diff --git a/script/translateToEn.js b/script/translateToEn.js
new file mode 100644
index 00000000000..3379f005d6b
--- /dev/null
+++ b/script/translateToEn.js
@@ -0,0 +1,198 @@
+import fs from "fs/promises";
+import path from "path";
+import { fileURLToPath } from 'url';
+import { config } from "dotenv";
+import { request } from "./util.js";
+
+const __filename = fileURLToPath(import.meta.url);
+const __dirname = path.dirname(__filename);
+const envPath = path.resolve(__dirname, '../.env');
+config({ path: envPath });
+
+const isTranslate = false;
+
+const askAI = async (content) => {
+ const url = "https://dashscope.aliyuncs.com/api/v1/services/aigc/text-generation/generation"
+ const header = {
+ "Content-Type": "application/json",
+ "Authorization": "Bearer "+ process.env.QWEN_TOKEN
+ }
+ const body = {
+ "model": "qwen-max",
+ "input":{
+ "messages": [
+ {
+ "role": "system",
+ "content": "你是SEO领域的专家,擅长总结文章并输出有利于SEO的关键词。你还是一个翻译专家,擅长以开源微服务为技术背景做中/英文之间的翻译,生成与中文文档格式完全一致的英文翻译稿"
+ },
+ {
+ "role": "user",
+ "content": `请阅读以下markdown格式的文章,并严格按照下面的格式输出回答,方便后续进行正则匹配和内容截取(关键词、描述信息、翻译后的英文文章},这些小标题一个字都不允许变),同时请注意关键词与描述信息需要为中文内容:
+
+ 1. 关键词: "总结的中文关键词,要求格式为数组,多个关键词用英文逗号分隔,不超过5个",
+ 2. 描述信息: "总结的内容,语言为中文,大概在150-160字之间,不要超过200字"
+ 3. 翻译后的英文文章:"根据原文翻译之后的英文文章。需要与我给你的格式完全相同,即需要有front-matter。不需要为markdown格式,为字符串格式即可"
+ 请根据以下原文进行生成返回内容:
+ ${content}
+ `
+ }
+ ]
+
+ },
+ "parameters": {
+ "result_format": "message"
+ }
+ }
+ try {
+ let res = await request(url, "POST", header, body);
+ return res.output.choices[0].message.content
+ } catch (error) {
+ throw(error)
+ }
+
+}
+
+async function traverseDirectorySync (dir) {
+ try {
+ const files = await fs.readdir(dir,{ withFileTypes: true })
+ // console.log("-======",files)
+ for (const file of files) {
+ const filePath = path.join(dir, file.name);
+ if (file.isDirectory()) {
+ // 如果是文件夹,输出文件夹路径并递归遍历
+ console.log(`目录:${filePath}`);
+ traverseDirectorySync(filePath);
+ } else if (file.name.endsWith('.md') || file.name.endsWith('.mdx')) {
+ const data = await fs.readFile(filePath, 'utf8');
+ let res = await askAI(data);
+ console.log(file.name, "回答问题完成");
+ console.log("======",res)
+ const { keywords, description, content } = regContent(res);
+
+ // 替换中文文档内容
+ await replaceZhContent( filePath, data, keywords, description );
+ // 生成英文翻译文件
+ isTranslate && await generateEnFile(filePath, content)
+ }
+ }
+ } catch (err) {
+ console.error(err);
+ }
+}
+
+function regContent(res) {
+ const keywordsRegex = /关键词:\s*"([^"]*)"/;
+ const regDescrip = /描述信息:\s*"([^"]*)"/;
+ const regContent = /翻译后的英文文章:\s*"([\s\S]*)"/;
+
+ const keywordsMatch = res.match(keywordsRegex);
+ const keywords = keywordsMatch ? keywordsMatch[1].trim() : '';
+
+ const descripMatch = res.match(regDescrip);
+ const description = descripMatch ? descripMatch[1].trim() : '';
+
+ const contentMatch = res.match(regContent);
+ const content = contentMatch ? contentMatch[1].trim() : '';
+ return { keywords, description, content };
+}
+// const filename = "activity-preview-nacos.md"
+// fs.readFile(path.resolve(__dirname, '../src/content/blog/activity-preview-nacos.md'), 'utf8')
+// .then(async data => {
+// // let res = await askAI(data);
+// // console.log(res)
+// const keywordsRegex = /关键词:\s*"([^"]*)"/;
+// const regDescrip = /描述信息:\s*"([^"]*)"/;
+// const regContent = /翻译后的英文文章:\s*"([\s\S]*)"/;
+
+// // const keywordsMatch = res.match(keywordsRegex);
+// // const keywords = keywordsMatch ? keywordsMatch[1].trim() : '';
+// // console.log("关键词:", keywords);
+
+// // const descripMatch = res.match(regDescrip);
+// // const description = descripMatch ? descripMatch[1].trim() : '';
+// // console.log("描述信息:", description);
+
+// // const contentMatch = res.match(regContent);
+// // const content = contentMatch ? contentMatch[1].trim() : '';
+// // console.log("翻译后的英文文章:\n", content);
+
+// const keywords = "Nacos 0.1.0, Review活动, 社区交流, GitHub issue, 贡献流程, 小礼品奖励";
+// const description = "文章概述了Nacos 0.1.0版本的Review活动设计,包括任务列表如官网与文档审查、功能测试、提供建议等,参与方式涉及加入社区微信群与提交GitHub issue,奖励机制鼓励突出贡献者,并强调沟通透明与建议采纳原则。";
+// const content = `
+// ---
+// title: Nacos 0.1.0 Version Review Event Design
+// keywords: [Nacos 0.1.0, Review event, community engagement, GitHub issue, contribution process, reward]
+// description: This article outlines the design of the Nacos 0.1.0 version review event, featuring tasks such as reviewing official website and documentation, testing functionalities, and providing suggestions. It details participation methods including joining the community WeChat group and submitting GitHub issues, with a reward mechanism to incentivize notable contributors, emphasizing transparent communication and the principle of suggestion adoption.
+// date: \"2018-11-15\"
+// category: activity-preview
+// ---
+
+// # Nacos 0.1.0 Version Review Event Design
+
+// ## I. Activity Task List
+
+// - Review official content, identify bugs, and suggest improvements for both Chinese and English websites.
+// - Read through the Chinese and English documentation, find errors, and propose enhancements, especially focusing on poor English translations.
+// - Test the code compilation, Nacos server startup/shutdown流程, and suggest improvements.
+// - Attempt to configure and launch multi-node Nacos cluster mode, offering improvement advice.
+// - Utilize the Nacos Java SDK and provide feedback for enhancements.
+// - Try the Nacos Open API and suggest improvements.
+// - Follow the 'How to Contribute to Nacos Documentation TODO' guide, test the contribution process, and give feedback.
+// - Submit requirements, development plans, ideas, and requests for Nacos.
+
+// ## II. Participation Methods
+
+// - Scan the WeChat QR code of 'Super Brother' to join the 'Nacos Community Chat Group'.
+// - Choose one or more tasks from Section I.
+// - Report issues or bugs found following the 'Issue Reporting Process' in Section III, assigning them to @github user[xuechaos](https://github.com/xuechaos).
+
+// ## III. Issue Reporting Process
+
+// * Process details TODO
+
+// ## IV. Reward Mechanism
+
+// * Customized small gifts are being prepared for contributors who make outstanding contributions, with consideration for shipping to those who stand out during the process.
+// * The gifts, though modest, aim to express gratitude for your assistance.
+
+// ## V. Additional Notes
+
+// * Not every suggestion will necessarily be adopted, but we will endeavor to communicate our considerations if your suggestion is not implemented.
+// * Prefer using email lists or reporting issues over reporting problems in the WeChat group to document and facilitate our communication process.`
+// replaceZhContent(path.resolve(__dirname, '../src/content/blog/activity-preview-nacos.md'),data, keywords, description);
+// // generateEnFile(filename, content )
+// })
+
+const replaceZhContent = async (filePath, data, keywords, description) => {
+ const keywordsRegex = /keywords: \[\s*([^"]*)\]\n/s;
+ const regDescrip = /description:\s*([^\n]*)\n/;
+ const oriKeywordMatch = data.match(keywordsRegex);
+ const originKeywords = oriKeywordMatch ? oriKeywordMatch[0].trim() : '';
+ const oriDescripMatch = data.match(regDescrip);
+ const originDescrip = oriDescripMatch ? oriDescripMatch[0].trim() : '';
+
+ const newData = data.replace(originKeywords,`keywords: [${keywords}]`).replace(originDescrip, `description: ${description}`);
+
+ try {
+ await fs.writeFile(filePath, newData, 'utf8');
+ console.log(`${filePath} 已被更新完成`);
+ } catch (err) {
+ console.error(err);
+ }
+}
+
+const generateEnFile = async (filename, content) => {
+ const enFilename = filename.replace('zh-cn', 'en');
+ try {
+ await fs.writeFile(enFilename, content, 'utf8');
+ console.log(`${enFilename} 已被生成`);
+ } catch (err) {
+ console.log("err",err)
+ }
+}
+
+await traverseDirectorySync(path.resolve(__dirname, '../src/content/docs/ebook/zh-cn'))
+
+
+
+
diff --git a/script/util.js b/script/util.js
new file mode 100644
index 00000000000..ed96d0eb38c
--- /dev/null
+++ b/script/util.js
