new BufferList([ callback ])
+ * bl.length
+ * bl.append(buffer)
+ * bl.get(index)
+ * bl.slice([ start[, end ] ])
+ * bl.copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])
+ * bl.duplicate()
+ * bl.consume(bytes)
+ * bl.toString([encoding, [ start, [ end ]]])
+ * bl.readDoubleBE()
, bl.readDoubleLE()
, bl.readFloatBE()
, bl.readFloatLE()
, bl.readInt32BE()
, bl.readInt32LE()
, bl.readUInt32BE()
, bl.readUInt32LE()
, bl.readInt16BE()
, bl.readInt16LE()
, bl.readUInt16BE()
, bl.readUInt16LE()
, bl.readInt8()
, bl.readUInt8()
+ * Streams
+
+--------------------------------------------------------
+
+### new BufferList([ callback | Buffer | Buffer array | BufferList | BufferList array | String ])
+The constructor takes an optional callback, if supplied, the callback will be called with an error argument followed by a reference to the **bl** instance, when `bl.end()` is called (i.e. from a piped stream). This is a convenient method of collecting the entire contents of a stream, particularly when the stream is *chunky*, such as a network stream.
+
+Normally, no arguments are required for the constructor, but you can initialise the list by passing in a single `Buffer` object or an array of `Buffer` object.
+
+`new` is not strictly required, if you don't instantiate a new object, it will be done automatically for you so you can create a new instance simply with:
+
+```js
+var bl = require('bl')
+var myinstance = bl()
+
+// equivilant to:
+
+var BufferList = require('bl')
+var myinstance = new BufferList()
+```
+
+--------------------------------------------------------
+
+### bl.length
+Get the length of the list in bytes. This is the sum of the lengths of all of the buffers contained in the list, minus any initial offset for a semi-consumed buffer at the beginning. Should accurately represent the total number of bytes that can be read from the list.
+
+--------------------------------------------------------
+
+### bl.append(Buffer | Buffer array | BufferList | BufferList array | String)
+`append(buffer)` adds an additional buffer or BufferList to the internal list. `this` is returned so it can be chained.
+
+--------------------------------------------------------
+
+### bl.get(index)
+`get()` will return the byte at the specified index.
+
+--------------------------------------------------------
+
+### bl.slice([ start, [ end ] ])
+`slice()` returns a new `Buffer` object containing the bytes within the range specified. Both `start` and `end` are optional and will default to the beginning and end of the list respectively.
+
+If the requested range spans a single internal buffer then a slice of that buffer will be returned which shares the original memory range of that Buffer. If the range spans multiple buffers then copy operations will likely occur to give you a uniform Buffer.
+
+--------------------------------------------------------
+
+### bl.copy(dest, [ destStart, [ srcStart [, srcEnd ] ] ])
+`copy()` copies the content of the list in the `dest` buffer, starting from `destStart` and containing the bytes within the range specified with `srcStart` to `srcEnd`. `destStart`, `start` and `end` are optional and will default to the beginning of the `dest` buffer, and the beginning and end of the list respectively.
+
+--------------------------------------------------------
+
+### bl.duplicate()
+`duplicate()` performs a **shallow-copy** of the list. The internal Buffers remains the same, so if you change the underlying Buffers, the change will be reflected in both the original and the duplicate. This method is needed if you want to call `consume()` or `pipe()` and still keep the original list.Example:
+
+```js
+var bl = new BufferList()
+
+bl.append('hello')
+bl.append(' world')
+bl.append('\n')
+
+bl.duplicate().pipe(process.stdout, { end: false })
+
+console.log(bl.toString())
+```
+
+--------------------------------------------------------
+
+### bl.consume(bytes)
+`consume()` will shift bytes *off the start of the list*. The number of bytes consumed don't need to line up with the sizes of the internal Buffers—initial offsets will be calculated accordingly in order to give you a consistent view of the data.
+
+--------------------------------------------------------
+
+### bl.toString([encoding, [ start, [ end ]]])
+`toString()` will return a string representation of the buffer. The optional `start` and `end` arguments are passed on to `slice()`, while the `encoding` is passed on to `toString()` of the resulting Buffer. See the [Buffer#toString()](http://nodejs.org/docs/latest/api/buffer.html#buffer_buf_tostring_encoding_start_end) documentation for more information.
+
+--------------------------------------------------------
+
+### bl.readDoubleBE(), bl.readDoubleLE(), bl.readFloatBE(), bl.readFloatLE(), bl.readInt32BE(), bl.readInt32LE(), bl.readUInt32BE(), bl.readUInt32LE(), bl.readInt16BE(), bl.readInt16LE(), bl.readUInt16BE(), bl.readUInt16LE(), bl.readInt8(), bl.readUInt8()
+
+All of the standard byte-reading methods of the `Buffer` interface are implemented and will operate across internal Buffer boundaries transparently.
+
+See the [Buffer](http://nodejs.org/docs/latest/api/buffer.html)
documentation for how these work.
+
+--------------------------------------------------------
+
+### Streams
+**bl** is a Node **[Duplex Stream](http://nodejs.org/docs/latest/api/stream.html#stream_class_stream_duplex)**, so it can be read from and written to like a standard Node stream. You can also `pipe()` to and from a **bl** instance.
+
+--------------------------------------------------------
+
+## Contributors
+
+**bl** is brought to you by the following hackers:
+
+ * [Rod Vagg](https://github.com/rvagg)
+ * [Matteo Collina](https://github.com/mcollina)
+ * [Jarett Cruger](https://github.com/jcrugzz)
+
+=======
+
+
+## License & copyright
+
+Copyright (c) 2013-2014 bl contributors (listed above).
