-service/argocd-server patched
-```
-
-### Step 9. Log in to Argo CD
-
-```
-argocd login localhost
-WARNING: server certificate had error: x509: certificate signed by unknown authority. Proceed insecurely (y/n)? y
-Username: admin
-Password:
-'admin:login' logged in successfully
-Context 'localhost' updated
-```
-
-### Step 10. Update the password
-
-```
-% argocd account update-password
-*** Enter password of currently logged in user (admin):
-*** Enter new password for user admin:
-*** Confirm new password for user admin:
-Password updated
-Context 'localhost' updated
-ajeetraina@Ajeets-MacBook-Pro ~ %
-```
-
-### Step 11. Register a Cluster to Deploy Apps to
-
-As we are running it on Docker Desktop, we will add it accordingly.
-
-```
-argocd cluster add docker-desktop
-WARNING: This will create a service account `argocd-manager` on the cluster referenced by context `docker-desktop` with full cluster level admin privileges. Do you want to continue [y/N]? y
-INFO[0002] ServiceAccount "argocd-manager" created in namespace "kube-system"
-INFO[0002] ClusterRole "argocd-manager-role" created
-INFO[0002] ClusterRoleBinding "argocd-manager-role-binding" created
-Cluster 'https://kubernetes.docker.internal:6443' added
-```
-
-### Step 12. Create a New Redis application
-
-Click “Create” and provide a repository URL as [https://github.com/argoproj/argo-cd/tree/master/manifests/base/redis](https://github.com/argoproj/argo-cd/tree/master/manifests/base/redis)
-
-
-
-
-
-```
-argocd app list
-NAME CLUSTER NAMESPACE PROJECT STATUS HEALTH SYNCPOLICY CONDITIONS REPO PATH TARGET
-redisdemo https://kubernetes.docker.internal:6443 argocd default Synced Healthy https://github.com/argoproj/argo-cd manifests/base/redis HEAD
-
-
-```
-
-
-
-
-
-
-
-### Step 13. Delete the Redis app
-
-```
-argocd app delete redisdemo
-```
-
-#### Example: Voting App
-
-Let’s try to deploy a voting app. The voting application only accepts one vote per client. It does not register votes if a vote has already been submitted from a client.
-
-
-
-- A Python web app which lets you vote between two options
-- A Redis queue which collects new votes
-- A .NET worker which consumes votes and stores them in …
-- A Postgres database backed by a Docker volume
-- A Node.js web app which shows the results of the voting in real time
-
-Go to the Argo CD dashboard, enter the [repository URL](https://github.com/dockersamples/example-voting-app/tree/master/k8s-specifications), and supply the right PATH. Click “Create App” to deploy the application on your Docker Desktop.
-
-
-
-Next, visualize the complete application by choosing “Group by Node.”
-
-
-
-### Step 14. Grouping by Parent Resources
-
-
-
-Keep a close watch over the events by clicking on “Events” section.
-
-
-
-Below is the complete overview of the voting application.
-
-
-
-Access the app via [http://localhost:31000/](http://localhost:31000/)
-
-
-
-The results are accessible via [http://localhost:31001/](http://localhost:31001/)
-
-
-
-### Additional References:
-
-- [https://argo-cd.readthedocs.io/en/stable/](https://argo-cd.readthedocs.io/en/stable/)
-- [https://github.com/argoproj/argo-cd](https://github.com/argoproj/argo-cd)
-- [https://blog.argoproj.io/doing-gitops-at-scale-6313f5889775](https://blog.argoproj.io/doing-gitops-at-scale-6313f5889775)
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-auth.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-auth.png
deleted file mode 100644
index 785fc96..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-auth.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config.png
deleted file mode 100644
index 4310637..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config2.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config2.png
deleted file mode 100644
index e60fab6..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-config2.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-demoapp.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-demoapp.png
deleted file mode 100644
index 2298de6..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-demoapp.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-login.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-login.png
deleted file mode 100644
index e2a50f1..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-login.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-merge.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-merge.png
deleted file mode 100644
index b617c4e..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-merge.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-proj.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-proj.png
deleted file mode 100644
index ef3df1a..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-proj.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-redis.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-redis.png
deleted file mode 100644
index 12b1341..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circleci-redis.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circlecidiagram.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/circlecidiagram.png
deleted file mode 100644
index 311413a..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/circlecidiagram.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-envir.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-envir.png
deleted file mode 100644
index 7383c25..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-envir.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-setup.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-setup.png
deleted file mode 100644
index da28f04..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-setup.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-trigger.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-trigger.png
deleted file mode 100644
index 3a81b72..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/heroku-trigger.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/images/rate-limiting-example.png b/docs/support/_continuous-integration-continuous-deployment/circleci/images/rate-limiting-example.png
deleted file mode 100644
index 1b6657e..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/circleci/images/rate-limiting-example.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/circleci/index-circleci.mdx b/docs/support/_continuous-integration-continuous-deployment/circleci/index-circleci.mdx
deleted file mode 100644
index 7b62550..0000000
--- a/docs/support/_continuous-integration-continuous-deployment/circleci/index-circleci.mdx
+++ /dev/null
@@ -1,169 +0,0 @@
----
-id: index-circleci
-title: 'CircleCI: What It Is and Why It Should Be Part of Your Redis CI/CD'
-sidebar_label: Redis using CircleCI
-slug: /operate/continuous-integration-continuous-deployment/circleci
-authors: [talon, ajeet]
----
-
-
-
-
-
-## **What is CircleCI?**
-
-
-
-CircleCI is a CI/CD platform built by DevOps professionals to help you fine-tune your entire development process from start to finish. It helps engineering teams build, test, and deploy software while checking code changes in real time with the CircleCI dashboard UI. More control over pipelines is possible with the ability to build in the CI/CD process per project or configure workflows to decide on when and how jobs run, plus data- and image-caching options that optimize continuous delivery.
-
-CircleCI supports many different languages and a varied amount of cloud-hosted compute types such as Docker, Linux VMs, macOS, Windows, and more for a simplified approach to infrastructure. With the CircleCI dashboard, it's possible to gather insights on build changes to continuously optimize pipelines.
-
-To deploy, CircleCI takes artifacts from your pipeline and sends them to wherever you need them deployed, whether it's Docker, Heroku, Kubernetes, cloud platforms, and more.
-
-## **How does CircleCI work?**
-
-
-
-After GitHub or Bitbucket are authorized and added as a project to circleci.com, every code change triggers CircleCI jobs. CircleCI sends an email notification of success or failure after the test completes. CircleCI finds and runs `config.yml`, tests the build, runs security scans, goes through approval steps, and then deploys.
-
-## **What are CircleCI’s capabilities?**
-
-As a DevOps engineer or developer, you can:
-
-- SSH into any job to debug build issues.
-- Set up jobs to run in parallel to reduce time.
-- Configure a Redis cache with two simple keys to reuse data from previous jobs in your workflow.
-- Configure self-hosted runners for unique platform support.
-- Access Arm resources for the machine executor.
-- Use reusable packages of configuration to integrate with third parties.
-- Use a pre-built Redis Docker image in a variety of languages.
-- Use the API to retrieve information about jobs and workflows.
-- Use the CLI to access advanced tools locally.
-- Get flaky test detection with test insights.
-
-## **Deploy a Redis Rate Limiting application on Heroku using CircleCI**
-
-## **Prerequisites:**
-
-- A CircleCI account
-- A GitHub account
-- A Heroku account
-
-## **Getting started**
-
-In this demo, we will be using the Redis Rate Limiting app built using Python and Redis.
-
-Rate limiting is a mechanism that many developers may have to deal with at some point in their life. It’s useful for a variety of purposes, such as sharing access to limited resources or limiting the number of requests made to an API endpoint and responding with a 429 status code. The complete source code of the project is [hosted over at GitHub](https://github.com/redis-developer/basic-rate-limiting-demo-python). In this example, we will configure CircleCI to deploy the Rate Limiting app directly on the Heroku platform.
-
-## Step 1. Log in to CircleCI
-
-Go to [https://circleci.com](https://circleci.com) and log in using your GitHub account:
-
-
-
-Choose your preferred login method. To make it easier, let us choose “GitHub” for this demonstration.
-
-## Step 2. Verify your permissions on GitHub
-
-
-
-## Step 3. Select your project repository and click “Setup Project”
-
-We will be using the Redis rate-limiting project for this demonstration.
-
-
-
-## Step 4. Create a new CircleCI configuration file
-
-CircleCI believes in _configuration as code_. As a result, the entire delivery process from build to deploy is orchestrated through a single file called `config.yml`. The `config.yml` file is located in a folder called `.circleci` at the top of your project. CircleCI uses the YAML syntax for config.
-
-
-
-As we haven’t yet created a `config.yml` file, let’s choose the “Fast” option to create a new `config.yml` based on the available template that is editable.
-
-
-
-Once you click “Set Up Project” it will ask you to select sample configs as shown in the following screenshot:
-
-
-
-Add the following content under `.circleci/config.yml` and save the file:
-
-```yaml
-version: 2.1
-orbs:
- heroku: circleci/heroku@1.2.6
-workflows:
- heroku_deploy:
- jobs:
- - heroku/deploy-via-git
-```
-
-In the configuration above, we pull in the Heroku orb `circleci/heroku@1.2.6`, which automatically gives us access to a powerful set of Heroku jobs and commands.
-
-One of those jobs is `heroku/deploy-via-git`, which deploys your application straight from your GitHub repo to your Heroku account.
-
-## Step 5. Merge the pull request
-
-Once you make the new change, it will ask you to raise a new PR. Go ahead and merge the changes as of now.
-
-
-
-## Step 6. Set up a Heroku account
-
-[Follow these steps](https://developer.redis.com/create/heroku/portal) to set up a Heroku account and create a new app `rate-limit-python`. You will need the Heroku API key for this demo.
-
-
-
-## Step 7. Configuring Heroku access on CircleCI
-
-Before you push your project to Heroku from CircleCI, you will need to configure an authenticated handshake between CircleCI and Heroku. Configure the handshake by creating two [environment variables](https://circleci.com/docs/2.0/env-vars/) in the settings for your CircleCI project:
-
-- `HEROKU_APP_NAME` is the name of your Heroku application (in this case, `simple-node-api-circleci`)
-- `HEROKU_API_KEY` is your Heroku account API key. This can be found under the Account tab of your Heroku account under Account Settings. Scroll to the API Key section and click **Reveal** to copy your API key.
-
-## Step 8. Set up the environment variables on CircleCI
-
-On the sidebar menu of the settings page, click **Environment Variables** under Build Settings. On the environment variables page, create two variables named `HEROKU_APP_NAME` and `HEROKU_API_KEY` and enter the values for them.
-
-With these in place, our CircleCI configuration will be able to make authenticated deployments to the Heroku platform.
-
-
-
-## Step 9. Trigger the build
-
-As soon as you merge the pull request, the tool will trigger the build automatically.
-
-
-
-You should now be able to access your application.
-
-```
-remote: Collecting attrs>=19.2.0
-remote: Downloading attrs-21.4.0-py2.py3-none-any.whl (60 kB)
-remote: Collecting wrapt<2,>=1.10
-remote: Downloading wrapt-1.14.0-cp310-cp310-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl (77 kB)
-remote: Collecting pyparsing!=3.0.5,>=2.0.2
-remote: Downloading pyparsing-3.0.7-py3-none-any.whl (98 kB)
-remote: Installing collected packages: wrapt, pyparsing, typing-extensions, sqlparse, packaging, deprecated, async-timeout, asgiref, tomli, redis, py, pluggy, iniconfig, h11, Django, click, attrs, uvloop, uvicorn, urllib3, sniffio, rfc3986, pytz, python-dotenv, pytest, django-redis, django-ipware, django-cors-headers
-remote: Successfully installed Django-4.0.3 asgiref-3.5.0 async-timeout-4.0.2 attrs-21.4.0 click-8.1.0 deprecated-1.2.13 django-cors-headers-3.11.0 django-ipware-4.0.2 django-redis-5.2.0 h11-0.13.0 iniconfig-1.1.1 packaging-21.3 pluggy-1.0.0 py-1.11.0 pyparsing-3.0.7 pytest-7.1.1 python-dotenv-0.20.0 pytz-2022.1 redis-4.2.0 rfc3986-2.0.0 sniffio-1.2.0 sqlparse-0.4.2 tomli-2.0.1 typing-extensions-4.1.1 urllib3-1.26.9 uvicorn-0.17.6 uvloop-0.16.0 wrapt-1.14.0
-remote: -----> $ python server/manage.py collectstatic --noinput
-remote: 133 static files copied to '/tmp/build_3850bcfb/server/static_root'.
-remote:
-remote: -----> Discovering process types
-remote: Procfile declares types -> web
-remote:
-remote: -----> Compressing...
-remote: Done: 75.3M
-remote: -----> Launching...
-remote: Released v11
-remote: https://*****************.herokuapp.com/ deployed to Heroku
-```
-
-
-
-## Additional references:
-
-- [What is a CI/CI Pipeline?](https://circleci.com/blog/what-is-a-ci-cd-pipeline/) (article at circleci.com)
-- [Getting Started with CircleCI](https://circleci.com/docs/2.0/getting-started/) (article at circleci.com)
-- [Creating a Redis database on Heroku](https://developer.redis.com/create/heroku/portal)
diff --git a/docs/support/_continuous-integration-continuous-deployment/index-continuous-integration-continuous-deployment.mdx b/docs/support/_continuous-integration-continuous-deployment/index-continuous-integration-continuous-deployment.mdx
deleted file mode 100644
index 8cca1b9..0000000
--- a/docs/support/_continuous-integration-continuous-deployment/index-continuous-integration-continuous-deployment.mdx
+++ /dev/null
@@ -1,10 +0,0 @@
----
-id: index-continuous-integration-continuous-deployment
-title: Continuous Integration/Deployment
-sidebar_label: Overview
-slug: /operate/continuous-integration-continuous-deployment
----
-
-import RedisCard from '@theme/RedisCard';
-
-The following links show you the different ways to embed Redis into your continuous integration and continuous deployment process.
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image1.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image1.png
deleted file mode 100644
index be4748c..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image1.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image10.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image10.png
deleted file mode 100644
index a6a42da..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image10.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image11.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image11.png
deleted file mode 100644
index 705a131..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image11.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image12.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image12.png
deleted file mode 100644
index 961c00a..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image12.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image13.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image13.png
deleted file mode 100644
index 58c194b..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image13.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image14.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image14.png
deleted file mode 100644
index 3d84c8f..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image14.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image15.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image15.png
deleted file mode 100644
index 92ba513..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image15.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image16.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image16.png
deleted file mode 100644
index 46ea31c..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image16.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image17.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image17.png
deleted file mode 100644
index 44a09fc..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image17.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image18.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image18.png
deleted file mode 100644
index b6b004e..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image18.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image19.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image19.png
deleted file mode 100644
index 0cf0525..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image19.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image2.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image2.png
deleted file mode 100644
index ffa5543..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image2.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image20.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image20.png
deleted file mode 100644
index 840270f..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image20.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image3.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image3.png
deleted file mode 100644
index 906696c..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image3.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image4.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image4.png
deleted file mode 100644
index 7ec8a7f..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image4.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image5.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image5.png
deleted file mode 100644
index b0fc8f1..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image5.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image6.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image6.png
deleted file mode 100644
index eebb83e..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image6.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image7.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image7.png
deleted file mode 100644
index 8dacf34..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image7.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image8.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image8.png
deleted file mode 100644
index 62892d4..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image8.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image9.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image9.png
deleted file mode 100644
index 68b4ac9..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/image9.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/status.png b/docs/support/_continuous-integration-continuous-deployment/jenkins/images/status.png
deleted file mode 100644
index 6d2b60d..0000000
Binary files a/docs/support/_continuous-integration-continuous-deployment/jenkins/images/status.png and /dev/null differ
diff --git a/docs/support/_continuous-integration-continuous-deployment/jenkins/index-jenkins.mdx b/docs/support/_continuous-integration-continuous-deployment/jenkins/index-jenkins.mdx
deleted file mode 100644
index 2b7786d..0000000
--- a/docs/support/_continuous-integration-continuous-deployment/jenkins/index-jenkins.mdx
+++ /dev/null
@@ -1,250 +0,0 @@
----
-id: index-jenkins
-title: How to Deploy a Redis Enterprise Database from a Jenkins Pipeline
-sidebar_label: Redis using Jenkins
-slug: /operate/continuous-integration-continuous-deployment/jenkins
-authors: [ajeet, matthew]
----
-
-
-
-
-
-[Jenkins](https://www.jenkins.io/) is currently [the most popular CI tool](https://cd.foundation/announcement/2019/08/14/jenkins-celebrates-15-years/), with ~15M users. It is an open source automation server which enables developers to reliably build, test, and deploy their software. It was forked in 2011 from a project called Hudson after a [dispute with Oracle](https://www.infoq.com/news/2011/01/jenkins/), and is used for [Continuous Integration and Continuous Delivery (CI/CD)](https://stackoverflow.com/questions/28608015/continuous-integration-vs-continuous-delivery-vs-continuous-deployment) and test automation. Jenkins is based on Java and provides over [1700 plugins](https://plugins.jenkins.io/) to automate your developer workflow and save a lot of your time in executing your repetitive tasks.
-
-
-
-[Source:](https://www.datanyze.com/market-share/ci--319) Datanyze market analysis
-
-[Jenkins Pipeline](https://www.jenkins.io/solutions/pipeline/) performs Continuous Delivery tasks declared in a `Jenkinsfile` stored alongside your project's code. The [Pipeline plugin](https://plugins.jenkins.io/workflow-aggregator) has a fairly comprehensive [tutorial](https://github.com/jenkinsci/pipeline-plugin/blob/master/TUTORIAL.md) checked into its source tree. Using Pipeline, you can configure Jenkins to automatically deploy key pieces of infrastructure, such as a Redis database.
-
-### Architecture
-
-Jenkins Pipelines are the Continuous Delivery (CD) side of Jenkins. They use a `Jenkinsfile` declarative script to define the behavior of the pipeline. You can script actions in Groovy and run shell scripts from it, so you can make it do pretty much anything.
-
-The `Jenkinsfile` instructs Jenkins to export some environment variables from the Credentials store in order to connect to the Redis server, then executes the Python pipeline script with the Deployment Configuration file given as a parameter. An example `deployment-configuration-file.json` looks like:
-
-```
-{
- "database": {
- "name": "made-with-jenkins",
- "port": 12345,
- "size": "S",
- "operation": "CREATE"
- }
-}
-```
-
-The Python script uses predefined JSON template files that create Redis databases of fixed t-shirt sizes (S, M, L, XL). The Deployment Config file tells the Python script what the desired database name, port, and size are. A sample template file looks like:
-
-```
-{
- "name": "{NAME}",
- "type": "redis",
- "memory_size": 343597383
-}
-```
-
-The following is an architectural diagram of how a Jenkins pipeline adds a database to a Redis cluster.
-
-
-
-### Process
-
-1. The Jenkins pipeline clones a remote git repository, containing the application code and the pipeline code.
-2. The Redis host, port, user, and password are decrypted from the credentials store and are exported as environment variables.
-3. Jenkins runs the Python pipeline script, specifying the deployment configuration file in the git repo.
-4. The Python script uses the deployment configuration file to choose and customize a pre-populated template to use as the body of the REST create database request to Redis.
-
-### List of Pipeline Code Files
-
-- [jenkins-re-pipeline.py](https://github.com/masyukun/redis-jenkins-pipeline/blob/main/jenkins-re-pipeline.py)
- - The Python script that creates a Redis database through the Redis REST API.
-- [deployment-configuration-file.json](https://github.com/masyukun/redis-jenkins-pipeline/blob/main/deployment-configuration-file.json)
- - The user-specified configuration file for creating a database.
-- [redis-standard-size-s.json.template](https://github.com/masyukun/redis-jenkins-pipeline/blob/main/redis-standard-size-s.json.template)
-- [redis-standard-size-xl.json.template](https://github.com/masyukun/redis-jenkins-pipeline/blob/main/redis-standard-size-xl.json.template)
-
-## Configuring Jenkins
-
-### Installing Jenkins
-
-You can use [Docker Desktop](https://www.docker.com/products/docker-desktop) to quickly get a Jenkins instance up and running, exposing ports 8080 (web GUI) and 50000 (inbound agents).
-
-```
-docker run --name jenk -p 8080:8080 -p 50000:50000 jenkins/jenkins:lts-jdk11
-```
-
-The installation will generate a first-run password in the docker-cli output.
-
-Then open[ http://localhost:8080/](http://localhost:8080/) and enter the password to unlock your instance and begin installation.
-
-
-
-Choose "Install suggested plugins"
-
-
-
-Wait for the plugins to complete the installation process.
-
-
-
-Next, you’re prompted to create your admin user.
-
-
-
-Congratulations! Jenkins is ready!
-
-
-
-### Installing Python and custom libraries
-
-If you use an existing instance of Jenkins, you can install Python and the custom libraries from the command line interface of that machine.
-
-Docker instances of Jenkins can be accessed by shell using the following command:
-
-```
-docker exec -it -u root jenk bash
-```
-
-The Python pipeline script requires the libraries `click` and `requests`. It also requires Python.
-
-```
-apt-get update
-apt-get install -y python3-pip
-
-pip install --upgrade pip
-pip install click
-pip install requests
-```
-
-Alternatively, if you are creating a new Jenkins from scratch, you can include these dependencies in a separate `Dockerfile` that builds off the base Jenkins image:
-
-```
-FROM jenkins:latest
-USER root
-RUN apt-get update
-RUN apt-get install -y python-pip
-
-# Install app dependencies
-RUN pip install --upgrade pip
-RUN pip3 install click
-RUN pip3 install requests
-```
-
-### Add credentials to Secret Store
-
-Using the left-side menu, select **Manage Jenkins**, then select **Manage Credentials**, then click the link **(global)**.
-
-
-
-
-
-
-
-From here, you can specify Kind: **Secret text** for the 4 secrets required to connect with the Redis REST endpoint:
-
-- `REDIS_SERVER_FQDN`
- - Set to the 'https://server-address' of the target Redis instance.
-- `REDIS_SERVER_PORT`
- - Set to the Redis REST API port (default 9443).
-- `REDIS_USER`
- - Set to the Redis admin user allowed to create databases.
-- `REDIS_PASS`
- - Set to the Redis admin user's password.
-
-
-
-If you are using a private code repository, you may also wish to include a Personal Access Token here.
-
-### Create the Jenkins pipeline
-
-From the dashboard, click **New Item**.
-
-
-
-Enter in a name for the pipeline, and choose the **Pipeline** type.
-
-
-
-## Connect GitHub repository
-
-From the Pipeline configuration page that appears, check the **GitHub** box and enter the git clone URL, complete with any credentials needed to read the repository. For GitHub access, the password should be a Personal Access Token rather than the actual user password.
-
-
-
-### Redis pipeline Jenkinsfile
-
-Scrolling down on this page to the **Advanced Project Options**, you can either past in the `Jenkinsfile`, or you can specify the filename if the file exists in the git repository.
-
-
-
-Here is an example `Jenkinsfile` containing the mapping of Credentials to the environment variables, and 2 separate stages – a Hello World which always succeeds, and a build stage that invokes the Python script. Paste this into the pipeline script section.
-
-```
-pipeline {
- agent any
-
- environment {
- REDIS_SERVER_FQDN = credentials('REDIS_SERVER_FQDN')
- REDIS_SERVER_PORT = credentials('REDIS_SERVER_PORT')
- REDIS_USER = credentials('REDIS_USER')
- REDIS_PASS = credentials('REDIS_PASS')
- }
-
- stages {
- stage('Hello') {
- steps {
- echo 'Hello World'
- }
- }
-
- stage('build') {
- steps {
- git branch: 'main', url: 'https://github.com/masyukun/redis-jenkins-pipeline.git'
- sh 'python3 jenkins-re-pipeline.py --deployfile deployment-configuration-file.json'
- }
- }
- }
-}
-```
-
-Click "Save" when the job spec is complete.
-
-### Run the Jenkins pipeline
-
-Click on the pipeline you created:
-
-
-
-Click the "Build Now" icon on the left side menu.
-
-
-
-Click the **Status** icon on the left side menu in order to see the results of all the output from each of the stages of your pipeline.