@@ -0,0 +1,10 @@
+export const request = async (url, method = "GET", headers = {}, body = {}) => {
+ const res = await fetch(url, { headers, method, body: JSON.stringify(body) });
+ if (!res.ok) throw new Error(res.statusText);
+ const data = await res.json();
+ if (data.error) {
+ throw new Error(data.error)
+ } else {
+ return data;
+ }
+};
\ No newline at end of file
diff --git a/src/content/blog/faq/NACOS-user-question-history15791.md b/src/content/blog/faq/NACOS-user-question-history15791.md
new file mode 100644
index 00000000000..19c74c18925
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15791.md
@@ -0,0 +1,61 @@
+---
+id: "question-history-15791"
+title: "nacos配置中心原理 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "Nacos配置中心的工作原理主要基于以下几个核心步骤,结合您提供的知识内容,我们可以深入解析这一过程:### 1. **服务启动与配置初始化**- **添加依赖**:在Spring Boot项目中,通过添加`nacos-config-spring-boot-starter`依赖,使得应用能够与Nac"
+---
+
+## 用户问题 :
+ nacos配置中心原理 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+Nacos配置中心的工作原理主要基于以下几个核心步骤,结合您提供的知识内容,我们可以深入解析这一过程:
+
+### 1. **服务启动与配置初始化**
+
+- **添加依赖**:在Spring Boot项目中,通过添加`nacos-config-spring-boot-starter`依赖,使得应用能够与Nacos配置服务集成。
+- **配置Nacos地址**:在`application.properties`或`application.yml`中指定Nacos服务器的地址,确保应用能连接到Nacos服务器。
+- **加载配置源**:使用`@NacosPropertySource`注解,可以在应用启动时从Nacos服务器加载指定的配置文件(dataId)。此步骤实现了配置的初始加载。
+
+### 2. **动态配置更新**
+
+- **自动刷新**:通过在`@NacosPropertySource`注解中设置`autoRefreshed=true`,可以使应用在Nacos中的配置发生变化时自动刷新这些配置。这依赖于Nacos客户端监听Nacos服务器上的配置变更事件。
+- **属性注入**:使用`@NacosValue`注解,可以将Nacos中的配置值直接注入到Bean的字段中,并支持自动刷新。这意味着应用运行时的配置可以做到实时更新而无需重启服务。
+
+### 3. **服务注册与发现**
+
+- **添加依赖**:通过引入`nacos-discovery-spring-boot-starter`,使应用具备服务注册与发现的能力。
+- **配置发现服务器地址**:同样在`application.properties`中设置Nacos发现服务的地址。
+- **服务实例注册**:应用启动时,会自动向Nacos服务器注册自身信息(如IP、端口等),使得其他服务可以通过Nacos发现并调用它。
+- **服务发现**:应用可以通过Nacos提供的API查询注册在Nacos上的其他服务实例,实现服务间的调用和负载均衡。
+
+### 原理解释
+
+Nacos配置中心的核心在于其提供了一个集中式的配置管理和服务发现平台。应用通过Spring Boot Starter与Nacos集成后,能够在启动时自动从Nacos服务器获取配置信息,并在配置变更时自动更新,保证了配置的一致性和实时性。同时,Nacos的服务发现机制允许微服务在启动时注册自身信息,并能发现其他服务实例,为微服务架构中的服务调用提供了基础。
+
+### 结论
+
+综上所述,Nacos配置中心原理涉及到配置的初始化加载、动态更新以及服务的注册与发现,通过Spring Boot与Nacos的紧密集成,极大地简化了分布式系统中的配置管理和服务治理工作。如果您有更细节的技术疑问或实践操作上的问题,欢迎继续提问。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 融合 Spring Boot,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring-boot)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15801)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15792.md b/src/content/blog/faq/NACOS-user-question-history15792.md
new file mode 100644
index 00000000000..75dad6c715c
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15792.md
@@ -0,0 +1,52 @@
+---
+id: "question-history-15792"
+title: "如何快速搭建nacos配置中心? nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "要快速搭建 Nacos 配置中心,请按照以下步骤操作:1. **下载并启动 Nacos Server**: 首先,您需要从 Nacos 的官方 GitHub 仓库下载 Nacos 并启动 Nacos Server。这一步是使用 Nacos 的前提条件。具体操作步骤可以参考 Nacos 官方文档的"
+---
+
+## 用户问题 :
+ 如何快速搭建nacos配置中心? nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+要快速搭建 Nacos 配置中心,请按照以下步骤操作:
+
+1. **下载并启动 Nacos Server**:
+ 首先,您需要从 Nacos 的官方 GitHub 仓库下载 Nacos 并启动 Nacos Server。这一步是使用 Nacos 的前提条件。具体操作步骤可以参考 Nacos 官方文档的[快速入门](../quickstart/quick-start.md)部分。这个环节涉及到解压下载的 Nacos 压缩包,然后通过命令行启动 Nacos 服务器。
+
+2. **配置 Nacos Server**:
+ 在启动 Nacos Server 时,您可能需要根据实际情况调整一些配置项,比如端口号、数据存储方式等。这些配置通常在 Nacos 的 `conf` 目录下的 `application.properties` 文件中完成。
+
+3. **在 Spring 应用中集成 Nacos 配置管理**:
+ - **添加依赖**: 在您的 Spring 项目的 `pom.xml` 文件中添加 Nacos Spring Context 的依赖。确保使用的版本是最新的,您可以在 [mvnrepository.com](https://mvnrepository.com/artifact/com.alibaba.nacos/nacos-spring-context) 查找最新版本号。
+ - **配置启用**: 在 Spring 配置类上添加 `@EnableNacosConfig` 注解以启用 Nacos 配置管理,并通过 `@NacosPropertySource` 加载特定的配置文件,例如 `dataId` 为 `example` 的配置源。同时,设置 `globalProperties` 来指定 Nacos Server 的地址,如 `"127.0.0.1:8848"`。
+ - **使用注解读取配置**: 在需要使用配置的类或方法上,使用 `@NacosValue` 注解读取配置值,支持自动刷新配置。
+
+4. **验证配置管理功能**:
+ - 启动您的 Spring Boot 应用后,尝试通过 REST API 访问应用中受 Nacos 管理的配置项,验证配置是否生效以及是否能动态更新。比如,访问 `http://localhost:8080/config/get` 查看配置值。
+ - 动态修改 Nacos Server 上的配置,并观察 Spring 应用是否能实时获取到最新的配置信息。可以通过 Nacos 提供的 Open API 发布配置,例如更新 `example` 配置的 `useLocalCache` 值。
+
+以上步骤完成后,您就成功搭建并验证了一个基本的 Nacos 配置中心环境,可以开始在分布式系统中利用 Nacos 进行配置管理了。请确保每一步操作都符合您的实际开发和部署环境需求。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 融合 Spring,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15802)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15793.md b/src/content/blog/faq/NACOS-user-question-history15793.md
new file mode 100644
index 00000000000..c8d990a3cbe
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15793.md
@@ -0,0 +1,58 @@
+---
+id: "question-history-15793"
+title: "nacos配置中心密码加密 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "为了关于Nacos配置中心密码加密的问题,我们首先需要理解问题的具体背景和涉及的场景。根据提供的知识,我们可以分析如下:### 问题原因分析Nacos作为一款流行的配置管理平台,用户通常会面临敏感信息(如数据库凭据、API密钥等)保护的需求,以防止信息泄露。具体而言,这包括了三个主要场景:1. **"
+---
+
+## 用户问题 :
+ nacos配置中心密码加密 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+为了关于"Nacos配置中心密码加密"的问题,我们首先需要理解问题的具体背景和涉及的场景。根据提供的知识,我们可以分析如下:
+
+### 问题原因分析
+Nacos作为一款流行的配置管理平台,用户通常会面临敏感信息(如数据库凭据、API密钥等)保护的需求,以防止信息泄露。具体而言,这包括了三个主要场景:
+1. **Nacos Server数据库连接配置**的安全性。
+2. **Nacos Client与Nacos Server间通信**的安全性。
+3. **存储在Nacos配置中心的用户应用配置**的安全性。
+
+### 解决方案详解
+#### 针对Nacos Server数据库连接配置的加密
+- **自定义环境变量插件**:从Nacos 2.2版本起,你可以利用自定义环境变量插件来处理敏感配置,如数据库密码。社区提供了一个默认插件实现([https://github.com/nacos-group/nacos-plugin/tree/develop/nacos-custom-environment-plugin-ext](https://github.com/nacos-group/nacos-plugin/tree/develop/nacos-custom-environment-plugin-ext)),该插件允许你将数据库密码以Base64编码形式存储,从而实现一定程度的加密。你需要下载并安装此插件,然后按照文档指引配置。
+
+#### 针对存储在Nacos配置中心的用户应用配置加密
+- **配置加密插件**:自Nacos 2.1版本起,提供了配置加密插件([https://nacos.io/docs/latest/plugin/config-encryption-plugin/](https://nacos.io/docs/latest/plugin/config-encryption-plugin/)),它采用AES加密算法,默认实现允许你在配置存储和传输过程中加密敏感数据,仅在最终使用或通过控制台修改时展示为明文。请按照官方文档的指导,分别在客户端和服务端集成此插件。
+
+#### 针对Nacos Client与Nacos Server通信安全
+- 对于这部分,虽然Nacos本身没有直接提供加密插件,但客户端与服务端的通信可以通过SSL/TLS等标准安全协议进行加密,确保数据在传输过程中的安全性。此外,用户也可以在自己的应用程序层面,即在向Nacos Client传递敏感信息之前,对这些信息进行加密处理。
+
+### 解释
+以上步骤确保了Nacos配置中心中敏感信息的安全性,从配置存储的源头到数据传输过程都进行了相应的加密处理。使用Base64编码和AES加密算法都是业界广泛认可的安全实践,能有效提高配置信息的安全级别。对于未直接提供加密方案的部分(如Nacos Client配置),则推荐在应用层实施安全措施,这样既灵活也能保证整个系统的安全性。
+
+综上所述,Nacos配置中心的密码加密主要依赖于自定义环境变量插件和配置加密插件,以及在客户端与服务端通信时采取的标准安全协议。请根据实际情况选择合适的加密策略并正确实施。
+
+
+---------------
+
+
+## 参考链接 :
+
+*专家经验:Nacos 配置加密
+
+ *专家经验:nacos用户密码设置
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15803)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15794.md b/src/content/blog/faq/NACOS-user-question-history15794.md
new file mode 100644
index 00000000000..49af009a06d
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15794.md
@@ -0,0 +1,60 @@
+---
+id: "question-history-15794"
+title: "为什么选用nacos注册和配置中心 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "Nacos开源项目被选作注册和配置中心的原因主要包括以下几点,这些点可以从提供的知识内容中提炼出来:1. **强大的功能整合**:Nacos能够融合Spring框架(包括基础Spring和Spring Cloud),提供一站式的服务发现与配置管理解决方案。这意味着开发者可以使用统一的平台处理微服务架"
+---
+
+## 用户问题 :
+ 为什么选用nacos注册和配置中心 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+Nacos开源项目被选作注册和配置中心的原因主要包括以下几点,这些点可以从提供的知识内容中提炼出来:
+
+1. **强大的功能整合**:Nacos能够融合Spring框架(包括基础Spring和Spring Cloud),提供一站式的服务发现与配置管理解决方案。这意味着开发者可以使用统一的平台处理微服务架构中的关键组件,简化了开发和运维的复杂度。
+
+2. **动态配置管理**:Nacos支持配置的动态变更,无需重启应用即可实时更新配置,这对于需要频繁调整配置的生产环境尤为重要。例如,通过Nacos Spring的`@NacosPropertySource`和`@NacosValue`注解,可以轻松实现配置的自动刷新,保证了配置的灵活性和时效性。
+
+3. **服务注册与发现**:Nacos提供了高效的服务注册与发现机制,使得微服务实例能够在分布式环境中自动注册并发现其他服务,这对于构建高可用的微服务架构至关重要。不论是通过Nacos Spring直接集成还是利用Spring Cloud的starter,都能简便地实现这一功能。
+
+4. **易用性与兼容性**:Nacos提供了友好的界面操作和丰富的API接口,便于管理和监控服务状态。同时,它对Spring生态的高度兼容,意味着现有的Spring或Spring Cloud应用可以较为平滑地迁移到Nacos上,降低了技术迁移的成本。
+
+5. **社区与生态支持**:作为阿里巴巴开源的项目,Nacos背后有强大的技术支持和活跃的开发者社区,这意味着遇到问题时可以获得及时的帮助,同时也预示着Nacos会持续迭代发展,适应更多的应用场景。
+
+综上所述,Nacos之所以成为注册和配置中心的优选,是因为它集成了配置管理与服务发现的双重能力,提供了动态配置、服务高可用性保障以及良好的生态系统支持,极大地方便了微服务架构的构建与维护。
+
+从知识内容中并未发现直接给出的步骤性建议,但根据上述分析,若要实施Nacos作为注册和配置中心,可以遵循以下基于分析的步骤:
+
+- **准备阶段**:确保理解Nacos的基本概念和工作原理,阅读官方文档和参考资料。
+- **环境搭建**:按照Nacos快速入门指南([Nacos 快速入门](../quickstart/quick-start.md))下载并启动Nacos服务器。