+
+bl is licensed under the MIT license. All rights not explicitly granted in the MIT license are reserved. See the included LICENSE.md file for more details.
diff --git a/node_modules/bl/bl.js b/node_modules/bl/bl.js
new file mode 100644
index 0000000..f585df1
--- /dev/null
+++ b/node_modules/bl/bl.js
@@ -0,0 +1,243 @@
+var DuplexStream = require('readable-stream/duplex')
+ , util = require('util')
+
+
+function BufferList (callback) {
+ if (!(this instanceof BufferList))
+ return new BufferList(callback)
+
+ this._bufs = []
+ this.length = 0
+
+ if (typeof callback == 'function') {
+ this._callback = callback
+
+ var piper = function piper (err) {
+ if (this._callback) {
+ this._callback(err)
+ this._callback = null
+ }
+ }.bind(this)
+
+ this.on('pipe', function onPipe (src) {
+ src.on('error', piper)
+ })
+ this.on('unpipe', function onUnpipe (src) {
+ src.removeListener('error', piper)
+ })
+ } else {
+ this.append(callback)
+ }
+
+ DuplexStream.call(this)
+}
+
+
+util.inherits(BufferList, DuplexStream)
+
+
+BufferList.prototype._offset = function _offset (offset) {
+ var tot = 0, i = 0, _t
+ for (; i < this._bufs.length; i++) {
+ _t = tot + this._bufs[i].length
+ if (offset < _t)
+ return [ i, offset - tot ]
+ tot = _t
+ }
+}
+
+
+BufferList.prototype.append = function append (buf) {
+ var i = 0
+ , newBuf
+
+ if (Array.isArray(buf)) {
+ for (; i < buf.length; i++)
+ this.append(buf[i])
+ } else if (buf instanceof BufferList) {
+ // unwrap argument into individual BufferLists
+ for (; i < buf._bufs.length; i++)
+ this.append(buf._bufs[i])
+ } else if (buf != null) {
+ // coerce number arguments to strings, since Buffer(number) does
+ // uninitialized memory allocation
+ if (typeof buf == 'number')
+ buf = buf.toString()
+
+ newBuf = Buffer.isBuffer(buf) ? buf : new Buffer(buf)
+ this._bufs.push(newBuf)
+ this.length += newBuf.length
+ }
+
+ return this
+}
+
+
+BufferList.prototype._write = function _write (buf, encoding, callback) {
+ this.append(buf)
+
+ if (typeof callback == 'function')
+ callback()
+}
+
+
+BufferList.prototype._read = function _read (size) {
+ if (!this.length)
+ return this.push(null)
+
+ size = Math.min(size, this.length)
+ this.push(this.slice(0, size))
+ this.consume(size)
+}
+
+
+BufferList.prototype.end = function end (chunk) {
+ DuplexStream.prototype.end.call(this, chunk)
+
+ if (this._callback) {
+ this._callback(null, this.slice())
+ this._callback = null
+ }
+}
+
+
+BufferList.prototype.get = function get (index) {
+ return this.slice(index, index + 1)[0]
+}
+
+
+BufferList.prototype.slice = function slice (start, end) {
+ return this.copy(null, 0, start, end)
+}
+
+
+BufferList.prototype.copy = function copy (dst, dstStart, srcStart, srcEnd) {
+ if (typeof srcStart != 'number' || srcStart < 0)
+ srcStart = 0
+ if (typeof srcEnd != 'number' || srcEnd > this.length)
+ srcEnd = this.length
+ if (srcStart >= this.length)
+ return dst || new Buffer(0)
+ if (srcEnd <= 0)
+ return dst || new Buffer(0)
+
+ var copy = !!dst
+ , off = this._offset(srcStart)
+ , len = srcEnd - srcStart
+ , bytes = len
+ , bufoff = (copy && dstStart) || 0
+ , start = off[1]
+ , l
+ , i
+
+ // copy/slice everything
+ if (srcStart === 0 && srcEnd == this.length) {
+ if (!copy) // slice, just return a full concat
+ return Buffer.concat(this._bufs)
+
+ // copy, need to copy individual buffers
+ for (i = 0; i < this._bufs.length; i++) {
+ this._bufs[i].copy(dst, bufoff)
+ bufoff += this._bufs[i].length
+ }
+
+ return dst
+ }
+
+ // easy, cheap case where it's a subset of one of the buffers
+ if (bytes <= this._bufs[off[0]].length - start) {
+ return copy
+ ? this._bufs[off[0]].copy(dst, dstStart, start, start + bytes)
+ : this._bufs[off[0]].slice(start, start + bytes)
+ }
+
+ if (!copy) // a slice, we need something to copy in to
+ dst = new Buffer(len)
+
+ for (i = off[0]; i < this._bufs.length; i++) {
+ l = this._bufs[i].length - start
+
+ if (bytes > l) {
+ this._bufs[i].copy(dst, bufoff, start)
+ } else {
+ this._bufs[i].copy(dst, bufoff, start, start + bytes)
+ break
+ }
+
+ bufoff += l
+ bytes -= l
+
+ if (start)
+ start = 0
+ }
+
+ return dst
+}
+
+BufferList.prototype.toString = function toString (encoding, start, end) {
+ return this.slice(start, end).toString(encoding)
+}
+
+BufferList.prototype.consume = function consume (bytes) {
+ while (this._bufs.length) {
+ if (bytes >= this._bufs[0].length) {
+ bytes -= this._bufs[0].length
+ this.length -= this._bufs[0].length
+ this._bufs.shift()
+ } else {
+ this._bufs[0] = this._bufs[0].slice(bytes)
+ this.length -= bytes
+ break
+ }
+ }
+ return this
+}
+
+
+BufferList.prototype.duplicate = function duplicate () {
+ var i = 0
+ , copy = new BufferList()
+
+ for (; i < this._bufs.length; i++)
+ copy.append(this._bufs[i])
+
+ return copy
+}
+
+
+BufferList.prototype.destroy = function destroy () {
+ this._bufs.length = 0
+ this.length = 0
+ this.push(null)
+}
+
+
+;(function () {
+ var methods = {
+ 'readDoubleBE' : 8
+ , 'readDoubleLE' : 8
+ , 'readFloatBE' : 4
+ , 'readFloatLE' : 4
+ , 'readInt32BE' : 4
+ , 'readInt32LE' : 4
+ , 'readUInt32BE' : 4
+ , 'readUInt32LE' : 4
+ , 'readInt16BE' : 2
+ , 'readInt16LE' : 2
+ , 'readUInt16BE' : 2
+ , 'readUInt16LE' : 2
+ , 'readInt8' : 1
+ , 'readUInt8' : 1
+ }
+
+ for (var m in methods) {
+ (function (m) {
+ BufferList.prototype[m] = function (offset) {
+ return this.slice(offset, offset + methods[m])[m](0)
+ }
+ }(m))
+ }
+}())
+
+
+module.exports = BufferList
diff --git a/node_modules/bl/node_modules/isarray/.npmignore b/node_modules/bl/node_modules/isarray/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/.npmignore
@@ -0,0 +1 @@
+node_modules
diff --git a/node_modules/bl/node_modules/isarray/.travis.yml b/node_modules/bl/node_modules/isarray/.travis.yml
new file mode 100644
index 0000000..cc4dba2
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/.travis.yml
@@ -0,0 +1,4 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
diff --git a/node_modules/bl/node_modules/isarray/Makefile b/node_modules/bl/node_modules/isarray/Makefile
new file mode 100644
index 0000000..787d56e
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/Makefile
@@ -0,0 +1,6 @@
+
+test:
+ @node_modules/.bin/tape test.js
+
+.PHONY: test
+
diff --git a/node_modules/bl/node_modules/isarray/README.md b/node_modules/bl/node_modules/isarray/README.md
new file mode 100644
index 0000000..16d2c59
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/README.md
@@ -0,0 +1,60 @@
+
+# isarray
+
+`Array#isArray` for older browsers.