-
-
-
-Hover over the **build** stage and click the **Logs** button of the most recent build in order to see the Python script’s output.
-
-
-
-Sample output: you should see a verbose response from Redis’s REST service in the “Shell Script” accordion pane.
-
-There’s also a “Git” output log, in case you need to debug something at that level. Any time you update the branch in the remote git repository, you should see evidence in that log that the latest changes have successfully checked out into the local Jenkins git repository.
-
-
-
-Open your Redis Enterprise Secure Management UI at `https://servername:8443` and click on the **databases** menu item to verify that your database was created with the name, port, and size specified in the `deployment-configuration-file.json` file.
-
-
-
-Congratulations! You have deployed a Redis Enterprise database using a Jenkins Pipeline!
-
-The GitHub repository is currently: [https://github.com/masyukun/redis-jenkins-pipeline](https://github.com/masyukun/redis-jenkins-pipeline)
-
-### Further Reading
-
-- [How to Embed Redis into Your CI-CD Process](https://redis.com/blog/how-to-embed-redis-into-your-continuous-integration-and-continuous-deployment-ci-cd-process/)
-- [Top Redis Headaches for DevOps](https://redis.com/blog/top-redis-headaches-for-devops-replication-timeouts/)
-- [Top 5 Reasons why DevOps Love Redis Enterprise ](https://redis.com/blog/why-devops-teams-love-redis-enterprise/)
diff --git a/docs/support/devops.png b/docs/support/devops.png
deleted file mode 100644
index f23b6db..0000000
Binary files a/docs/support/devops.png and /dev/null differ
diff --git a/docs/support/devops1.png b/docs/support/devops1.png
deleted file mode 100644
index 04d6757..0000000
Binary files a/docs/support/devops1.png and /dev/null differ
diff --git a/docs/support/_index-operate.mdx b/docs/support/index-support.mdx
similarity index 86%
rename from docs/support/_index-operate.mdx
rename to docs/support/index-support.mdx
index 676998d..c0f5df9 100644
--- a/docs/support/_index-operate.mdx
+++ b/docs/support/index-support.mdx
@@ -1,11 +1,11 @@
---
-id: index-operate
+id: index-support
title: Operate Your Redis Database
sidebar_label: Overview
-slug: /operate
+slug: /support
---
-import RedisCard from '@theme/RedisCard';
+import ThreeTenCard from '@theme/ThreeTenCard';
The following links demonstrate various ways to provision Redis and accelerate app deployment using Devops.
@@ -17,14 +17,14 @@ The following links demonstrate various ways to provision Redis and accelerate a
-
-
-
-
-
-
-
-
-
diff --git a/docs/support/observability/_prometheus/images/image1.png b/docs/support/observability/_prometheus/images/image1.png
deleted file mode 100644
index dc183d7..0000000
Binary files a/docs/support/observability/_prometheus/images/image1.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image2.png b/docs/support/observability/_prometheus/images/image2.png
deleted file mode 100644
index e51a5ab..0000000
Binary files a/docs/support/observability/_prometheus/images/image2.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image_3.png b/docs/support/observability/_prometheus/images/image_3.png
deleted file mode 100644
index 7fd9515..0000000
Binary files a/docs/support/observability/_prometheus/images/image_3.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image_4.png b/docs/support/observability/_prometheus/images/image_4.png
deleted file mode 100644
index 9579b22..0000000
Binary files a/docs/support/observability/_prometheus/images/image_4.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image_6.png b/docs/support/observability/_prometheus/images/image_6.png
deleted file mode 100644
index d92dfaf..0000000
Binary files a/docs/support/observability/_prometheus/images/image_6.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image_7.png b/docs/support/observability/_prometheus/images/image_7.png
deleted file mode 100644
index 562d2f1..0000000
Binary files a/docs/support/observability/_prometheus/images/image_7.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/image_8.png b/docs/support/observability/_prometheus/images/image_8.png
deleted file mode 100644
index 423880f..0000000
Binary files a/docs/support/observability/_prometheus/images/image_8.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/images/prometheus.png b/docs/support/observability/_prometheus/images/prometheus.png
deleted file mode 100644
index 22f3c69..0000000
Binary files a/docs/support/observability/_prometheus/images/prometheus.png and /dev/null differ
diff --git a/docs/support/observability/_prometheus/index-prometheus.mdx b/docs/support/observability/_prometheus/index-prometheus.mdx
deleted file mode 100644
index 7da6f6f..0000000
--- a/docs/support/observability/_prometheus/index-prometheus.mdx
+++ /dev/null
@@ -1,217 +0,0 @@
----
-id: index-prometheus
-title: How to monitor Redis with Prometheus and Grafana for Real-Time Analytics
-sidebar_label: Using Time Series data model in Redis Stack along with Prometheus and Grafana
-slug: /operate/observability/prometheus
-authors: [ajeet]
----
-
-
-
-
-
-
-
-Time-series data is basically a series of data stored in time order and produced continuously over a long period of time. These measurements and events are tracked, monitored, downsampled, and aggregated over time. The events could be, for example, IoT sensor data. Every sensor is a source of time-series data. Each data point in the series stores the source information and other sensor measurements as labels. Data labels from every source may not conform to the same structure or order.
-
-A time-series database is a database system designed to store and retrieve such data for each point in time. Timestamped data can include data generated at regular intervals as well as data generated at unpredictable intervals.
-
-### When do you use a time-series database?
-
-- When your application needs data that accumulates quickly and your other databases aren’t designed to handle that scale.
-- For financial or industrial applications.
-- When your application needs to perform real-time analysis of billions of records.
-- When your application needs to perform online queries at millisecond timescales, and support CPU-efficient ad-hoc queries.
-
-### Challenges with the existing traditional databases
-
-You might find numerous solutions that still store time-series data in a relational database, but they’re quite inefficient and come with their own set of drawbacks. A typical time-series database is usually built to only manage time-series data, hence one of the challenges it faces is with use cases that involve some sort of computation on top of time-series data. One good example could be capturing a live video feed in a time-series database. If you want to run an AI model for face recognition, you would have to extract the time-series data, apply some sort of data transformation and then do computation.
-Relational databases carry the overhead of locking and synchronization that aren’t required for the immutable time-series data. This results in slower-than-required performance for both ingest and queries. When scaling out, it also means investing in additional compute resources. These databases enforce a rigid structure for labels and can’t accommodate unstructured data. They also require scheduled jobs for cleaning up old data. Beyond the time-series use case, these databases are also used for other use cases, which means overuse of running time-series queries may affect other workloads.
-
-### What is Redis Stack ?
-
-[Redis Stack](https://redis.io/docs/stack/about/) extends the core capabilities of Redis OSS and provides a complete developer experience for debugging and more. In addition to all of the features of Redis OSS, Redis Stack supports:
-
-- Queryable JSON documents
-- Querying across hashes and JSON documents
-- Time series data support (ingestion & querying), including full-text search
-- Probabilistic data structures
-
-### What is the Time Series data model in Redis Stack?
-
-[Redis Stack](https://redis.io/docs/stack/about/) supports time-series data model that addresses the needs of handling time-series data. It removes the limitations enforced by relational databases and enables you to collect, manage, and deliver time-series data at scale. As an in-memory database, Redis can ingest over 500,000 records per second on a standard node. Our benchmarks show that you can ingest over 11.5 million records per second with a cluster of 16 Redis shards.
-
-Time Series support in Redis Stack is resource-efficient. With Redis, you can add rules to compact data by downSampling. For example, if you’ve collected more than one billion data points in a day, you could aggregate the data by every minute in order to downSample it, thereby reducing the dataset size to 1,440 data points (24 \* 60 = 1,440). You can also set data retention policies and expire the data by time when you don’t need them anymore. Redis allows you to aggregate data by average, minimum, maximum, sum, count, range, first, and last. You can run over 100,000 aggregation queries per second with sub-millisecond latency. You can also perform reverse lookups on the labels in a specific time range.
-
-### Notables features related to Time Series in Redis Stack includes:
-
-- High volume inserts, low latency reads
-- Query by start time and end-time
-- Aggregated queries (Min, Max, Avg, Sum, Range, Count, First, Last, STD.P, STD.S, Var.P, Var.S) for any time bucket
-- Configurable maximum retention period
-- DownSampling/Compaction - automatically updated aggregate time series
-- Secondary index - each time series has labels (field value pairs) which will allows to query by labels
-
-### Why Prometheus?
-
-Prometheus is an open-source systems monitoring and alerting toolkit. It collects and stores its metrics as time series data, i.e. metrics information. The metrics are numeric measurements in a time series, meaning changes recorded over time. These metrics are stored with the timestamp at which it was recorded, alongside optional key-value pairs called labels. Metrics play an important role in understanding why your application is working in a certain way.
-
-### Prometheus remote storage adapter for Time Series data model of Redis Stack
-
-In the [Time Series Database over Redis](https://github.com/RedisTimeSeries) organization you can find projects that help you integrate Time Series data model of Redis Stack with other tools, including Prometheus and Grafana. The Prometheus remote storage adapter for Redis is hosted [on GitHub here](https://github.com/RedisTimeSeries/prometheus-redistimeseries-adapter.) It’s basically a read/write adapter to use Redis Stack as a backend database. This timeSeries Adapter receives Prometheus metrics via the remote write, and writes to Redis.
-
-## Getting Started
-
-### Prerequisites:
-
-- Install GIT
-- Install Docker
-- Install Docker Compose
-
-### Step 1. Clone the repository
-
-```
- git clone https://github.com/RedisTimeSeries/prometheus-redistimeseries-adapter
-```
-
-### Step 2. Examining the Docker Compose File
-
-This Docker compose defines 4 services -
-
-1. Prometheus
-2. Adapter
-3. Grafana
-4. Redis
-
-```yaml
- version: '3'
- services:
- prometheus:
- image: "prom/prometheus:v2.8.0"
- command: ["--config.file=/prometheus.yml"]
- volumes:
- - ./prometheus.yaml:/prometheus.yml
- ports:
- - 9090:9090
- adapter:
- image: "redislabs/prometheus-redistimeseries-adapter:master"
- command: ["-redis-address", "redis:6379", "-web.listen-address", "0.0.0.0:9201"]
- redis:
- image: "redislabs/redistimeseries:edge"
- ports:
- - "6379:6379"
- grafana:
- build: ./grafana/
- ports:
- - "3000:3000"
-```
-
-#### Prometheus
-
-The `prometheus` service directly uses an image “prom/prometheus” that’s pulled from Docker Hub. It then binds the container and the host machine to the exposed port, 9090. The Prometheus configuration file is accessed by mounting the volume on the host and container.
-
-#### Storage Adapter
-
-The `adapter` service uses an image “`redislabs/prometheus-redistimeseries-adapter:master`” that’s pulled from Docker Hub. Sets the default command for the container: `-redis-address", "redis:6379 and listen to the address 0.0.0.0:9201. `
-
-#### Redis
-
-The `Redis` service directly uses an image “`redislabs/redistimeseries:edge`” that’s pulled from Docker Hub. It then binds the container and the host machine to the exposed port, `6379`
-
-#### Grafana
-
-The `grafana` service uses an image that’s built from the `Dockerfile` in the current directory. It then binds the container and the host machine to the exposed port, `3000`.
-
-### Step 3. Run the Docker Compose
-
-Change directory to compose and execute the following command:
-
-```bash
- docker-compose up -d
-```
-
-```bash
- docker-compose ps
- NAME COMMAND SERVICE STATUS PORTS
- compose-adapter-1 "/adapter/redis-ts-a…" adapter running
- compose-grafana-1 "/run.sh" grafana running 0.0.0.0:3000->3000/tcp
- compose-prometheus-1 "/bin/prometheus --c…" prometheus running 0.0.0.0:9090->9090/tcp
- compose-redis-1 "docker-entrypoint.s…" redis running 0.0.0.0:6379->6379/tcp
-```
-
-### Step 4. Accessing Grafana
-
-Open `http://hostIP:3000` to access the Grafana dashboard. The default username and password is admin/admin.
-
-### Step 5. Add Prometheus Data Source
-
-In the left sidebar, you will see the “Configuration” option. Select “Data Source” and choose Prometheus.
-
-
-
-Click “Save and Test”.
-
-### Step 6. Importing Prometheus Data Source
-
-Click on “Import” for all the Prometheus dashboards.
-
-
-
-### Step 7. Adding Redis Data Source
-
-Again, click on “Data Sources” and add Redis.
-
-
-
-Click "Import".
-
-
-
-### Step 8. Running the Sensor Script
-
-It’s time to test drive a few demo scripts built by the Redis team. To start with, clone the following repository:
-
-```
- git clone https://github.com/RedisTimeSeries/prometheus-demos
-```
-
-This repo contains a set of basic demos showcasing the integration of Time Series data model of Redis Stack with Prometheus and Grafana. Let’s pick up a sensor script.
-
-```
- python3 weather_station/sensors.py
-```
-
-This script will add random measurements for temperature and humidity for a number of sensors.
-
-Go to “Add Panel” on the top right corner of the Grafana dashboard and start adding temperature and humidity values.
-
-
-
-### Step 9. Accessing Prometheus Dashboard
-
-Open up `https://HOSTIP:9090` to access the Prometheus dashboard for the sensor values without any further configuration.
-
-
-
-### Further References:
-
-- [Prometheus remote storage adapter for Time Series with Redis Stack](https://github.com/RedisTimeSeries/prometheus-redistimeseries-adapter)
-- [Remote Storage Integration](https://prometheus.io/docs/prometheus/latest/storage/#remote-storage-integrations)
-- [Time Series Demos with Redis Stack](https://github.com/RedisTimeSeries/prometheus-demos)
-
-##
-
-
diff --git a/docs/support/observability/_redisexplorer/cluster_databases_dashboard.png b/docs/support/observability/_redisexplorer/cluster_databases_dashboard.png
deleted file mode 100644
index 9588cd7..0000000
Binary files a/docs/support/observability/_redisexplorer/cluster_databases_dashboard.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/cluster_nodes.png b/docs/support/observability/_redisexplorer/cluster_nodes.png
deleted file mode 100644
index 977ff8e..0000000
Binary files a/docs/support/observability/_redisexplorer/cluster_nodes.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/cluster_nodes_dashboard.png b/docs/support/observability/_redisexplorer/cluster_nodes_dashboard.png
deleted file mode 100644
index a3bd45c..0000000
Binary files a/docs/support/observability/_redisexplorer/cluster_nodes_dashboard.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/cluster_overview.png b/docs/support/observability/_redisexplorer/cluster_overview.png
deleted file mode 100644
index f145ad1..0000000
Binary files a/docs/support/observability/_redisexplorer/cluster_overview.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/cluster_overview_dashboard.png b/docs/support/observability/_redisexplorer/cluster_overview_dashboard.png
deleted file mode 100644
index 56059c1..0000000
Binary files a/docs/support/observability/_redisexplorer/cluster_overview_dashboard.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/datasource.png b/docs/support/observability/_redisexplorer/datasource.png
deleted file mode 100644
index 899db48..0000000
Binary files a/docs/support/observability/_redisexplorer/datasource.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/enterprise_cluster_dashboard.png b/docs/support/observability/_redisexplorer/enterprise_cluster_dashboard.png
deleted file mode 100644
index a7a210b..0000000
Binary files a/docs/support/observability/_redisexplorer/enterprise_cluster_dashboard.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/explorer_options.png b/docs/support/observability/_redisexplorer/explorer_options.png
deleted file mode 100644
index f864e64..0000000
Binary files a/docs/support/observability/_redisexplorer/explorer_options.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/grafana.png b/docs/support/observability/_redisexplorer/grafana.png
deleted file mode 100644
index 0ecfe0f..0000000
Binary files a/docs/support/observability/_redisexplorer/grafana.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/index-redisexplorer.mdx b/docs/support/observability/_redisexplorer/index-redisexplorer.mdx
deleted file mode 100644
index 7602bfc..0000000
--- a/docs/support/observability/_redisexplorer/index-redisexplorer.mdx
+++ /dev/null
@@ -1,130 +0,0 @@
----
-id: index-redisexplorer
-title: How to create Grafana Dashboards for Redis Enterprise cluster in 5 Minutes
-sidebar_label: Grafana Dashboards for Redis Enterprise Cluster
-slug: /operate/observability/redisexplorer
-authors: [ajeet]
----
-
-
-
-
-
-Redis Enterprise clusters are a set of nodes, typically two or more, providing database services. Clusters are inherently multi-tenant, and a single cluster can manage multiple databases accessed through individual endpoints. Redis Enterprise software provides REST API to retrieve information about cluster, database, nodes and metrics.
-
-Redis Explorer plugin is the latest plugin in the Grafana Labs that adds support for Redis Enterprise software. It is a plugin for Grafana that connects to Redis Enterprise software clusters using REST API. It provides application pages to add Redis Data Sources for managed databases and dashboards to see cluster configuration.
-
-
-
-Redis Explorer allows you to create the following dashboard over Grafana:
-
-#### Enterprise Clusters Dashboard
-
-The Enterprise Clusters dashboard provides basic information about the cluster, license, and displays most important metrics.
-
-
-
-#### Cluster Overview Dashboard
-
-The Cluster Overview dashboard provides the most important information and metrics for the selected cluster.
-
-
-
-#### Cluster Nodes Dashboard
-
-Cluster Nodes dashboard provides information and metrics for each node participating in the cluster.
-
-
-
-#### Cluster Databases Dashboard
-
-The Cluster Databases dashboard provides information and metrics for each databases managed by cluster.
-
-
-
-### Getting Started
-
-### Pre-requisites
-
-- Grafana 8.0+ is required for Redis Explorer 2.X.
-- Grafana 7.1+ is required for Redis Explorer 1.X.
-- Docker
-- Redis Enterprise Cluster
-
-### Step 1. Setup Redis Enterprise Cluster
-
-[Follow these steps](/create/docker/) to setup Redis Enterprise cluster nodes.
-
-
-
-
-
-### Step 2. Install Grafana
-
-```bash
- brew install grafana
-```
-
-### Step 3. Install redis-explorer-app
-
-Use the grafana-cli tool to install from the command line:
-Redis Application plugin and Redis Data Source will be auto-installed as dependencies.
-
-```bash
- grafana-cli plugins install redis-explorer-app
-```
-
-### Step 4. Using Docker
-
-You can even run Redis Explorer plugin using Docker:
-
-```bash
- docker run -p 3000:3000 --name=grafana -e "GF_INSTALL_PLUGINS=redis-explorer-app" grafana/grafana
-```
-
-Open `https://IP:3000` to access grafana. The default username/password is admin/admin.
-
-### Step 5. Log in to Grafana
-
-
-
-### Step 6. Choose Redis Explorer in the sidebar
-
-Once you add the datasource, you should be able to choose the right option:
-
-
-
-### Step 7. Getting the Redis Enterprise Cluster Overview
-
-
-
-### Step 8. Displaying the Redis Enterprise Cluster Nodes
-
-
-
-### Further References
-
-- [Redis Explorer plugin for Grafana](https://grafana.com/grafana/plugins/redis-explorer-app/)
-- [Redis Plugins for Grafana Quickstart Guide](https://redisgrafana.github.io/quickstart/)
-- [Introducing the Redis Data Source Plug-in for Grafana](https://redis.com/blog/how-to-use-the-new-redis-data-source-for-grafana-plug-in/)
-- [How to Use the New Redis Data Source for Grafana Plug-in](https://redis.com/blog/how-to-use-the-new-redis-data-source-for-grafana-plug-in/)
-- [3 Real-Life Apps Built with Redis Data Source for Grafana](https://redis.com/blog/3-real-life-apps-built-with-redis-data-source-for-grafana/)
-- [How to Manage Real-Time IoT Sensor Data in Redis](https://redis.com/blog/how-to-manage-real-time-iot-sensor-data-in-redis/)
-- [Real-time observability with Redis and Grafana](https://grafana.com/go/observabilitycon/real-time-observability-with-redis-and-grafana/)
-
-##
-
-
diff --git a/docs/support/observability/_redisexplorer/redis_enterprise_cluster.png b/docs/support/observability/_redisexplorer/redis_enterprise_cluster.png
deleted file mode 100644
index 87b885e..0000000
Binary files a/docs/support/observability/_redisexplorer/redis_enterprise_cluster.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/redisexplorer.png b/docs/support/observability/_redisexplorer/redisexplorer.png
deleted file mode 100644
index 54f5813..0000000
Binary files a/docs/support/observability/_redisexplorer/redisexplorer.png and /dev/null differ
diff --git a/docs/support/observability/_redisexplorer/tryfree1.png b/docs/support/observability/_redisexplorer/tryfree1.png
deleted file mode 100644
index f16da8a..0000000
Binary files a/docs/support/observability/_redisexplorer/tryfree1.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/datadog-redis.png b/docs/support/observability/datadog/images/datadog-redis.png
deleted file mode 100644
index 49b0d16..0000000
Binary files a/docs/support/observability/datadog/images/datadog-redis.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image1.png b/docs/support/observability/datadog/images/image1.png
deleted file mode 100644
index f4e80e7..0000000
Binary files a/docs/support/observability/datadog/images/image1.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image10.png b/docs/support/observability/datadog/images/image10.png
deleted file mode 100644
index 9010eb8..0000000
Binary files a/docs/support/observability/datadog/images/image10.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image11.png b/docs/support/observability/datadog/images/image11.png
deleted file mode 100644
index 6486cd6..0000000
Binary files a/docs/support/observability/datadog/images/image11.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image12.png b/docs/support/observability/datadog/images/image12.png
deleted file mode 100644
index b6de9d4..0000000
Binary files a/docs/support/observability/datadog/images/image12.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image13.png b/docs/support/observability/datadog/images/image13.png
deleted file mode 100644
index 2165194..0000000
Binary files a/docs/support/observability/datadog/images/image13.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image14.png b/docs/support/observability/datadog/images/image14.png
deleted file mode 100644
index 3c6163b..0000000
Binary files a/docs/support/observability/datadog/images/image14.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image15.png b/docs/support/observability/datadog/images/image15.png
deleted file mode 100644
index 0e02262..0000000
Binary files a/docs/support/observability/datadog/images/image15.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image16.png b/docs/support/observability/datadog/images/image16.png
deleted file mode 100644
index 75871d6..0000000
Binary files a/docs/support/observability/datadog/images/image16.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image2.png b/docs/support/observability/datadog/images/image2.png
deleted file mode 100644
index be5fce7..0000000
Binary files a/docs/support/observability/datadog/images/image2.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image3.png b/docs/support/observability/datadog/images/image3.png
deleted file mode 100644
index 0c74e7f..0000000
Binary files a/docs/support/observability/datadog/images/image3.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image4.png b/docs/support/observability/datadog/images/image4.png
deleted file mode 100644
index 41cc514..0000000
Binary files a/docs/support/observability/datadog/images/image4.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image5.png b/docs/support/observability/datadog/images/image5.png
deleted file mode 100644
index 129f957..0000000
Binary files a/docs/support/observability/datadog/images/image5.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image6.png b/docs/support/observability/datadog/images/image6.png
deleted file mode 100644
index e07c57c..0000000
Binary files a/docs/support/observability/datadog/images/image6.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image7.png b/docs/support/observability/datadog/images/image7.png
deleted file mode 100644
index e646eae..0000000
Binary files a/docs/support/observability/datadog/images/image7.png and /dev/null differ
diff --git a/docs/support/observability/datadog/images/image8.png b/docs/support/observability/datadog/images/image8.png
deleted file mode 100644
index 6d5b5be..0000000
Binary files a/docs/support/observability/datadog/images/image8.png and /dev/null differ
diff --git a/docs/support/observability/datadog/index-datadog.mdx b/docs/support/observability/datadog/index-datadog.mdx
deleted file mode 100644
index b838a80..0000000
--- a/docs/support/observability/datadog/index-datadog.mdx
+++ /dev/null
@@ -1,391 +0,0 @@
----
-id: index-datadog
-title: Redis Enterprise Observability with Datadog
-sidebar_label: Redis Enterprise Observability with Datadog
-slug: /operate/observability/datadog
-authors: [ajeet, christian]
----
-
-
-
-
-
-
-
-Devops and SRE practitioners are already keenly aware of the importance of system reliability, as it’s one of the shared goals in every high performing organization. Defining clear reliability targets based on solid data is crucial for productive collaboration between developers and SREs. This need spans the entire infrastructure from application to backend database services.