+- **配置管理集成**:在Spring应用中添加Nacos依赖,使用`@EnableNacosConfig`和`@NacosPropertySource`等注解启用配置管理服务,并配置相应的属性以实现配置的动态加载与更新。
+- **服务发现集成**:根据所使用的Spring版本(基础Spring或Spring Cloud),选择合适的依赖(如`nacos-spring-context`或`spring-cloud-starter-alibaba-nacos-discovery`)并配置相应的注解(如`@EnableNacosDiscovery`),实现服务的注册与发现功能。
+- **测试与验证**:通过API调用或UI界面检查配置是否能动态更新,服务注册与发现是否正常工作。
+- **监控与优化**:利用Nacos提供的监控工具和日志,监控系统运行状态,根据实际运行情况调整配置策略,优化性能。
+
+以上步骤是基于Nacos与Spring集成的一般流程,具体实施时还需结合项目实际情况进行调整。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 融合 Spring,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring)
+
+ *[Nacos 融合 Spring Cloud,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring-cloud)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15804)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15795.md b/src/content/blog/faq/NACOS-user-question-history15795.md
new file mode 100644
index 00000000000..fde5051e696
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15795.md
@@ -0,0 +1,70 @@
+---
+id: "question-history-15795"
+title: "spring cloud 如何集成 nacos配置中心 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "要集成Nacos配置中心到Spring Cloud应用中,您可以遵循以下步骤操作,这些步骤基于提供的知识内容:### 1. 确保前提条件- **安装并启动Nacos Server**:首先,您需要下载并运行Nacos服务器。具体操作指南请参考[Nacos快速入门](../quickstart/qui"
+---
+
+## 用户问题 :
+ spring cloud 如何集成 nacos配置中心 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+要集成Nacos配置中心到Spring Cloud应用中,您可以遵循以下步骤操作,这些步骤基于提供的知识内容:
+
+### 1. 确保前提条件
+- **安装并启动Nacos Server**:首先,您需要下载并运行Nacos服务器。具体操作指南请参考[Nacos快速入门](../quickstart/quick-start.md)。
+
+### 2. 添加依赖
+在您的Spring Cloud项目的`pom.xml`文件中,加入Nacos Spring Cloud的起步依赖。确保使用的是适合您项目的最新版本,您可以通过访问[mvnrepository.com](https://mvnrepository.com/artifact/com.alibaba.cloud/spring-cloud-starter-alibaba-nacos-config)来查找最新的版本号。
+
+```xml
+
+ com.alibaba.cloud
+ spring-cloud-starter-alibaba-nacos-config
+ ${latest.version}
+
+```
+
+### 3. 配置Spring Cloud以使用Nacos Config
+- 在您的Spring Boot应用的`application.properties`或`bootstrap.properties`中,配置Nacos服务器地址和相关参数。例如:
+
+```properties
+spring.cloud.nacos.config.server-addr=127.0.0.1:8848
+# 如果有需要,可以配置namespace和group等其他参数
+```
+
+- 如果您想通过Java配置类的方式启用,可以使用`@EnableNacosConfig`注解(根据知识内容,直接使用`@EnableNacosConfig`可能不直接适用于Spring Cloud,但原理相似,应使用Spring Cloud提供的相应注解配置)以及相应的`@NacosPropertySource`来指定配置数据ID等。
+
+### 4. 应用配置更新
+- 在您的应用程序中,通过`@Value`注解或者`@ConfigurationProperties`来注入Nacos管理的配置值。Spring Cloud会自动处理配置的动态更新。
+
+### 5. 测试配置变更
+- 启动您的Spring Cloud应用后,可以通过Nacos控制台或API更改配置,观察应用是否能自动感知并应用新的配置值。
+
+### 解释:
+以上步骤基于Nacos与Spring Cloud的深度集成,允许您的微服务应用从Nacos配置中心动态获取和更新配置。通过Nacos Config模块,您可以实现配置的集中管理和动态刷新,无需重启应用即可生效配置变更,极大地提高了运维效率和灵活性。同时,Nacos Discovery模块虽然未在集成配置中心的直接步骤中提及,但它与配置中心紧密配合,能够帮助您实现服务的注册与发现,是构建完整微服务架构的重要组成部分。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 融合 Spring Cloud,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring-cloud)
+
+ *[Nacos 融合 Spring,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15805)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15796.md b/src/content/blog/faq/NACOS-user-question-history15796.md
new file mode 100644
index 00000000000..a43d49f7924
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15796.md
@@ -0,0 +1,59 @@
+---
+id: "question-history-15796"
+title: "nacos配置中心的缓存目录和容灾目录 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "关于您的问题「nacos-opensource nacos配置中心的缓存目录和容灾目录」,根据提供的知识内容,我们可以这样分析和解答:### 缓存目录的配置与管理1. **Nacos Server的缓存目录**:默认固定在`$nacos.home/data`目录下,目前官方说明指出这个目录是不能修改"
+---
+
+## 用户问题 :
+ nacos配置中心的缓存目录和容灾目录 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+关于您的问题「nacos-opensource nacos配置中心的缓存目录和容灾目录」,根据提供的知识内容,我们可以这样分析和解答:
+
+### 缓存目录的配置与管理
+
+1. **Nacos Server的缓存目录**:默认固定在`${nacos.home}/data`目录下,目前官方说明指出这个目录是不能修改的。这意味着Nacos服务器端的缓存位置不可自定义调整。
+
+2. **Nacos Client的缓存目录**:支持自定义修改,通过设置JVM参数`-Dnacos.client.cache.directory=/path/to/your/cache`(注意这里的变量名应根据实际情况调整,原参考中变量名可能有误,应为更通用的形式而非特定的`JM.SNAPSHOT.PATH`,后者更倾向于指快照路径)。这样可以让客户端的配置和服务信息缓存到指定的目录下,通常包含`config`和`naming`两个子目录分别用于配置和服务缓存。
+
+### 容灾与数据一致性
+
+虽然直接询问中未明确提及"容灾目录"的概念,但从数据一致性和可靠性角度出发,Nacos通过以下机制保障数据的一致性和容灾能力:
+
+- **数据同步与一致性保障**:Nacos采用自研的通知协议确保集群间配置的即时更新,利用MD5校验保证缓存与数据库数据的一致性。并且,无论使用内置Derby还是外置数据库如MySQL,都强调了数据库实例的统一和高可用性配置,这是实现跨节点数据一致性的基础。
+
+- **定期对账机制**:为应对异常情况,Nacos实施了定期的全量MD5对账策略,服务端与数据库每6小时,客户端与服务端每5分钟进行一次,确保长期运行中数据的最终一致性。
+
+### 解释与建议
+
+- **针对缓存目录**:如果您意图调整客户端的缓存位置,确保按照正确的JVM参数格式设置,并考虑此操作对应用部署和维护的影响。
+
+- **关于容灾考虑**:虽然没有直接描述“容灾目录”,但通过维护数据库层面的高可用性(如MySQL集群)、利用Nacos的自我修复和对账机制,以及合理规划Nacos集群部署(避免多集群共用同一数据库源的错误做法),可以构建起有效的容灾体系。
+
+综上所述,Nacos配置中心的缓存目录在客户端侧是可以灵活配置的,而服务器端则相对固定。对于容灾方面,更多依赖于合理的部署架构和数据库的高可用设计,以及Nacos内置的容错和一致性保障机制。希望这些信息能帮助您更好地理解Nacos配置中心的缓存与容灾策略。
+
+
+---------------
+
+
+## 参考链接 :
+
+*专家经验:Nacos缓存目录修改
+
+ *专家经验:Nacos 配置中心的数据同步和一致性问题
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15806)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15797.md b/src/content/blog/faq/NACOS-user-question-history15797.md
new file mode 100644
index 00000000000..f59cdef5bc0
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15797.md
@@ -0,0 +1,95 @@
+---
+id: "question-history-15797"
+title: "springboot如何集成nacos配置中心 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "要集成Nacos作为Spring Boot应用的配置中心,您可以遵循以下步骤操作,这些步骤基于提供的知识内容:### 1. 确保前提条件- **启动Nacos Server**:首先,确保您已下载并启动了Nacos服务器。如果您尚未完成这一步,请参照[Nacos快速入门](../quickstart"
+---
+
+## 用户问题 :
+ springboot如何集成nacos配置中心 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+要集成Nacos作为Spring Boot应用的配置中心,您可以遵循以下步骤操作,这些步骤基于提供的知识内容:
+
+### 1. 确保前提条件
+- **启动Nacos Server**:首先,确保您已下载并启动了Nacos服务器。如果您尚未完成这一步,请参照[Nacos快速入门](../quickstart/quick-start.md)进行操作。
+
+### 2. 添加依赖
+- 在您的Spring Boot项目的`pom.xml`文件中,添加Nacos Spring Context的依赖。确保替换`${latest.version}`为您查询到的最新版本号,您可以通过[mvnrepository.com](https://mvnrepository.com/artifact/com.alibaba.nacos/nacos-spring-context)获取。
+
+```xml
+
+ com.alibaba.nacos
+ nacos-spring-context
+ ${latest.version}
+
+```
+
+### 3. 配置Nacos属性源
+- 在Spring Boot的配置类中,使用`@EnableNacosConfig`和`@NacosPropertySource`注解来启用Nacos配置管理服务并加载配置。
+
+```java
+@Configuration
+@EnableNacosConfig(globalProperties = @NacosProperties(serverAddr = "127.0.0.1:8848"))
+@NacosPropertySource(dataId = "application", autoRefreshed = true)
+public class NacosConfig {
+}
+```
+这里,`dataId`通常对应您的配置文件名(如`application.properties`),`serverAddr`为Nacos服务器地址。
+
+### 4. 使用Nacos配置
+- 在需要使用配置的类或方法上,使用`@NacosValue`注解来注入配置值。
+
+```java
+@RestController
+public class ConfigController {
+
+ @NacosValue("${your.property.key}")
+ private String yourProperty;
+
+ @GetMapping("/config")
+ public String getConfigValue() {
+ return yourProperty;
+ }
+}
+```
+
+### 5. 动态刷新配置
+- 如示例所示,配置可以被Nacos动态更新。当Nacos中的配置发生改变时,Spring Boot应用会自动感知并更新相应的配置值。
+
+### 可选:服务发现
+- 如果您还希望使用Nacos进行服务注册与发现,需在配置类中添加`@EnableNacosDiscovery`注解,并在需要的地方注入`NamingService`来获取服务实例信息。
+
+### 注意事项
+- 确保Nacos Server运行正常且网络可达。
+- 根据实际情况调整`dataId`、`group`等参数以匹配您的配置需求。
+- 通过Nacos控制台或API发布配置后,Spring Boot应用能够自动获取最新的配置信息。
+
+以上步骤详细介绍了如何将Spring Boot应用与Nacos配置中心集成。如果在集成过程中遇到具体问题,建议查阅Nacos官方文档或提供的参考资料,如[Nacos Spring Project使用手册](https://github.com/nacos-group/nacos-spring-project/wiki/Nacos-Spring-Project-0.3.1-%E6%96%B0%E5%8A%9F%E8%83%BD%E4%BD%BF%E7%94%A8%E6%89%8B%E5%86%8C)以及Spring Cloud Alibaba的相关文档。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 融合 Spring,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring)
+
+ *专家经验:nacos 与 springboot 联合使用
+
+ *[Nacos 融合 Spring Cloud,成为注册配置中心](https://nacos.io/docs/latest/ecology/use-nacos-with-spring-cloud)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15807)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15799.md b/src/content/blog/faq/NACOS-user-question-history15799.md
new file mode 100644
index 00000000000..3e78a4d7589
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15799.md
@@ -0,0 +1,82 @@
+---
+id: "question-history-15799"
+title: "nacos配置中心客户端鉴权 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "要实现Nacos配置中心客户端的鉴权,您可以按照以下步骤操作:### 对于Java SDK的鉴权1. **初始化配置服务**: - 准备好Nacos服务器地址(`serverAddr`),例如:`String serverAddr = 您的Nacos服务器地址;` - 创建`Properti"
+---
+
+## 用户问题 :