+
+[](http://travis-ci.org/juliangruber/isarray)
+[](https://www.npmjs.org/package/isarray)
+
+[
+](https://ci.testling.com/juliangruber/isarray)
+
+## Usage
+
+```js
+var isArray = require('isarray');
+
+console.log(isArray([])); // => true
+console.log(isArray({})); // => false
+```
+
+## Installation
+
+With [npm](http://npmjs.org) do
+
+```bash
+$ npm install isarray
+```
+
+Then bundle for the browser with
+[browserify](https://github.com/substack/browserify).
+
+With [component](http://component.io) do
+
+```bash
+$ component install juliangruber/isarray
+```
+
+## License
+
+(MIT)
+
+Copyright (c) 2013 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
+of the Software, and to permit persons to whom the Software is furnished to do
+so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/node_modules/bl/node_modules/isarray/component.json b/node_modules/bl/node_modules/isarray/component.json
new file mode 100644
index 0000000..9e31b68
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/component.json
@@ -0,0 +1,19 @@
+{
+ "name" : "isarray",
+ "description" : "Array#isArray for older browsers",
+ "version" : "0.0.1",
+ "repository" : "juliangruber/isarray",
+ "homepage": "https://github.com/juliangruber/isarray",
+ "main" : "index.js",
+ "scripts" : [
+ "index.js"
+ ],
+ "dependencies" : {},
+ "keywords": ["browser","isarray","array"],
+ "author": {
+ "name": "Julian Gruber",
+ "email": "mail@juliangruber.com",
+ "url": "http://juliangruber.com"
+ },
+ "license": "MIT"
+}
diff --git a/node_modules/bl/node_modules/isarray/index.js b/node_modules/bl/node_modules/isarray/index.js
new file mode 100644
index 0000000..a57f634
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/index.js
@@ -0,0 +1,5 @@
+var toString = {}.toString;
+
+module.exports = Array.isArray || function (arr) {
+ return toString.call(arr) == '[object Array]';
+};
diff --git a/node_modules/bl/node_modules/isarray/package.json b/node_modules/bl/node_modules/isarray/package.json
new file mode 100644
index 0000000..3f088fb
--- /dev/null
+++ b/node_modules/bl/node_modules/isarray/package.json
@@ -0,0 +1,73 @@
+{
+ "_from": "isarray@~1.0.0",
+ "_id": "isarray@1.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-u5NdSFgsuhaMBoNJV6VKPgcSTxE=",
+ "_location": "/bl/isarray",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "isarray@~1.0.0",
+ "name": "isarray",
+ "escapedName": "isarray",
+ "rawSpec": "~1.0.0",
+ "saveSpec": null,
+ "fetchSpec": "~1.0.0"
+ },
+ "_requiredBy": [
+ "/bl/readable-stream"
+ ],
+ "_resolved": "https://registry.npmjs.org/isarray/-/isarray-1.0.0.tgz",
+ "_shasum": "bb935d48582cba168c06834957a54a3e07124f11",
+ "_spec": "isarray@~1.0.0",
+ "_where": "C:\\Users\\matth\\Documents\\GitHub\\Moonglow\\node_modules\\bl\\node_modules\\readable-stream",
+ "author": {
+ "name": "Julian Gruber",
+ "email": "mail@juliangruber.com",
+ "url": "http://juliangruber.com"
+ },
+ "bugs": {
+ "url": "https://github.com/juliangruber/isarray/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {},
+ "deprecated": false,
+ "description": "Array#isArray for older browsers",
+ "devDependencies": {
+ "tape": "~2.13.4"
+ },
+ "homepage": "https://github.com/juliangruber/isarray",
+ "keywords": [
+ "browser",
+ "isarray",
+ "array"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "isarray",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/juliangruber/isarray.git"
+ },
+ "scripts": {
+ "test": "tape test.js"
+ },
+ "testling": {
+ "files": "test.js",
+ "browsers": [
+ "ie/8..latest",
+ "firefox/17..latest",
+ "firefox/nightly",
+ "chrome/22..latest",
+ "chrome/canary",
+ "opera/12..latest",
+ "opera/next",
+ "safari/5.1..latest",
+ "ipad/6.0..latest",
+ "iphone/6.0..latest",
+ "android-browser/4.2..latest"
+ ]
+ },
+ "version": "1.0.0"
+}
diff --git a/node_modules/bl/node_modules/process-nextick-args/.travis.yml b/node_modules/bl/node_modules/process-nextick-args/.travis.yml
new file mode 100644
index 0000000..36201b1
--- /dev/null
+++ b/node_modules/bl/node_modules/process-nextick-args/.travis.yml
@@ -0,0 +1,12 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
+ - "0.11"
+ - "0.12"
+ - "1.7.1"
+ - 1
+ - 2
+ - 3
+ - 4
+ - 5
diff --git a/node_modules/bl/node_modules/process-nextick-args/index.js b/node_modules/bl/node_modules/process-nextick-args/index.js
new file mode 100644
index 0000000..a4f40f8
--- /dev/null
+++ b/node_modules/bl/node_modules/process-nextick-args/index.js
@@ -0,0 +1,43 @@
+'use strict';
+
+if (!process.version ||
+ process.version.indexOf('v0.') === 0 ||
+ process.version.indexOf('v1.') === 0 && process.version.indexOf('v1.8.') !== 0) {
+ module.exports = nextTick;
+} else {
+ module.exports = process.nextTick;
+}
+
+function nextTick(fn, arg1, arg2, arg3) {
+ if (typeof fn !== 'function') {
+ throw new TypeError('"callback" argument must be a function');
+ }
+ var len = arguments.length;
+ var args, i;
+ switch (len) {
+ case 0:
+ case 1:
+ return process.nextTick(fn);
+ case 2:
+ return process.nextTick(function afterTickOne() {
+ fn.call(null, arg1);
+ });
+ case 3:
+ return process.nextTick(function afterTickTwo() {
+ fn.call(null, arg1, arg2);
+ });
+ case 4:
+ return process.nextTick(function afterTickThree() {
+ fn.call(null, arg1, arg2, arg3);
+ });
+ default:
+ args = new Array(len - 1);
+ i = 0;
+ while (i < args.length) {
+ args[i++] = arguments[i];
+ }
+ return process.nextTick(function afterTick() {
+ fn.apply(null, args);
+ });
+ }
+}