-
-Service Level Objectives (SLOs) provide a powerful interface for all teams to set clear performance and reliability goals based on Service Level Indicators (SLIs) or data points. A good model is to think of the SLIs as the data and the SLO as the information one uses to make critical decisions.
-
-Further Read: [https://cloud.google.com/blog/products/devops-sre/sre-fundamentals-slis-slas-and-slos](https://cloud.google.com/blog/products/devops-sre/sre-fundamentals-slis-slas-and-slos)
-
-#### Redis
-
-Redis is a popular multi-model NoSQL database server that provides in-memory data access speeds for search, messaging, streaming, caching, and graph—amongst other capabilities. Highly performant sites such as Twitter, Snapchat, Freshworks, GitHub, Docker, Pinterest, and Stack Overflow all look to Redis to move data in real time.
-
-Redis SLOs can be broken down into three main categories:
-
-| Category | Definition | Example SLO | Example SLI |
-| ---------- | ---------------------------------------------------------------------------- | ------------------------------------------------------------------- | ----------------------------------- |
-| Throughput | Number of operations being pushed through the service in a given time period | System should be capable of performing 200M operations per second | redisenterprise.total_req |
-| Latency | Elapsed time it takes for an operation | Average write latency should not exceed 1 millisecond | redis_enterprise.avg_latency |
-| Capacity | Memory/storage/network limits of the underlying data source | Database should have 20% memory overhead available to handle bursts | redisenterprise.used_memory_percent |
-
-### Why Datadog?
-
-Running your own performance data platform is time consuming and difficult. Datadog provides an excellent platform with an open source agent to collect metrics and allows them to be displayed easily and alerted upon when necessary.
-
-Datadog allows you to:
-
-- Collect metrics from various infrastructure components out of the box
-- Display that data in easy to read dashboards
-- Monitor performance metrics and alert accordingly
-- Correlate log entries with metrics to quickly drill down to root causes
-
-### Key Performance Indicators
-
-### 1. Latency
-
-#### Definition
-
-##### redisenterprise.avg_latency (unit: microseconds)
-
-This is the average amount of time that a request takes to return from the time that it first hits the Redis Enterprise proxy until the response is returned. It does not include the full time from the remote client’s perspective.
-
-##### Characteristics
-
-Since Redis is popular due to performance, generally you would expect most operations to return in single digit milliseconds. Tune any alerts to match your SLA. It’s generally recommended that you also measure Redis operation latency at the client side to make it easier to determine if a server slow down or an increase in network latency is the culprit in any performance issues.
-
-##### Possible Causes
-
-| Cause | Factors |
-| ------------------------------ | ------------------------------------------------------------------------------------------------------------------ |
-| Spike in requests | Check both the Network Traffic and Operations Per Second metrics to determine if there is a corresponding increase |
-| Slow-running queries | Check the slow log in the Redis Enterprise UI for the database |
-| Insufficient compute resources | Check to see if the CPU Usage, Memory Usage Percentage, or Evictions are increasing |
-
-##### Remediation
-
-| Action | Method |
-| ------------------- | -------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| Increase resources | The database can be scaled up online by going to the Web UI and enabling clustering on the database. In extreme cases, more nodes can be added to the cluster and resources rebalanced |
-| Inefficient queries | Redis allows you to view a slow log with a tunable threshold. It can be viewed either in the Redis Enterprise UI or by running: redis-cli -h HOST -p PORT -a PASSWORD SLOWLOG GET 100 |
-
-### 2. Memory Usage Percentage
-
-#### Definition
-
-##### redisenterprise.memory_usage_percent (unit: percentage)
-
-This is the percentage of used memory over the memory limit set for the database.
-
-##### Characteristics
-
-In Redis Enterprise, all databases have a maximum memory limit set to ensure isolation in a multi-tenant environment. This is also highly recommended when running open source Redis. Be aware that Redis does not immediately free memory upon key deletion. Depending on the size of the database, generally between 80-95% is a safe threshold.
-
-##### Possible Causes
-
-| Cause | Factors |
-| ---------------------------- | ------------------------------------------------------------------------------------------------------------------ |
-| Possible spike in activity | Check both the Network Traffic and Operations Per Second metrics to determine if there is a corresponding increase |
-| Database sized incorrectly | View the Memory Usage raw bytes over time to see if a usage pattern has changed |
-| Incorrect retention policies | Check to see if keys are being Evicted or Expired |
-
-##### Remediation
-
-| Action | Method |
-| ------------------ | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
-| Increase resources | The database memory limit can be raised online with no downtime through either the Redis Enterprise UI or the API |
-| Retention Policy | In a caching use case, setting a TTL for unused data to expire is often helpful. In addition, Eviction policies can be set, however, these may often not be able to keep up in extremely high throughput environments with very tight resource constraints |
-
-### 3. Cache Hit Rate
-
-##### Definition
-
-##### redisenterprise.cache_hit_rate (unit: percent)
-
-This is the percentage of time that Redis is accessing a key that already exists.
-
-##### Characteristics
-
-This metric is useful only in the caching use case and should be ignored for all other use cases. There are tradeoffs between the freshness of the data in the cache and efficacy of the cache mitigating traffic to any backend data service. These tradeoffs should be considered carefully when determining the threshold for alerting.
-
-##### Possible Causes
-
-This is highly specific to the application caching with no general rules that are applicable in the majority of cases.
-
-##### Remediation
-
-Note that Redis commands return information on whether or not a key or field already exists. For example, the [HSET](https://redis.io/commands/hset) command returns the number of fields in the hash that were added.
-
-### 4. Evictions
-
-##### Definition
-
-##### redisenterprise.evicted_objects (unit: count)
-
-This is the count of items that have been evicted from the database.
-
-##### Characteristics
-
-Eviction occurs when the database is close to capacity. In this condition, the [eviction policy](https://docs.redis.com/latest/rs/administering/database-operations/eviction-policy/) starts to take effect. While [Expiration](https://redis.io/commands/expire) is fairly common in the caching use case, Eviction from the cache should generally be a matter of concern. At very high throughput and very restricted resource use cases, sometimes the eviction sweeps cannot keep up with memory pressure. Relying on Eviction as a memory management technique should be considered carefully.
-
-##### Possible Causes
-
-[See Memory Usage Percentage Possible Causes](#possible-causes-1)
-
-##### Remediation
-
-[See Memory Usage Percentage Remediation](#remediation-1)
-
-### Secondary Indicators
-
-### 1. Network Traffic
-
-##### Definition
-
-##### redisenterprise.ingress_bytes/redisenterprise.egress_bytes (unit: bytes)
-
-Counters for the network traffic coming into the database and out from the database.
-
-##### Characteristics
-
-While these two metrics will not help you pinpoint a root cause, network traffic is an excellent leading indicator of trouble. Changes in network traffic patterns indicate corresponding changes in database behavior and further investigation is usually warranted.
-
-### 2. Connection Count
-
-##### Definition
-
-##### redisenterprise.conns (unit: count)
-
-The number of current client connections to the database.
-
-##### Characteristics
-
-This metric should be monitored with both a minimum and maximum number of connections. The minimum number of connections not being met is an excellent indicator of either networking or application configuration errors. The maximum number of connections being exceeded may indicate a need to tune the database.
-
-##### Possible Causes
-
-| Cause | Factors |
-| ---------------------------- | ----------------------------------------------------------------------------------- |
-| Minimum clients not met | Incorrect client configuration, network firewall, or network issues |
-| Maximum connections exceeded | Client library is not releasing connections or an increase in the number of clients |
-
-##### Remediation
-
-| Action | Method |
-| --------------------- | --------------------------------------------------------------------------------------------------- |
-| Clients Misconfigured | Confirm client configurations |
-| Networking issue | Issue the PING command from a client node TELNET to the endpoint |
-| Too many connections | Be sure that you are using pooling on your client library and that your pools are sized accordingly |
-| Too many connections | Using rladmin, run: tune proxy PROXY_NUMBER threads VALUE threads VALUE |
-
-You can access the complete list of metrics [here](https://github.com/DataDog/integrations-extras/blob/master/redisenterprise/metadata.csv).
-
-## Getting Started
-
-Follow the steps below to set up the Datadog agent to monitor your Redis Enterprise cluster, as well as database metrics:
-
-### Quickstart Guide:
-
-#### Prerequisites:
-
-- [Follow this link](https://docs.redis.com/latest/rs/installing-upgrading/) to setup your Redis Enterprise cluster and database
-- Setup a Read-only user account by logging into your Redis Enterprise instance and visiting the “Access Control” section
-
-
-
-- Add a new user account with Cluster View Permissions.
-
-
-
-### Step 1. Set Up a Datadog Agent
-
-Before we jump into the installation, let’s look at the various modes that you can run the Datadog agent in:
-
-- External Monitor Mode
-- Localhost Mode
-
-#### External Monitor Mode
-
-
-
-In external monitor mode, a Datadog agent running outside of the cluster can monitor multiple Redis Enterprise clusters, as shown in the diagram above.
-
-#### Localhost Mode
-
-Using localhost mode, the integration can be installed on every node of a Redis Enterprise cluster. This allows the user to correlate OS level metrics with Redis-specific metrics for faster root cause analysis. Only the Redis Enterprise cluster leader will submit metrics and events to Datadog. In the event of a migration of the cluster leader, the new cluster leader will begin to submit data to Datadog.
-
-
-
-For this demo, we will be leveraging localhost mode as we just have two nodes to configure.
-
-### Step 2. Launch the Datadog agent on the Master node
-
-Pick up your preferred OS distribution and install the Datadog agent
-
-
-
-Run the following command to install the integration wheel with the Agent. Replace the integration version with 1.0.1.
-
-```bash
- datadog-agent integration install -t datadog-redisenterprise==
-```
-
-### Step 3. Configuring Datadog configuration file
-
-Copy the [sample configuration](https://github.com/DataDog/integrations-extras/blob/master/redisenterprise/datadog_checks/redisenterprise/data/conf.yaml.example) and update the required sections to collect data from your Redis Enterprise cluster:
-
-#### For Localhost Mode
-
-The following minimal configuration should be added to the Enterprise Master node.
-
-```
- sudo vim /etc/datadog-agent/conf.d/redisenterprise.d/conf.yaml
-```
-
-```bash
- #################################################################
- # Base configuration
- init_config:
-
- instances:
- - host: localhost
- username: user@example.com
- password: secretPassword
- port: 9443
-```
-
-Similarly, you need to add the edit the configuration file for the Enterprise Follower to add the following:
-
-```bash
- sudo vim /etc/datadog-agent/conf.d/redisenterprise.d/conf.yaml
-```
-
-```bash
- #################################################################
- # Base configuration
- init_config:
-
- instances:
- - host: localhost
- username: user@example.com
- password: secretPassword
- port: 9443
-```
-
-#### For External Monitor Mode
-
-The following configuration should be added to the Monitor node
-
-```
-# Base configuration
-init_config:
-
-instances:
- - host: cluster1.fqdn
- username: user@example.com
- password: secretPassword
- port: 9443
-
- - host: cluster2.fqdn
- username: user@example.com
- password: secretPassword
- port: 9443
-```
-
-### Step 4. Restart the Datadog Agent service
-
-```
- sudo service datadog-agent restart
-```
-
-### Step 5. Viewing the Datadog Dashboard UI
-
-Find the Redis Enterprise Integration under the Integration Menu:
-
-
-
-Displaying the host reporting data to Datadog:
-
-
-
-Listing the Redis Enterprise dashboards:
-
-
-
-Host details under Datadog Infrastructure list:
-
-
-
-Datadog dashboard displaying host metrics of the 1st host (CPU, Memory Usage, Load Average etc):
-
-
-
-Datadog dashboard displaying host metrics of the 2nd host:
-
-
-
-### Step 6. Verifying the Datadog Agent Status
-
-Running the `datadog-agent` command shows that the Redis Enterprise integration is working correctly.
-
-```
- sudo datadog-agent status
-```
-
-```bash
- redisenterprise (1.0.1)
- -----------------------
- Instance ID: redisenterprise:ef4cd60aadac5744 [OK]
- Configuration Source: file:/etc/datadog-agent/conf.d/redisenterprise.d/conf.yaml
- Total Runs: 2
- Metric Samples: Last Run: 0, Total: 0
- Events: Last Run: 0, Total: 0
- Service Checks: Last Run: 0, Total: 0
- Average Execution Time : 46ms
- Last Execution Date : 2021-10-28 17:27:10 UTC (1635442030000)
- Last Successful Execution Date : 2021-10-28 17:27:10 UTC (1635442030000)
-
-```
-
-### Redis Enterprise Cluster Top View
-
-
-
-Let’s run a memory benchmark tool called redis-benchmark to simulate an arbitrary number of clients connecting at the same time and performing actions on the server, measuring how long it takes for the requests to be completed.
-
-```bash
- memtier_benchmark --server localhost -p 19701 -a password
- [RUN #1] Preparing benchmark client...
- [RUN #1] Launching threads now...
-```
-
-
-
-This command instructs memtier_benchmark to connect to your Redis Enterprise database and generates a load doing the following:
-
-- Write objects only, no reads.
-- Each object is 500 bytes.
-- Each object has random data in the value.
-- Each key has a random pattern, then a colon, followed by a random pattern.
-
-Run this command until it fills up your database to where you want it for testing. The easiest way to check is on the database metrics page.
-
-```
- memtier_benchmark --server localhost -p 19701 -a Oracle9ias12# -R -n allkeys -d 500 --key-pattern=P:P --ratio=1:0
- setting requests to 50001
- [RUN #1] Preparing benchmark client...
- [RUN #1] Launching threads now...
-```
-
-
-
-The Datadog Events Stream shows an instant view of your infrastructure and services events to help you troubleshoot issues happening now or in the past. The event stream displays the most recent events generated by your infrastructure and the associated monitors, as shown in the diagram below.
-
-
-
-### References:
-
-- [https://docs.datadoghq.com/integrations/redisenterprise/](https://docs.datadoghq.com/integrations/redisenterprise/)
-- [Datadog Sample Configuration](https://github.com/DataDog/integrations-extras/blob/master/redisenterprise/datadog_checks/redisenterprise/data/conf.yaml.example) for Redis Enterprise
-- [Complete List of Performance Metrics](https://github.com/DataDog/integrations-extras/blob/master/redisenterprise/metadata.csv)
diff --git a/docs/support/observability/redisdatasource/grafana.png b/docs/support/observability/redisdatasource/grafana.png
deleted file mode 100644
index 060c315..0000000
Binary files a/docs/support/observability/redisdatasource/grafana.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource.png b/docs/support/observability/redisdatasource/grafana_datasource.png
deleted file mode 100644
index d36f991..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource2.png b/docs/support/observability/redisdatasource/grafana_datasource2.png
deleted file mode 100644
index 9e60831..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource2.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource3.png b/docs/support/observability/redisdatasource/grafana_datasource3.png
deleted file mode 100644
index 6c7f762..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource3.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource4.png b/docs/support/observability/redisdatasource/grafana_datasource4.png
deleted file mode 100644
index c0502ff..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource4.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource5.png b/docs/support/observability/redisdatasource/grafana_datasource5.png
deleted file mode 100644
index bbc27b1..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource5.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource6.png b/docs/support/observability/redisdatasource/grafana_datasource6.png
deleted file mode 100644
index 55f4ddf..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource6.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/grafana_datasource7.png b/docs/support/observability/redisdatasource/grafana_datasource7.png
deleted file mode 100644
index 90b548f..0000000
Binary files a/docs/support/observability/redisdatasource/grafana_datasource7.png and /dev/null differ
diff --git a/docs/support/observability/redisdatasource/index-redisdatasource.mdx b/docs/support/observability/redisdatasource/index-redisdatasource.mdx
deleted file mode 100644
index 0ec8395..0000000
--- a/docs/support/observability/redisdatasource/index-redisdatasource.mdx
+++ /dev/null
@@ -1,234 +0,0 @@
----
-id: index-redisdatasource
-title: How to add Redis as a datasource in Grafana and build customize dashboards for Analytics
-sidebar_label: Redis Data Source for Grafana
-slug: /operate/observability/redisdatasource
-authors: [ajeet]
----
-
-import Tabs from '@theme/Tabs';
-import TabItem from '@theme/TabItem';
-
-
-
-
-The Redis Data Source for Grafana is a plug-in that allows users to connect to the Redis database and build dashboards in Grafana to easily monitor Redis and application data. It provides an out-of-the-box predefined dashboard, but also lets you build customized dashboards tuned to your specific needs.
-
-
-
-## Features and Capabilities
-
-- Grafana 7.1 and later with a new plug-in platform supported.
-- Data Source can connect to any Redis database. No special configuration is required.
-- Redis Cluster and Sentinel supported since version 1.2.
-- Data Source supports:
-
- - [Redis Time Series](https://oss.redis.com/redistimeseries/): `TS.GET`, `TS.INFO`, `TS.MRANGE`, `TS.QUERYINDEX`, `TS.RANGE`
- - [Triggers and Functions](https://oss.redis.com/redisgears/): `RG.DUMPREGISTRATIONS`, `RG.PYEXECUTE`, `RG.PYSTATS`
- - [Search](https://oss.redis.com/redisearch/): `FT.INFO`
- - [Graph](https://oss.redis.com/redisgraph/): `GRAPH.QUERY`, `GRAPH.SLOWLOG`
-
-
-
-
-#### Using Homebrew
-
-#### Step 1. Install Grafana
-
-```bash
- brew install grafana
-```
-
-#### Step 2. Install Redis Datasource
-
-```bash
- grafana-cli plugins install redis-datasource
-```
-
-Homebrew downloads and untars the files into /usr/local/Cellar/grafana/version.
-
-#### Step 3. Start Grafana service
-
-```bash
- brew services start grafana
-```
-
-#### Step 4. Access Grafana dashboard
-
-Open `https://IP:3000` to access grafana. The default username/password is admin/admin.
-
-
-
-#### Step 5. Click "Configuration"
-
-
-
-#### Step 6. Add Redis as a Data Source
-
-
-
-#### Step 7. Select "Redis" as data source type
-
-
-
-#### Step 8. Add Redis Database name, Endpoint URL and password
-
-Assuming that you already have Redis server and database up and running in your infrastructure.
-You can also leverage Redis Enterprise Cloud as showcased in the below example.
-
-
-
-#### Step 9. Click "Import" under Dashboard
-
-
-
-#### Step 10.Access the Redis datasource Dashboard
-
-
-
-
-
-
-
-#### Using Docker
-
-You can install and run Grafana using the official Docker image.
-
-#### Step 1. Install Docker
-
-The first step is to install Docker for your operating system. Run the `docker version` command in a terminal window to make sure that Docker is installed correctly.
-
-:::note
-
-On Windows and Mac, install Docker version 18.03 or higher. You can run `docker version` to find out your Docker version.
-
-:::
-
-#### Step 2. Run Grafana Docker container
-
-Specify the plugins you want installed to Docker as a comma-separated list in the `GF_INSTALL_PLUGINS` environment. This sends each plugin name to `grafana-cli plugins install ${plugin}` and installs them when Grafana starts.
-In our case, we will be using redis-datasource.
-
-```bash
- docker run -d -p 3000:3000 --name=grafana -e "GF_INSTALL_PLUGINS=redis-datasource" grafana/grafana
-```
-
-#### Step 3. Accessing the Grafana dashboard
-
-Open `https://IP:3000` to access Grafana. The default username/password is admin/admin.
-
-
-
-#### Step 4. Click "Configuration"
-
-
-
-#### Step 5. Add Redis as a Data Source
-
-
-
-#### Step 6. Select "Redis" as data source type
-
-
-
-#### Step 7. Add Redis Database name, Endpoint URL and password
-
-We'll assume that you already have a Redis server and up and running in your infrastructure.
-You can also leverage Redis Enterprise Cloud as demonstrated below.
-
-
-
-#### Step 8. Click "Import" under Dashboard
-
-
-
-#### Step 9.Access the Redis datasource Dashboard
-
-
-
-
-
-
-#### Using Docker Compose
-
-Assuming that Docker Compose is already installed in your system, follow these steps:
-
-#### Step 1. Clone the repository
-
-```bash
- git clone https://github.com/RedisGrafana/grafana-redis-datasource
- cd grafana-redis-datasource
-```
-
-#### Step 2. Execute the docker-compose CLI
-
-The project provides a `docker-compose.yml` file tha starts Redis with all modules and Grafana.
-
-```bash
- docker-compose up -d
-```
-
-#### Step 3. Access Grafana dashboard
-
-
-
-#### Step 4. Click "Configuration"
-
-
-
-#### Step 5. Add Redis as a Data Source
-
-
-
-#### Step 6. Select "Redis" as data source type
-
-
-
-#### Step 7. Add Redis Database name, Endpoint URL and password
-
-We assume that you already have a Redis server up and running in your infrastructure.
-You can also leverage Redis Enterprise Cloud as shown below:
-
-
-
-#### Step 8. Click "Import" under Dashboard
-
-
-
-#### Step 9.Access the Redis datasource Dashboard
-
-
-
-
-
-
-#### Supported commands
-
-Data Source supports various Redis commands using custom components and provides a unified interface to query any command.
-
-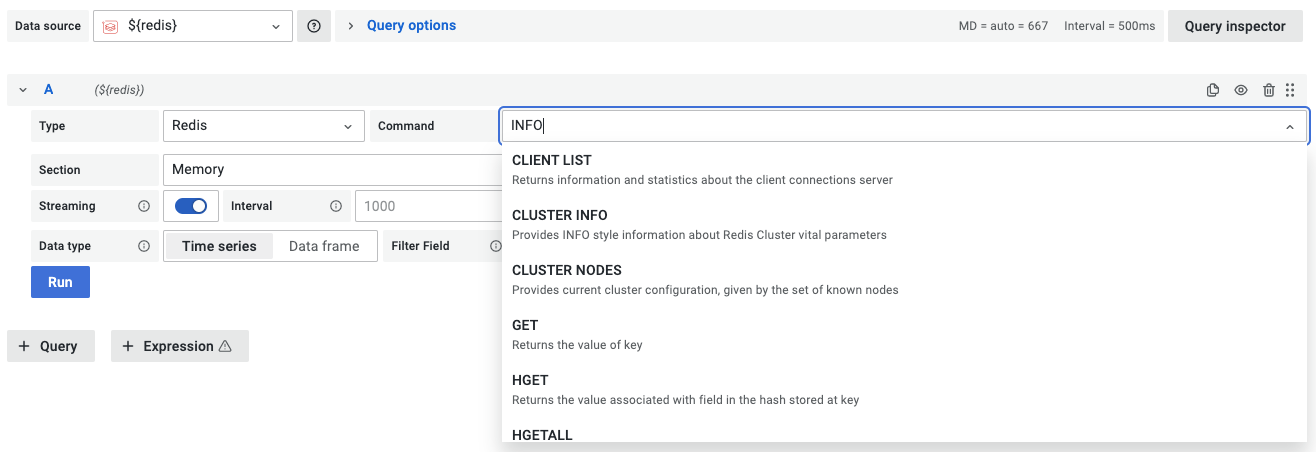
-
-
-
-
-
-### Further References
-
-- [Introducing the Redis Data Source Plug-in for Grafana](https://redis.com/blog/introducing-the-redis-data-source-plug-in-for-grafana/)
-- [How to Use the New Redis Data Source for Grafana Plug-in](https://redis.com/blog/how-to-use-the-new-redis-data-source-for-grafana-plug-in/)
-- [3 Real-Life Apps Built with Redis Data Source for Grafana](https://redis.com/blog/3-real-life-apps-built-with-redis-data-source-for-grafana/)
-- [How to Manage Real-Time IoT Sensor Data in Redis](https://redis.com/blog/how-to-manage-real-time-iot-sensor-data-in-redis/)
-- [Real-time observability with Redis and Grafana](https://grafana.com/go/observabilitycon/real-time-observability-with-redis-and-grafana/)
diff --git a/docs/support/observability/redisdatasource/redis.png b/docs/support/observability/redisdatasource/redis.png
deleted file mode 100644
index 62db87d..0000000
Binary files a/docs/support/observability/redisdatasource/redis.png and /dev/null differ
diff --git a/docs/support/provisioning/_azure-cache-terraform-private/index-azure-cache-terraform-private.mdx b/docs/support/provisioning/_azure-cache-terraform-private/index-azure-cache-terraform-private.mdx
deleted file mode 100644
index 33862ec..0000000
--- a/docs/support/provisioning/_azure-cache-terraform-private/index-azure-cache-terraform-private.mdx
+++ /dev/null
@@ -1,124 +0,0 @@
----
-id: index-azure-cache-terraform-private
-title: Azure Cache for Redis Enterprise using Terraform with Private Link
-sidebar_label: Azure Cache for Redis Enterprise using Terraform with Private Link
-slug: /operate/provisioning/azure-cache-terraform-private
----
-
-Azure Private Link for Azure Cache for Redis provides private connectivity from a virtual network to your cache instance. This means that you can now use Azure Private Link to connect to an Azure Cache for Redis instance from your virtual network via a private endpoint, which is assigned a private IP address in a subnet within the virtual network. It simplifies the network architecture and secures the connection between endpoints in Azure by eliminating data exposure to the public internet. Private Link carries traffic privately, reducing your exposure to threats and helps you meet compliance standards.
