-# -*- coding: utf-8 -*-
-"""
-The entry point of the web UI that renders the log (can be still running).
-"""
-
-importre
-frommultiprocessingimportProcess,Queue
-
-importtime
-fromtypingimportGenerator,List,Any
-
-
-try:
- importgradioasgr
- fromgradio_groupchatimportGroupChat
-exceptImportError:
- gr=None
- GroupChat=Any
-
-try:
- fromagentscope.constantsimportMSG_TOKEN
-exceptImportError:
- PACKAGE_NAME="agentscope"
- MSG_TOKEN=f"<{PACKAGE_NAME}_msg>"
-
-
-
-[docs]
-deffollow_read(
- logfile_path:str,
- skip_existing_content:bool=False,
-)->Generator:
-"""Read a file in online and iterative manner
-
- Args:
- logfile_path (`str`):
- The file path to be read.
- skip_existing_content (`bool`, defaults to `False`):
- If True, read from the end, otherwise read from the beginning.
-
- Returns:
- One line string of the file content.
- """
- # in most unix file systems, the read operation is safe
- # for a file being target file of another "write process"
- withopen(logfile_path,"r",encoding="utf-8",errors="ignore")aslogfile:
- ifskip_existing_content:
- # move to the file's end, similar to `tail -f`
- logfile.seek(0,2)
-
- whileTrue:
- line=logfile.readline()
- ifnotline:
- # no new line, wait to avoid CPU override
- time.sleep(0.1)
- continue
- yieldline
-[docs]
-defis_chat_message_format(s:str)->bool:
-"""Check the string to be parsed, whether be a chat-message.
-
- Args:
- s (`str`): the message as string.
-
- Returns:
- `bool`: the indicator as a bool
- """
- iflog_pattern.match(s):
- returnFalse
- ifchat_message_pattern.match(s):
- returnTrue
- returnFalse
-
-
-
-# TODO:
-# - show image, audio, and data in other modality
-# - make the dialog box expensive to show detailed info
-
-
-MAX_CHAT_SIZE=20
-FULL_LOG_MAX_LINE=50
-SKIP_HISTORY_LOG=False
-
-glb_queue_chat_msg=Queue()
-glb_queue_system_log=Queue()
-glb_queue_file_name=Queue()
-glb_current_file_name=None
-
-glb_history_chat=[]
-glb_history_system_log=[]
-
-
-
-[docs]
-defput_file_name(file_name:str)->str:
-"""Store the file_name given by user, via the queue
-
- Args:
- file_name (`str`):
- The file_name given by user
-
- Returns:
- `str`: The given file name.
- """
- globalglb_queue_file_name
- iffile_name:
- glb_queue_file_name.put(file_name)
- returnfile_name
-
-
-
-
-[docs]
-defget_chat()->List[List]:
-"""Load the chat info from the queue, and put it into the history
-
- Returns:
- `List[List]`: The parsed history, list of tuple, [(role, msg), ...]
-
- """
- globalglb_history_chat,glb_queue_chat_msg
- ifnotglb_queue_chat_msg.empty():
- line=glb_queue_chat_msg.get(block=False)
- line=line.replace(MSG_TOKEN,"")
- match_res=chat_message_pattern.match(line)
- ifmatch_resisnotNone:
- role=match_res.group("role")
- message=match_res.group("message")
- glb_history_chat+=[[role,message]]
- glb_history_chat=glb_history_chat[:MAX_CHAT_SIZE]
- returnglb_history_chat
-
-
-
-
-[docs]
-defget_system_log()->str:
-"""Load the system log info from the queue, and collect the res into a str
-
- Returns:
- The collected string.
- """
- globalglb_history_system_log,glb_queue_system_log
- ifnotglb_queue_system_log.empty():
- line=glb_queue_system_log.get(block=False)
- glb_history_system_log.append(line)
- glb_history_system_log=glb_history_system_log[:FULL_LOG_MAX_LINE]
- return"".join(glb_history_system_log)
- return"".join(glb_history_system_log)
-
-
-
-
-[docs]
-defhandle_file_selection(
- queue_file_name:Queue,
- queue_system_log:Queue,
- queue_chat:Queue,
-)->None:
-"""
-
- Args:
- queue_file_name (`Queue`):
- The queue to store and pass `file_name` given by user.