diff --git a/node_modules/bl/node_modules/process-nextick-args/license.md b/node_modules/bl/node_modules/process-nextick-args/license.md
new file mode 100644
index 0000000..c67e353
--- /dev/null
+++ b/node_modules/bl/node_modules/process-nextick-args/license.md
@@ -0,0 +1,19 @@
+# Copyright (c) 2015 Calvin Metcalf
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+**THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.**
diff --git a/node_modules/bl/node_modules/process-nextick-args/package.json b/node_modules/bl/node_modules/process-nextick-args/package.json
new file mode 100644
index 0000000..ecaf610
--- /dev/null
+++ b/node_modules/bl/node_modules/process-nextick-args/package.json
@@ -0,0 +1,47 @@
+{
+ "_from": "process-nextick-args@~1.0.6",
+ "_id": "process-nextick-args@1.0.7",
+ "_inBundle": false,
+ "_integrity": "sha1-FQ4gt1ZZCtP5EJPyWk8q2L/zC6M=",
+ "_location": "/bl/process-nextick-args",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "process-nextick-args@~1.0.6",
+ "name": "process-nextick-args",
+ "escapedName": "process-nextick-args",
+ "rawSpec": "~1.0.6",
+ "saveSpec": null,
+ "fetchSpec": "~1.0.6"
+ },
+ "_requiredBy": [
+ "/bl/readable-stream"
+ ],
+ "_resolved": "https://registry.npmjs.org/process-nextick-args/-/process-nextick-args-1.0.7.tgz",
+ "_shasum": "150e20b756590ad3f91093f25a4f2ad8bff30ba3",
+ "_spec": "process-nextick-args@~1.0.6",
+ "_where": "C:\\Users\\matth\\Documents\\GitHub\\Moonglow\\node_modules\\bl\\node_modules\\readable-stream",
+ "author": "",
+ "bugs": {
+ "url": "https://github.com/calvinmetcalf/process-nextick-args/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "process.nextTick but always with args",
+ "devDependencies": {
+ "tap": "~0.2.6"
+ },
+ "homepage": "https://github.com/calvinmetcalf/process-nextick-args",
+ "license": "MIT",
+ "main": "index.js",
+ "name": "process-nextick-args",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/calvinmetcalf/process-nextick-args.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.7"
+}
diff --git a/node_modules/bl/node_modules/process-nextick-args/readme.md b/node_modules/bl/node_modules/process-nextick-args/readme.md
new file mode 100644
index 0000000..78e7cfa
--- /dev/null
+++ b/node_modules/bl/node_modules/process-nextick-args/readme.md
@@ -0,0 +1,18 @@
+process-nextick-args
+=====
+
+[](https://travis-ci.org/calvinmetcalf/process-nextick-args)
+
+```bash
+npm install --save process-nextick-args
+```
+
+Always be able to pass arguments to process.nextTick, no matter the platform
+
+```js
+var nextTick = require('process-nextick-args');
+
+nextTick(function (a, b, c) {
+ console.log(a, b, c);
+}, 'step', 3, 'profit');
+```
diff --git a/node_modules/bl/node_modules/readable-stream/.npmignore b/node_modules/bl/node_modules/readable-stream/.npmignore
new file mode 100644
index 0000000..38344f8
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/.npmignore
@@ -0,0 +1,5 @@
+build/
+test/
+examples/
+fs.js
+zlib.js
\ No newline at end of file
diff --git a/node_modules/bl/node_modules/readable-stream/.travis.yml b/node_modules/bl/node_modules/readable-stream/.travis.yml
new file mode 100644
index 0000000..1b82118
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/.travis.yml
@@ -0,0 +1,52 @@
+sudo: false
+language: node_js
+before_install:
+ - npm install -g npm@2
+ - npm install -g npm
+notifications:
+ email: false
+matrix:
+ fast_finish: true
+ allow_failures:
+ - env: TASK=browser BROWSER_NAME=ipad BROWSER_VERSION="6.0..latest"
+ - env: TASK=browser BROWSER_NAME=iphone BROWSER_VERSION="6.0..latest"
+ include:
+ - node_js: '0.8'
+ env: TASK=test
+ - node_js: '0.10'
+ env: TASK=test
+ - node_js: '0.11'
+ env: TASK=test
+ - node_js: '0.12'
+ env: TASK=test
+ - node_js: 1
+ env: TASK=test
+ - node_js: 2
+ env: TASK=test
+ - node_js: 3
+ env: TASK=test
+ - node_js: 4
+ env: TASK=test
+ - node_js: 5
+ env: TASK=test
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=android BROWSER_VERSION="4.0..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=ie BROWSER_VERSION="9..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=opera BROWSER_VERSION="11..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=chrome BROWSER_VERSION="-3..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=firefox BROWSER_VERSION="-3..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=ipad BROWSER_VERSION="6.0..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=iphone BROWSER_VERSION="6.0..latest"
+ - node_js: 5
+ env: TASK=browser BROWSER_NAME=safari BROWSER_VERSION="5..latest"
+script: "npm run $TASK"
+env:
+ global:
+ - secure: rE2Vvo7vnjabYNULNyLFxOyt98BoJexDqsiOnfiD6kLYYsiQGfr/sbZkPMOFm9qfQG7pjqx+zZWZjGSswhTt+626C0t/njXqug7Yps4c3dFblzGfreQHp7wNX5TFsvrxd6dAowVasMp61sJcRnB2w8cUzoe3RAYUDHyiHktwqMc=
+ - secure: g9YINaKAdMatsJ28G9jCGbSaguXCyxSTy+pBO6Ch0Cf57ZLOTka3HqDj8p3nV28LUIHZ3ut5WO43CeYKwt4AUtLpBS3a0dndHdY6D83uY6b2qh5hXlrcbeQTq2cvw2y95F7hm4D1kwrgZ7ViqaKggRcEupAL69YbJnxeUDKWEdI=
diff --git a/node_modules/bl/node_modules/readable-stream/.zuul.yml b/node_modules/bl/node_modules/readable-stream/.zuul.yml
new file mode 100644
index 0000000..96d9cfb
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/.zuul.yml
@@ -0,0 +1 @@
+ui: tape
diff --git a/node_modules/bl/node_modules/readable-stream/LICENSE b/node_modules/bl/node_modules/readable-stream/LICENSE
new file mode 100644
index 0000000..e3d4e69
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/LICENSE
@@ -0,0 +1,18 @@
+Copyright Joyent, Inc. and other Node contributors. All rights reserved.