-
-Azure Resource Manager (a.k.a AzureRM) is the deployment and management service for Azure. It provides a management layer that enables you to create, update, and delete resources in your Azure account. You can use management features, like access control, locks, and tags, to secure and organize your resources after deployment. The "azurerm_redis_enterprise_cluster" is a resource that manages a Redis Enterprise cluster. This is a template to get started with the 'azurerm_redis_enterprise_cluster' resource available in the 'azurerm' provider with Terraform.
-
-### Prerequisites
-
-1. [Terraform](https://terraform.io)
-2. [Azure CLI](https://docs.microsoft.com/en-us/cli/azure/install-azure-cli)
-
-### Step 1. Getting Started
-
-Login in to Azure using the Azure CLI
-
-```bash
-az login
-```
-
-> Login with a Service Principal will also work
-
-Login using an Azure Service Principal
-
-```bash
-az login --service-principal --username APP_ID --tenant TENANT_ID --password [password || /path/to/cert]
-```
-
-### Step 2: Clone the repository
-
-```bash
-git clone https://github.com/redis-developer/acre-terraform
-```
-
-### Step 3: Initialize the repository
-
-```bash
-cd acre-terraform
-terraform init
-```
-
-> The output should include: `Terraform has been successfully initialized`
-
-### Step 4: Modify the variables (optional)
-
-The default variables are setup to deploy the smallest 'E10' instance into the 'East US' region.
-Changes can be made by updating the `variables.tf` file.
-
-### Step 5: Verify the plan
-
-The 'plan' output will show you everything being created by the template.
-
-```bash
-terraform plan
-```
-
-> The output should include: `Plan: 18 to add, 0 to change, 0 to destroy.`
-
-### Step 6: Apply the plan
-
-When the plan looks good, 'apply' the template.
-
-```bash
-terraform apply
-```
-
-> The output should include: `Apply complete! Resources: 18 added, 0 changed, 0 destroyed.`
-
-### Step 7: Connect using generated output
-
-The access key is sensitive, so viewing the outputs must be requested specifically.
-The output is also in JSON format.
-
-```bash
-terraform output redisgeek_config
-```
-
-> Example output:
-
-```
-{
-"hostname" = "redisgeek-8jy4.eastus.redisenterprise.cache.azure.net"
-"access_key" = "DQYABC3uRMXXXXXXXXXXXXXXXXTRkfgOXXXPjs82Y="
-"port" = "10000"
-}
-```
-
-### Resources
-
-##### 1. How to use Redis Cache for Redis like a Pro
-
-
-
-
-
-##### 2. Do More with Azure Cache for Redis, Enterprise Tiers
-
-
-
-
-
-### References
-
-- [Azure Cache for Redis Enterprise](https://azuremarketplace.microsoft.com/en-us/marketplace/apps/garantiadata.redis_enterprise_1sp_public_preview?ocid=redisga_redislabs_cloudpartner_cta1)
-- [Accelerate Modern Application Delivery with Redis Enterprise on Microsoft Azure](https://query.prod.cms.rt.microsoft.com/cms/api/am/binary/RWGGx3)
-- [Quickstart: Create a Redis Enterprise cache](https://docs.microsoft.com/en-us/azure/azure-cache-for-redis/quickstart-create-redis-enterprise)
diff --git a/docs/support/provisioning/_azure-cache-terraform/index-azure-cache-terraform.mdx b/docs/support/provisioning/_azure-cache-terraform/index-azure-cache-terraform.mdx
deleted file mode 100644
index adb02b9..0000000
--- a/docs/support/provisioning/_azure-cache-terraform/index-azure-cache-terraform.mdx
+++ /dev/null
@@ -1,135 +0,0 @@
----
-id: index-azure-cache-terraform
-title: Azure Cache for Redis Enterprise using Terraform
-sidebar_label: Azure Cache for Redis Enterprise using Terraform
-slug: /operate/provisioning/azure-cache-terraform
----
-
-The Enterprise Tiers of Azure Cache for Redis are generally available as a native fully managed service on Microsoft Azure. This offering combines Azure’s global presence, flexibility, security, and compliance with Redis Enterprise’s unmatched availability, performance, and extended data structure functionality to create the best experience for enterprises. Enterprise features include:
-
-- [Open source Redis 6.0](https://redis.com/blog/diving-into-redis-6/)
-- [Zone redundancy, with up to 99.99% availability](https://docs.microsoft.com/en-us/azure/azure-cache-for-redis/cache-high-availability#zone-redundancy)
-- [Active geo-replication, with up to 99.999% availability](https://redis.com/redis-enterprise/technology/active-active-geo-distribution/) - Preview
-- [Redis on Flash (RoF)](https://redis.com/redis-enterprise/technology/redis-on-flash/)
-- [Disk persistence with recovery](https://redis.com/redis-enterprise/technology/durable-redis/) - Preview
-- Redis Enterprise features:
- - [Search and Query](https://redis.com/blog/redisearch-2-build-modern-applications-interactive-search/)
- - [TimeSeries data model](https://redis.com/modules/redis-timeseries/)
- - [Probabilistic data model](https://redis.com/modules/redis-bloom/)
- - More feature details at [Redis Stack](https://redis.io/docs/stack/about/)
-- Scaling
- - Datasets up to 13TB
- - Up to 2M concurrent client connections
- - Over 1M ops/second
-- Security
- - [Private Link support](https://docs.microsoft.com/en-us/azure/azure-cache-for-redis/cache-private-link)
- - TLS connectivity
-- Integrated billing and the ability to apply Azure-commitment spend
-
-Azure Resource Manager (a.k.a AzureRM) is the deployment and management service for Azure. It provides a management layer that enables you to create, update, and delete resources in your Azure account. You use management features, like access control, locks, and tags, to secure and organize your resources after deployment.
-
-The "azurerm_redis_enterprise_cluster" is a resource that manages a Redis Enterprise cluster. This is a template to get started with the 'azurerm_redis_enterprise_cluster' resource available in the 'azurerm' provider with Terraform.
-
-### Prerequisites
-
-1. [Terraform CLI](https://terraform.io)
-2. [Azure CLI](https://docs.microsoft.com/en-us/cli/azure/install-azure-cli)
-
-### Step 1. Getting Started
-
-Login in to Azure using the Azure CLI
-
-```bash
-az login
-```
-
-### Step 2: Clone the repository
-
-```bash
-git clone https://github.com/redis-developer/acre-terraform-simple
-```
-
-### Step 3: Initialize the repository
-
-```bash
-cd acre-terraform-simple
-terraform init
-```
-
-> The output should include: `Terraform has been successfully initialized`
-
-### Step 4: Modify the variables(optional)
-
-The default variables are setup to deploy the smallest 'E10' instance into the 'East US' region.
-Changes can be made by updating the `variables.tf` file.
-
-### Step 5: Verify the plan
-
-The 'plan' output will show you everything being created by the template.
-
-```bash
-terraform plan
-```
-
-> The plan step does not make any changes in Azure
-
-### Step 6: Apply the plan
-
-When the plan looks good, 'apply' the template.
-
-```bash
-terraform apply
-```
-
-### Step 7: Connect using generated output
-
-The access key is sensitive, so viewing the outputs must be requested specifically.
-The output is also in JSON format.
-
-```bash
-terraform output redisgeek_config
-```
-
-> Example output:
-
-```
-{
-"hostname" = "redisgeek-8jy4.eastus.redisenterprise.cache.azure.net"
-"access_key" = "DQYABC3uRMyDguEXXXXXXXXXXWTRkfgOPjs82Y="
-"port" = "10000"
-}
-```
-
-### Resources
-
-##### How to use Redis Cache for Redis like a Pro
-
-
-
-
-
-##### Do More with Azure Cache for Redis, Enterprise Tiers
-
-
-
-
-
-### References
-
-- [Azure Cache for Redis Enterprise](https://azuremarketplace.microsoft.com/en-us/marketplace/apps/garantiadata.redis_enterprise_1sp_public_preview?ocid=redisga_redislabs_cloudpartner_cta1)
-- [Accelerate Modern Application Delivery with Redis Enterprise on Microsoft Azure](https://query.prod.cms.rt.microsoft.com/cms/api/am/binary/RWGGx3)
-- [Quickstart: Create a Redis Enterprise cache](https://docs.microsoft.com/en-us/azure/azure-cache-for-redis/quickstart-create-redis-enterprise)
diff --git a/docs/support/provisioning/_index-provisioning.mdx b/docs/support/provisioning/_index-provisioning.mdx
deleted file mode 100644
index 555726d..0000000
--- a/docs/support/provisioning/_index-provisioning.mdx
+++ /dev/null
@@ -1,21 +0,0 @@
----
-id: index-provisioning
-title: Provisioning
-sidebar_label: Overview
-slug: /operate/provisioning
----
-
-import RedisCard from '@theme/RedisCard';
-
-The following links are a guide to the various options to automate delivery of Redis to your organization.
-
-
-
-
-
-
-
diff --git a/docs/support/provisioning/terraform/accessmanagement.png b/docs/support/provisioning/terraform/accessmanagement.png
deleted file mode 100644
index d3ab123..0000000
Binary files a/docs/support/provisioning/terraform/accessmanagement.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/accessmanagement1.png b/docs/support/provisioning/terraform/accessmanagement1.png
deleted file mode 100644
index d935e06..0000000
Binary files a/docs/support/provisioning/terraform/accessmanagement1.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/index-terraform.mdx b/docs/support/provisioning/terraform/index-terraform.mdx
deleted file mode 100644
index 09fc421..0000000
--- a/docs/support/provisioning/terraform/index-terraform.mdx
+++ /dev/null
@@ -1,567 +0,0 @@
----
-id: index-terraform
-title: How to Deploy and Manage Redis Databases on AWS Using Terraform
-sidebar_label: Deploy and Manage Redis Database on AWS using Terraform
-slug: /operate/provisioning/terraform
-authors: [ajeet, rahul]
----
-
-
-
-
-
-
-
-Development teams today are embracing more and more DevOps principles, such as continuous integration and continuous delivery (CI/CD). Therefore, the need to manage infrastructure-as-code (IaC) has become an essential capability for any cloud service. IaC tools allow you to manage infrastructure with configuration files rather than through a graphical user interface. IaC allows you to build, change, and manage your infrastructure in a safe, consistent, and repeatable way by defining resource configurations that you can version, reuse, and share.
-
-A leading tool in the IaC space is HashiCorp Terraform, which supports the major cloud providers and services with its providers and modules cloud infrastructure automation ecosystem for provisioning, compliance, and management of any cloud, infrastructure, and service
-
-### What is Terraform?
-
-Terraform is an open source IaC software tool that provides a consistent CLI workflow to manage hundreds of cloud services. Terraform codifies cloud APIs into declarative configuration files, which can then be shared amongst team members, treated as code, edited, reviewed, and versioned. It enables you to safely and predictably create, change, and improve infrastructure.
-
-### Capabilities of Terraform
-
-- Terraform is not just a configuration management tool. It also focuses on the higher-level abstraction of the data center and associated services, while allowing you to use configuration management tools on individual systems.
-- It supports multiple cloud providers, such as AWS, GCP, Azure, DigitalOcean, etc.
-- It provides a single unified syntax, instead of requiring operators to use independent and non-interoperable tools for each platform and service.
-- Manages both existing service providers and custom in-house solutions.
-- Terraform is easily portable to any other provider.
-- Provides immutable infrastructure where configuration changes smoothly.
-- Supports client-only architecture, so no need for additional configuration management on a server.
-- Terraform is very flexible, using a plugin-based model to support providers and provisioners, giving it the ability to support almost any service that exposes APIs.
-- It is not intended to give low-level programmatic access to providers, but instead provides a high-level syntax for describing how cloud resources and services should be created, provisioned, and combined.
-- It provides a simple, unified syntax, allowing almost any resource to be managed without learning new tooling.
-
-### The HashiCorp Terraform Redis Enterprise Cloud provider
-
-Redis has developed a Terraform provider for Redis Enterprise Cloud. The HashiCorp Terraform Redis Enterprise Cloud provider allows customers to deploy and manage Redis Enterprise Cloud subscriptions, databases, and network peering easily as code, on any cloud provider. It is a plugin for Terraform that allows Redis Enterprise Cloud Flexible customers to manage the full life cycle of their subscriptions and related Redis databases.
-
-The Redis Enterprise Cloud provider is used to interact with the resources supported by Redis Enterprise Cloud. The provider needs to be configured with the proper credentials before it can be used. Use the navigation to the left to read about the available provider resources and data sources.
-
-
-
-Before we jump into the implementation, let us take a moment to better understand the Terraform configuration. A Terraform configuration is a complete document in the Terraform language that tells Terraform how to manage a given collection of infrastructure. A configuration can consist of multiple files and directories. Terraform is broken down into three main components:
-
-- Providers
-- Data sources
-- Resources
-
-### Providers
-
-A provider is the first resource that will need to be defined in any project under the Terraform configuration file. The provider gives you access to the API you will be interacting with to create resources. Once the provider has been configured and authenticated, a vast amount of resources are now available to be created. Terraform has more than 100+ cloud providers it serves.
-
-A provider defines resources and data for a particular infrastructure, such as AWS. As shown below, the terraform block {} contains terraform settings, including the required providers Terraform will use to provision your infrastructure (for example, rediscloud provider).
-
-```
- terraform {
- required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
- }
- }
-```
-
-The provider {} block configures the specific provider. In the following example, it is AWS.
-
-```
- cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
- }
-```
-
-### Resources
-
-Resources are the most important element in the Terraform language. This is where you describe the piece of infrastructure to be created, and this can range from a compute instance to defining specific permissions and much more.
-
-As shown below, the resource {} block is used to define components of your infrastructure. A resource might be a physical or virtual component, such as EC2, or it could be a logical component, such as a Heroku application.
-
-```
- resource "random_password" "passwords" {
- count = 2
- length = 20
- upper = true
- lower = true
- number = true
-}
-```
-
-The resource {} block has two strings before the block: resource types and resource names. The prefix of the type maps to the name of the provider. For example, the resource type “random_password” and the resource name “passwords” form a unique identifier of the resource. Terraform uses this ID to identify the resource.
-
-### Data sources
-
-Data sources allow Terraform to use information defined outside of Terraform, defined by another separate Terraform configuration, or modified by functions. Each provider may offer data sources alongside its set of resource types. A data source is accessed via a special kind of resource known as a data resource, declared using a data block.
-
-```
- data "rediscloud_payment_method" "card" {
- card_type = "Visa"
- last_four_numbers = "XXXX"
- }
-```
-
-A data block requests that Terraform read from a given data source ("rediscloud_payment_method") and export the result under the given local name ("card"). The name is used to refer to this resource from elsewhere in the same Terraform module, but has no significance outside of the scope of a module.
-
-Within the block body (between { and }) are query constraints defined by the data source. Most arguments in this section depend on the data source, and indeed in this example card_type and last_four_numbers are all arguments defined specifically for the rediscloud_payment_method data source.
-
-### Configure Redis Enterprise Cloud programmatic access
-
-In order to set up authentication with the Redis Enterprise Cloud provider, a programmatic API key must be generated for Redis Enterprise Cloud. The Redis Enterprise Cloud documentation contains the most up-to-date instructions for creating and managing your key(s) and IP access.
-
-:::tip
-
-Flexible and Annual Redis Enterprise Cloud subscriptions can leverage a RESTful API that permits operations against a variety of resources, including servers, services, and related infrastructure. The REST API is not supported for Fixed or Free subscriptions.
-
-:::
-
-```
- provider "rediscloud" { } # Example resource configuration
- resource "rediscloud_subscription" "example" { # ... }
-```
-
-### Prerequisites:
-
-- Install Terraform on MacOS.
-- Create a free Redis Enterprise Cloud account.
-- Create your first subscription.
-- Enable API
-
-### Step 1: Install Terraform on MacOS
-
-Use Homebrew to install Terraform on MacOS as shown below:
-
-```
- brew install terraform
-```
-
-### Step 2: Sign up for a free Redis Enterprise Cloud account
-
-[Follow this tutorial](https://developer.redis.com/create/aws/redis-on-aws) to sign up for a free Redis Enterprise Cloud account.
-
-
-
-### Step 3: Enable Redis Enterprise Cloud API
-
-If you have a Flexible (or Annual) Redis Enterprise Cloud subscription, you can use a REST API to manage your subscription programmatically. The Redis Cloud REST API is available only to Flexible or Annual subscriptions. It is not supported for Fixed or Free subscriptions.
-
-For security reasons, the Redis Cloud API is disabled by default. To enable the API:
-
-- Sign in to your Redis Cloud subscription as an account owner.
-- From the menu, choose Access Management.
-
-- When the Access Management screen appears, select the API Keys tab.
-
-
-
-If a Copy button appears to the right of the API account key, the API is enabled. This button copies the account key to the clipboard.
-
-If you see an Enable API button, select it to enable the API and generate your API account key.
-
-To authenticate REST API calls, you need to combine the API account key with an API user key to make API calls.
-
-
-
-### Step 4: Create a main.tf file
-
-It’s time to create an empty “main.tf” file and start adding the provider, resource and data sources as shown below:
-
-```
- terraform {
- required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
- }
- }
-# Provide your credit card details
-data "rediscloud_payment_method" "card" {
-card_type = "Visa"
-last_four_numbers = "XXXX"
-}
-# Generates a random password for the database
-resource "random_password" "passwords" {
-count = 2
-length = 20
-upper = true
-lower = true
-number = true
-special = false
-}
-resource "rediscloud_subscription" "rahul-test-terraform" {
-name = "rahul-test-terraform"
-payment_method_id = data.rediscloud_payment_method.card.id
-memory_storage = "ram"
-cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
-}
-database {
- name = "db-json"
- protocol = "redis"
- memory_limit_in_gb = 1
- replication = true
- data_persistence = "aof-every-1-second"
- module {
- name = "RedisJSON"
- }
- throughput_measurement_by = "operations-per-second"
- throughput_measurement_value = 10000
- password = random_password.passwords[1].result
-}
-}
-```
-
-### Step 5: Create an execution plan
-
-The Terraform plan command creates an execution plan, which lets you preview the changes that Terraform plans to make to your infrastructure. By default, when Terraform creates a plan, it reads the current state of any already existing remote objects to make sure that Terraform state is up to date. It then compares the current configuration to the prior state and then proposes a set of change actions that should make the remote object match the configuration.
-
-```
- % terraform plan
-
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- + create
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # random_password.passwords[1] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # rediscloud_subscription.rahul-test-terraform will be created
- + resource "rediscloud_subscription" "rahul-test-terraform" {
- + id = (known after apply)
- + memory_storage = "ram"
- + name = "rahul-test-terraform"
- + payment_method_id = "XXXX"
- + persistent_storage_encryption = true
-
- + cloud_provider {
- + cloud_account_id = "1"
- + provider = "AWS"
-
- + region {
- + multiple_availability_zones = false
- + networking_deployment_cidr = "10.0.0.0/24"
- + networks = (known after apply)
- + preferred_availability_zones = [
- + "us-east-1a",
- ]
- + region = "us-east-1"
- }
- }
-
- + database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 3 to add, 0 to change, 0 to destroy.
-
-───────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────────
-
-:::note
-
-You didn't use the -out option to save this plan, so Terraform can't guarantee to take exactly these actions if you run "terraform apply" now.
-
-:::
-
-```
-
-### Step 6: Execute the action
-
-The Terraform apply command executes the actions proposed in a Terraform plan.
-
-```
- terraform apply
-
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- + create
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # random_password.passwords[1] will be created
- + resource "random_password" "passwords" {
- + id = (known after apply)
- + length = 20
- + lower = true
- + min_lower = 0
- + min_numeric = 0
- + min_special = 0
- + min_upper = 0
- + number = true
- + result = (sensitive value)
- + special = false
- + upper = true
- }
-
- # rediscloud_subscription.rahul-test-terraform will be created
- + resource "rediscloud_subscription" "rahul-test-terraform" {
- + id = (known after apply)
- + memory_storage = "ram"
- + name = "rahul-test-terraform"
- + payment_method_id = "XXXX"
- + persistent_storage_encryption = true
-
- + cloud_provider {
- + cloud_account_id = "1"
- + provider = "AWS"
-
- + region {
- + multiple_availability_zones = false
- + networking_deployment_cidr = "10.0.0.0/24"
- + networks = (known after apply)
- + preferred_availability_zones = [
- + "us-east-1a",
- ]
- + region = "us-east-1"
- }
- }
-
- + database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 3 to add, 0 to change, 0 to destroy.
-
-Do you want to perform these actions?
- Terraform will perform the actions described above.
- Only 'yes' will be accepted to approve.
-
- Enter a value: yes
-
-random_password.passwords[0]: Creating...
-random_password.passwords[1]: Creating...
-random_password.passwords[1]: Creation complete after 0s [id=none]
-random_password.passwords[0]: Creation complete after 0s [id=none]
-rediscloud_subscription.rahul-test-terraform: Creating...
-rediscloud_subscription.rahul-test-terraform: Still creating... [10s elapsed]
-rediscloud_subscription.rahul-test-terraform: Still creating... [20s elapsed]
-rediscloud_subscription.rahul-test-terraform: Creation complete after 8m32s [id=1649277]
-
-Apply complete! Resources: 3 added, 0 changed, 0 destroyed.
-```
-
-### Step 7: Verify the database
-
-You can now verify the new database created under Subscription named “db-json.”
-
-Deploy a Redis Database with JSON and [other]([Redis Stack](https://redis.io/docs/stack/about/)
-) features on AWS using Terraform:
-
-```
-terraform {
-required_providers {
- rediscloud = {
- source = "RedisLabs/rediscloud"
- version = "0.2.2"
- }
-}
-}
-# Provide your credit card details
-data "rediscloud_payment_method" "card" {
-card_type = "Visa"
-last_four_numbers = "XXXX"
-}
-# Generates a random password for the database
-resource "random_password" "passwords" {
-count = 2
-length = 20
-upper = true
-lower = true
-number = true
-special = false
-}
-resource "rediscloud_subscription" "rahul-test-terraform" {
-name = "rahul-test-terraform"
-payment_method_id = data.rediscloud_payment_method.card.id
-memory_storage = "ram"
-cloud_provider {
-
- provider = "AWS"
- cloud_account_id = 1
- region {
- region = "us-east-1"
- networking_deployment_cidr = "10.0.0.0/24"
- preferred_availability_zones = ["us-east-1a"]
- }
-}
-database {
- name = "db-json"
- protocol = "redis"
- memory_limit_in_gb = 1
- replication = true
- data_persistence = "aof-every-1-second"
- module {
- name = "RedisJSON"
- }
- throughput_measurement_by = "operations-per-second"
- throughput_measurement_value = 10000
- password = random_password.passwords[1].result
-}
-}
-```
-
-### Step 8: Cleanup
-
-The Terraform destroy command is a convenient way to destroy all remote objects managed by a particular Terraform configuration. While you will typically not want to destroy long-lived objects in a production environment, Terraform is sometimes used to manage ephemeral infrastructure for development purposes, in which case you can use terraform destroy’ to conveniently clean up all of those temporary objects once you are finished with your work.