- queue_system_log (`Queue`):
- The queue to store and pass system log within the file
- queue_chat (`Queue`):
- The queue to store and pass chat msg within the file
- """
- globalglb_current_file_name
- total_msg_cnt=0
- full_msg=""
- whileTrue:
- ifglb_current_file_name:
- forlineinfollow_read(
- glb_current_file_name,
- skip_existing_content=SKIP_HISTORY_LOG,
- ):
- msg_cnt=line.count(MSG_TOKEN)
- total_msg_cnt+=msg_cnt
- # print("line is: ", line)
- # print("total_msg_cnt is: ", total_msg_cnt)
- ifmsg_cnt%2==0andmsg_cnt!=0:
- # contain both begin and end <msg> in the same line
- queue_chat.put(line)
- elifmsg_cnt%2==0andtotal_msg_cnt%2==1:
- # no or paired msg token this line,
- # but still waiting for the end of msg
- full_msg+=line.strip()
- elifmsg_cnt%2==1andtotal_msg_cnt%2==0:
- # the left msg token, waiting for the end of the msg
- parts=line.split(MSG_TOKEN)
- full_msg+="".join(parts[1:])# the right remaining parts
- elifmsg_cnt%2==1andtotal_msg_cnt%2==1:
- # the right msg token
- parts=line.split(MSG_TOKEN)
- full_msg+="".join(parts[:-1])# the left remaining parts
- # reset the full_msg
- queue_chat.put(full_msg)
- full_msg=""
- else:
- queue_system_log.put(line)
-
- # using the up-to-date file name within the queue
- find_new_file_to_read=False
- whilenotqueue_file_name.empty():
- glb_current_file_name=queue_file_name.get(block=False)
- find_new_file_to_read=True
- iffind_new_file_to_read:
- break
- else:
- # wait users to determine the log file
- time.sleep(1)
- whilenotqueue_file_name.empty():
- glb_current_file_name=queue_file_name.get(block=False)
diff --git a/_sources/agentscope.web_ui.rst.txt b/_sources/agentscope.web.rst.txt
similarity index 80%
rename from _sources/agentscope.web_ui.rst.txt
rename to _sources/agentscope.web.rst.txt
index edc09867a..3fc7529bc 100644
--- a/_sources/agentscope.web_ui.rst.txt
+++ b/_sources/agentscope.web.rst.txt
@@ -4,7 +4,7 @@ Web UI package
app module
-----------------------------
-.. automodule:: agentscope.web_ui.app
+.. automodule:: agentscope.web.app
:members:
:undoc-members: gradio_groupchat
:show-inheritance:
diff --git a/_sources/index.rst.txt b/_sources/index.rst.txt
index 611e16542..9033b1ee1 100644
--- a/_sources/index.rst.txt
+++ b/_sources/index.rst.txt
@@ -35,7 +35,7 @@ AgentScope Documentation
agentscope.service
agentscope.rpc
agentscope.utils
- agentscope.web_ui
+ agentscope.web
agentscope
Indices and tables
diff --git a/_sources/tutorial/102-concepts.md.txt b/_sources/tutorial/102-concepts.md.txt
index a83da431e..745c2cc50 100644
--- a/_sources/tutorial/102-concepts.md.txt
+++ b/_sources/tutorial/102-concepts.md.txt
@@ -29,7 +29,7 @@ AgentScope
│ | ├── service # Services offering functions independent of memory and state.
│ | ├── utils # Auxiliary utilities and helper functions.
│ | ├── message.py # Definitions and implementations of messaging between agents.
-| | ├── web_ui # WebUI used to show dialogs.
+| | ├── web # WebUI used to show dialogs.
│ | ├── prompt.py # Prompt engineering module for model input.
│ | ├── ... ..
│ | ├── ... ..
diff --git a/_sources/tutorial/105-logging.md.txt b/_sources/tutorial/105-logging.md.txt
index 42e05b5ff..dd6cbbc56 100644
--- a/_sources/tutorial/105-logging.md.txt
+++ b/_sources/tutorial/105-logging.md.txt
@@ -64,45 +64,32 @@ logger.error("The agent encountered an unexpected error while processing a reque
## Integrating logging with WebUI
-To visualize these logs, we provide a customized gradio component in `src/agentscope/web_ui`.
+To visualize these logs and running details, AgentScope provides a simple
+web interface.
### Quick Running
-For convince, we provide the pre-built app in a wheel file, you can run the WebUI in the following command:
+You can run the WebUI in the following python code:
-```shell
-pip install gradio_groupchat-0.0.1-py3-none-any.whl
-python app.py
-```
-
-After the init and entering the UI port printed by `app.py`, e.g., `http://127.0.0.1:7860/`, you can choose `run.log.demo` in the top-middle `FileSelector` window (it's a demo log file provided by us). Then, the dialog and system log should be shown correctly in the bottom windows.
+```python
+import agentscope
-
+agentscope.web.init(
+ path_save="YOUR_SAVE_PATH"
+)
+```
-### For Other Customization
+By this way, you can see all the running instances and projects in `http://127.
+0.0.1:5000` as follows:
-To customize the backend, or the frontend of the provided WebUI, you can
+
-```shell
-# generate the template codes
-# for network connectivity problem, try to run
-# `npm config rm proxy && npm config rm https-proxy` first
-gradio cc create GroupChat --template Chatbot
-# replace the generated app.py into our built-in app.py
-cp -f app.py groupchat/demo
-# debug and develop your web_ui
-cd groupchat
-# edit the app.py, or other parts you want, reference link:
-# https://www.gradio.app/guides/custom-components-in-five-minutes
-gradio cc dev
-```
+By clicking a running instance, we can observe more details.