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to
+deal in the Software without restriction, including without limitation the
+rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
+sell copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
+IN THE SOFTWARE.
diff --git a/node_modules/bl/node_modules/readable-stream/README.md b/node_modules/bl/node_modules/readable-stream/README.md
new file mode 100644
index 0000000..86b95a3
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/README.md
@@ -0,0 +1,36 @@
+# readable-stream
+
+***Node-core v5.8.0 streams for userland*** [](https://travis-ci.org/nodejs/readable-stream)
+
+
+[](https://nodei.co/npm/readable-stream/)
+[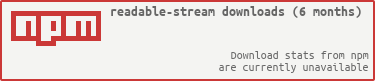](https://nodei.co/npm/readable-stream/)
+
+
+[](https://saucelabs.com/u/readable-stream)
+
+```bash
+npm install --save readable-stream
+```
+
+***Node-core streams for userland***
+
+This package is a mirror of the Streams2 and Streams3 implementations in
+Node-core, including [documentation](doc/stream.markdown).
+
+If you want to guarantee a stable streams base, regardless of what version of
+Node you, or the users of your libraries are using, use **readable-stream** *only* and avoid the *"stream"* module in Node-core, for background see [this blogpost](http://r.va.gg/2014/06/why-i-dont-use-nodes-core-stream-module.html).
+
+As of version 2.0.0 **readable-stream** uses semantic versioning.
+
+# Streams WG Team Members
+
+* **Chris Dickinson** ([@chrisdickinson](https://github.com/chrisdickinson)) <christopher.s.dickinson@gmail.com>
+ - Release GPG key: 9554F04D7259F04124DE6B476D5A82AC7E37093B
+* **Calvin Metcalf** ([@calvinmetcalf](https://github.com/calvinmetcalf)) <calvin.metcalf@gmail.com>
+ - Release GPG key: F3EF5F62A87FC27A22E643F714CE4FF5015AA242
+* **Rod Vagg** ([@rvagg](https://github.com/rvagg)) <rod@vagg.org>
+ - Release GPG key: DD8F2338BAE7501E3DD5AC78C273792F7D83545D
+* **Sam Newman** ([@sonewman](https://github.com/sonewman)) <newmansam@outlook.com>
+* **Mathias Buus** ([@mafintosh](https://github.com/mafintosh)) <mathiasbuus@gmail.com>
+* **Domenic Denicola** ([@domenic](https://github.com/domenic)) <d@domenic.me>
diff --git a/node_modules/bl/node_modules/readable-stream/doc/stream.markdown b/node_modules/bl/node_modules/readable-stream/doc/stream.markdown
new file mode 100644
index 0000000..0bc3819
--- /dev/null
+++ b/node_modules/bl/node_modules/readable-stream/doc/stream.markdown
@@ -0,0 +1,1760 @@
+# Stream
+
+ Stability: 2 - Stable
+
+A stream is an abstract interface implemented by various objects in
+Node.js. For example a [request to an HTTP server][http-incoming-message] is a
+stream, as is [`process.stdout`][]. Streams are readable, writable, or both. All
+streams are instances of [`EventEmitter`][].
+
+You can load the Stream base classes by doing `require('stream')`.
+There are base classes provided for [Readable][] streams, [Writable][]
+streams, [Duplex][] streams, and [Transform][] streams.
+
+This document is split up into 3 sections:
+
+1. The first section explains the parts of the API that you need to be
+ aware of to use streams in your programs.
+2. The second section explains the parts of the API that you need to
+ use if you implement your own custom streams yourself. The API is designed to
+ make this easy for you to do.
+3. The third section goes into more depth about how streams work,
+ including some of the internal mechanisms and functions that you
+ should probably not modify unless you definitely know what you are
+ doing.
+
+
+## API for Stream Consumers
+
+
+
+Streams can be either [Readable][], [Writable][], or both ([Duplex][]).
+
+All streams are EventEmitters, but they also have other custom methods
+and properties depending on whether they are Readable, Writable, or
+Duplex.
+
+If a stream is both Readable and Writable, then it implements all of
+the methods and events. So, a [Duplex][] or [Transform][] stream is
+fully described by this API, though their implementation may be
+somewhat different.
+
+It is not necessary to implement Stream interfaces in order to consume
+streams in your programs. If you **are** implementing streaming
+interfaces in your own program, please also refer to
+[API for Stream Implementors][].
+
+Almost all Node.js programs, no matter how simple, use Streams in some
+way. Here is an example of using Streams in an Node.js program:
+
+```js
+const http = require('http');
+
+var server = http.createServer( (req, res) => {
+ // req is an http.IncomingMessage, which is a Readable Stream
+ // res is an http.ServerResponse, which is a Writable Stream
+
+ var body = '';
+ // we want to get the data as utf8 strings
+ // If you don't set an encoding, then you'll get Buffer objects
+ req.setEncoding('utf8');
+
+ // Readable streams emit 'data' events once a listener is added
+ req.on('data', (chunk) => {
+ body += chunk;
+ });
+
+ // the end event tells you that you have entire body
+ req.on('end', () => {
+ try {
+ var data = JSON.parse(body);
+ } catch (er) {
+ // uh oh! bad json!
+ res.statusCode = 400;
+ return res.end(`error: ${er.message}`);
+ }
+
+ // write back something interesting to the user:
+ res.write(typeof data);
+ res.end();
+ });
+});
+
+server.listen(1337);
+
+// $ curl localhost:1337 -d '{}'
+// object
+// $ curl localhost:1337 -d '"foo"'
+// string
+// $ curl localhost:1337 -d 'not json'
+// error: Unexpected token o
+```
+
+### Class: stream.Duplex
+
+Duplex streams are streams that implement both the [Readable][] and
+[Writable][] interfaces.
+
+Examples of Duplex streams include:
+
+* [TCP sockets][]
+* [zlib streams][zlib]
+* [crypto streams][crypto]
+
+### Class: stream.Readable
+
+
+
+The Readable stream interface is the abstraction for a *source* of
+data that you are reading from. In other words, data comes *out* of a
+Readable stream.