+ nacos配置中心客户端鉴权 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+要实现Nacos配置中心客户端的鉴权,您可以按照以下步骤操作:
+
+### 对于Java SDK的鉴权
+
+1. **初始化配置服务**:
+ - 准备好Nacos服务器地址(`serverAddr`),例如:`String serverAddr = "您的Nacos服务器地址";`
+ - 创建`Properties`对象并设置服务器地址:`Properties properties = new Properties(); properties.put("serverAddr", serverAddr);`
+
+2. **添加用户名和密码**:
+ - 如果Nacos配置中心需要用户名和密码认证,向`Properties`对象中添加这些凭据:
+ ```java
+ properties.put("username", "您的用户名");
+ properties.put("password", "您的密码");
+ ```
+
+3. **创建配置服务实例**:
+ - 使用`NacosFactory`创建`ConfigService`实例,这一步骤会处理鉴权逻辑:
+ ```java
+ try {
+ ConfigService configService = NacosFactory.createConfigService(properties);
+ } catch (NacosException e) {
+ e.printStackTrace();
+ // 处理异常情况
+ }
+ ```
+
+### Open-API鉴权流程
+
+1. **登录获取AccessToken**:
+ - 使用`curl`命令或类似HTTP客户端登录Nacos服务器并获取AccessToken:
+ ```shell
+ curl -X POST 'http://您的Nacos服务器地址/nacos/v1/auth/login' -d 'username=您的用户名&password=您的密码'
+ ```
+ 成功后会返回AccessToken。
+
+2. **使用AccessToken进行后续请求**:
+ - 在所有需要鉴权的API请求URL后面添加`accessToken=${accessToken}`,其中`${accessToken}`是从上一步获取的访问令牌。例如,查询配置信息:
+ ```shell
+ curl -X GET 'http://您的Nacos服务器地址/nacos/v1/cs/configs?accessToken=${accessToken}&dataId=配置ID&group=配置组'
+ ```
+
+### 自定义鉴权插件(高级需求)
+
+- 如果有特殊鉴权需求,可以开发自定义鉴权插件,实现`com.alibaba.nacos.plugin.auth.spi.client.ClientAuthService`接口,并遵循Nacos提供的SPI机制进行插件集成。
+
+根据上述步骤,您可以实现Nacos配置中心客户端的鉴权功能。请确保您已经阅读并理解了Nacos官方文档中关于鉴权的详细说明,以应对实际部署中的特定安全需求和配置细节。如果您在实施过程中遇到具体技术问题,欢迎再次咨询。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Authorization](https://nacos.io/docs/latest/guide/user/auth)
+
+ *[鉴权插件](https://nacos.io/docs/latest/plugin/auth-plugin)
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15809)给我们反馈。
diff --git a/src/content/blog/faq/NACOS-user-question-history15800.md b/src/content/blog/faq/NACOS-user-question-history15800.md
new file mode 100644
index 00000000000..c3f35b06cd1
--- /dev/null
+++ b/src/content/blog/faq/NACOS-user-question-history15800.md
@@ -0,0 +1,79 @@
+---
+id: "question-history-15800"
+title: "nacos配置中心性能测试修改哪些参数 nacos-opensource"
+date: "2024-06-21"
+category: "expertConsultation"
+description: "要进行Nacos配置中心的性能测试并修改相关参数以优化其表现,我们可以依据提供的知识进行分析与指导。根据提及的内容,这里直接给出基于知识的分析与建议步骤,不涉及额外提问,因为问题直接关联到Nacos配置中心的性能调优。### 分析原因在进行Nacos配置中心的性能测试前,需了解几个关键因素可能影响其"
+---
+
+## 用户问题 :
+ nacos配置中心性能测试修改哪些参数 nacos-opensource
+
+---------------
+## 专家官方解答 :
+
+要进行Nacos配置中心的性能测试并修改相关参数以优化其表现,我们可以依据提供的知识进行分析与指导。根据提及的内容,这里直接给出基于知识的分析与建议步骤,不涉及额外提问,因为问题直接关联到Nacos配置中心的性能调优。
+
+### 分析原因
+
+在进行Nacos配置中心的性能测试前,需了解几个关键因素可能影响其性能,包括但不限于JVM配置、集群设置、数据库配置以及网络环境等。特别是根据Nacos 2.0的压测报告,单节点在特定硬件条件下可支持的客户端连接数为4.2万个,要达到百万级别需构建相应规模的集群。
+
+### 具体步骤与说明
+
+#### 1. **修改JVM参数**
+
+- **操作位置**:`nacos/bin/startup.sh`
+- **目的**:优化内存分配、垃圾回收策略等,以提升服务端处理能力。
+- **示例**:增加堆内存大小,调整新生代与老年代比例等。
+
+#### 2. **配置集群**
+
+- **操作位置**:`conf/cluster.conf`
+- **目的**:设置集群模式及各节点信息,以分散请求负载,提高并发处理能力。
+- **示例**:列出所有参与集群的Nacos服务器IP地址。
+
+#### 3. **调整数据库配置**
+
+- **操作位置**:`conf/application.properties`
+- **目的**:优化数据库连接池配置、事务处理等,确保数据访问高效稳定。
+- **示例**:合理设置`db.num`、`db.connection.timeout`等参数。
+
+#### 4. 参考官方压测报告进行调优
+
+- **链接**:[Nacos 2.0配置中心性能测试报告](https://nacos.io/docs/latest/guide/admin/nacos2-config-benchmark/)
+- **目的**:根据报告中的测试环境与结果,对比自身需求,适当调整硬件资源或软件配置。
+
+#### 5. 监控与评估
+
+- **工具**:利用Nacos自带或第三方监控工具持续观察性能指标。
+- **目的**:实时了解系统运行状态,及时发现并解决性能瓶颈。
+
+### 解释
+
+上述步骤首先通过优化JVM参数增强服务端处理能力,确保内存管理高效;接着通过集群部署分散请求,提高系统整体承载量;调整数据库配置是为了保证数据访问的效率和稳定性,这是配置中心性能的关键一环。参考官方压测报告进行调优,是因为这些数据提供了实际场景下的性能基准,有助于更精确地规划和优化。最后,持续监控是任何性能调优不可或缺的部分,它帮助我们验证调优效果并及时响应异常情况。
+
+综上所述,根据Nacos开源资料和性能测试报告,通过细致的配置调整与合理的集群规划,可以有效提升Nacos配置中心的性能,满足高并发场景下的需求。
+
+
+---------------
+
+
+## 参考链接 :
+
+*[Nacos 2.0 升级文档](https://nacos.io/docs/latest/upgrading/200-upgrading)
+
+ *专家经验:Nacos用作配置中心的话,能够支持百万级别的客户端连接嘛
+
+ *专家经验:主流的配置中心有哪些?
+
+
+ ---------------
+
+
+
+## 答疑服务说明:
+
+本内容经由技术专家审阅的用户问答的镜像生成,我们提供了专家智能答疑服务,在页面的右下的浮窗”专家答疑“。您也可以访问 : [全局专家答疑](https://answer.opensource.alibaba.com/docs/intro) 。 咨询其他产品的的问题
+
+### 反馈
+如问答有错漏,欢迎点:[差评](https://ai.nacos.io/user/feedbackByEnhancerGradePOJOID?enhancerGradePOJOId=15810)给我们反馈。
diff --git a/src/content/docs/ebook/zh-cn/agxdnq.mdx b/src/content/docs/ebook/zh-cn/agxdnq.mdx
index 1afc9305b7f..f5d08c158ae 100644
--- a/src/content/docs/ebook/zh-cn/agxdnq.mdx
+++ b/src/content/docs/ebook/zh-cn/agxdnq.mdx
@@ -1,6 +1,6 @@
---
title: Nacos 一致性协议
-keywords: [ Nacos ]
+keywords: [Nacos, 一致性协议, Raft, Distro, 微服务, 服务注册发现, 配置管理, CP/AP协议, 数据一致性, 分布式存储]
description: 廖春涛(春少) Nacos PMC为什么 Nacos 需要一致性协议Nacos 在开源支持就定下了一个目标,尽可能的减少用户部署以及运维成本,做到用户只需要一个程序包,就可以快速以单机模式启动 Nacos 或者以集群模式启动 Nacos。而 Nacos 是一个需要存储数据的一个组件,因此,为了...
---
> 廖春涛(春少) Nacos PMC
diff --git a/src/content/docs/ebook/zh-cn/ais5np.mdx b/src/content/docs/ebook/zh-cn/ais5np.mdx
index 7744166fe08..e151f7e394e 100644
--- a/src/content/docs/ebook/zh-cn/ais5np.mdx
+++ b/src/content/docs/ebook/zh-cn/ais5np.mdx
@@ -1,7 +1,7 @@
---
title: Nacos Eook
-keywords: [ Nacos ]
-description: Nacos Eook
+keywords: [Nacos, 电子书, 导航, 配置管理, 服务发现]
+description: Nacos电子书深入讲解了如何有效利用Nacos进行服务发现、配置管理及动态配置更新,是微服务架构下的核心组件指南,适合想深入了解Nacos的开发者阅读。
---
import Lock from "@components/common/EbookLock.astro";
diff --git a/src/content/docs/ebook/zh-cn/akfgmk.mdx b/src/content/docs/ebook/zh-cn/akfgmk.mdx
index 7744166fe08..0d94b877a45 100644
--- a/src/content/docs/ebook/zh-cn/akfgmk.mdx
+++ b/src/content/docs/ebook/zh-cn/akfgmk.mdx
@@ -1,7 +1,7 @@
---
title: Nacos Eook
-keywords: [ Nacos ]
-description: Nacos Eook
+keywords: [Nacos, Eook, 概述, 微服务, 配置管理]
+description: Nacos Eook深入介绍Nacos作为微服务架构中的核心组件,重点阐述配置管理、服务发现和服务健康监测功能,是微服务实践者不可多得的参考指南。
---
import Lock from "@components/common/EbookLock.astro";
diff --git a/src/content/docs/ebook/zh-cn/dl5k6n.mdx b/src/content/docs/ebook/zh-cn/dl5k6n.mdx
index 4caef406935..3af61247c38 100644
--- a/src/content/docs/ebook/zh-cn/dl5k6n.mdx
+++ b/src/content/docs/ebook/zh-cn/dl5k6n.mdx
@@ -1,7 +1,7 @@
---
title: 配置一致性模型
-keywords: [ Nacos ]
-description: 李艳林(彦林) Nacos PMCNacos 配置管理一致性协议分为两个大部分,第一部分是 Server 间一致性协议,一个是 SDK 与 Server 的一致性协议,配置作为分布式系统中非强一致数据,在出现脑裂的时候可用性高于一致性,因此阿里配置中心是采用 AP 一致性协议。Server 间...
+keywords: [Nacos, 配置管理, 一致性协议, Server间一致性, SDK与Server一致性, AP一致性, Raft协议, MD5校验, Nacos 1.X, Nacos 2.X]
+description: 本文详细介绍了Nacos配置管理的一致性模型,包括Server间与SDK与Server的两部分协议。首先阐述了在分布式系统中,配置数据的AP一致性选择,接着解析了有DB模式下的读写分离架构及无DB模式下的Raft协议应用。进一步说明了Nacos 1.X与2.X版本中如何利用MD5校验确保配置同步,其中2.X升级为长链接模式,提高了配置变更的通信效率。
---
> 李艳林(彦林) Nacos PMC
diff --git a/src/content/docs/ebook/zh-cn/ex6h8a.mdx b/src/content/docs/ebook/zh-cn/ex6h8a.mdx
index 7744166fe08..0d94b877a45 100644
--- a/src/content/docs/ebook/zh-cn/ex6h8a.mdx
+++ b/src/content/docs/ebook/zh-cn/ex6h8a.mdx
@@ -1,7 +1,7 @@
---
title: Nacos Eook
-keywords: [ Nacos ]
-description: Nacos Eook
+keywords: [Nacos, Eook, 概述, 微服务, 配置管理]
+description: Nacos Eook深入介绍Nacos作为微服务架构中的核心组件,重点阐述配置管理、服务发现和服务健康监测功能,是微服务实践者不可多得的参考指南。
---
import Lock from "@components/common/EbookLock.astro";
diff --git a/src/content/docs/latest/en/archive/activity.md b/src/content/docs/latest/en/archive/activity.md
index bb002239641..86864e017dd 100644
--- a/src/content/docs/latest/en/archive/activity.md
+++ b/src/content/docs/latest/en/archive/activity.md
@@ -1,50 +1,41 @@
---
-title: Nacos activity
-keywords: [Nacos,activity]
-description: Nacos award activity
+title: Introduction to the Nacos Reward Program
+keywords: [Nacos, Reward Program, Community Contribution, GitHub Issue, Customized Gifts]
+description: Nacos launches a reward program to encourage users to participate in product improvements, including identifying errors on the official website and documentation, optimizing startup procedures, testing cluster mode, contributing suggestions for Java SDK and OpenAPI, streamlining contribution processes, among others. Participants must join the Nacos WeChat community group, submit found issues or suggestions via reporting GitHub Issues, with opportunities to win customized gifts. Due to resource constraints, the event is limited to the first 40 registrants. Communication via mailing lists or Issues is encouraged for documentation and sharing purposes.