-
-```
-% terraform destroy
-random_password.passwords[0]: Refreshing state... [id=none]
-random_password.passwords[1]: Refreshing state... [id=none]
-rediscloud_subscription.rahul-test-terraform: Refreshing state... [id=1649277]
-
-Terraform used the selected providers to generate the following execution plan. Resource actions are indicated with the following symbols:
- - destroy
-
-Terraform will perform the following actions:
-
- # random_password.passwords[0] will be destroyed
- - resource "random_password" "passwords" {
- - id = "none" -> null
- - length = 20 -> null
- - lower = true -> null
- - min_lower = 0 -> null
- - min_numeric = 0 -> null
- - min_special = 0 -> null
- - min_upper = 0 -> null
- - number = true -> null
- - result = (sensitive value)
- - special = false -> null
- - upper = true -> null
- }
-
- # random_password.passwords[1] will be destroyed
- - resource "random_password" "passwords" {
- - id = "none" -> null
- - length = 20 -> null
- - lower = true -> null
- - min_lower = 0 -> null
- - min_numeric = 0 -> null
- - min_special = 0 -> null
- - min_upper = 0 -> null
- - number = true -> null
- - result = (sensitive value)
- - special = false -> null
- - upper = true -> null
- }
-
- # rediscloud_subscription.rahul-test-terraform will be destroyed
- - resource "rediscloud_subscription" "rahul-test-terraform" {
- - id = "1649277" -> null
- - memory_storage = "ram" -> null
- - name = "rahul-test-terraform" -> null
- - payment_method_id = "XXXX" -> null
- - persistent_storage_encryption = true -> null
-
- - cloud_provider {
- - cloud_account_id = "1" -> null
- - provider = "AWS" -> null
-
- - region {
- - multiple_availability_zones = false -> null
- - networking_deployment_cidr = "10.0.0.0/24" -> null
- - networks = [
- - {
- - networking_deployment_cidr = "10.0.0.0/24"
- - networking_subnet_id = "subnet-0055e8e3ee3ea796e"
- - networking_vpc_id = ""
- },
- ] -> null
- - preferred_availability_zones = [
- - "us-east-1a",
- ] -> null
- - region = "us-east-1" -> null
- }
- }
-
- - database {
- # At least one attribute in this block is (or was) sensitive,
- # so its contents will not be displayed.
- }
- }
-
-Plan: 0 to add, 0 to change, 3 to destroy.
-
-Do you really want to destroy all resources?
- Terraform will destroy all your managed infrastructure, as shown above.
- There is no undo. Only 'yes' will be accepted to confirm.
-
- Enter a value: yes
-
-rediscloud_subscription.rahul-test-terraform: Destroying... [id=1649277]
-…
-rediscloud_subscription.rahul-test-terraform: Destruction complete after 1m34s
-random_password.passwords[0]: Destroying... [id=none]
-random_password.passwords[1]: Destroying... [id=none]
-random_password.passwords[0]: Destruction complete after 0s
-random_password.passwords[1]: Destruction complete after 0s
-
-Destroy complete! Resources: 3 destroyed.
-```
-
-### Further References:
-
-- [Provision and Manage Redis Enterprise Cloud Anywhere with HashiCorp Terraform](https://redis.com/blog/provision-manage-redis-enterprise-cloud-hashicorp-terraform/)
-- [The HashiCorp Terraform Redis Enterprise Cloud provider](https://registry.terraform.io/providers/RedisLabs/rediscloud/latest)
diff --git a/docs/support/provisioning/terraform/newsubscription.png b/docs/support/provisioning/terraform/newsubscription.png
deleted file mode 100644
index b69d9c5..0000000
Binary files a/docs/support/provisioning/terraform/newsubscription.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/subscription.png b/docs/support/provisioning/terraform/subscription.png
deleted file mode 100644
index 6016836..0000000
Binary files a/docs/support/provisioning/terraform/subscription.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/subscription2.png b/docs/support/provisioning/terraform/subscription2.png
deleted file mode 100644
index 9be6010..0000000
Binary files a/docs/support/provisioning/terraform/subscription2.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/terraform_arch.png b/docs/support/provisioning/terraform/terraform_arch.png
deleted file mode 100644
index 4ac779e..0000000
Binary files a/docs/support/provisioning/terraform/terraform_arch.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/terraform_rediscloud.png b/docs/support/provisioning/terraform/terraform_rediscloud.png
deleted file mode 100644
index 6699e7a..0000000
Binary files a/docs/support/provisioning/terraform/terraform_rediscloud.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/tryfree.png b/docs/support/provisioning/terraform/tryfree.png
deleted file mode 100644
index d5188c7..0000000
Binary files a/docs/support/provisioning/terraform/tryfree.png and /dev/null differ
diff --git a/docs/support/provisioning/terraform/verifydatabase.png b/docs/support/provisioning/terraform/verifydatabase.png
deleted file mode 100644
index da78e22..0000000
Binary files a/docs/support/provisioning/terraform/verifydatabase.png and /dev/null differ
diff --git a/docs/support/redis-at-scale/course-wrap-up/index-wrap-up.mdx b/docs/support/redis-at-scale/course-wrap-up/index-wrap-up.mdx
deleted file mode 100644
index 8b60b56..0000000
--- a/docs/support/redis-at-scale/course-wrap-up/index-wrap-up.mdx
+++ /dev/null
@@ -1,45 +0,0 @@
----
-id: index-wrap-up
-title: Conclusion of Running Redis at Scale
-sidebar_label: Course Wrap-up
-slug: /operate/redis-at-scale/course-wrap-up/
-authors: [justin, elena, kurt]
----
-
-
-
-
-
-You've made it! Thanks again for trying out this course on the Redis Developer Site. We hope you've enjoyed it, and we hope it's provided you with the tools you need to successfully scale Redis with your applications.
-
-
-
-
-
-If you would like to receive a certificate of completion for this course, head to [Redis University](https://university.redis.com/courses/ru301/) to enroll in the full-format class which includes homework for each course section. If you pass the course with a grade of sixty-five percent or greater, you'll be able to generate your certificate and post it to your LinkedIn profile.
-
-
-
-
-
-
-Please consider subscribing to our [Youtube Channel](https://www.youtube.com/redisinc) to stay up to date with all of our latest tutorials, interviews, and general news.
-
-And if you have any feedback or insights you want to share with the Redis University team, don't hesitate to leave a note in our online chat on our Discord server found [here](https://discord.gg/WT6fbUMuWU).
-
-Again, we're grateful you've taken the time to work through our course. Happy learning and see you next time!
-
-Best wishes,
-
-Elena, Kurt, and Justin
diff --git a/docs/support/redis-at-scale/high-availability/basic-replication/index-basic-replication.mdx b/docs/support/redis-at-scale/high-availability/basic-replication/index-basic-replication.mdx
deleted file mode 100644
index 0cd8478..0000000
--- a/docs/support/redis-at-scale/high-availability/basic-replication/index-basic-replication.mdx
+++ /dev/null
@@ -1,40 +0,0 @@
----
-id: index-basic-replication
-title: 3.1 Basic Replication
-sidebar_label: 3.1 Basic Replication
-slug: /operate/redis-at-scale/high-availability/basic-replication
-custom_edit_url: null
----
-
-
-
-
-
-
-
- Replication in Redis follows a simple primary-replica model where the
- replication happens in one direction - from the primary to one or multiple
- replicas. Data is only written to the primary instance and replicas are kept
- in sync so that they’re exact copies of the primaries.
-
-
-To create a replica, you instantiate a Redis server instance with the configuration directive replicaof set to the address and port of the primary instance. Once the replica instance is up and running, the replica will try to sync with the primary. To transfer all of its data as efficiently as possible, the primary instance will produce a compacted version of the data in a snapshot (.rdb) file and send it to the replica.
-
-The replica will then read the snapshot file and load all of its data into memory, which will bring it to the same state the primary instance had at the moment of creating the .rdb file. When the loading stage is done, the primary instance will send the backlog of any write commands run since the snapshot was made. Finally, the primary instance will send the replica a live stream of all subsequent commands.
-
-By default, replication is asynchronous. This means that if you send a write command to Redis you will receive your acknowledged response first, and only then will the command be replicated to the replica.
-
-If the primary goes down after acknowledging a write but before the write can be replicated, then you might have data loss. To avoid this, the client can use the WAIT command. This command blocks the current client until all of the previous write commands are successfully transferred and acknowledged by at least some specified number of replicas.
-
-For example, if we send the command WAIT 2 0, the client will block (will not return a response to the client) until all of the previous write commands issued on that connection have been written to at least 2 replicas. The second argument - 0 - will instruct the server to block indefinitely, but we could set it to a number (in milliseconds) so that it times out after a while and returns the number of replicas that successfully acknowledged the commands.
-
-Replicas are read-only. This means that you can configure your clients to read from them, but you cannot write data to them. If you need additional read throughput, you can configure your Redis client to read from replicas as well as from your primary node. However, it's often easier just to scale out your cluster. This lets you scale reads and writes without writing any complex client logic.
-
-Also, you should know about Active-Active, an advanced feature of Redis Enterprise and Redis Cloud. Active-Active replicates entire databases across geographically-distributed clusters. With Active-Active, you can write locally to any replica databases, and those writes will be reflected globally. Something to keep in mind when you're really scaling out!
diff --git a/docs/support/redis-at-scale/high-availability/exercise-1/index-exercise-1.mdx b/docs/support/redis-at-scale/high-availability/exercise-1/index-exercise-1.mdx
deleted file mode 100644
index 787b740..0000000
--- a/docs/support/redis-at-scale/high-availability/exercise-1/index-exercise-1.mdx
+++ /dev/null
@@ -1,106 +0,0 @@
----
-id: index-exercise-1
-title: 3.2 Exercise - Enabling Basic Replication
-sidebar_label: 3.2 Exercise - Enabling Basic Replication
-slug: /operate/redis-at-scale/high-availability/exercise-1
-custom_edit_url: null
----
-
-## Step 1
-
-First let’s create and configure the primary instance. We’ll start with a few configuration changes in its `primary.conf` configuration file.
-
-```bash
-$ touch primary.conf # Create the configuration file
-```
-
-Now open the `primary.conf` file with your favorite text editor and set the following configuration directives:
-
-```bash
-# Create a strong password here
-requirepass a_strong_password
-
-# AUTH password of the primary instance in case this instance becomes a replica
-masterauth a_strong_password
-
-# Enable AOF file persistence
-appendonly yes
-
-# Choose a name for the AOF file
-appendfilename "primary.aof"
-```
-
-Finally, let’s start the primary instance:
-
-```bash
-$ redis-server ./primary.conf
-```
-
-## Step 2
-
-Next, let’s prepare the configuration file for the replica:
-
-```bash
-$ touch replica.conf
-```
-
-Let’s add some settings to the file we just created:
-
-```bash
-# Port on which the replica should run
-port 6380
-
-# Address of the primary instance
-replicaof 127.0.0.1 6379
-
-# AUTH password of the primary instance
-masterauth a_strong_password
-
-# AUTH password for the replica instance
-requirepass a_strong_password
-```
-
-And let’s start the replica:
-
-```bash
-$ redis-server ./replica.conf
-```
-
-## Step 3
-
-Open two terminal tabs and use them to start connections to the primary and replica instances:
-
-```bash
-# Tab 1 (primary)
-$ redis-cli
-```
-
-```bash
-# Tab 2 (replica)
-$ redis-cli -p 6380
-```
-
-Authenticate on both tabs by running the command `AUTH` followed by your password:
-
-```bash
-AUTH a_strong_password
-```
-
-On the second (replica) tab run the `MONITOR` command which will allow you to see every command executed against that instance.
-
-Go back to the first (primary) tab and execute any write command, for example
-
-```bash
-127.0.0.1:6379> SET foo bar
-```
-
-In the second tab you should see that the command was already sent to the replica:
-
-```bash
-1617230062.389077 [0 127.0.0.1:6379] "SELECT" "0"
-1617230062.389092 [0 127.0.0.1:6379] "set" "foo" "bar"
-```
-
-## Step 4
-
-Keep the instances running, or at least their configuration files around. We’ll need them for the next exercise.
diff --git a/docs/support/redis-at-scale/high-availability/exercise-2/index-exercise-2.mdx b/docs/support/redis-at-scale/high-availability/exercise-2/index-exercise-2.mdx
deleted file mode 100644
index d55a145..0000000
--- a/docs/support/redis-at-scale/high-availability/exercise-2/index-exercise-2.mdx
+++ /dev/null
@@ -1,90 +0,0 @@
----
-id: index-exercise-2
-title: 3.4 Exercise - Sentinel Hands-on
-sidebar_label: 3.4 Exercise - Sentinel Hands-on
-slug: /operate/redis-at-scale/high-availability/exercise-2
-custom_edit_url: null
----
-
-## Step 1
-
-If you still have the primary and replica instances we set up in the previous exercise (3.2) - great! We’ll reuse them to create our Sentinel setup. If not - refer back to the [instructions](/operate/redis-at-scale/high-availability/exercise-1) and go through them again.
-
-When done, you will have a primary Redis instance with one replica.
-
-## Step 2
-
-To initialise a Redis Sentinel, you need to provide a configuration file, so let’s go ahead and create one:
-
-```bash
-$ touch sentinel1.conf
-```
-
-Open the file and paste in the following settings:
-
-```bash
-port 5000
-sentinel monitor myprimary 127.0.0.1 6379 2
-sentinel down-after-milliseconds myprimary 5000
-sentinel failover-timeout myprimary 60000
-sentinel auth-pass myprimary a_strong_password
-```
-
-Breakdown of terms:
-
-- `port` - The port on which the Sentinel should run
-- `sentinel monitor` - monitor the Primary on a specific IP address and port. Having the address of the Primary the Sentinels will be able to discover all the replicas on their own. The last argument on this line is the number of Sentinels needed for quorum. In our example - the number is 2.
-- `sentinel down-after-milliseconds` - how many milliseconds should an instance be unreachable so that it’s considered down
-- `sentinel failover-timeout` - if a Sentinel voted another Sentinel for the failover of a given master, it will wait this many milliseconds to try to failover the same master again.
-- `sentinel auth-pass` - In order for Sentinels to connect to Redis server instances when they are configured with `requirepass`, the Sentinel configuration must include the sentinel auth-pass directive.
-
-## Step 3
-
-Make two more copies of this file - `sentinel2.conf` and `sentinel3.conf` and edit them so that the `PORT` configuration is set to 5001 and 5002, respectively.
-
-## Step 4
-
-Let’s initialise the three Sentinels in three different terminal tabs:
-
-```bash
-# Tab 1
-$ redis-server ./sentinel1.conf --sentinel
-```
-
-```bash
-# Tab 2
-$ redis-server ./sentinel2.conf --sentinel
-```
-
-```bash
-# Tab3
-$ redis-server ./sentinel3.conf --sentinel
-```
-
-## Step 5
-
-If you connected to one of the Sentinels now you would be able to run many new commands that would give an error if run on a Redis instance. For example:
-
-```bash
-# Provides information about the Primary
-SENTINEL master myprimary
-
-# Gives you information about the replicas connected to the Primary
-SENTINEL replicas myprimary
-
-# Provides information on the other Sentinels
-SENTINEL sentinels myprimary
-
-# Provides the IP address of the current Primary
-SENTINEL get-master-addr-by-name myprimary
-```
-
-## Step 6
-
-If we killed the primary Redis instance now by pressing Ctrl+C or by running the `redis-cli -p 6379 DEBUG sleep 30` command, we’ll be able to observe in the Sentinels’ logs that the failover process will start in about 5 seconds. If you run the command that returns the IP address of the Primary again you will see that the replica has been promoted to a Primary:
-
-```bash
-redis> SENTINEL get-master-addr-by-name myprimary
-1) "127.0.0.1"
-2) "6380"
-```
diff --git a/docs/support/redis-at-scale/high-availability/index-high-availability.mdx b/docs/support/redis-at-scale/high-availability/index-high-availability.mdx
deleted file mode 100644
index 256935c..0000000
--- a/docs/support/redis-at-scale/high-availability/index-high-availability.mdx
+++ /dev/null
@@ -1,10 +0,0 @@
----
-id: index-high-availability
-title: Ensuring High Availability in Redis
-sidebar_label: High Availability
-slug: /operate/redis-at-scale/high-availability
-authors: [justin]
-custom_edit_url: null
----
-
-Hello World!
diff --git a/docs/support/redis-at-scale/high-availability/introduction/index-index-introduction.mdx b/docs/support/redis-at-scale/high-availability/introduction/index-index-introduction.mdx
deleted file mode 100644
index 85cae71..0000000
--- a/docs/support/redis-at-scale/high-availability/introduction/index-index-introduction.mdx
+++ /dev/null
@@ -1,34 +0,0 @@
----
-id: index-introduction
-title: 3.0 Introduction to High Availability
-sidebar_label: 3.0 Introduction to High Availability
-slug: /operate/redis-at-scale/high-availability/introduction
-custom_edit_url: null
----
-
-
-
-
-
-
-
- High availability is a computing concept describing systems that guarantee a
- high level of uptime, designed to be fault-tolerant, highly dependable,
- operating continuously without intervention and without a single point of
- failure.
-
-
-What does this mean for Redis specifically? Well, it means that if your primary Redis server fails, a backup will kick in, and you, as a user, will see little to no disruption in the service. There are two components needed for this to be possible: replication and automatic failover.
-
-Replication is the continuous copying of data from a primary database to a backup, or a replica database. The two databases are usually located on different physical servers, so that we can have a functional copy of our data in case we lose the server where our primary database sits.
-
-But having a backup of our data is not enough for high availability. We also have to have a mechanism that will automatically kick in and redirect all requests towards the replica in the event that the primary fails. This mechanism is called automatic failover.
-
-In the rest of this section we’ll see how Redis handles replication and which automatic failover solutions it offers. Let’s dig in.
diff --git a/docs/support/redis-at-scale/high-availability/understanding-sentinels/index-understanding-sentinels.mdx b/docs/support/redis-at-scale/high-availability/understanding-sentinels/index-understanding-sentinels.mdx
deleted file mode 100644
index 4f9d9b9..0000000
--- a/docs/support/redis-at-scale/high-availability/understanding-sentinels/index-understanding-sentinels.mdx
+++ /dev/null
@@ -1,42 +0,0 @@
----
-id: index-understanding-sentinels
-title: 3.3 Understanding Sentinels
-sidebar_label: 3.3 Understanding Sentinels
-slug: /operate/redis-at-scale/high-availability/understanding-sentinels
-custom_edit_url: null
----
-
-In the beginning of this unit, we learned that we can’t have high availability without replication and automatic failover. We covered replication in the previous two chapters, and now we’ll explain Sentinel - a tool that provides the automatic failover.
-
-Redis Sentinel is a distributed system consisting of multiple Redis instances started in sentinel mode. We call these instances Sentinels.
-
-The group of Sentinels monitors a primary Redis instance and its replicas. If the sentinels detect that the primary instance has failed, the sentinel processes will look for the replica that has the latest data and will promote that replica to be the new primary. This way, the clients talking to the database will be able to reconnect to the new primary and continue functioning as usual, with minimal disruption to the users.
-
-
-
-
-
-## Deciding that a primary instance is down
-
-In order for the Sentinels to be able to decide that a primary instance is down we need to have enough Sentinels agree that the server is unreachable from their point of view.
-
-Having a number of Sentinels agreeing that they need to take an action is called reaching a quorum. If the Sentinels can’t reach quorum, they cannot decide that the primary has failed. The exact number of Sentinels needed for quorum is configurable.
-
-## Triggering a failover
-
-Once the Sentinels have decided that a primary instance is down, they need to elect and authorize a leader (a Sentinel instance) that will do the failover. A leader can only be chosen if the majority of the Sentinels agree on it.
-
-In the final step, the leader will reconfigure the chosen replica to become a primary by sending the command `REPLICAOF NO ONE` and it will reconfigure the other replicas to follow the newly promoted primary.
-
-## Sentinel and Client Libraries
-
-If you have a system that uses Sentinel for high availability, then you need to have a client that supports Sentinel. Not all libraries have this feature, but most of the popular ones do, so make sure you add it to your list of requirements when choosing your library.
-
-## Further Reading
-
-For more information on Redis Sentinel, check out the [documentation](https://redis.io/topics/sentinel) on redis.io.
diff --git a/docs/support/redis-at-scale/index-redis-at-scale.mdx b/docs/support/redis-at-scale/index-redis-at-scale.mdx
deleted file mode 100644
index 70f0642..0000000
--- a/docs/support/redis-at-scale/index-redis-at-scale.mdx
+++ /dev/null
@@ -1,81 +0,0 @@
----
-id: index-redis-at-scale
-title: Introduction to Running Redis at Scale
-sidebar_label: Overview
-slug: /operate/redis-at-scale
-authors: [justin, elena, kurt]
-custom_edit_url: null
----
-
-
-
-
-
-## Welcome
-
-
-
-
-
-
-
-
- The world's data is growing exponentially. That exponential growth means that
- database systems must scale. This is a course about running Redis, one of the
- most popular databases, at scale.
-
-
-So, how do you run Redis at scale? There are two general answers to this question, and it's important that we address them right away. That's because the easiest and most common way to run Redis at scale is to let someone else manage your Redis deployment for you.
-
-The convenience of "database-as-a-service" offerings means that you don't have to know much about how your database scales, and that saves a lot of time and potential false starts.
-
-We at Redis offer Redis Cloud, a highly available cloud-based Redis service that provides a lot of features you can't find anywhere else, like active-active, geo-distribution.
-
-Redis Cloud is also really easy to use and has a free tier so you can get going quickly. So, that's the first answer. To run Redis these days, you might just use a fully-managed offering like Redis Cloud. But not everyone can or wants to use a cloud-hosted database.
-
-There are a bunch of reasons for this. For example, maybe you're a large enterprise with your own data centers and dedicated ops teams. Or perhaps you're a mission-critical application whose SLAs are so rigid that you need to be able to dig deeply into any potential performance issue. This often rules out cloud-based deployments, since the cloud hides away the hardware and networks you're operating in. In this case, you're deploying Redis on your own. And for that, you need to know how Redis scales.
-
-Learning this isn't just useful; it's also genuinely interesting. Sharding, replication, high availability, and disaster recovery are all important concepts that anyone can understand with the right explanation. These concepts aren't rocket science. They're no harder to understand than basic high school math, and knowing about them makes you a better developer.In this course, we'll look closely at how open source Redis scales. And you'll learn by doing, as we present a lot of the ideas through hands-on labs.
-
-These ideas will apply whether you're deploying open source Redis on your own or managing a Redis Enterprise cluster - which is, ultimately, what you'll want to reach for if you ever outgrow open source Redis. These are some important topics to consider during your time with this course. But let's first learn how to walk before we run.
-
-We sincerely hope you enjoy what you learn with us about scaling Redis, and as always, it's my pleasure to help.
-
-## Course Overview
-
-This course is broken up into units covering topics around scaling Redis for production deployment.
-
-Scaling means more than just performance. We have tried to identify key topics that will help you have a performant, stable, and secure deployment of Redis. This course is divided into the following units:
-
-- Talking to Redis: connection management and tuning of Redis.
-- Persistence/Durability: options persisting Redis data to disk.
-- High Availability: how to make sure Redis and your data is always there.
-- Scalability: scaling Redis for both higher throughput and capacity.
-- Observability: Visibility into your Redis deployment (metrics, etc.).
-
-Our goal is to give you all the information you need to run Redis at scale, in whichever way is best for your organization. We hope you enjoy the course, and please don't hesitate to reach out on the course [Discord channel](https://discord.gg/ZnjZbDMDub) if you have any questions along the way.
-
-## Prerequisites
-
-- Access to a Linux-based system and familiarity with it
-- Redis server and redis-cli installed (examples and exercises assume redis-server is in the $PATH)
- docker and docker-compose installed
-- A git client and access to clone repos in Github. Some exercises will come from the following repository: https://github.com/redislabs-training/ru301
-
-:::note
-
-This repo contains sample demonstrations of Redis running in various scaled configurations, and is not directly correlated with all of the exercises in this course. See the specific exercise instructions for usage.