+
+A Readable stream will not start emitting data until you indicate that
+you are ready to receive it.
+
+Readable streams have two "modes": a **flowing mode** and a **paused
+mode**. When in flowing mode, data is read from the underlying system
+and provided to your program as fast as possible. In paused mode, you
+must explicitly call [`stream.read()`][stream-read] to get chunks of data out.
+Streams start out in paused mode.
+
+**Note**: If no data event handlers are attached, and there are no
+[`stream.pipe()`][] destinations, and the stream is switched into flowing
+mode, then data will be lost.
+
+You can switch to flowing mode by doing any of the following:
+
+* Adding a [`'data'`][] event handler to listen for data.
+* Calling the [`stream.resume()`][stream-resume] method to explicitly open the
+ flow.
+* Calling the [`stream.pipe()`][] method to send the data to a [Writable][].
+
+You can switch back to paused mode by doing either of the following:
+
+* If there are no pipe destinations, by calling the
+ [`stream.pause()`][stream-pause] method.
+* If there are pipe destinations, by removing any [`'data'`][] event
+ handlers, and removing all pipe destinations by calling the
+ [`stream.unpipe()`][] method.
+
+Note that, for backwards compatibility reasons, removing [`'data'`][]
+event handlers will **not** automatically pause the stream. Also, if
+there are piped destinations, then calling [`stream.pause()`][stream-pause] will
+not guarantee that the stream will *remain* paused once those
+destinations drain and ask for more data.
+
+Examples of readable streams include:
+
+* [HTTP responses, on the client][http-incoming-message]
+* [HTTP requests, on the server][http-incoming-message]
+* [fs read streams][]
+* [zlib streams][zlib]
+* [crypto streams][crypto]
+* [TCP sockets][]
+* [child process stdout and stderr][]
+* [`process.stdin`][]
+
+#### Event: 'close'
+
+Emitted when the stream and any of its underlying resources (a file
+descriptor, for example) have been closed. The event indicates that
+no more events will be emitted, and no further computation will occur.
+
+Not all streams will emit the `'close'` event.
+
+#### Event: 'data'
+
+* `chunk` {Buffer|String} The chunk of data.
+
+Attaching a `'data'` event listener to a stream that has not been
+explicitly paused will switch the stream into flowing mode. Data will
+then be passed as soon as it is available.
+
+If you just want to get all the data out of the stream as fast as
+possible, this is the best way to do so.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.on('data', (chunk) => {
+ console.log('got %d bytes of data', chunk.length);
+});
+```
+
+#### Event: 'end'
+
+This event fires when there will be no more data to read.
+
+Note that the `'end'` event **will not fire** unless the data is
+completely consumed. This can be done by switching into flowing mode,
+or by calling [`stream.read()`][stream-read] repeatedly until you get to the
+end.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.on('data', (chunk) => {
+ console.log('got %d bytes of data', chunk.length);
+});
+readable.on('end', () => {
+ console.log('there will be no more data.');
+});
+```
+
+#### Event: 'error'
+
+* {Error Object}
+
+Emitted if there was an error receiving data.
+
+#### Event: 'readable'
+
+When a chunk of data can be read from the stream, it will emit a
+`'readable'` event.
+
+In some cases, listening for a `'readable'` event will cause some data
+to be read into the internal buffer from the underlying system, if it
+hadn't already.
+
+```javascript
+var readable = getReadableStreamSomehow();
+readable.on('readable', () => {
+ // there is some data to read now
+});
+```
+
+Once the internal buffer is drained, a `'readable'` event will fire
+again when more data is available.
+
+The `'readable'` event is not emitted in the "flowing" mode with the
+sole exception of the last one, on end-of-stream.
+
+The `'readable'` event indicates that the stream has new information:
+either new data is available or the end of the stream has been reached.
+In the former case, [`stream.read()`][stream-read] will return that data. In the
+latter case, [`stream.read()`][stream-read] will return null. For instance, in
+the following example, `foo.txt` is an empty file:
+
+```js
+const fs = require('fs');
+var rr = fs.createReadStream('foo.txt');
+rr.on('readable', () => {
+ console.log('readable:', rr.read());
+});
+rr.on('end', () => {
+ console.log('end');
+});
+```
+
+The output of running this script is:
+
+```
+$ node test.js
+readable: null
+end
+```
+
+#### readable.isPaused()
+
+* Return: {Boolean}
+
+This method returns whether or not the `readable` has been **explicitly**
+paused by client code (using [`stream.pause()`][stream-pause] without a
+corresponding [`stream.resume()`][stream-resume]).
+
+```js
+var readable = new stream.Readable
+
+readable.isPaused() // === false
+readable.pause()
+readable.isPaused() // === true
+readable.resume()
+readable.isPaused() // === false
+```
+
+#### readable.pause()
+
+* Return: `this`
+
+This method will cause a stream in flowing mode to stop emitting
+[`'data'`][] events, switching out of flowing mode. Any data that becomes
+available will remain in the internal buffer.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.on('data', (chunk) => {
+ console.log('got %d bytes of data', chunk.length);
+ readable.pause();
+ console.log('there will be no more data for 1 second');
+ setTimeout(() => {
+ console.log('now data will start flowing again');
+ readable.resume();
+ }, 1000);
+});
+```
+
+#### readable.pipe(destination[, options])
+
+* `destination` {stream.Writable} The destination for writing data
+* `options` {Object} Pipe options
+ * `end` {Boolean} End the writer when the reader ends. Default = `true`
+
+This method pulls all the data out of a readable stream, and writes it
+to the supplied destination, automatically managing the flow so that
+the destination is not overwhelmed by a fast readable stream.
+
+Multiple destinations can be piped to safely.
+
+```js
+var readable = getReadableStreamSomehow();
+var writable = fs.createWriteStream('file.txt');
+// All the data from readable goes into 'file.txt'
+readable.pipe(writable);
+```
+
+This function returns the destination stream, so you can set up pipe
+chains like so:
+
+```js
+var r = fs.createReadStream('file.txt');
+var z = zlib.createGzip();
+var w = fs.createWriteStream('file.txt.gz');
+r.pipe(z).pipe(w);
+```
+
+For example, emulating the Unix `cat` command:
+
+```js
+process.stdin.pipe(process.stdout);
+```
+
+By default [`stream.end()`][stream-end] is called on the destination when the
+source stream emits [`'end'`][], so that `destination` is no longer writable.
+Pass `{ end: false }` as `options` to keep the destination stream open.