---
+# Introduction to the Nacos Reward Program
-# Nacos activity
+## I. Activity Task List
-## I. Activity task list
+- Review the official website content, locate bugs, and provide constructive suggestions for both Chinese and English versions.
+- Read through both Chinese and English documentation, identify errors, and suggest improvements, especially focusing on suboptimal English translations.
+- Test the code compilation, packaging, Nacos server startup/shutdown process, and propose enhancements.
+- Configure and initiate multi-node Nacos cluster mode tasks, offering improvement feedback.
+- Utilize the Nacos Java SDK and provide improvement suggestions.
+- Try the Nacos Open API and contribute suggestions for improvement.
+- Follow the 'How to Contribute to Nacos Documentation' guide and propose enhancements to the contributor process.
+- Share your requirements, development plans, ideas, and expectations for Nacos.
-* Read the official website copy, find the official website bug, and make constructive suggestions for the official website of Chinese and English.
-* Read Chinese and English documents, find document bugs, and give suggestions for improving Chinese and English documents (especially pay attention to places where English translation is not good, because English is our own programmers)
-* Try the code -> compile and package -> start Nacos server -> stop Nacos server process, propose improvements
-* Try to configure and start multi-node Nacos cluster mode tasks, suggestions for improvement
-* Try to use the Nacos Java SDK to give suggestions for improvements to the Java SDK
-* Try to improve the OpenAPI with the Nacos Open API
-* Try to contribute to the contributor process by trying to contribute to the contribution process based on How to Contribute to Nacos Documentation
-* Ask Nacos for needs, development plans, ideas and requirements, etc.
+## II. Participation Method
-## II. Activity participation method
+- Scan the WeChat QR code of "Super Brother" to be included in the "Nacos Community Discussion Group."
+- Select one or more tasks from Section I.
+- After discovering issues or bugs, follow the 'Issue Reporting Method' detailed in Section III and assign it to the GitHub user [xuechaos](https://github.com/xuechaos).
-* Scan "超哥" WeChat 2 microcode, let "超哥"" help join "Nacos community exchange group"
+## III. Issue Reporting Method
+- Submit issues based on the issue template in the Nacos GitHub repository.
+- For code repository issues, submit pull requests for fixes, which upon review and approval will merge into the main branch, earning you the title of Contributor.
+## IV. Task Rewards
-
+- Customized gifts featuring the Nacos logo are being prepared for participants who make significant contributions, with consideration for shipping these gifts to standout contributors.
+- Although the gifts are modest, they symbolize our gratitude for your assistance.
+- With limited funding, we can only offer 40 gifts at present, hence participation is capped at 40 individuals on a first-come, first-served basis.
+- Recipients are encouraged, if willing, to share a photo (with the option to conceal their face) with the gift for potential use in future community event materials.
+## V. Additional Notes
-* Select one or more experience tasks in (I)
-* After discovering the problem or bug, follow the "Report Report Method" in (III), send a corresponding github issue, and assign it to @github account [xuechaos] ([https://github.com/xuechaos](https://github.com/xuechaos))
-
-## III. Problem Report Mode
-
-* Submit questions in the nacos repository at github according to the issues template.
-* Code warehouse problem, submit pull request repair, after review, merge into the trunk, it becomes a Contributor.
-* [https://github.com/alibaba/nacos](https://github.com/alibaba/nacos)
-* [https://github.com/nacos-group/nacos-group.github.io](https://github.com/nacos-group/nacos-group.github.io)
-
-## IV. Mission Rewards
-
-* We are customizing some small gifts with Nacos Logo for the small partners who have participated and made outstanding contributions, and will consider the courier to the small partners who have made outstanding contributions in the process.
-* The gift is light, but I hope I can express my gratitude for your help.
-* Due to limited funds, we can only provide 40 gifts at present, so we limit the number of participants to 40 people, first come first served.
-* If you wish, the partner who wishes to receive the gift can send a photo with the gift (can hide the face) for us as a photo material for our community activities.
-
-## V. Other instructions
-
-* We are not sure that every proposal will be adopted in the end, but we will try to explain what kind of consideration we are based on, and we did not recommend your proposal.
-* Try to report problems by mailing list or report issue instead of reporting on WeChat group to document and facilitate our communication process.
-
+- While we cannot guarantee the adoption of every suggestion, we strive to explain our considerations for those not implemented.
+- Preferably, utilize the mailing list or report issues instead of WeChat group discussions to document and facilitate communication and knowledge preservation.
+---
\ No newline at end of file
diff --git a/src/content/docs/latest/en/archive/cli-guide.md b/src/content/docs/latest/en/archive/cli-guide.md
index b9609816ef5..57891247d6a 100644
--- a/src/content/docs/latest/en/archive/cli-guide.md
+++ b/src/content/docs/latest/en/archive/cli-guide.md
@@ -1,7 +1,6 @@
---
title: CLI Guide
-keywords: [CLI,Guide]
-description: In plan with Nacos 1.x.x
+keywords: [CLI, Guide, Nacos, 1.x.x, Planned]
+description: This article outlines the Command Line Interface (CLI) guide for Nacos 1.x.x version, highlighting the features and improvements planned.
---
-
-**IN PLAN** with Nacos 1.x.x
+**PLANNED** for Nacos 1.x.x
\ No newline at end of file
diff --git a/src/content/docs/latest/en/archive/contributing-dev.md b/src/content/docs/latest/en/archive/contributing-dev.md
index 57c031c34c6..de30d8b4835 100644
--- a/src/content/docs/latest/en/archive/contributing-dev.md
+++ b/src/content/docs/latest/en/archive/contributing-dev.md
@@ -1,130 +1,86 @@
---
title: Contributing to Nacos
-keywords: [Nacos,contribute]
-description: Welcome to Nacos! This document is a guideline about how to contribute to Nacos.
+keywords: [Nacos, Contribution, Code of Conduct, GitHub, Pull Request, Community Roles, Committer]
+description: This guide outlines the steps for contributing to the Nacos project, from adhering to the code of conduct, submitting issues, engaging in the development workflow, understanding code review standards, to becoming a Committer.
---
-
# Contributing to Nacos
+Welcome to Nacos! This document serves as a guide on how to contribute to Nacos.
-Welcome to Nacos! This document is a guideline about how to contribute to Nacos.
-
-If you find something incorrect or missing, please leave comments / suggestions.
+If you spot inaccuracies or missing content, please leave feedback and suggestions.
-## Before you get started
+## Before You Begin
### Code of Conduct
-Please make sure to read and observe our [Code of Conduct](https://github.com/alibaba/nacos/blob/master/CODE_OF_CONDUCT.md).
-
-## Contributing
-
-Nacos welcome new participants of any role, including user, contributor, committer and PMC.
-
-
-
-
-We encourage new comers actively join in Nacos projects and involving from user role to committer role, and even PMC role. In order to accomplish this, new comers needs to actively contribute in Nacos project. The following paragraph introduce how to contribute in Nacos way.
-
-#### Open / pickup an issue for preparation
-
-If you find a typo in document, find a bug in code, or want new features, or want to give suggestions, you can [open an issue on GitHub](https://github.com/alibaba/Nacos/issues/new) to report it.
+Ensure you read and abide by our [Code of Conduct](https://github.com/alibaba/nacos/blob/master/CODE_OF_CONDUCT.md).
-If you just want to contribute directly you can choose the issue below.
+## Contributions and the Nacos Community Structure
-- [Contribution Welcome](https://github.com/alibaba/nacos/labels/contribution%20welcome): Heavily needed issue, but currently short of hand.
-
-- [good first issue](https://github.com/alibaba/nacos/labels/good%20first%20issue): Good for newcomers, new comer can pickup one for warm-up.
-
+Nacos warmly welcomes new participants in any role, including users, contributors, committers, and PMC members.
-We strongly value documentation and integration with other projects such as Spring Cloud, Kubernetes, Dubbo, etc. We are very glad to work on any issue for these aspects.
+
-Please note that any PR must be associated with a valid issue. Otherwise the PR will be rejected.
+We encourage newcomers to actively engage in the Nacos community, which has mechanisms in place to facilitate progression from a user role to a committer or even a PMC role. To achieve this, newcomers must actively participate in the Nacos community. The following paragraphs outline how to contribute to Nacos and advance within the community.
-#### Begin your contribute
+#### Reporting Issues
-Now if you want to contribute, please create a new pull request.
+If you find typos in the documentation, errors in the code, desire new features, or wish to offer suggestions, you can [open an issue on GitHub](https://github.com/alibaba/Nacos/issues/new) to report them.
-We use the `develop` branch as the development branch, which indicates that this is a unstable branch.
+If you're eager to get started, consider issues labeled in our GitHub repository:
+- [good first issue](https://github.com/alibaba/nacos/labels/good%20first%20issue): Ideal entry points for beginners.
+- [contribution welcome](https://github.com/alibaba/nacos/labels/contribution%20welcome): Critical issues or important modules needing attention and contributions.
-Further more, our branching model complies to [https://nvie.com/posts/a-successful-git-branching-model/](https://nvie.com/posts/a-successful-git-branching-model/). We strongly suggest new comers walk through the above article before creating PR.
+We highly value documentation and integrations with projects like Spring Cloud, Kubernetes, Dubbo, etc. Research into these areas is greatly appreciated.
-Now, if you are ready to create PR, here is the workflow for contributors:
+Note that any PR (Pull Request) must be associated with a valid issue; otherwise, the PR will be rejected.
-1. Fork to your own
-
-2. Clone fork to local repository
-
-3. Create a new branch and work on it
-
-4. Keep your branch in sync
-
-5. Commit your changes (make sure your commit message concise)
-
-6. Push your commits to your forked repository
-
-7. Create a pull request to **develop** branch.
-
+#### Starting Your Contribution
-When creating pull request:
+To contribute, create a new pull request.
-1. Please follow [the pull request template](https://github.com/alibaba/nacos/blob/master/.github/PULL_REQUEST_TEMPLATE.md).
-
-2. Please create the request to **develop** branch.
-
-3. Please make sure the PR has a corresponding issue.
-
-4. If your PR contains large changes, e.g. component refactor or new components, please write detailed documents about its design and usage.
-
-5. Note that a single PR should not be too large. If heavy changes are required, it's better to separate the changes to a few individual PRs.
-
-6. After creating a PR, one or more reviewers will be assigned to the pull request.
-
-7. Before merging a PR, squash any fix review feedback, typo, merged, and rebased sorts of commits. The final commit message should be clear and concise.
-
+Our `develop` branch serves as the development branch, indicating instability.
-If your PR contains large changes, e.g. component refactor or new components, please write detailed documents about its design and usage.
+Moreover, our branching model adheres to [https://nvie.com/posts/a-successful-git-branching-model/](https://nvie.com/posts/a-successful-git-branching-model). We strongly advise new members to review this article before creating a PR.
-### Code review guidance
+When ready, follow this workflow:
-Committers will rotate reviewing the code to make sure all the PR will be reviewed timely and by at least one committer before merge. If we aren't doing our job (sometimes we drop things). And as always, we welcome volunteers for code review.
+1. Fork the Nacos repository to your GitHub account.
+2. Clone the forked repository locally.
+3. Create a new branch and make changes.
+4. Keep your branch synchronized.
+5. Commit your changes (ensuring commit messages are concise).
+6. Push your commits to your GitHub remote repository.
+7. Initiate a Pull Request targeting the **develop** branch.
-Some principles:
+When creating a PR:
-- Readability - Important code should be well-documented. API should have Javadoc. Code style should be complied with the existing one.