-
-:::
-
-## Assumptions
-
-- Comfortable with Linux Bash shell exercises
-- Legacy terminology in Redis uses 'master' and 'slave' but in the course we will use 'primary' and 'replica'. You will still see the legacy terms in many commands, configurations, and field names.
-- We will use $ to indicate command line prompt and > to indicate a redis-cli prompt
diff --git a/docs/support/redis-at-scale/observability/data-points-in-redis/index-data-points-in-redis.mdx b/docs/support/redis-at-scale/observability/data-points-in-redis/index-data-points-in-redis.mdx
deleted file mode 100644
index 4f28532..0000000
--- a/docs/support/redis-at-scale/observability/data-points-in-redis/index-data-points-in-redis.mdx
+++ /dev/null
@@ -1,358 +0,0 @@
----
-id: index-data-points-in-redis
-title: 5.1 Data points in Redis
-sidebar_label: 5.1 Data points in Redis
-slug: /operate/redis-at-scale/observability/data-points-in-redis
-custom_edit_url: null
----
-
-There are several Redis metrics that can be viewed through `redis-cli`.
-
-## Redis INFO command
-
-Running the `INFO` command provides many of the metrics available in a single view.
-
-```bash
-127.0.0.1:6379> INFO
-# Server
-redis_version:6.0.1
-redis_git_sha1:00000000
-redis_git_dirty:0
-redis_build_id:e02d1d807e41d65
-redis_mode:standalone
-os:Linux 4.19.121-linuxkit x86_64
-…
-```
-
-There are several sections that can be pulled individually. For example, if you wanted to just get the `CLIENTS` section you can pass that section as an argument to the `INFO` command.
-
-```bash
-127.0.0.1:6379> INFO CLIENTS
-# Clients
-connected_clients:1
-client_recent_max_input_buffer:2
-client_recent_max_output_buffer:0
-blocked_clients:0
-tracking_clients:0
-clients_in_timeout_table:0
-```
-
-## Sections
-
-Server: the current Redis server info.
-
-Metrics of note:
-
-- `redis_version`
-- `process_id`
-- `config_file`
-- `uptime_in_seconds`
-- `uptime_in_days`
-
-
-Clients: available data on clients connected or failed connections.
-
-Metrics of note:
-
-- `connected_clients`
-- `blocked_clients`
-
-
-Memory: memory usage and stats
-
-Metrics of note:
-
-- `used_memory`
-- `mem_fragmentation_ratio`
-
-
-Persistence: RDB or AOF metrics
-
-Metrics of note:
-
-- `rdb_last_save_time`
-- `rdb_changes_since_last_save`
-- `aof_rewrite_in_progress`
-
-
-Stats: some general statistics
-
-Metrics of note:
-
-- `keyspace_hits`
-- `keyspace_misses`
-- `expired_keys`
-- `evicted_keys`
-- `instantaneous_ops_per_sec`
-
-
-Replication: replication data including primary/replica identifiers
-and offsets
-
-Metrics of note:
-
-- `master_link_down_since`
-- `connected_slaves`
-- `master_last_io_seconds_ago`
-
-
-CPU: compute consumption stats
-
-Metrics of note:
-
-- `used_cpu_sys`
-- `used_cpu_user`
-
-
-Modules: data from any loaded modules
-
-Metrics of note (per module):
-
-- `ver`
-- `options`
-
-
-Cluster: whether cluster is enabled
-
-Metric of note:
-
-- `cluster_enabled`
-
-
-Keyspace: keys and expiration data
-
-Metrics of note (per db):
-
-- `keys`
-- `expires`
-- `avg_ttl`
-
-The output can be read from the results or piped into a file.
-
-```bash
-127.0.0.1:6379> redis-cli INFO STATS > redis-info-stats
-```
-
-This could be done at intervals and consumed by a local or third party monitoring service.
-
-Some of the data returned by `INFO` are going to be static. For example the Redis version which won't change until an update is made. Other data is dynamic, for `keyspace_hits ÷ keyspace_misses`. The latter could be taken to compute a hit ratio and observed as a long term metric. The replication section field `master_link_down_since` could be a metric to connect an alert.
-
-Some examples of possible alerts that could be setup for a given metric:
-
-| Metric | Example Alert |
-| ------------------------ | ------------------------------------------------------- |
-| `uptime_in_seconds` | < 300 seconds: to ensure the server is staying up |
-| `connected_clients` | < minimum number of expected application connections |
-| `master_link_down_since` | > 30 seconds: replication should be operational |
-| `rdb_last_save_time` | > maximum acceptable interval without taking a snapshot |
-
-
-:::note
-
-This is not an exhaustive list, but just to give you an idea of how the metrics in INFO could be used.
-
-:::
-
-## Latency and stats data via redis-cli options
-
-The redis-cli client has some built-in options that allow you to pull some real-time latency and stats data.
-
-
-:::note
-
-These are not available as commands from Redis but as options in redis-cli.
-
-:::
-
-Latency options:
-
-Continuously sample latency:
-
-```bash
-$ redis-cli --latency
-min: 1, max: 17, avg: 4.03 (927 samples)
-```
-
-The `raw` or `csv` output flag can be added:
-
-```bash
-$ redis-cli --latency --csv
-1,4,1.94,78
-```
-
-In order to sample for longer than one second you can use `latency-history` which has a default interval of 15 seconds but can be specified using the `-i` param.
-
-```bash
-$ redis-cli --latency-history -i 60
-min: 1, max: 30, avg: 4.84 (328 samples)
-```
-
-This can also be combined with the `csv` or `raw` output format flag.
-
-```bash
-$ redis-cli --latency-history -i 60 --csv
-13,13,13.00,1
-5,13,9.00,2
-3,13,7.00,3
-3,13,6.00,4
-3,13,5.60,5
-2,13,5.00,6
-2,13,5.43,7
-2,13,5.62,8
-2,13,5.22,9
-2,13,5.00,10
-1,13,4.64,11
-…
-```
-
-Both of these could be piped to a file as well.
-
-The `latency-dist` option shows latency as a spectrum. The default interval is one second but can be changed using the `-i` param.
-
-
-Stats option:
-
-Get rolling stats from the server using the `stat` flag.
-
-```bash
-$ redis-cli --stat
-------- data ------ --------------------- load -------------------- - child -
-keys mem clients blocked requests connections
-4 9.98M 51 0 8168035 (+0) 4132
-4 9.98M 51 0 8181542 (+13507) 4132
-4 9.98M 51 0 8196100 (+14558) 4132
-4 9.98M 51 0 8209794 (+13694) 4132
-4 9.98M 51 0 8223420 (+13626) 4132
-4 9.98M 51 0 8236624 (+13204) 4132
-4 9.98M 51 0 8251376 (+14752) 4132
-4 9.98M 51 0 8263417 (+12041) 4182
-4 9.98M 51 0 8276781 (+13364) 4182
-4 9.90M 51 0 8289693 (+12912) 4182
-```
-
-## Memory stats
-
-Redis includes a `MEMORY` command that includes a subcommand to get stats.
-
-```bash
-127.0.0.1:6379> memory stats
- 1) "peak.allocated"
- 2) (integer) 11912984
- 3) "total.allocated"
- 4) (integer) 8379168
- 5) "startup.allocated"
- 6) (integer) 5292168
- 7) "replication.backlog"
- 8) (integer) 0
- 9) "clients.slaves"
-10) (integer) 0
-11) "clients.normal"
-12) (integer) 16986
-13) "aof.buffer"
-14) (integer) 0
-```
-
-These values are available in the `INFO MEMORY` command as well, but here they are returned in a typical Redis `RESP` Array reply.
-
-There is also a `LATENCY DOCTOR` subcommand with an analysis report of the current memory metrics.
-
-## Latency Monitoring
-
-As we know Redis is fast and as a result is often used in very extreme scenarios where low latency is a must. Redis has a feature called Latency Monitoring which allows you to dig into possible latency issues. Latency monitoring is composed of the following conceptual parts:
-
-- Latency hooks that sample different latency sensitive code paths.
-- Time series recording of latency spikes split by different events.
-- A reporting engine to fetch raw data from the time series.
-- Analysis engine to provide human readable reports and hints according to the measurements.
-
-By default this feature is disabled because most of the time it is not needed. In order to enable it you can update the threshold time in milliseconds that you want to monitor in your Redis configuration. Events that take longer than the threshold will be logged as latency spikes. The threshold configuration should be set accordingly if the requirement is to identify all events blocking the server for a time of 10 milliseconds or more.
-
-```bash
-latency-monitor-threshold 10
-```
-
-If the debugging session is intended to be temporary the threshold can be set via redis-cli.
-
-```bash
-127.0.0.1:6379> CONFIG SET latency-monitor-threshold 10
-```
-
-To disable the latency framework the threshold should be set back to 0.
-
-```bash
-127.0.0.1:6379> CONFIG SET latency-monitor-threshold 0
-```
-
-The latency data can be viewed using the `LATENCY` command with it's subcommands:
-
-- `LATENCY LATEST` - latest samples for all events
-- `LATENCY HISTORY` - latest time series for a given event
-- `LATENCY RESET` - resets the time series data
-- `LATENCY GRAPH` - renders an ASCII-art graph
-- `LATENCY DOCTOR` - analysis report
-
-In order to make use of these commands you need to make yourself familiar with the different events that the latency monitoring framework is tracking. (taken from [https://redis.io/topics/latency-monitor](https://redis.io/topics/latency-monitor)" )
-
-| Event | Description |
-| ------------------------- | ------------------------------------------------------------------------------------------- |
-| `command` | regular commands |
-| `fast-command` | `O(1)` and `O(log N)` commands |
-| `fork` | the `fork(2)` system call |
-| `comrdb-unlink-temp-file` | the `unlink(2)` system call |
-| `aof-write` | writing to the AOF - a catchall event `fsync(2)` system calls |
-| `aof-fsync-always` | the `fsync(2)` system call when invoked by the appendfsync allways policy |
-| `aof-write-pending-fsync` | the `fsync(2)` system call when there are pending writes |
-| `aof-write-active-child` | the `fsync(2)` system call when performed by a child process |
-| `aof-write-alone` | the `fsync(2)` system call when performed by the main process |
-| `aof-fstat` | the `fstat(2)` system call |
-| `aof-rename` | the `rename(2)` system call for renaming the temporary file after completing `BGREWRITEAOF` |
-| `aof-rewrite-diff-write` | writing the differences accumulated while performing `BGREWRITEAOF` |
-| `active-defrag-cycle` | the active defragmentation cycle |
-| `expire-cycle` | the expiration cycle |
-| `eviction-cycle` | the eviction cycle |
-| `eviction-del` | deletes during the eviction cycle |
-
-For example, you can use the `LATENCY LATEST` subcommand and you may see some data like this:
-
-```bash
-127.0.0.1:6379> latency latest
-1) 1) "command"
- 2) (integer) 1616372606
- 3) (integer) 600
- 4) (integer) 600
-2) 1) "fast-command"
- 2) (integer) 1616372434
- 3) (integer) 12
- 4) (integer) 12
-```
-
-The results of this command provide the timestamp, latency and max latency for this event. Utilizing the events table above I can see we had latency spikes for a regular command with the latest and max latency of 600 MS while a O(1) or O(log N) command had a latency spike of 12 MS.
-
-Some of the latency commands require a specific event be passed.
-
-```bash
-127.0.0.1:6379> latency graph command
-command - high 600 ms, low 100 ms (all time high 600 ms)
---------------------------------------------------------------------------------
- _##
- o|||
- o||||
-_#|||||
-
-3222184
-05308ss
-sssss
-```
-
-While the cost of enabling latency monitoring is near zero and memory requirements are very small it will raise your baseline memory usage so if you are getting the required performance out of Redis there is no need to leave this enabled.
-
-## Monitoring Tools
-
-There are many open source monitoring tools and services to visualize your Redis metrics - some of which also provide alerting capabilities.
-
-One example of this is the [Redis Data Source for Grafana](https://grafana.com/grafana/plugins/redis-datasource/). It is a [Grafana](https://grafana.com/) plug-in that allows users to connect to the Redis database and build dashboards to easily observe Redis data. It provides an out-of-the-box predefined dashboard but also lets you build customized dashboards tuned to your specific needs.
diff --git a/docs/support/redis-at-scale/observability/exercise-1/index-exercise-1.mdx b/docs/support/redis-at-scale/observability/exercise-1/index-exercise-1.mdx
deleted file mode 100644
index 4de1496..0000000
--- a/docs/support/redis-at-scale/observability/exercise-1/index-exercise-1.mdx
+++ /dev/null
@@ -1,124 +0,0 @@
----
-id: index-exercise-1
-title: 5.2 Getting Redis Statistics
-sidebar_label: 5.2 Getting Redis Statistics
-slug: /operate/redis-at-scale/observability/exercise-1
-custom_edit_url: null
----
-
-Clone this repo if you have not already: [https://github.com/redislabs-training/ru301](https://github.com/redislabs-training/ru301)
-
-Change into the `observability-stats` directory.
-
-Requirements
-
-- docker
-- docker-compose
-- internet connection
-
-Starting Environment
-
-```bash
-$ docker-compose up -d
-```
-
-Connect to the Environment
-
-In a terminal run this command to get a shell prompt inside the running Docker container:
-
-```bash
-$ docker-compose exec redis_stats bash
-```
-
-Generate load
-
-A simple way to to generate some load is to open another terminal and run:
-
-```bash
-$ docker-compose exec redis_stats redis-benchmark
-```
-
-Info
-
-Since most of the stats data comes from the `INFO` command you should first run this to view that there.
-
-```bash
-$ redis-cli INFO
-```
-
-Try piping this output to a file.
-
-Memory usage
-
-Since we generally recommend setting the `maxmemory` size, it is possible to calculate the percentage of memory in use and alert based on results of the `maxmemory` configuration value and the `used_memory` stat.
-
-First set the `maxmemory`.
-
-```bash
-$ redis-cli config set maxmemory 100000
-```
-
-Then you can pull the two data points to see how that could be used to calculate memory usage.
-
-```bash
-$ redis-cli INFO | grep used_memory:
-$ redis-cli CONFIG GET maxmemory
-```
-
-Client data
-
-You can pull the `clients` section of the `INFO` command:
-
-```bash
-$ redis-cli info clients
-```
-
-or maybe a particular metric you would want to track:
-
-```bash
-$ redis-cli info clients | grep connected_clients
-```
-
-Stats section
-
-Use `redis-cli` to list the full 'stats' section.
-
-Hit ratio
-
-A cache hit/miss ratio could be generated using two data points in the stats section.
-
-```bash
-$ redis-cli INFO stats | grep keyspace
-```
-
-Evicted keys
-
-Eviction occurs when Redis has reached its maximum memory and `maxmemory-policy` in `redis.conf` is set to something other than `volatile-lru`.
-
-```bash
-$ redis-cli INFO stats | grep evicted_keys
-```
-
-Expired keys
-
-It is a good idea to keep an eye on the expirations to make sure Redis is performing as expected.
-
-```bash
-$ redis-cli INFO stats | grep expired_keys
-```
-
-Keyspace
-
-The following data could be used for graphing the size of the keyspace as a quick drop or spike in the number of keys is a good indicator of issues.
-
-```bash
-$ redis-cli INFO keyspace
-```
-
-Workload (connections received, commands processed)
-
-The following stats are a good indicator of workload on the Redis server.
-
-```bash
-$ redis-cli INFO stats | egrep "^total_"
-```
diff --git a/docs/support/redis-at-scale/observability/identifying-issues/index-identifying-issues.mdx b/docs/support/redis-at-scale/observability/identifying-issues/index-identifying-issues.mdx
deleted file mode 100644
index 9288098..0000000
--- a/docs/support/redis-at-scale/observability/identifying-issues/index-identifying-issues.mdx
+++ /dev/null
@@ -1,224 +0,0 @@
----
-id: index-identifying-issues
-title: 5.3 Identifying Issues
-sidebar_label: 5.3 Identifying Issues
-slug: /operate/redis-at-scale/observability/identifying-issues
-custom_edit_url: null
----
-
-Besides the metrics from the data points from info, memory and the latency framework in the sections above, you may need to pull data from other sources when troubleshooting.
-
-## Availability
-
-The Redis server will respond to the `PING` command when running properly:
-
-```bash
-$ redis-cli -h redis.example.com -p 6379 PING
-PONG
-```
-
-## Slow Log
-
-Redis Slow Log is a system to log queries that exceed a specific execution time which does not include I/O operations like client communication. It is enabled by default with two configuration parameters.
-
-```bash
-slowlog-log-slower-than 1000000
-```
-
-This indicates if there is an execution time longer than the time in microseconds, in this case one second, it will be logged. The slow log can be disabled using a value of -1. It can also be set to log every command with a value of 0.
-
-```bash
-slowlog-max-len 128
-```
-
-This sets the length of the slow log. When a new command is logged the oldest one is removed from the queue.
-
-These values can also be changed at runtime using the `CONFIG SET` command.
-
-You can view the current length of the slow log using the `LEN` subcommand:
-
-```bash
-redis.cloud:6379> slowlog len
-(integer) 11
-```
-
-Entries can be pulled off of the slow log using the `GET` subcommand.
-
-```bash
-redis.cloud:6379> slowlog get 2
-1) 1) (integer) 10
- 2) (integer) 1616372606
- 3) (integer) 600406
- 4) 1) "debug"
- 2) "sleep"
- 3) ".6"
- 5) "172.17.0.1:60546"
- 6) ""
-2) 1) (integer) 9
- 2) (integer) 1616372602
- 3) (integer) 600565
- 4) 1) "debug"
- 2) "sleep"
- 3) ".6"
- 5) "172.17.0.1:60546"
- 6) ""
-```
-
-The slow log can be reset using the `RESET` subcommand.
-
-```bash
-redis.cloud:6379> slowlog reset
-OK
-redis.cloud:6379> slowlog len
-(integer) 0
-```
-
-## Scanning keys
-
-There are a few options that can be passed to redis-cli that will trigger a keyspace analysis. They use the `SCAN` command so they should be safe to run without impacting operations. You can see in the output of all of them there is a throttling option if needed.
-
----
-
-**Big Keys:** This option will scan the dataset for big keys and provide information about them.
-
-```bash
-$ redis-cli --bigkeys
-
-# Scanning the entire keyspace to find biggest keys as well as
-# average sizes per key type. You can use -i 0.1 to sleep 0.1 sec
-# per 100 SCAN commands (not usually needed).
-
-[00.00%] Biggest string found so far '"counter:__rand_int__"' with 6 bytes
-[00.00%] Biggest hash found so far '"myhash"' with 1 fields
-[00.00%] Biggest list found so far '"mylist"' with 200000 items
-
--------- summary -------
-
-Sampled 4 keys in the keyspace!
-Total key length in bytes is 48 (avg len 12.00)
-
-Biggest list found '"mylist"' has 200000 items
-Biggest hash found '"myhash"' has 1 fields
-Biggest string found '"counter:__rand_int__"' has 6 bytes
-
-1 lists with 200000 items (25.00% of keys, avg size 200000.00)
-1 hashs with 1 fields (25.00% of keys, avg size 1.00)
-2 strings with 9 bytes (50.00% of keys, avg size 4.50)
-0 streams with 0 entries (00.00% of keys, avg size 0.00)
-0 sets with 0 members (00.00% of keys, avg size 0.00)
-0 zsets with 0 members (00.00% of keys, avg size 0.00)
-```
-
----
-
-**Mem Keys:** Similarly to big keys, mem keys will look for the biggest keys but also report on the average sizes.
-
-```bash
-$ redis-cli --memkeys
-
-# Scanning the entire keyspace to find biggest keys as well as
-# average sizes per key type. You can use -i 0.1 to sleep 0.1 sec
-# per 100 SCAN commands (not usually needed).
-
-[00.00%] Biggest string found so far '"counter:__rand_int__"' with 62 bytes
-[00.00%] Biggest string found so far '"key:__rand_int__"' with 63 bytes
-[00.00%] Biggest hash found so far '"myhash"' with 86 bytes
-[00.00%] Biggest list found so far '"mylist"' with 860473 bytes
-
--------- summary -------
-
-Sampled 4 keys in the keyspace!
-Total key length in bytes is 48 (avg len 12.00)
-
-Biggest list found '"mylist"' has 860473 bytes
-Biggest hash found '"myhash"' has 86 bytes
-Biggest string found '"key:__rand_int__"' has 63 bytes
-
-1 lists with 860473 bytes (25.00% of keys, avg size 860473.00)
-1 hashs with 86 bytes (25.00% of keys, avg size 86.00)
-2 strings with 125 bytes (50.00% of keys, avg size 62.50)
-0 streams with 0 bytes (00.00% of keys, avg size 0.00)
-0 sets with 0 bytes (00.00% of keys, avg size 0.00)
-0 zsets with 0 bytes (00.00% of keys, avg size 0.00)
-```
-
----
-
-**Hot Keys:** The hot keys scan is only available when the `maxmemory-policy` is set to `volatile-lfu` or `allkeys-lfu`. If you need to identity hot keys you can add this argument to redis-cli.
-
-```bash
-$ redis-cli --hotkeys
-
-# Scanning the entire keyspace to find hot keys as well as
-# average sizes per key type. You can use -i 0.1 to sleep 0.1 sec
-# per 100 SCAN commands (not usually needed).
-
-[00.00%] Hot key '"key:__rand_int__"' found so far with counter 37
-
--------- summary -------
-
-Sampled 5 keys in the keyspace!
-hot key found with counter: 37 keyname: "key:__rand_int__"
-```
-
----
-
-**Monitor:** The `MONITOR` command allows you to see a stream of every command running against your Redis instance.
-
-```bash
-127.0.0.1:6379 > monitor
-OK
-1616541192.039933 [0 127.0.0.1:57070] "PING"
-1616541276.052331 [0 127.0.0.1:57098] "set" "user:2398423hu" "KutMo"
-```
-
-:::caution
-
-Since `MONITOR` streams back all commands, its use comes at a cost. It has been known to reduce performance by up to 50% so use with caution!
-
-:::
-
-## Setting up and using the Redis Log File
-
-The Redis log file is the other important log you need to be aware of. It contains useful information for troubleshooting configuration and deployment errors. If you don't configure Redis logging, troubleshooting will be significantly harder.
-
-Redis has four logging levels, which you can configure directly in `redis.conf` file.
-
-Log Levels:
-
-- `WARNING`
-- `NOTICE`
-- `VERBOSE`
-- `DEBUG`
-
-Redis also supports sending the log files to a remote logging server through the use of syslog.
-
-Remote logging is important to many security professionals. These remote logging servers are frequently used to monitor security events and manage incidents. These centralized log servers perform three common functions: ensure the integrity of your log files, ensure that logs are retained for a specific period of time, and to correlate logs against other system logs to discover potential attacks on your infrastructure.
-
-Let's set up logging on our Redis deployment. First we'll open our `redis.conf` file:
-
-```bash
-$ sudo vi /etc/redis/redis.conf
-```
-
-The `redis.conf` file has an entire section dedicated to logging.
-
-First, find the logfile directive in the `redis.conf` file. This will allow you to define the logging directory. For this example lets use `/var/log/redis/redis.log`.
-
-If you'd like to use a remote logging server, then you'll need to uncomment the lines `syslog-enabled`, `syslog-ident` and `syslog-facility`, and ensure that `syslog-enabled` is set to yes.
-
-Next, we'll restart the Redis server.
-
-You should see the log events indicating that Redis is starting.
-
-```bash
-$ sudo tail -f /var/log/redis/redis.log
-```
-
-And next let's check that we are properly writing to syslog. You should see these same logs.