+
+This keeps `writer` open so that "Goodbye" can be written at the
+end.
+
+```js
+reader.pipe(writer, { end: false });
+reader.on('end', () => {
+ writer.end('Goodbye\n');
+});
+```
+
+Note that [`process.stderr`][] and [`process.stdout`][] are never closed until
+the process exits, regardless of the specified options.
+
+#### readable.read([size])
+
+* `size` {Number} Optional argument to specify how much data to read.
+* Return {String|Buffer|Null}
+
+The `read()` method pulls some data out of the internal buffer and
+returns it. If there is no data available, then it will return
+`null`.
+
+If you pass in a `size` argument, then it will return that many
+bytes. If `size` bytes are not available, then it will return `null`,
+unless we've ended, in which case it will return the data remaining
+in the buffer.
+
+If you do not specify a `size` argument, then it will return all the
+data in the internal buffer.
+
+This method should only be called in paused mode. In flowing mode,
+this method is called automatically until the internal buffer is
+drained.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.on('readable', () => {
+ var chunk;
+ while (null !== (chunk = readable.read())) {
+ console.log('got %d bytes of data', chunk.length);
+ }
+});
+```
+
+If this method returns a data chunk, then it will also trigger the
+emission of a [`'data'`][] event.
+
+Note that calling [`stream.read([size])`][stream-read] after the [`'end'`][]
+event has been triggered will return `null`. No runtime error will be raised.
+
+#### readable.resume()
+
+* Return: `this`
+
+This method will cause the readable stream to resume emitting [`'data'`][]
+events.
+
+This method will switch the stream into flowing mode. If you do *not*
+want to consume the data from a stream, but you *do* want to get to
+its [`'end'`][] event, you can call [`stream.resume()`][stream-resume] to open
+the flow of data.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.resume();
+readable.on('end', () => {
+ console.log('got to the end, but did not read anything');
+});
+```
+
+#### readable.setEncoding(encoding)
+
+* `encoding` {String} The encoding to use.
+* Return: `this`
+
+Call this function to cause the stream to return strings of the specified
+encoding instead of Buffer objects. For example, if you do
+`readable.setEncoding('utf8')`, then the output data will be interpreted as
+UTF-8 data, and returned as strings. If you do `readable.setEncoding('hex')`,
+then the data will be encoded in hexadecimal string format.
+
+This properly handles multi-byte characters that would otherwise be
+potentially mangled if you simply pulled the Buffers directly and
+called [`buf.toString(encoding)`][] on them. If you want to read the data
+as strings, always use this method.
+
+Also you can disable any encoding at all with `readable.setEncoding(null)`.
+This approach is very useful if you deal with binary data or with large
+multi-byte strings spread out over multiple chunks.
+
+```js
+var readable = getReadableStreamSomehow();
+readable.setEncoding('utf8');
+readable.on('data', (chunk) => {
+ assert.equal(typeof chunk, 'string');
+ console.log('got %d characters of string data', chunk.length);
+});
+```
+
+#### readable.unpipe([destination])
+
+* `destination` {stream.Writable} Optional specific stream to unpipe
+
+This method will remove the hooks set up for a previous [`stream.pipe()`][]
+call.
+
+If the destination is not specified, then all pipes are removed.
+
+If the destination is specified, but no pipe is set up for it, then
+this is a no-op.
+
+```js
+var readable = getReadableStreamSomehow();
+var writable = fs.createWriteStream('file.txt');
+// All the data from readable goes into 'file.txt',
+// but only for the first second
+readable.pipe(writable);
+setTimeout(() => {
+ console.log('stop writing to file.txt');
+ readable.unpipe(writable);
+ console.log('manually close the file stream');
+ writable.end();
+}, 1000);
+```
+
+#### readable.unshift(chunk)
+
+* `chunk` {Buffer|String} Chunk of data to unshift onto the read queue
+
+This is useful in certain cases where a stream is being consumed by a
+parser, which needs to "un-consume" some data that it has
+optimistically pulled out of the source, so that the stream can be
+passed on to some other party.
+
+Note that `stream.unshift(chunk)` cannot be called after the [`'end'`][] event
+has been triggered; a runtime error will be raised.
+
+If you find that you must often call `stream.unshift(chunk)` in your
+programs, consider implementing a [Transform][] stream instead. (See [API
+for Stream Implementors][].)
+
+```js
+// Pull off a header delimited by \n\n
+// use unshift() if we get too much
+// Call the callback with (error, header, stream)
+const StringDecoder = require('string_decoder').StringDecoder;
+function parseHeader(stream, callback) {
+ stream.on('error', callback);
+ stream.on('readable', onReadable);
+ var decoder = new StringDecoder('utf8');
+ var header = '';
+ function onReadable() {
+ var chunk;
+ while (null !== (chunk = stream.read())) {
+ var str = decoder.write(chunk);
+ if (str.match(/\n\n/)) {
+ // found the header boundary
+ var split = str.split(/\n\n/);
+ header += split.shift();
+ var remaining = split.join('\n\n');
+ var buf = new Buffer(remaining, 'utf8');
+ if (buf.length)
+ stream.unshift(buf);
+ stream.removeListener('error', callback);
+ stream.removeListener('readable', onReadable);
+ // now the body of the message can be read from the stream.
+ callback(null, header, stream);
+ } else {
+ // still reading the header.
+ header += str;
+ }
+ }
+ }
+}
+```
+
+Note that, unlike [`stream.push(chunk)`][stream-push], `stream.unshift(chunk)`
+will not end the reading process by resetting the internal reading state of the
+stream. This can cause unexpected results if `unshift()` is called during a
+read (i.e. from within a [`stream._read()`][stream-_read] implementation on a
+custom stream). Following the call to `unshift()` with an immediate
+[`stream.push('')`][stream-push] will reset the reading state appropriately,
+however it is best to simply avoid calling `unshift()` while in the process of
+performing a read.
+
+#### readable.wrap(stream)
+
+* `stream` {Stream} An "old style" readable stream
+
+Versions of Node.js prior to v0.10 had streams that did not implement the
+entire Streams API as it is today. (See [Compatibility][] for
+more information.)
+
+If you are using an older Node.js library that emits [`'data'`][] events and
+has a [`stream.pause()`][stream-pause] method that is advisory only, then you
+can use the `wrap()` method to create a [Readable][] stream that uses the old
+stream as its data source.
+
+You will very rarely ever need to call this function, but it exists
+as a convenience for interacting with old Node.js programs and libraries.