-
-- Elegance: New functions, classes or components should be well designed.
-
-- Testability - 80% of the new code should be covered by unit test cases.
-
-- Maintainability - Comply with our [PMD spec](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md), and 3-month-frequency update should be maintained at least.
-
+1. Adhere to the [Pull Request template](https://github.com/alibaba/nacos/blob/master/.github/PULL_REQUEST_TEMPLATE.md).
+2. Direct the PR to the **develop** branch.
+3. Ensure the PR links to a corresponding issue.
+4. If your PR involves substantial changes such as component refactoring or new components, document their design and usage thoroughly.
+5. Avoid overly large PRs; split extensive changes into several smaller PRs if necessary.
+6. After creating a PR, reviewers will be assigned.
+7. Prior to merging, pay attention to the commit nodes, including addressing review feedback, typos, merges. The final commit message should be clear and concise.
-### Now how about try become a committer?
+### Code Review Guidelines
-Generally speaking, contribute 8 non-trivial patches and get at least three different people to review them (you'll need three people to support you). Then ask someone to nominate you. You're demonstrating your:
+Committers rotate reviewing code to ensure timely review of all PRs by at least one committer. If we fall short (which happens occasionally), we welcome volunteers for code reviews.
-- at least 8 PR and the associated issues to the project,
-
-- ability to collaborate with the team,
-
-- understanding of the projects' code base and coding style,
-
-- ability to write good code (last but certainly not least)
-
+Key principles include readability, elegance, testability, and maintainability, adhering to our [coding conventions](https://github.com/alibaba/nacos/blob/master/style/codeStyle.md), with updates maintained at least every three months.
-A current committer nominates you by slacking the team on the Nacos issue with label "nomination"
+### Becoming a Committer
-- your first and last name
-
-- a link to your Git profile
-
-- an explanation of why you should be a committer,
-
-- Elaborate the top 3 PR and the associated issues the nominator has worked with you that can demonstrate your ability.
-
+Typically, by contributing eight non-trivial patches and having at least three different individuals review them (indicating community support), you may then request nomination. Demonstrate:
-Two other committer need to second your nomination. If no one objects in 5 working days (China), you're a committer. If anyone objects or wants more information, the committers discuss and usually come to a consensus (within the 5 working days). If issues cannot be resolved, there's a vote among current committers.
+- At least eight PRs and related issues,
+- Collaborative skills with the team,
+- Familiarity with the project's codebase and coding style,
+- Ability to write high-quality code.
-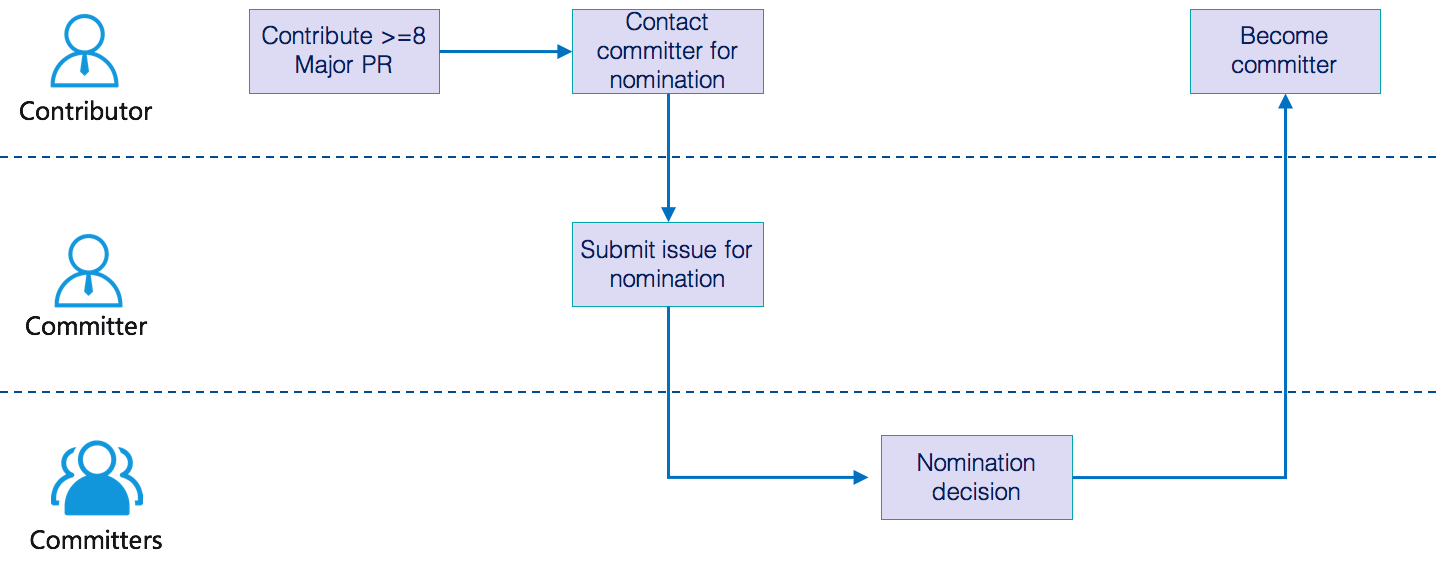
+Current committers nominate via Nacos issues, sharing:
+- Your full name,
+- A link to your Git profile,
+- Explanation for your suitability as a Committer,
+- Highlighting your top three PRs and related issues.
-In the worst case, this can drag out for two weeks. Keep contributing! Even in the rare cases where a nomination fails, the objection is usually something easy to address like "more patches" or "not enough people are familiar with this person's work."
+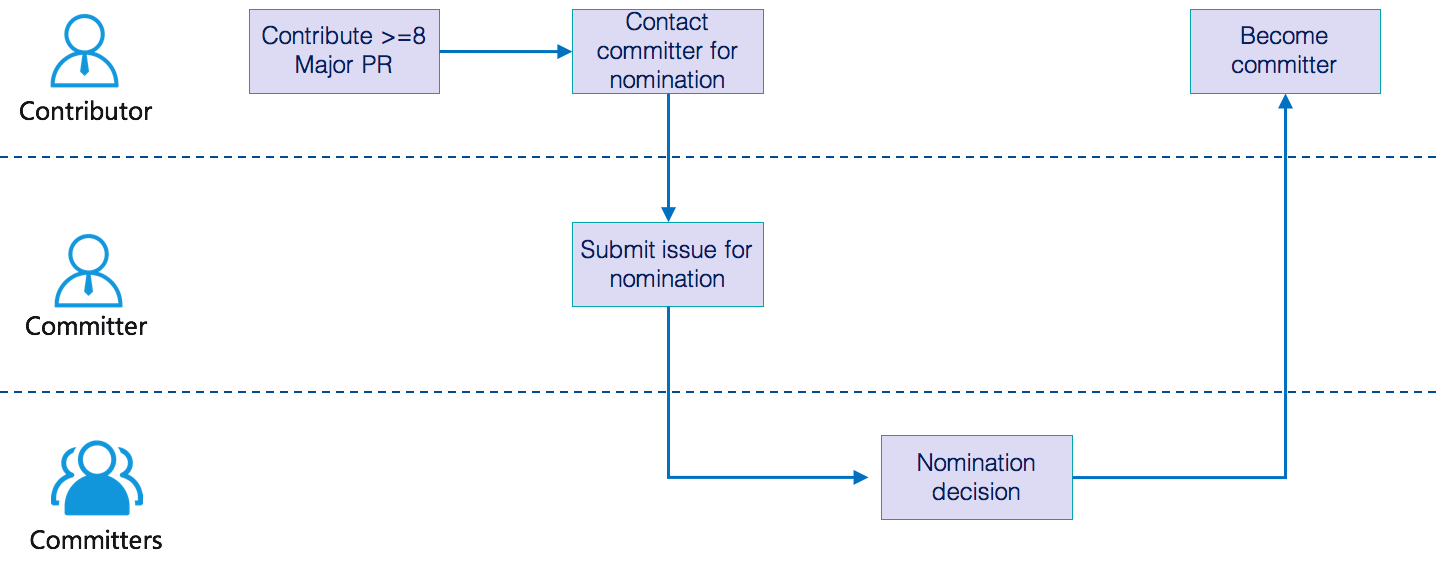
\ No newline at end of file
diff --git a/src/content/docs/latest/en/archive/use-nacos-with-springcloud.md b/src/content/docs/latest/en/archive/use-nacos-with-springcloud.md
index 30b9fcc317d..0b5064b925c 100644
--- a/src/content/docs/latest/en/archive/use-nacos-with-springcloud.md
+++ b/src/content/docs/latest/en/archive/use-nacos-with-springcloud.md
@@ -1,7 +1,7 @@
---
title: Nacos Spring Cloud
-keywords: [nacos,Spring Cloud]
-description: Nacos Spring Cloud
+keywords: [Nacos, Spring Cloud, microservice configuration, service discovery, Alibaba Cloud]
+description: Nacos Spring Cloud is an open-source microservices management tool from Alibaba that seamlessly integrates with the Spring Cloud framework, offering powerful and flexible service discovery and configuration management features. Learn its applications through Nacos Config Example and Nacos Discovery Example, with related projects including Nacos, Nacos Spring, among others – making it an ideal choice for building cloud-native applications.
---
# Nacos Spring Cloud
diff --git a/src/content/docs/latest/en/community/community.md b/src/content/docs/latest/en/community/community.md
index 3dfb43b612e..64477883451 100644
--- a/src/content/docs/latest/en/community/community.md
+++ b/src/content/docs/latest/en/community/community.md
@@ -1,6 +1,6 @@
---
title: Community
-keywords: [community,contact]
+keywords: [Community, Contact Information]
description: Community
sidebar:
order: 1
@@ -8,23 +8,23 @@ sidebar:
# Community
-## Contact us
+## Contact Us
-### Nacos Gitter-[https://gitter.im/alibaba/nacos](https://gitter.im/alibaba/nacos)
-### Nacos weibo-[https://weibo.com/u/6574374908](https://weibo.com/u/6574374908)
-### Nacos segmentfault-[https://segmentfault.com/t/nacos](https://segmentfault.com/t/nacos)
+### Nacos Gitter - [https://gitter.im/alibaba/nacos](https://gitter.im/alibaba/nacos)
+### Nacos Weibo - [https://weibo.com/u/6574374908](https://weibo.com/u/6574374908)
+### Nacos SegmentFault - [https://segmentfault.com/t/nacos](https://segmentfault.com/t/nacos)
-### Mailing list
+### Mailing List
-Mailing list is recommended for discussing almost anything related to Nacos. Please refer to this?[guide](https://github.com/apache/incubator-dubbo/wiki/Mailing-list-subscription-guide)?for detailed documentation on how to subscribe to our mailing lists.
+The mailing list is recommended for discussions on anything related to Nacos. Please refer to the [Reference Manual](https://github.com/apache/incubator-dubbo/wiki/Mailing-list-subscription-guide) for instructions on subscribing to our mailing lists.
-* [dev-nacos@googlegroups.com](mailto:dev-nacos%2Bsubscribe@googlegroups.com): The develop mailing list. You can ask questions here if you encounter any problem when using or developing Nacos.
-* [commits-nacos@googlegroups.com](mailto:commits-nacos%2Bsubscribe@googlegroups.com): All commits will be sent to this mailing list. You can subscribe to it if you are interested in Nacos' development.
-* [users-nacos@googlegroups.com](mailto:users-nacos%2Bsubscribe@googlegroups.com): All Github?[issue](https://github.com/alibaba/nacos/issues)?updates and?[pull request](https://github.com/alibaba/nacos/pulls)?updates will be sent to this mailing list.
-* [nacos_dev@linux.alibaba.com](mailto:nacos_dev@linux.alibaba.com).
+* [dev-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): Development mailing list. If you encounter any issues while using or developing Nacos, feel free to ask here.
+* [commits-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): All commits will be sent to this mailing list. Subscribe if you are interested in the development of Nacos.