-
-```bash
-$ less /var/log/syslog | grep redis
-```
-
-Finally, you’ll need to send your logs to your remote logging server to ensure your logs will be backed up to this server. To do this, you’ll also have to modify the rsyslog configuration. This configuration varies depending on your remote logging server provider.
diff --git a/docs/support/redis-at-scale/observability/index-observability.mdx b/docs/support/redis-at-scale/observability/index-observability.mdx
deleted file mode 100644
index c617f64..0000000
--- a/docs/support/redis-at-scale/observability/index-observability.mdx
+++ /dev/null
@@ -1,10 +0,0 @@
----
-id: index-observability
-title: Ensuring Observability in Redis
-sidebar_label: Observability
-slug: /operate/redis-at-scale/observability
-authors: [justin]
-custom_edit_url: null
----
-
-Hello World! Observability
diff --git a/docs/support/redis-at-scale/observability/introduction/index-introduction.mdx b/docs/support/redis-at-scale/observability/introduction/index-introduction.mdx
deleted file mode 100644
index aa7b255..0000000
--- a/docs/support/redis-at-scale/observability/introduction/index-introduction.mdx
+++ /dev/null
@@ -1,41 +0,0 @@
----
-id: index-introduction
-title: 5.0 Introduction to Observability
-sidebar_label: 5.0 Introduction to Observability
-slug: /operate/redis-at-scale/observability/introduction
-custom_edit_url: null
----
-
-
-
-
-
-
-
- The last thing you want to do after successfully deploying and scaling Redis
- is to be stuck working on the weekend because performance is down or the
- service is unavailable!
-
-
-If you're running a managed service like Redis Cloud, you won't have to worry about these questions as much. But even then, it's still worthwhile to know about certain key Redis metrics.
-
-Some of the question you always want to be able to answer include:
-
-- Is Redis up and running right now?
-- Where is my Redis capacity at?
-- Is Redis accessible at this moment?
-- Is Redis performing the way we expect?
-- When failures occur… what exactly happened to Redis?
-
-Then of course you must ask...
-
-- How can I find this out ahead of time?
-
-Let's dig into these questions and more as we look into observability with Redis.
diff --git a/docs/support/redis-at-scale/persistence-and-durability/exercise/index-exercise.mdx b/docs/support/redis-at-scale/persistence-and-durability/exercise/index-exercise.mdx
deleted file mode 100644
index 299ed97..0000000
--- a/docs/support/redis-at-scale/persistence-and-durability/exercise/index-exercise.mdx
+++ /dev/null
@@ -1,71 +0,0 @@
----
-id: index-exercise
-title: '2.2 Exercise: Saving a Snapshot'
-sidebar_label: '2.2 Exercise: Saving a Snapshot'
-slug: /operate/redis-at-scale/persistence-and-durability/exercise
-custom_edit_url: null
----
-
-As we learned in the previous unit, Redis will save a snapshot of your database every hour if at least one key has changed, every five minutes if at least 100 keys have changed, or every 60 seconds if at least 10000 keys have changed.
-
-Let’s update this to a simplified hypothetical scenario where we want to save a snapshot if three keys have been modified in 20 seconds.
-
-## Step 1
-
-Create a directory named 2.2 and in it prepare a `redis.conf` file.
-
-```bash
-$ mkdir 2.2
-$ cd 2.2
-$ vim redis.conf
-```
-
-The `redis.conf` file should specify a filename that will be used for the rdb file and a directive that will trigger the creation of a snapshot if 3 keys have been modified in 20 seconds, as described above.
-
-```bash
-dbfilename my_backup_file.rdb
-save 20 3
-```
-
-## Step 2
-
-In the 2.2 directory, start a Redis server - passing it the `redis.conf` configuration file you just created.
-
-```bash
-$ redis-server ./redis.conf
-```
-
-In a separate terminal tab use the `redis-cli` to create three random keys, one after the other. For example:
-
-```bash
-127.0.0.1:6379> SET a 1
-127.0.0.1:6379> SET b 2
-127.0.0.1:6379> SET c 3
-```
-
-Run the `ls` command in the first terminal to list all the files in the 2.2 directory. What changed?
-
-## Step 3
-
-Now we’re ready to take our persistence a level higher and set up an `AOF` file. Modify your `redis.conf` file so that the server will log every new write command and force writing it to disk.
-
-Be careful! We have a running server and we want this configuration to be applied without restarting it.
-
-```bash
-127.0.0.1:6379> CONFIG SET appendonly yes
-127.0.0.1:6379> CONFIG SET appendfsync always
-```
-
-In order for these settings to be persisted to the `redis.conf` file we need to save them:
-
-```bash
-127.0.0.1:6379> CONFIG REWRITE
-```
-
-## Step 4
-
-Create a few random keys through `redis-cli`. Check the contents of the directory 2.2 again. What changed?
-
-## Step 5
-
-As a final step, restart the Redis server process (you can press Ctrl+C in the terminal to stop the process and re-run it again). If you run the `SCAN 0` command you will see that all the keys you created are still in the database, even though we restarted the process.
diff --git a/docs/support/redis-at-scale/persistence-and-durability/index-persistence-and-durability.mdx b/docs/support/redis-at-scale/persistence-and-durability/index-persistence-and-durability.mdx
deleted file mode 100644
index e804c88..0000000
--- a/docs/support/redis-at-scale/persistence-and-durability/index-persistence-and-durability.mdx
+++ /dev/null
@@ -1,10 +0,0 @@
----
-id: index-persistence-and-durability
-title: Ensuring Persistence & Durability in Redis
-sidebar_label: Persistence & Durability
-slug: /operate/redis-at-scale/persistence-and-durability
-authors: [justin]
-custom_edit_url: null
----
-
-Hello World! Persistence & Durability
diff --git a/docs/support/redis-at-scale/persistence-and-durability/introduction/index-introduction.mdx b/docs/support/redis-at-scale/persistence-and-durability/introduction/index-introduction.mdx
deleted file mode 100644
index 372931b..0000000
--- a/docs/support/redis-at-scale/persistence-and-durability/introduction/index-introduction.mdx
+++ /dev/null
@@ -1,30 +0,0 @@
----
-id: index-introduction
-title: '2.0 Introduction to Persistence and Durability'
-sidebar_label: 2.0 Introduction to Persistence and Durability'
-slug: /operate/redis-at-scale/persistence-and-durability/introduction
-custom_edit_url: null
----
-
-
-
-
-
-
-
- Hello! Congrats on completing Section 1. Section 2 is a bit shorter but
- contains some important information on persistence and durability.
-
-
-As I am sure you know, Redis serves all data directly from memory. But Redis is also capable of persisting data to disk. Persistence preserves data in the event of a server restart.
-
-In the following video and exercise, we'll look at the options for persisting data to disk. We'll show you how to enable persistence, and you'll then do a hands-on exercise setting up snapshots of your Redis instance.
-
-Good luck, and we'll see you in the next sections.
diff --git a/docs/support/redis-at-scale/persistence-and-durability/persistence-options-in-redis/index-persistence-options-in-redis.mdx b/docs/support/redis-at-scale/persistence-and-durability/persistence-options-in-redis/index-persistence-options-in-redis.mdx
deleted file mode 100644
index 9a54d66..0000000
--- a/docs/support/redis-at-scale/persistence-and-durability/persistence-options-in-redis/index-persistence-options-in-redis.mdx
+++ /dev/null
@@ -1,55 +0,0 @@
----
-id: index-persistence-options-in-redis
-title: '2.1 Persistence options in Redis'
-sidebar_label: 2.1 Persistence options in Redis'
-slug: /operate/redis-at-scale/persistence-and-durability/persistence-options-in-redis
-custom_edit_url: null
----
-
-
-
-
-
-
-
- If a Redis server that only stores data in RAM is restarted, all data is lost.
- To prevent such data loss, there needs to be some mechanism for persisting the
- data to disk; Redis provides two of them: snapshotting and an
- append-only file, or AOF. You can configure your
- Redis instances to use either of the two, or a combination of both.
-
-
-When a snapshot is created, the entire point-in-time view of the dataset is written to persistent storage in a compact .rdb file. You can set up recurring backups, for example every 1, 12, or 24 hours and use these backups to easily restore different versions of the data set in case of disasters. You can also use these snapshots to create a clone of the server, or simply leave them in place for a future restart.
-
-Creating a .rdb file requires a lot of disk I/O. If performed in the main Redis process, this would reduce the server’s performance. That’s why this work is done by a forked child process. But even forking can be time-consuming if the dataset is large. This may result in decreased performance or in Redis failing to serve clients for a few milliseconds or even up to a second for very large datasets. Understanding this should help you decide whether this solution makes sense for your requirements.
-
-You can configure the name and location of the .rdb file with the dbfilename and dir configuration directives, either through the redis.conf file, or through the redis-cli as explained in [Section 1 Unit 2](https://developer.redis.com//operate/redis-at-scale/talking-to-redis/configuring-a-redis-server). And of course you can configure how often you want to create a snapshot. Here’s an excerpt from the redis.conf file showing the default values.
-
-As an example, this configuration will make Redis automatically dump the dataset to disk every 60 seconds if at least 1000 keys changed in that period. While snapshotting is a great strategy for the use cases explained above, it leaves a huge possibility for data loss. You can configure snapshots to run every few minutes, or after X writes against the database, but if the server crashes you lose all the writes since the last snapshot was taken. In many use cases, that kind of data loss can be acceptable, but in many others it is absolutely not. For all of those other use cases Redis offers the AOF persistence option.
-
-AOF, or append-only file works by logging every incoming write command to disk as it happens. These commands can then be replayed at server startup, to reconstruct the original dataset. Commands are logged using the same format as the Redis protocol itself, in an append-only fashion. The AOF approach provides greater durability than snapshotting, and allows you to configure how often file syncs happen.
-
-Depending on your durability requirements (or how much data you can afford to lose), you can choose which fsync policy is the best for your use case:
-
-- fsync every write: The safest policy: The write is
- acknowledged to the client only after it has been written to the AOF file and
- flushed to disk. Since in this approach we are writing to disk synchronously,
- we can expect a much higher latency than usual.
-- fsync every second: The default policy. Fsync is performed
- asynchronously, in a background thread, so write performance is still high.
- Choose this option if you need high performance and can afford to lose up to
- one second worth of writes.
-- no fsync: In this case Redis will log the command to the file
- descriptor, but will not force the OS to flush the data to disk. If the OS
- crashes we can lose a few seconds of data (Normally Linux will flush data
- every 30 seconds with this configuration, but it's up to the kernel’s exact
- tuning.).
-
-The relevant configuration directives for AOF are shown on the screen. AOF contains a log of all the operations that modified the database in a format that’s easy to understand and parse. When the file gets too big, Redis can automatically rewrite it in the background, compacting it in a way that only the latest state of the data is preserved.
diff --git a/docs/support/redis-at-scale/scalability/clustering-in-redis/index-scalability.mdx b/docs/support/redis-at-scale/scalability/clustering-in-redis/index-scalability.mdx
deleted file mode 100644
index 350b4e9..0000000
--- a/docs/support/redis-at-scale/scalability/clustering-in-redis/index-scalability.mdx
+++ /dev/null
@@ -1,74 +0,0 @@
----
-id: index-clustering-in-redis
-title: 4.0 Clustering In Redis
-sidebar_label: 4.0 Clustering In Redis
-slug: /operate/redis-at-scale/scalability/lustering-in-redis
-authors: [justin]
-custom_edit_url: null
----
-
-
-
-
-
-
-
-
-
-
-
- Before we jump into the details, let's first address the elephant in the room:
- DBaaS offerings, or "database-as-a-service" in the cloud. No doubt, it's
- useful to know how Redis scales and how you might deploy it. But deploying and
- maintaining a Redis cluster is a fair amount of work. So if you don't want to
- deploy and manage Redis yourself, then consider signing up for Redis Cloud,
- our managed service, and let us do the scaling for you. Of course, that route
- is not for everyone. And as I said, there's a lot to learn here, so let's dive
- in.
-
-
-We'll start with scalability. Here's one definition:
-
-> “Scalability is the property of a system to handle a growing amount of work by adding resources to the system.”
-> [Wikipedia](https://en.wikipedia.org/wiki/Scalability)
-
-The two most common scaling strategies are vertical scaling and horizontal scaling. Vertical scaling, or also called “Scaling Up”, means adding more resources like CPU or memory to your server. Horizontal scaling, or “Scaling out”, implies adding more servers to your pool of resources. It's the difference between just getting a bigger server and deploying a whole fleet of servers.
-
-Let's take an example. Suppose you have a server with 128 GB of RAM, but you know that your database will need to store 300 GB of data. In this case, you’ll have two choices: you can either add more RAM to your server so it can fit the 300GB dataset, or you can add two more servers and split the 300GB of data between the three of them. Hitting your server’s RAM limit is one reason you might want to scale up, or out, but reaching the performance limit in terms of throughput, or operations per second, is also an indicator that scaling is necessary.
-
-Since Redis is mostly single-threaded, Redis cannot make use of the multiple cores of your server’s CPU for command processing. But if we split the data between two Redis servers, our system can process requests in parallel, increasing the throughput by almost 200%. In fact, performance will scale close to linearly by adding more Redis servers to the system. This database architectural pattern of splitting data between multiple servers for the purpose of scaling is called sharding. The resulting servers that hold chunks of the data are called shards.
-
-This performance increase sounds amazing, but it doesn’t come without some cost: if we divide and distribute our data across two shards, which are just two Redis server instances, how will we know where to look for each key? We need to have a way to consistently map a key to a specific shard. There are multiple ways to do this and different databases adopt different strategies. The one Redis chose is called “Algorithmic sharding” and this is how it works:
-
-In order to find the shard on which a key lives we compute a numeric hash value out of the key name and modulo divide it by the total number of shards. Because we are using a deterministic hash function the key “foo” will always end up on the same shard, as long as the number of shards stays the same.
-
-But what happens if we want to increase our shard count even further, a process commonly called resharding? Let’s say we add one new shard so that our total number of shards is three. When a client tries to read the key “foo” now, they will run the hash function and modulo divide by the number of shards, as before, but this time the number of shards is different and we’re modulo dividing with three instead of two. Understandably, the result may be different, pointing us to the wrong shard!
-
-Resharding is a common issue with the algorithmic sharding strategy and can be solved by rehashing all the keys in the keyspace and moving them to the shard appropriate to the new shard count. This is not a trivial task, though, and it can require a lot of time and resources, during which the database will not be able to reach its full performance or might even become unavailable.
-
-Redis chose a very simple approach to solving this problem: it introduced a new, logical unit that sits between a key and a shard, called a hash slot.
-
-One shard can contain many hash slots, and a hash slot contains many keys. The total number of hash slots in a database is always 16384 (16K). This time, the modulo division is not done with the number of shards anymore, but instead with the number of hash slots, that stays the same even when resharding and the end result will give us the position of the hash slot where the key we’re looking for lives. And when we do need to reshard, we simply move hash slots from one shard to another, distributing the data as required across the different redis instances.
-
-Now that we know what sharding is and how it works in Redis, we can finally introduce Redis Cluster. Redis Cluster provides a way to run a Redis installation where data is automatically split across multiple Redis servers, or shards. Redis Cluster also provides high availability. So, if you're deploying Redis Cluster, you don't need (or use) Redis Sentinel.
-
-Redis Cluster can detect when a primary shard fails and promote a replica to a primary without any manual intervention from the outside. How does it do it? How does it know that a primary shard has failed, and how does it promote its replica to be the new primary shard? We need to have replication enabled. Say we have one replica for every primary shard. If all our data is divided between three Redis servers, we would need a six-member cluster, with three primary shards and three replicas.
-
-All 6 shards are connected to each other over TCP and constantly PING each other and exchange messages using a binary protocol. These messages contain information about which shards have responded with a PONG, so are considered alive, and which haven’t.
-
-When enough shards report that a certain primary shard is not responding to them, they can agree to trigger a failover and promote the shard’s replica to become the new primary. How many shards need to agree that a shard is offline before a failover is triggered? Well, that’s configurable and you can set it up when you create a cluster, but there are some very important guidelines that you need to follow.
-
-If you have an even number of shards in the cluster, say six, and there’s a network partition that divides the cluster in two, you'll then have two groups of three shards. The group on the left side will not be able to talk to the shards from the group on the right side, so the cluster will think that they are offline and it will trigger a failover of any primary shards, resulting in a left side with all primary shards. On the right side, the three shards will see the shards on the left as offline, and will trigger a failover on any primary shard that was on the left side, resulting in a right side of all primary shards. Both sides, thinking they have all the primaries, will continue to receive client requests that modify data, and that is a problem, because maybe client A sets the key “foo” to “bar” on the left side, but a client B sets the same key’s value to “baz” on the right side.
-
-When the network partition is removed and the shards try to rejoin, we will have a conflict, because we have two shards - holding different data claiming to be the primary and we wouldn’t know which data is valid.
-
-This is called a split brain situation, and is a very common issue in the world of distributed systems. A popular solution is to always keep an odd number of shards in your cluster, so that when you get a network split, the left and right group will do a count and see if they are in the bigger or the smaller group (also called majority or minority). If they are in the minority, they will not try to trigger a failover and will not accept any client write requests.
-
-Here's the bottom line: to prevent split-brain situations in Redis Cluster, always keep an odd number of primary shards and two replicas per primary shard.
diff --git a/docs/support/redis-at-scale/scalability/exercise-1/index-exercise-1.mdx b/docs/support/redis-at-scale/scalability/exercise-1/index-exercise-1.mdx
deleted file mode 100644
index 8d1b55d..0000000
--- a/docs/support/redis-at-scale/scalability/exercise-1/index-exercise-1.mdx
+++ /dev/null
@@ -1,227 +0,0 @@
----
-id: index-exercise-1
-title: 4.1 Exercise - Creating a Redis Cluster
-sidebar_label: 4.1 Exercise - Creating a Redis Cluster
-slug: /operate/redis-at-scale/scalability/exercise-1
-custom_edit_url: null
----
-
-## Step 1
-
-To create a cluster, we need to spin up a few empty Redis instances and configure them to run in cluster mode.
-
-Here’s a minimal configuration file for Redis Cluster:
-
-```bash
-# redis.conf file
-port 7000
-cluster-enabled yes
-cluster-config-file nodes.conf
-cluster-node-timeout 5000
-appendonly yes
-```
-
-On the first line we specify the port on which the server should run, then we state that we want the server to run in cluster mode, with the `cluster-enabled yes` directive. `cluster-config-file` defines the name of the file where the configuration for this node is stored, in case of a server restart. Finally, `cluster-node-timeout` is the number of milliseconds a node must be unreachable for it to be considered in failure state.
-
-## Step 2
-
-Let’s create a cluster on your localhost with three primary shards and three replicas (remember, in production always use two replicas to protect against a split-brain situation). We’ll need to bring up six Redis processes and create a `redis.conf` file for each of them, specifying their port and the rest of the configuration directives above.
-
-First, create six directories:
-
-```bash
-mkdir -p {7000..7005}
-```
-
-## Step 3
-
-Then create the minimal configuration `redis.conf` file from above in each one of them, making sure you change the port directive to match the directory name.
-
-To copy the initial `redis.conf` file to each folder, run the following:
-
-```bash
-for i in {7000..7005}; do cp redis.conf $i; done
-```
-
-You should end up with the following directory structure:
-
-```bash
-- 7000
- - redis.conf
-- 7001
- - redis.conf
-- 7002
- - redis.conf
-- 7003
- - redis.conf
-- 7004
- - redis.conf
-- 7005
- - redis.conf
-```
-
-## Step 4
-
-Open six terminal tabs and start the servers by going into each one of the directories and starting a Redis instance:
-
-```bash
-# Terminal tab 1
-cd 7000
-/path/to/redis-server ./redis.conf
-# Terminal tab 2
-cd 7001
-/path/to/redis-server ./redis.conf
-... and so on.
-```
-
-## Step 5
-
-Now that you have six empty Redis servers running, you can join them in a cluster:
-
-```bash
-redis-cli --cluster create 127.0.0.1:7000 127.0.0.1:7001 \
-127.0.0.1:7002 127.0.0.1:7003 127.0.0.1:7004 127.0.0.1:7005 \
---cluster-replicas 1
-```
-
-Here we list the ports and IP addresses of all six servers and use the `create` command to instruct Redis to join them in a cluster, creating one replica for each primary. `redis-cli` will propose a configuration; accept it by typing yes. The cluster will be configured and joined, which means, instances will be bootstrapped into talking with each other.
-
-Finally, you should see a message saying:
-
-```bash
-[OK] All 16384 slots covered
-```
-
-This means that there is at least a master instance serving each of the 16384 slots available.
-
-## Step 6
-
-Let’s add a new shard to the cluster, which is something you might do when you need to scale.
-
-First, as before, we need to start two new empty Redis instances (primary and its replica) in cluster mode. We create new directories `7006` and `7007` and in them we copy the same `redis.conf` file we used before, making sure we change the port directive in them to the appropriate port (`7006` and `7007`).
-
-```bash
-$ mkdir 7006 7007
-$ cp 7000/redis.conf 7006/redis.conf
-$ cp 7000/redis.conf 7007/redis.conf
-```
-
-Update the port numbers in the files `./7006/redis.conf` and `./7007/redis.conf` to `7006` and `7007`, respectively.
-
-## Step 7
-
-Let’s start the Redis instances:
-
-```bash
-# Terminal tab 7
-$ cd 7006
-$ redis-server ./redis.conf
-# Terminal tab 8
-$ cd 7007
-$ redis-server ./redis.conf
-```
-
-## Step 8
-
-In the next step we join the new primary shard to the cluster with the `add-node` command. The first parameter is the address of the new shard, and the second parameter is the address of any of the current shards in the cluster.
-
-```bash
-redis-cli --cluster add-node 127.0.0.1:7006 127.0.0.1:7000
-```
-
-
-:::note
-
-The Redis commands use the term “Nodes” for what we call “Shards” in this training, so a command named “add-node” would mean “add a shard”.
-
-:::
-
-## Step 9
-
-Finally we need to join the new replica shard, with the same `add-node` command, and a few extra arguments indicating the shard is joining as a replica and what will be its primary shard. If we don’t specify a primary shard Redis will assign one itself.
-
-We can find the IDs of our shards by running the cluster nodes command on any of the shards:
-
-```bash
-$ redis-cli -p 7000 cluster nodes
-46a768cfeadb9d2aee91ddd882433a1798f53271 127.0.0.1:7006@17006 master - 0 1616754504000 0 connected
-1f2bc068c7ccc9e408161bd51b695a9a47b890b2 127.0.0.1:7003@17003 slave a138f48fe038b93ea2e186e7a5962fb1fa6e34fa 0 1616754504551 3 connected
-5b4e4be56158cf6103ffa3035024a8d820337973 127.0.0.1:7001@17001 master - 0 1616754505584 2 connected 5461-10922
-a138f48fe038b93ea2e186e7a5962fb1fa6e34fa 127.0.0.1:7002@17002 master - 0 1616754505000 3 connected 10923-16383
-71e078dab649166dcbbcec51520742bc7a5c1992 127.0.0.1:7005@17005 slave 5b4e4be56158cf6103ffa3035024a8d820337973 0 1616754505584 2 connected
-f224ecabedf39d1fffb34fb6c1683f8252f3b7dc 127.0.0.1:7000@17000 myself,master - 0 1616754502000 1 connected 0-5460
-04d71d5eb200353713da475c5c4f0a4253295aa4 127.0.0.1:7004@17004 slave f224ecabedf39d1fffb34fb6c1683f8252f3b7dc 0 1616754505896 1 connected
-```
-
-The port of the primary shard we added in the last step was `7006`, and we can see it on the first line. It’s id is `46a768cfeadb9d2aee91ddd882433a1798f53271`.
-
-The resulting command is:
-
-```bash
-$ redis-cli -p 7000 --cluster add-node 127.0.0.1:7007 127.0.0.1:7000 --cluster-slave --cluster-master-id 46a768cfeadb9d2aee91ddd882433a1798f53271
-```
-
-The flag `cluster-slave` indicates that the shard should join as a replica and `--cluster-master-id 46a768cfeadb9d2aee91ddd882433a1798f53271` specifies which primary shard it should replicate.
-
-## Step 10
-
-Now our cluster has eight shards (four primary and four replica), but if we run the cluster slots command we’ll see that the newly added shards don’t host any hash slots, and thus - data. Let’s assign some hash slots to them:
-
-```bash
-$ redis-cli -p 7000 --cluster reshard 127.0.0.1:7000
-```
-
-We use the command `reshard` and the address of any shard in the cluster as an argument here. In the next step we’ll be able to choose the shards we’ll be moving slots from and to.
-
-The first question you’ll get is about the number of slots you want to move. If we have 16384 slots in total, and four primary shards, let’s get a quarter of all shards, so the data is distributed equally. 16384 ÷ 4 is 4096, so let’s use that number.