-If you want to release the modification, you can do
+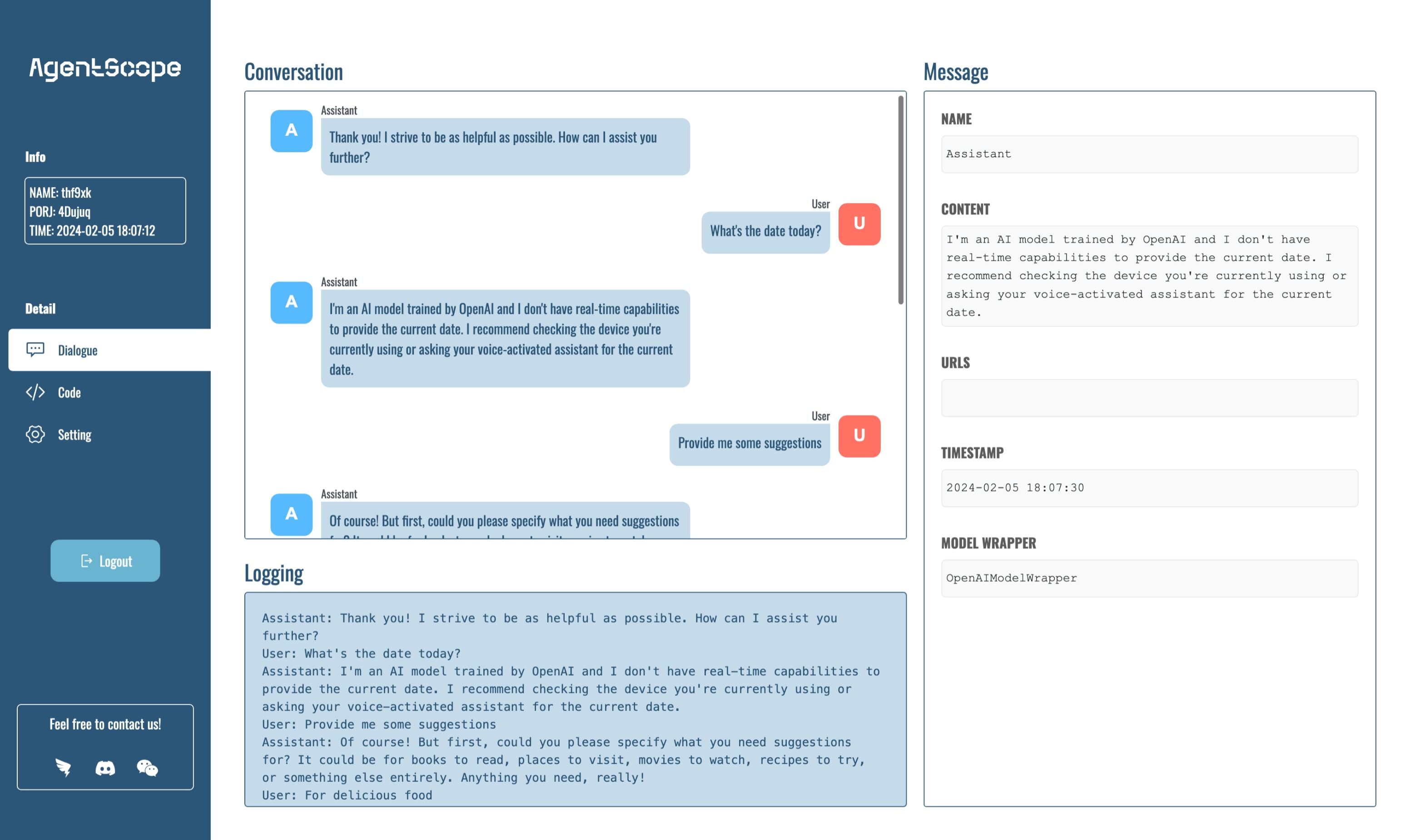
-```shell
-gradio cc build
-pip install
-python app.py
-```
+### Note
+The WebUI is still under development. We will provide more features and
+better user experience in the future.
[[Return to the top]](#logging-and-webui)
diff --git a/agentscope.agents.html b/agentscope.agents.html
index bcd25cea4..e1e2a656b 100644
--- a/agentscope.agents.html
+++ b/agentscope.agents.html
@@ -134,7 +134,7 @@
After the init and entering the UI port printed by app.py, e.g., http://127.0.0.1:7860/, you can choose run.log.demo in the top-middle FileSelector window (it’s a demo log file provided by us). Then, the dialog and system log should be shown correctly in the bottom windows.
-
+
By this way, you can see all the running instances and projects in http://127.0.0.1:5000 as follows:
+
+
By clicking a running instance, we can observe more details.
To customize the backend, or the frontend of the provided WebUI, you can
-
# generate the template codes
-# for network connectivity problem, try to run
-# `npm config rm proxy && npm config rm https-proxy` first
-gradiocccreateGroupChat--templateChatbot
-# replace the generated app.py into our built-in app.py
-cp-fapp.pygroupchat/demo
-# debug and develop your web_ui
-cdgroupchat
-# edit the app.py, or other parts you want, reference link:
-# https://www.gradio.app/guides/custom-components-in-five-minutes
-gradioccdev
-
-
-
If you want to release the modification, you can do