+
+For example:
+
+```js
+const OldReader = require('./old-api-module.js').OldReader;
+const Readable = require('stream').Readable;
+const oreader = new OldReader;
+const myReader = new Readable().wrap(oreader);
+
+myReader.on('readable', () => {
+ myReader.read(); // etc.
+});
+```
+
+### Class: stream.Transform
+
+Transform streams are [Duplex][] streams where the output is in some way
+computed from the input. They implement both the [Readable][] and
+[Writable][] interfaces.
+
+Examples of Transform streams include:
+
+* [zlib streams][zlib]
+* [crypto streams][crypto]
+
+### Class: stream.Writable
+
+
+
+The Writable stream interface is an abstraction for a *destination*
+that you are writing data *to*.
+
+Examples of writable streams include:
+
+* [HTTP requests, on the client][]
+* [HTTP responses, on the server][]
+* [fs write streams][]
+* [zlib streams][zlib]
+* [crypto streams][crypto]
+* [TCP sockets][]
+* [child process stdin][]
+* [`process.stdout`][], [`process.stderr`][]
+
+#### Event: 'drain'
+
+If a [`stream.write(chunk)`][stream-write] call returns `false`, then the
+`'drain'` event will indicate when it is appropriate to begin writing more data
+to the stream.
+
+```js
+// Write the data to the supplied writable stream one million times.
+// Be attentive to back-pressure.
+function writeOneMillionTimes(writer, data, encoding, callback) {
+ var i = 1000000;
+ write();
+ function write() {
+ var ok = true;
+ do {
+ i -= 1;
+ if (i === 0) {
+ // last time!
+ writer.write(data, encoding, callback);
+ } else {
+ // see if we should continue, or wait
+ // don't pass the callback, because we're not done yet.
+ ok = writer.write(data, encoding);
+ }
+ } while (i > 0 && ok);
+ if (i > 0) {
+ // had to stop early!
+ // write some more once it drains
+ writer.once('drain', write);
+ }
+ }
+}
+```
+
+#### Event: 'error'
+
+* {Error}
+
+Emitted if there was an error when writing or piping data.
+
+#### Event: 'finish'
+
+When the [`stream.end()`][stream-end] method has been called, and all data has
+been flushed to the underlying system, this event is emitted.
+
+```javascript
+var writer = getWritableStreamSomehow();
+for (var i = 0; i < 100; i ++) {
+ writer.write('hello, #${i}!\n');
+}
+writer.end('this is the end\n');
+writer.on('finish', () => {
+ console.error('all writes are now complete.');
+});
+```
+
+#### Event: 'pipe'
+
+* `src` {stream.Readable} source stream that is piping to this writable
+
+This is emitted whenever the [`stream.pipe()`][] method is called on a readable
+stream, adding this writable to its set of destinations.
+
+```js
+var writer = getWritableStreamSomehow();
+var reader = getReadableStreamSomehow();
+writer.on('pipe', (src) => {
+ console.error('something is piping into the writer');
+ assert.equal(src, reader);
+});
+reader.pipe(writer);
+```
+
+#### Event: 'unpipe'
+
+* `src` {[Readable][] Stream} The source stream that
+ [unpiped][`stream.unpipe()`] this writable
+
+This is emitted whenever the [`stream.unpipe()`][] method is called on a
+readable stream, removing this writable from its set of destinations.
+
+```js
+var writer = getWritableStreamSomehow();
+var reader = getReadableStreamSomehow();
+writer.on('unpipe', (src) => {
+ console.error('something has stopped piping into the writer');
+ assert.equal(src, reader);
+});
+reader.pipe(writer);
+reader.unpipe(writer);
+```
+
+#### writable.cork()
+
+Forces buffering of all writes.
+
+Buffered data will be flushed either at [`stream.uncork()`][] or at
+[`stream.end()`][stream-end] call.
+
+#### writable.end([chunk][, encoding][, callback])
+
+* `chunk` {String|Buffer} Optional data to write
+* `encoding` {String} The encoding, if `chunk` is a String
+* `callback` {Function} Optional callback for when the stream is finished
+
+Call this method when no more data will be written to the stream. If supplied,
+the callback is attached as a listener on the [`'finish'`][] event.
+
+Calling [`stream.write()`][stream-write] after calling
+[`stream.end()`][stream-end] will raise an error.
+
+```js
+// write 'hello, ' and then end with 'world!'
+var file = fs.createWriteStream('example.txt');
+file.write('hello, ');
+file.end('world!');
+// writing more now is not allowed!
+```
+
+#### writable.setDefaultEncoding(encoding)
+
+* `encoding` {String} The new default encoding
+
+Sets the default encoding for a writable stream.
+
+#### writable.uncork()
+
+Flush all data, buffered since [`stream.cork()`][] call.
+
+#### writable.write(chunk[, encoding][, callback])
+
+* `chunk` {String|Buffer} The data to write
+* `encoding` {String} The encoding, if `chunk` is a String
+* `callback` {Function} Callback for when this chunk of data is flushed
+* Returns: {Boolean} `true` if the data was handled completely.
+
+This method writes some data to the underlying system, and calls the
+supplied callback once the data has been fully handled.
+
+The return value indicates if you should continue writing right now.
+If the data had to be buffered internally, then it will return
+`false`. Otherwise, it will return `true`.
+
+This return value is strictly advisory. You MAY continue to write,
+even if it returns `false`. However, writes will be buffered in
+memory, so it is best not to do this excessively. Instead, wait for
+the [`'drain'`][] event before writing more data.
+
+
+## API for Stream Implementors
+
+
+
+To implement any sort of stream, the pattern is the same:
+
+1. Extend the appropriate parent class in your own subclass. (The
+ [`util.inherits()`][] method is particularly helpful for this.)
+2. Call the appropriate parent class constructor in your constructor,
+ to be sure that the internal mechanisms are set up properly.
+3. Implement one or more specific methods, as detailed below.
+
+The class to extend and the method(s) to implement depend on the sort
+of stream class you are writing:
+
+
+ Use-case + |
+
+ Class + |
+
+ Method(s) to implement + |
+
---|---|---|
+ Reading only + |
+
+ [Readable](#stream_class_stream_readable_1) + |
+
+
|
+
+ Writing only + |
+
+ [Writable](#stream_class_stream_writable_1) + |
+
+
|
+
+ Reading and writing + |
+
+ [Duplex](#stream_class_stream_duplex_1) + |
+
+
|
+
+ Operate on written data, then read the result + |
+
+ [Transform](#stream_class_stream_transform_1) + |
+
+
|
+