-
-The next question is about the receiving shard id; the ID of the primary shard we want to move the data to, which we learned how to get in the previous Step, with the cluster nodes command.
-
-Finally, we need to enter the IDs of the shards we want to copy data from. Alternatively, we can type “all” and the shard will move a number of hash slots from all available primary shards.
-
-```bash
-$ redis-cli -p 7000 --cluster reshard 127.0.0.1:7000
-....
-....
-....
-
-How many slots do you want to move (from 1 to 16384)? 4096
-What is the receiving node ID? 46a768cfeadb9d2aee91ddd882433a1798f53271
-Please enter all the source node IDs.
- Type 'all' to use all the nodes as source nodes for the hash slots.
- Type 'done' once you entered all the source nodes IDs.
-Source node #1: all
-
-Ready to move 4096 slots.
- Source nodes:
- M: f224ecabedf39d1fffb34fb6c1683f8252f3b7dc 127.0.0.1:7000
- slots:[0-5460] (5461 slots) master
- 1 additional replica(s)
- M: 5b4e4be56158cf6103ffa3035024a8d820337973 127.0.0.1:7001
- slots:[5461-10922] (5462 slots) master
- 1 additional replica(s)
- M: a138f48fe038b93ea2e186e7a5962fb1fa6e34fa 127.0.0.1:7002
- slots:[10923-16383] (5461 slots) master
- 1 additional replica(s)
- Destination node:
- M: 46a768cfeadb9d2aee91ddd882433a1798f53271 127.0.0.1:7006
- slots: (0 slots) master
- 1 additional replica(s)
- Resharding plan:
- Moving slot 5461 from 5b4e4be56158cf6103ffa3035024a8d820337973
- Moving slot 5462 from 5b4e4be56158cf6103ffa3035024a8d820337973
-
-Do you want to proceed with the proposed reshard plan (yes/no)?
-Moving slot 5461 from 127.0.0.1:7001 to 127.0.0.1:7006:
-Moving slot 5462 from 127.0.0.1:7001 to 127.0.0.1:7006:
-Moving slot 5463 from 127.0.0.1:7001 to 127.0.0.1:7006:
-....
-....
-....
-```
-
-Once the command finishes we can run the cluster slots command again and we’ll see that our new primary and replica shards have been assigned some hash slots:
-
-```bash
-$ redis-cli -p 7000 cluster slots
-```
diff --git a/docs/support/redis-at-scale/scalability/index-scalability.mdx b/docs/support/redis-at-scale/scalability/index-scalability.mdx
deleted file mode 100644
index 3fa84e8..0000000
--- a/docs/support/redis-at-scale/scalability/index-scalability.mdx
+++ /dev/null
@@ -1,10 +0,0 @@
----
-id: index-scalability
-title: Ensuring Scalability in Redis
-sidebar_label: Scalability
-slug: /operate/redis-at-scale/scalability
-authors: [justin]
-custom_edit_url: null
----
-
-Hello World! Scalability
diff --git a/docs/support/redis-at-scale/scalability/redis-cli-with-redis-cluster/index-redis-cli-with-redis-cluster.mdx b/docs/support/redis-at-scale/scalability/redis-cli-with-redis-cluster/index-redis-cli-with-redis-cluster.mdx
deleted file mode 100644
index ee4133e..0000000
--- a/docs/support/redis-at-scale/scalability/redis-cli-with-redis-cluster/index-redis-cli-with-redis-cluster.mdx
+++ /dev/null
@@ -1,19 +0,0 @@
----
-id: index-redis-cli-with-redis-cluster
-title: 4.2 Using Redis-CLI with a Redis Cluster
-sidebar_label: 4.2 Using Redis-CLI with a Redis Cluster
-slug: /operate/redis-at-scale/scalability/redis-cli-with-redis-cluster
-custom_edit_url: null
----
-
-When you use `redis-cli` to connect to a shard of a Redis Cluster, you are connected to that shard only, and cannot access data from other shards. If you try to access keys from the wrong shard, you will get a `MOVED` error.
-
-There is a trick you can use with `redis-cli` so you don’t have to open connections to all the shards, but instead you let it do the connect and reconnect work for you. It’s the `redis-cli` cluster support mode, triggered by the `-c` switch:
-
-```bash
-$ redis-cli -p 7000 -c
-```
-
-When in cluster mode, if the client gets an `(error) MOVED 15495 127.0.0.1:7002` error response from the shard it’s connected to, it will simply reconnect to the address returned in the error response, in this case 127.0.0.1:7002.
-
-Now it’s your turn: use `redis-cli` cluster mode to connect to your cluster and try accessing keys in different shards. Observe the response messages.
diff --git a/docs/support/redis-at-scale/scalability/redis-cluster-and-client-libraries/index-redis-cluster-and-client-libraries.mdx b/docs/support/redis-at-scale/scalability/redis-cluster-and-client-libraries/index-redis-cluster-and-client-libraries.mdx
deleted file mode 100644
index 5a2f907..0000000
--- a/docs/support/redis-at-scale/scalability/redis-cluster-and-client-libraries/index-redis-cluster-and-client-libraries.mdx
+++ /dev/null
@@ -1,51 +0,0 @@
----
-id: index-redis-cluster-and-client-libraries
-title: 4.3 Redis Cluster and Client Libraries
-sidebar_label: 4.3 Redis Cluster and Client Libraries
-slug: /operate/redis-at-scale/scalability/redis-cluster-and-client-libraries
-custom_edit_url: null
----
-
-To use a client library with Redis Cluster, the client libraries need to be cluster-aware. Clients that support Redis Cluster typically feature a special connection module for managing connections to the cluster. The process that some of the better client libraries follow usually goes like this:
-
-The client connects to any shard in the cluster and gets the addresses of the rest of the shards. The client also fetches a mapping of hash slots to shards so it can know where to look for a key in a specific hash slot. This hash slot map is cached locally.
-
-
-
-
-
-When the client needs to read/write a key, it first runs the hashing function `(crc16)` on the key name and then modulo divides by 16384, which results in the key’s hash slot number.
-
-In the example below the hash slot number for the key “foo” is 12182. Then the client checks the hash slot number against the hash slot map to determine which shard it should connect to. In our example, the hash slot number 12182 lives on shard `127.0.0.1:7002`.
-
-Finally, the client connects to the shard and finds the key it needs to work with.
-
-
-
-
-
-If the topology of the cluster changes for any reason and the key has been moved, the shard will respond with an `(error) MOVED 15495 127.0.0.1:7006` error, returning the address of the new shard responsible for that key. This indicates to the client that it needs to re-query the cluster for its topology and hash slot allocation, so it will do that and update its local hash slot map for future queries.
-
-Not every client library has this extra logic built in, so when choosing a client library, make sure to look for ones with cluster support.
-
-Another detail to check is if the client stores the hash slot map locally. If it doesn’t, and it relies on the `(error) MOVED` response to get the address of the right shard, you can expect to have a much higher latency than usual because your client may have to make two network requests instead of one for a big part of the requests.
-
-Examples of clients that support Redis cluster:
-
-- Java: Jedis
-- .NET: StackExchange.Redis
-- Go: Radix, go-redis/redis
-- Node.js: node-redis, ioredis
-- Python: redis-py
-
-Here's a list of Redis Clients: [https://redis.io/clients](https://redis.io/clients)
diff --git a/docs/support/redis-at-scale/talking-to-redis/client-performance-improvements/index-client-performance-improvements.mdx b/docs/support/redis-at-scale/talking-to-redis/client-performance-improvements/index-client-performance-improvements.mdx
deleted file mode 100644
index bb13126..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/client-performance-improvements/index-client-performance-improvements.mdx
+++ /dev/null
@@ -1,45 +0,0 @@
----
-id: index-client-performance-improvements
-title: 1.4 Client Performance Improvements
-sidebar_label: 1.4 Client Performance Improvements
-slug: /operate/redis-at-scale/talking-to-redis/client-performance-improvements
-custom_edit_url: null
----
-
-## Connection management - Pooling
-
-As we showed in the previous section, Redis clients are responsible for managing connections to the Redis server. Creating and recreating new connections over and over again, creates a lot of unnecessary load on the server. A good client library will offer some way of optimizing connection management, by setting up a connection pool, for example.
-With connection pooling, the client library will instantiate a series of (persistent) connections to the Redis server and keep them open. When the application needs to send a request, the current thread will get one of these connections from the pool, use it, and return it when done.
-
-
-
-
-So if possible, always try to choose a client library that supports pooling connections,
-because that decision alone can have a huge influence on your system’s performance.
-
-## Pipelining
-
-As in any client-server application, Redis can handle many clients simultaneously.
-Each client does a (typically blocking) read on a socket and waits for the server response. The server reads the request from the socket, parses it, processes it, and writes the response to the socket. The time the data packets take to travel from the client to the server, and then back again, is called network round trip time, or RTT.
-If, for example, you needed to execute 50 commands, you would have to send a request and wait for the response 50 times, paying the RTT cost every single time. To tackle this problem, Redis can process new requests even if the client hasn't already read the old responses. This way, you can send multiple commands to the server without waiting for the replies at all; the replies are read in the end, in a single step.
-
-
-
-
-This technique is called pipelining and is another good way to improve the performance
-of your system. Most Redis libraries support this technique out of the box.
-
-
-Supplemental Reading:
-
-- [Redis Pipelining](https://redis.io/topics/pipelining)
-- [Redis Client Handling](https://redis.io/topics/clients)
-- [Connection Pools for Serverless Functions and Backend Services](https://redislabs.com/blog/connection-pools-for-serverless-functions-and-backend-services/)
diff --git a/docs/support/redis-at-scale/talking-to-redis/command-line-tool/index-command-line-tool.mdx b/docs/support/redis-at-scale/talking-to-redis/command-line-tool/index-command-line-tool.mdx
deleted file mode 100644
index f282daf..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/command-line-tool/index-command-line-tool.mdx
+++ /dev/null
@@ -1,41 +0,0 @@
----
-id: index-command-line-tool
-title: '1.1 The Command Line Tool: Redis-CLI'
-sidebar_label: '1.1 The Command Line Tool: Redis-CLI'
-slug: /operate/redis-at-scale/talking-to-redis/command-line-tool
-custom_edit_url: null
----
-
-
-
-
-
-
-
- Redis-cli is a command line tool used to interact with the Redis server. Most
- package managers include redis-cli as part of the redis package. It can also
- be compiled from source, and you'll find the source code in the Redis
- repository on GitHub.
-
-
-There are two ways to use redis-cli :
-
-- an interactive mode where the user types commands and sees the replies;
-- a command mode where the command is provided as an argument to redis-cli, executed, and results sent to the standard output.
-
-Let’s use the CLI to connect to a Redis server running at 172.22.0.3 and port 7000. The arguments -h and -p are used to specify the host and port to connect to. They can be omitted if your server is running on the default host "localhost" port 6379.
-
-The redis-cli provides some useful productivity features. For example, you can scroll through your command history by pressing the up and down arrow keys. You can also use the TAB key to autocomplete a command, saving even more keystrokes. Just type the first few letters of a command and keep pressing TAB until the command you want appears on screen.
-
-Once you have the command name you want, the CLI will display syntax hints about the arguments so you don’t have to remember all of them, or open up the Redis command documentation.
-
-These three tips can save you a lot of time and take you a step closer to being a power user.
-
-You can do much more with redis-cli, like sending output to a file, scanning for big keys, get continuous stats, monitor commands and so on. For a much more detailed explanation refer to the [documentation](https://redis.io/docs/manual/cli/).
diff --git a/docs/support/redis-at-scale/talking-to-redis/configuring-a-redis-server/index-configuring-a-redis-server.mdx b/docs/support/redis-at-scale/talking-to-redis/configuring-a-redis-server/index-configuring-a-redis-server.mdx
deleted file mode 100644
index 70bb6e1..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/configuring-a-redis-server/index-configuring-a-redis-server.mdx
+++ /dev/null
@@ -1,45 +0,0 @@
----
-id: index-configuring-a-redis-server
-title: 1.2 Configuring a Redis Server
-sidebar_label: 1.2 Configuring a Redis Server
-slug: /operate/redis-at-scale/talking-to-redis/configuring-a-redis-server
-custom_edit_url: null
----
-
-The self-documented Redis configuration file called `redis.conf` has been mentioned many times as an example of well written documentation. In this file you can find all possible Redis configuration directives, together with a detailed description of what they do and their default values.
-
-You should always adjust the `redis.conf` file to your needs and instruct Redis to run based on it's parameters when running Redis in production.
-
-The way to do that is by providing the path to the file when starting up your server:
-
-```bash
-$ redis-server./path/to/redis.conf
-```
-
-When you’re only starting a Redis server instance for testing purposes you can pass configuration directives directly on the command line:
-
-```bash
-$ redis-server --port 7000 --replicaof 127.0.0.1:6379
-```
-
-The format of the arguments passed via the command line is exactly the same as the one used in the redis.conf file, with the exception that the keyword is prefixed with `--`.
-
-Note that internally this generates an in-memory temporary config file where arguments are translated into the format of `redis.conf`.
-
-It is possible to reconfigure a running Redis server without stopping or restarting it by using the special commands `CONFIG SET` and `CONFIG GET`.
-
-```bash
-127.0.0.1:6379> CONFIG GET *
-
-127.0.0.1:6379> CONFIG SET something
-
-127.0.0.1:6379> CONFIG REWRITE
-```
-
-Not all the configuration directives are supported in this way, but you can check the output of the command `CONFIG GET *` first for a list of all the supported ones.
-
-:::tip
-
-Modifying the configuration on the fly has no effect on the `redis.conf` file. At the next restart of Redis the old configuration will be used instead. If you want to force update the `redis.conf` file with your current configuration settings you can run the `CONFIG REWRITE` command, which will automatically scan your `redis.conf` file and update the fields which don't match the current configuration value.
-
-:::
diff --git a/docs/support/redis-at-scale/talking-to-redis/index-talking-to-redis.mdx b/docs/support/redis-at-scale/talking-to-redis/index-talking-to-redis.mdx
deleted file mode 100644
index fe9d1eb..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/index-talking-to-redis.mdx
+++ /dev/null
@@ -1,21 +0,0 @@
----
-id: index-talking-to-redis
-title: Talking to Redis
-sidebar_label: Talking to Redis
-slug: /operate/redis-at-scale/talking-to-redis
-authors: [justin]
-custom_edit_url: null
----
-
-
-
-
-
-
diff --git a/docs/support/redis-at-scale/talking-to-redis/initial-tuning/index-initial-tuning.mdx b/docs/support/redis-at-scale/talking-to-redis/initial-tuning/index-initial-tuning.mdx
deleted file mode 100644
index be386c9..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/initial-tuning/index-initial-tuning.mdx
+++ /dev/null
@@ -1,127 +0,0 @@
----
-id: index-initial-tuning
-title: 1.5 Initial Tuning
-sidebar_label: 1.5 Initial Tuning
-slug: /operate/redis-at-scale/talking-to-redis/initial-tuning
-custom_edit_url: null
----
-
-We love Redis because it’s fast (and fun!), so as we begin to consider scaling out Redis, we first want to make sure we've done everything we can to maximize its performance.
-
-Let's start by looking at some important tuning parameters.
-
-## Max Clients
-
-Redis has a default of max of 10,000 clients; after that maximum has been reached, Redis will respond to all new connections with an error. If you have a lot of connections (or a lot of application instances), then you may need to go higher. You can set the max number of simultaneous clients in the Redis config file:
-
-```bash
-maxclients 20000
-```
-
-## Max Memory
-
-By default, Redis has no max memory limit, so it will use all available system memory. If you are using replication, you will want to limit the memory usage in order to have overhead for replica output buffers. It’s also a good idea to leave memory for the system. Something like 25% overhead. You can update this setting in Redis config file:
-
-```bash
-# memory size in bytes
-maxmemory 1288490188
-```
-
-## Set TCP-BACKLOG
-
-The Redis server uses the value of `tcp-backlog` to specify the size of the complete connection queue.
-
-Redis passes this configuration as the second parameter of the `listen(int s, int backlog)` call.
-
-If you have many connections, you will need to set this higher than the default of 511. You can update this in Redis config file:
-
-```bash
-# TCP listen() backlog.
-#
-# In high requests-per-second environments you need an high backlog in order
-# to avoid slow clients connections issues. Note that the Linux kernel
-# will silently truncate it to the value of /proc/sys/net/core/somaxconn so
-# make sure to raise both the value of somaxconn and tcp_max_syn_backlog
-# in order to get the desired effect.
-tcp-backlog 65536
-```
-
-As the comment in `redis.conf` indicates, the value of `somaxconn` and `tcp_max_syn_backlog` may need to be increased at the OS level as well.
-
-## Set Read Replica Configurations
-
-One simple way to scale Redis is to add read replicas and take load off of the primary. This is most effective when you have a read-heavy (as opposed to write-heavy) workload. You will probably want to have the replica available and still serving stale data, even if the replication is not completed. You can update this in the Redis config:
-
-```bash
-slave-serve-stale-data yes
-```
-
-You will also want to prevent any writes from happening on the replicas. You can update this in the Redis config:
-
-```bash
-slave-read-only yes
-```
-
-## Kernel Memory
-
-Under high load, occasional performance dips can occur due to memory allocation. This is something Salvatore, the creator of Redis, blogged about in the past. The performance issue is related to transparent hugepages, which you can disable at the OS level if needed.
-
-```bash
-$ echo 'never' > /sys/kernel/mm/transparent_hugepage/enabled
-```
-
-## Kernel Network Stack
-
-If you plan on handling a large number of connections in a high performance environment, we recommend tuning the following kernel parameters:
-
-```bash
-vm.swappiness=0 # turn off swapping
-net.ipv4.tcp_sack=1 # enable selective acknowledgements
-net.ipv4.tcp_timestamps=1 # needed for selective acknowledgements
-net.ipv4.tcp_window_scaling=1 # scale the network window
-net.ipv4.tcp_congestion_control=cubic # better congestion algorithm
-net.ipv4.tcp_syncookies=1 # enable syn cookies
-net.ipv4.tcp_tw_recycle=1 # recycle sockets quickly
-net.ipv4.tcp_max_syn_backlog=NUMBER # backlog setting
-net.core.somaxconn=NUMBER # up the number of connections per port
-net.core.rmem_max=NUMBER # up the receive buffer size
-net.core.wmem_max=NUMBER # up the buffer size for all connections
-```
-
-## File Descriptor Limits
-
-If you do not set the correct number of file descriptors for the Redis user, you will see errors indicating that “Redis can’t set maximum open files..” You can increase the file descriptor limit at the OS level.
-
-Here's an example on Ubuntu using systemd:
-
-```bash
-/etc/systemd/system/redis.service
-[Service]
-...
-User=redis
-Group=redis
-...
-LimitNOFILE=65536
-...
-```
-
-You will then need to reload the daemon and restart the redis service.
-
-## Enabling RPS (Receive Packet Steering) and CPU preferences
-
-One way we can improve performance is to prevent Redis from running on the same CPUs as those handling any network traffic. This can be accomplished by enabling RPS for our network interfaces and creating some CPU affinity for our Redis process.
-
-Here is an example. First we can enable RPS on CPUs 0-1:
-
-```bash
-$ echo '3' > /sys/class/net/eth1/queues/rx-0/rps_cpus
-```
-
-Then we can set the CPU affinity for redis to CPUs 2-8:
-
-```bash
-# config is set to write pid to /var/run/redis.pid
-$ taskset -pc 2-8 `cat /var/run/redis.pid`
-pid 8946's current affinity list: 0-8
-pid 8946's new affinity list: 2-8
-```
diff --git a/docs/support/redis-at-scale/talking-to-redis/redis-clients/index-redis-clients.mdx b/docs/support/redis-at-scale/talking-to-redis/redis-clients/index-redis-clients.mdx
deleted file mode 100644
index 81a9771..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/redis-clients/index-redis-clients.mdx
+++ /dev/null
@@ -1,39 +0,0 @@
----
-id: index-redis-clients
-title: 1.3 Redis Clients
-sidebar_label: 1.3 Redis Clients
-slug: /operate/redis-at-scale/talking-to-redis/redis-clients
-custom_edit_url: null
----
-
-
-
-
-
-
-
- Redis has a client-server architecture and uses a request-response model.
- Applications send requests to the Redis server, which processes them and
- returns responses for each. The role of a Redis client library is to act as an
- intermediary between your application and the Redis server.
-
-
-Client libraries perform the following duties:
-
-- Implement the Redis wire protocol - the format used to send requests to and receive responses from the Redis server
-- Provide an idiomatic API for using Redis commands from a particular programming language
-
-## Managing the connection to Redis
-
-Redis clients communicate with the Redis server over TCP, using a protocol called [RESP](https://redis.io/docs/reference/protocol-spec/) (REdis Serialization Protocol) designed specifically for Redis.
-
-The RESP protocol is simple and text-based, so it is easily read by humans, as well as machines. A common request/response would look something like this. Note that we're using netcat here to send raw protocol:
-
-This simple, well documented protocol has resulted in Redis clients for almost every language you can think of. The [redis.io](https://redis.io/docs/clients/) client page lists over 200 client libraries for more than 50 programming languages.
diff --git a/docs/support/redis-at-scale/talking-to-redis/redis-server-overview/index-redis-server-overview.mdx b/docs/support/redis-at-scale/talking-to-redis/redis-server-overview/index-redis-server-overview.mdx
deleted file mode 100644
index 6bedc74..0000000
--- a/docs/support/redis-at-scale/talking-to-redis/redis-server-overview/index-redis-server-overview.mdx
+++ /dev/null
@@ -1,35 +0,0 @@
----
-id: index-redis-server-overview
-title: 1.0 Redis Server Overview
-sidebar_label: 1.0 Redis Server Overview
-slug: /operate/redis-at-scale/talking-to-redis/redis-server-overview
-custom_edit_url: null
----
-
-
-
-
-
-
-
- As you might already know, Redis is an open source data structure server
- written in C. You can store multiple data types, like strings, hashes, and
- streams and access them by a unique key name.
-
-
-For example, if you have a string value “Hello World” saved under the key name “greeting”, you can access it by running the GET command followed by the key name - greeting. All keys in a Redis database are stored in a flat keyspace. There is no enforced schema or naming policy, and the responsibility for organizing the keyspace is left to the developer.
-
-The speed Redis is famous for is mostly due to the fact that Redis stores and serves data entirely from RAM instead of disk, as most other databases do. Another contributing factor is its predominantly single-threaded nature: single-threading avoids race conditions and CPU-heavy context switching associated with threads.
-
-Indeed, this means that open source Redis can’t take advantage of the processing power of multiple CPU cores, although CPU is rarely the bottleneck with Redis. You are more likely to bump up against memory or network limitations before hitting any CPU limitations. That said, Redis Enterprise does let you take advantage of all of the cores on a single machine.
-
-Let’s now look at exactly what happens behind the scenes with every Redis request. When a client sends a request to a Redis server, the request is first read from the socket, then parsed and processed and finally, the response is written back to the socket and sent to the user. The reading and especially writing to a socket are expensive operations, so in Redis version 6.0 multi-threaded I/O was introduced. When this feature is enabled, Redis can delegate the time spent reading and writing to I/O sockets over to other threads, freeing up cycles for storing and retrieving data and boosting overall performance by up to a factor of two for some workloads.
-
-Throughout the rest of the section, you’ll learn how to use the Redis command-line interface, how to configure your Redis server, and how to choose and tune your Redis client library.
diff --git a/docusaurus.config.js b/docusaurus.config.js
index 3859bdf..8293216 100644
--- a/docusaurus.config.js
+++ b/docusaurus.config.js
@@ -36,7 +36,6 @@ module.exports = {
themeConfig:
/** @type {import('@docusaurus/preset-classic').ThemeConfig} */
({
- image: '/img/redis-hero-image.jpg',
// ...
// googleTagManager: {
// trackingID: 'GTM-W8Z6BLQ',
@@ -119,7 +118,7 @@ module.exports = {
disableSwitch: false,
},
announcementBar: {
- id: 'redis-7-2-release', // Any value that will identify this message.
+ id: 'yass-release', // Any value that will identify this message.
content:
'