+* [users-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): Issues raised on Github, updates, and [feature requests](https://github.com/alibaba/nacos/pulls) will be forwarded to this mailing list.
+* [nacos_dev@linux.alibaba.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1).
-### Nacos DingDing group
+### Nacos DingTalk Group
-Welcome to join Nacos community nail group
+You're welcome to join the Nacos DingTalk group.
-
\ No newline at end of file
+
\ No newline at end of file
diff --git a/src/content/docs/latest/en/community/nacos-dev.md b/src/content/docs/latest/en/community/nacos-dev.md
index 73ee7dd2bb3..f40c9f411b5 100644
--- a/src/content/docs/latest/en/community/nacos-dev.md
+++ b/src/content/docs/latest/en/community/nacos-dev.md
@@ -1,40 +1,41 @@
---
title: Developers
-keywords: [nacos,developers,committer,pmc,nacos team]
-description: Nacos developers page
+keywords: [Nacos, developers, Maintainer, Committer, Contributor]
+description: A detailed introduction to the developer system of the Nacos project, including definitions, rights, and obligations of roles such as Maintainer, Committer, and Contributor, along with showcasing key members of the development team, highlighting the responsibilities and contributions recognized in open-source projects.
sidebar:
order: 2
---
# Developers
-## Nacos Developer Roles
+## Roles of Nacos Developers
-Nacos developers include three roles: Maintainer, Committer, and Contributor. The standard definitions for each role are as follows.
+Nacos developers encompass three roles: Maintainer, Committer, and Contributor, each defined as follows.
### Maintainer
-Maintainer is an individual who has made a significant contribution to the evolution and development of the Nacos project, including projects under the nacos-group. Specifically includes the following criteria:
+Maintainers are individuals who have made significant contributions to the evolution and growth of the Nacos project (including projects under nacos-group). They typically:
-* Completing the design and development of multiple key modules or projects, is an core developer of the project
-* Continuous investment and passion, can actively participate in the maintenance of related matters such as community, official website, issue, PR, etc.
-* Has a visible influence in the community and is able to represent Nacos in important community meetings and events
-* Have the consciousness and ability to cultivate Committer and Contributor
+- Design and develop multiple key modules or projects, functioning as core developers;
+- Continuously invest time and passion, actively participating in community maintenance, official website management, issue handling, and PR activities;
+- Possess notable influence within the community, representing Nacos at important community conferences and events;
+- Demonstrate awareness and capability to nurture Committers and Contributors.
### Committer
-Committer is an individual with write access to the Nacos repository and includes the following criteria:
+Committers are individuals with write access to the Nacos repository, meeting criteria such as:
-* An individual who can contribute to the issue and PR continuously for a long time;
-* Participate in the maintenance of the issue list and discussion of important features;
-* Participate in code review;
+- Consistently contributing issues and PRs over an extended period;
+- Engaging in issue list maintenance and discussions on major features;
+- Participating in code reviews.
### Contributor
-Contributor is an individual who contributes to the Nacos project. The standard is:
+Contributors are individuals who have made contributions to the Nacos project, specifically by having their PRs merged.
-* Submitted a PR that is merged;
+### Rights and Obligations of Nacos Developers
+The rights and obligations of Nacos developers are outlined in a chart which visually represents these aspects.
### Nacos Developer Rights and Obligations
diff --git a/src/content/docs/latest/en/concepts.md b/src/content/docs/latest/en/concepts.md
index 8f980b59078..c96c712bc37 100644
--- a/src/content/docs/latest/en/concepts.md
+++ b/src/content/docs/latest/en/concepts.md
@@ -1,101 +1,77 @@
---
title: Nacos Concepts
-keywords: [Nacos,Concepts]
-description: Nacos Concepts
+keywords: [Nacos, Concepts, Configuration Management, Service Discovery, Health Check]
+description: Nacos, as a dynamic configuration and service discovery platform, introduces key concepts such as regions, availability zones, configurations, and namespaces, highlighting the importance of configuration management and the mechanisms for service registration and discovery to ensure system high availability and flexibility.
---
-
# Nacos Concepts
-> NOTE: Nacos introduces some basic concepts and systematic understanding of these concepts can help you better understand and correct use Nacos products.
+> NOTE: Nacos introduces fundamental concepts; understanding these systematically can help you better comprehend and effectively utilize the Nacos product.
## Region
+A physical data center where resources, once created, cannot be changed.
-Physical data centers, unalterable after resources are created.
-
-## Available Zone
-
-Physical areas with independent power grids and networks in one region. The network latency for instances in the same zone is lower.
+## Availability Zone
+A physically independent region within the same region with separate power and network. Instances within the same availability zone have lower network latency.
-## Endpoint
-
-The entry domain name of a service in each region.
+## Access Point
+The entry domain name of a service in a region.
## Namespace
-
-For configuration isolation by tenants. Different namespaces may have configurations with the same Group or Data ID. One of the common scenarios for namespace is to differentiate and isolate the configurations in different environments, as in development and test environment and production environment.
+For tenant-level configuration isolation. Different namespaces can contain identical Group or Data ID configurations. A common scenario for namespaces is the separation of resources (like configurations, services) between development/test environments and production.
## Configuration
-
-During system development, developers usually extract some parameters or variables that need to be changed from the code and manage them in a separate configuration file. This enables the static system artifacts or deliverables (such as WAR and JAR packages) to fit with the physical operating environment in a better way. Configuration management is usually a part of system deployment, which is executed by the administrator or operation and maintenance personnel. Configuration modification is an effective way to adjust the behavior of a running system.
+During system development, developers typically separate variables requiring changes from code into standalone managed configurations. This aims to adapt static system artifacts or deliverables (e.g., WAR, JAR files) to actual runtime environments more efficiently. Configuration management generally includes activities such as editing, storing, distributing, change management, historical versioning, and audit trails related to configurations.
## Configuration Management
-
-Configuration-related activities including editing, storage, distribution, modification management, release version management, and modification audit.
+All activities involving configurations, including editing, storage, distribution, change management, history, and auditing.
## Configuration Item
-
-A specific configurable parameter with its value range, generally in the form of param-key=param-value. For example, the log output level (logLevel=INFO|WARN|ERROR) of a system is regarded as a configuration item.
+An individual configurable parameter and its value range, typically in the form of param-key=param-value. For example, setting a system's log output level (logLevel=INFO|WARN|ERROR) is a configuration item.
## Configuration Set
+A collection of related or unrelated configuration items is a configuration set. In a system, a configuration file is often a configuration set containing various system configurations. A configuration set may include settings for databases, thread pools, log levels, etc.
-A collection of related or unrelated configuration items.In a system, a configuration file is generally a configuration set which contains all the configurations of the system. For example, a configuration set may contain configuration items such as data sources, thread pools, and log levels.
-
-## Data ID
+## Configuration Set ID
+The ID of a specific configuration set in Nacos. The Data ID is one of the dimensions for organizing configurations, typically following a naming rule akin to Java packages (e.g., com.taobao.tc.refund.log.level) for global uniqueness, though not mandatory.
-The ID of a configuration set in Nacos. It is one of the dimensions according to which configurations are organized. Data ID is generally used to organize the system configuration sets. A system or application can contain multiple configuration sets, each of which can be identified by a meaningful name. The Data ID usually uses the naming rule similar to Java packages (for example, com.taobao.tc.refund.log.level) to ensure global uniqueness. This naming rule is not mandatory.
-
-## Group
-
-The group of configuration sets in Nacos. It is one of the dimensions according to which configurations are organized. The configuration sets are always grouped by a meaningful string such as Buy or Trade to differentiate the configuration sets with the same Data ID. When you create a configuration on Nacos, the group name is replaced by DEFAULT\_GROUP by default if not specified. A typical scenario of Group is when the same configuration type is used for different applications or components, such as database\_url configuration and MQ\_topic configuration.
+## Configuration Group
+A group of configuration sets in Nacos, another dimension for organization. Meaningful strings (e.g., Buy or Trade) distinguish configuration sets with identical Data IDs. If the configuration group name is not specified when creating a configuration in Nacos, it defaults to DEFAULT_GROUP. Common scenarios include different applications or components using the same configuration types, like database_url and MQ_topic.
## Configuration Snapshot
-
-The Nacos client SDK can generate snapshots of configurations on local machines. Snapshots can be used to indicate the overall disaster recovery capabilities of the system when the client cannot connect to the Nacos server. Configuration snapshot is similar to local commit in Git, or cache, which is updated at the appropriate time, but does not have the notion of expiration as in cache.
+The Nacos client SDK generates local snapshots of configurations. These serve to enhance the system's overall disaster recovery capability when the client cannot connect to the Nacos Server. Configuration snapshots resemble local commits in Git or caches, updated at appropriate times but without expiration.
## Service
-
-Software functions which are provided to the client via the network through a predefined interface.
+Software functionality provided over a network via predefined interfaces for clients.
## Service Name
-
-Identifier provided by the service, by which the service it refers to can be uniquely determined.
+The identifier for a service, allowing unique determination of the referred service.
## Service Registry
-
-Database which stores the instances of services and the load balancing policies for services.
+A database storing service instances and load balancing strategies.
## Service Discovery
-
-On a computer network, the address and metadata of an instance under the service are probed (usually using a service name) and provided to the client for querying with a predefined interface.
+In computer networks,探测服务实例的地址和元数据(usually by service name) and providing them to clients through predefined interfaces.
## Metadata
-
-Custom configuration information, such as a disaster recovery policy, a load balancing policy, an authentication configuration, and various tags. From the scope of action, it is divided into meta-information of service level, meta-information of virtual cluster, and meta-information of instance.
+Descriptive information of Nacos data (configurations and services), such as service versions, weights, disaster recovery strategies, load balancing policies, authentication configurations, and various custom labels (labels). Ranging from service-level, cluster-level, to instance-level metadata.
## Application
-
-Property of service which can be used to identify the service provider.
+An attribute of a service identifying the service provider.
## Service Group
-
-Different services can be categorized into the same service group.
+Different services can be categorized under the same group.
## Virtual Cluster
-
-Service instances under the same service can be further classified. One possible unit of this classification is Virtual Cluster.
+All service instances under the same service form a default cluster, which can be further divided into virtual clusters as needed.
## Instance
-
-A process with an accessible network address (IP:Port) that provides one or more services.
+A process with an accessible network address (IP:Port) providing one or more services.
## Weight
-
-Instance-level configuration. Weight is a floating-point number. The greater the weight, the greater the traffic that the instance expects to be allocated.
+An instance-level configuration. Weights are floating-point numbers. Higher weights result in more traffic directed to that instance.
## Health Check
+Inspects the health of instances attached to a service to determine if they can provide service. Based on the check results, instances are marked healthy or unhealthy. When requesting resolution for a service, unhealthy instances are not returned to clients.
-Health check of the instances under a service in a specified manner to ensure that the instances can work properly. Instances are judged to be healthy or unhealthy according to the inspection results. Unhealthy instances are not returned to the client when initiating a resolution request to the service.
-
-## Protect Threshold
-
-To prevent traffic from flowing to healthy instances because of some unhealthy instances, which causes traffic pressure, healthy instance collapse, and finally an avalanche, the health protection threshold should be defined as a floating point number between 0 and 1. When the proportion of the domain name healthy instance to the total instance is smaller than this value, the instance is returned to the client regardless of the health of the instance. Although this can result in a loss of some of the traffic, we ensure that the remaining healthy instances can work normally.
+## Health Protection Threshold
+To prevent healthy instances from being overwhelmed by traffic due to too many unhealthy instances, this threshold, a float between 0 and 1, ensures that even unhealthy instances are returned to clients when the ratio of healthy instances to total service instances falls below this value. Although some traffic is lost, this safeguards the remaining healthy instances from collapsing under pressure and avoids a cascading failure.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/contribution/contributing-flow.md b/src/content/docs/latest/en/contribution/contributing-flow.md
index 88ce07fb0f7..570e55a9208 100644
--- a/src/content/docs/latest/en/contribution/contributing-flow.md
+++ b/src/content/docs/latest/en/contribution/contributing-flow.md
@@ -1,74 +1,67 @@
---
-title: Contributing Flow
-keywords: [Contributing,Source Code]
-description: This contribution flow is applicable to all Nacos community content, including but not limited to Nacos, Nacos wiki/doc, Nacos SDK.
-sidebar:
- order: 2
+title: Nacos Contribution Workflow
+keywords: [Nacos, Contribution Process, Source Code, GitHub, Community Contribution, Pull Request, Rebase]
+description: This contribution workflow is applicable to all Nacos community content, including but not limited to `Nacos`, `Nacos Wiki/Doc`, and `Nacos SDK`.
---
-# Nacos Contributing Flow
+# Nacos Contribution Workflow
-This contribution flow is applicable to all Nacos community content, including but not limited to `Nacos`, `Nacos wiki/doc`, `Nacos SDK`.
+This contribution workflow is universal for all Nacos community content, encompassing elements like `Nacos`, `Nacos Wiki/Documentation`, and `Nacos SDK`.
-The following use contributing `Nacos` as an example to explain the contribution flow in detail.
+Below, we use contributing to `Nacos` as an example to elaborate on the contribution process.
-## 1. Fork Alibaba/Nacos repository to your Github.
+## 1. Fork the Alibaba/Nacos Project to Your GitHub Repository
-## 2. Clone your fork Nacos repository to local.
+## 2. Clone or Download the Forked Nacos Code Repository Locally
-```
+```shell
git clone ${your fork nacos repo address}
cd nacos
```
-## 3. Add Alibaba/Nacos repository as upstream repo.
+## 3. Add the Alibaba/Nacos Repository as the Upstream Repository
-```
+```shell
git remote add upstream https://github.com/alibaba/nacos.git
git remote -v
- origin ${your fork nacos repo address} (fetch)
- origin ${your fork nacos repo address} (push)
- upstream https://github.com/alibaba/nacos.git (fetch)
- upstream https://github.com/alibaba/nacos.git (push)
+ origin ${your fork nacos repo address} (fetch)
+ origin ${your fork nacos repo address} (push)
+ upstream https://github.com/alibaba/nacos.git (fetch)
+ upstream https://github.com/alibaba/nacos.git (push)
git fetch origin
git fetch upstream
```
-## 4. Choose a basic branch of development usually upstream/develop,and create a new branch based on it.
+## 4. Choose a Development Base Branch, Typically upstream/develop, and Create a New Branch from It
-```
-(checkout branch from remote repo to local)
+```shell
+# Fetch the branch from the remote repository to local
git checkout -b upstream-develop upstream/develop
-(Create a development branch from the local branch, usually using the issue number as the development branch name)
+# From the local branch, create a development branch, usually named after the related issue number
git checkout -b develop-issue#${issue-number}
-
```
-## 5. Do your change in your local develop branch.
-
-First please make sure you read and set the `Nacos code style` correctly, please read the related content [Code of Conduct](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md).
-
-When making changes, please ensure that the changes on this branch are **only relevant to the issue**, and try to be as small as possible, so that **only one thing is modified in one branch, and only one thing is modified in one PR**.
+## 5. Make Modifications on the Newly Created Local Development Branch
-At the same time, please use your English description as much as possible for your commits. It is mainly described by **predicate + object**, such as: `Fix xxx problem/bug`.
+Before modifying, ensure you've read and set up the `Nacos Code Style`. Refer to [Nacos Coding Convention](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md).
-Some simple commits can be described using `For xxx`, such as: `For codestyle`.
+When making changes, ensure they are **solely related to the issue** and as granular as possible, adhering to the principle of **one branch, one modification, one PR**.
-If the commits is related to an ISSUE, you can add the ISSUE number as a prefix, such as: `For #10000, Fix xxx problem/bug`.
+Commit messages should primarily be in English, structured as **Verb + Object**, e.g., `Fix xxx problem/bug`. For minor adjustments, use `For xxx`, e.g., `For codestyle`. If the commit relates to a specific ISSUE, prefix it with the ISSUE number, e.g., `For #10000, Fix xxx problem/bug`.
-## 6. Rebase develop branch
+## 6. Rebase the Base Branch with the Development Branch
-When you make changes, other people's changes may have commited and merged. At this time, there may be conflicts. Please use the rebase command to merge and resolve. There are two main benefits:
+During your modifications, others' changes might have been merged, potentially causing conflicts. Use the rebase command to resolve these, which benefits in two ways:
-1. Your submission record will be very clean, without the words `Merge xxxx branch`.
-2. After rebase, the commit log of your branch is also a single chain, it is easier to check back.
+1. Your commit history will be clean, devoid of `Merge xxxx branch` entries.
+2. After rebasing, your branch's commit log forms a single chain, simplifying traceback.
-```
+```shell
git fetch upstream
git rebase -i upstream/develop
@@ -77,31 +70,27 @@ git rebase -i upstream/develop
OR
-```
+```shell
git checkout upstream-develop
git pull
git checkout develop-issue#${issue-number}
git rebase -i upstream-develop
```
-**If you are using Intellij IDEA**, it is recommended to use the IDE version control, which has a more convenient visual panel to resolve conflicts and squash operations.
+**For IntelliJ IDEA users**, the version control module provides a visual interface for conflict resolution and squashing operations.
-## 7. Push your develop branch to your fork repository.
+## 7. Push Your Developed and Rebased Branch to Your Forked Repository
-```
+```shell
git push origin develop-issue#${issue-number}
```
-## 8. Create Pull Request according to the pull request template
-
-[pull request template](./pull-request.md)
-
-The Nacos community will review your Pull Request and may propose comments.
-
-You can return to step 5 to modify code according to the comments and use step 6 to resubmit.
+## 8. Create a Pull Request Following the Pull Request Template
-**If you are prompted that there are conflicts when you push to fork repo again, Force push to your fork branch will be ok.** The reason of conflicts is that the commit ID has changed after you rebase with others changes.
+[Pull Request Template](./pull-request.md)
+The Nacos community will review your Pull Request and may provide feedback for adjustments. You can return to step 5 for modifications and use step 6 to resubmit.
-## 9. If no more problem, Nacos community will merge your PR. Congratulations for you becoming a official contributor of Nacos.
+**If prompted about commit record conflicts upon resubmission, force push to your forked branch since your commit ID changed post-rebase due to new merges in the base branch.**
+## 9. Once approved, your changes will be merged into the base branch, congratulating you on becoming an official Nacos contributor.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/contribution/contributing.md b/src/content/docs/latest/en/contribution/contributing.md
index 227b5311ecf..18b37b14ecb 100644
--- a/src/content/docs/latest/en/contribution/contributing.md
+++ b/src/content/docs/latest/en/contribution/contributing.md
@@ -1,59 +1,56 @@
---
title: How to Contribute
-keywords: [contribute,code]
-description: We are always very happy to have contributions, whether for trivial cleanups or big new features.
+keywords: [Contribution, Source Code]
+description: We warmly welcome your contributions and participation, whether it's minor cleanups or major new features.
sidebar:
order: 1
---
# How to Contribute
-Nacos is released under the non-restrictive Apache 2.0 license, and follows a very standard Github development process, using Github tracker for issues and merging pull requests into master. If you want to contribute even something trivial, please do not hesitate, but follow the guidelines below.
+We warmly welcome your contributions and involvement, be it trivial cleanups or substantial new features. Our aim is to provide high-quality, well-documented code for every programming language.
-We are always very happy to have contributions, whether for trivial cleanups or big new features.
-We want to have high quality, well documented codes for each programming language.
+Code isn't the only way to contribute to the project. We highly value improvements in documentation, integration with other projects, and gladly accept enhancements in these areas.
-Nor is code the only way to contribute to the project. We strongly value documentation, integration with other project, and gladly accept improvements for these aspects.
+## Reach Out to Us
-## Contact us
+#### Nacos Gitter - [https://gitter.im/alibaba/nacos](https://gitter.im/alibaba/nacos)
+#### Nacos Weibo - [https://weibo.com/u/6574374908](https://weibo.com/u/6574374908)
+#### Nacos SegmentFault - [https://segmentfault.com/t/nacos](https://segmentfault.com/t/nacos)
-##### Nacos Gitter- [https://gitter.im/alibaba/nacos](https://gitter.im/alibaba/nacos)
-##### Nacos weibo- [https://weibo.com/u/6574374908](https://weibo.com/u/6574374908)
-##### Nacos segmentfault- [https://segmentfault.com/t/nacos](https://segmentfault.com/t/nacos)
+#### Mailing List
-#### Mailing list
+The mailing list is recommended for discussing anything related to Nacos. Refer to the [Reference Manual](https://github.com/apache/incubator-dubbo/wiki/Mailing-list-subscription-guide) for instructions on subscribing to our mailing lists.
-Mailing list is recommended for discussing almost anything related to Nacos. Please refer to this?[guide](https://github.com/apache/incubator-dubbo/wiki/Mailing-list-subscription-guide)?for detailed documentation on how to subscribe to our mailing lists.
-
-* [dev-nacos@googlegroups.com](mailto:dev-nacos%2Bsubscribe@googlegroups.com): The develop mailing list. You can ask questions here if you encounter any problem when using or developing Nacos.
-* [commits-nacos@googlegroups.com](mailto:commits-nacos%2Bsubscribe@googlegroups.com): All commits will be sent to this mailing list. You can subscribe to it if you are interested in Nacos' development.
-* [users-nacos@googlegroups.com](mailto:users-nacos%2Bsubscribe@googlegroups.com): All Github?[issue](https://github.com/alibaba/nacos/issues)?updates and?[pull request](https://github.com/alibaba/nacos/pulls)?updates will be sent to this mailing list.
-* [nacos_dev@linux.alibaba.com](mailto:nacos_dev@linux.alibaba.com).
+- [dev-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): Development mailing list. If you encounter any issues while using or developing Nacos, feel free to ask here.
+- [commits-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): All commits are sent to this mailing list. Subscribe if you're interested in Nacos' development.
+- [users-nacos@googlegroups.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1): Issues raised, updates, and [feature requests](https://github.com/alibaba/nacos/pulls) on Github are sent here.
+- [nacos_dev@linux.alibaba.com](https://lark.alipay.com/nacos/nacosdocs/vl19q1).
## Contributing Code
-### Notice
+### Guidelines for Contributing Code
-To submit a change for inclusion, please do the following:
+Before contributing code, please confirm and check the following:
-#### Read Nacos [Code of Conduct](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md), and make sure your IDE has set code style and install plugin.
+#### Read Nacos' [Coding Convention](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md) and set up your IDE's code style and validation plugins accordingly.
-#### If the change is non-trivial, please include unit tests that cover the new functionality.
+#### If changes are minor, write some unit tests that cover the new functionality.
-#### If you are introducing a completely new feature or API, it is a good idea to start a wiki and get consensus on the basic design first.
+#### If introducing a brand-new feature or API, initiate a wiki discussion and reach a consensus on the basic design before proceeding.
-### Contribution flow
+### Contribution Workflow
-This is a rough outline of what a contributor's workflow looks like:
+Here is a general workflow for contributors:
-* Fork the current repository.
-* Create a topic branch from where to base the contribution. This is usually the master branch.
-* Make commits of logical units.
-* Make sure commit messages are in the proper format (see below).
-* Push changes in a topic branch to your forked repository.
-* Follow the checklist in the [pull request template](./pull-request.md).
-* Before you send the pull request, please sync your forked repository with remote repository. This will make your pull request simple and clear. See guide below:
-```
+* Fork the current GitHub repository.
+* Create a branch as the base for your contribution, typically the develop branch.
+* Make some changes and commit them.
+* Ensure commit messages follow the correct format (see below).
+* Push your changes to your forked repository.
+* Follow the checklist in the [Pull Request Template](./pull-request.md).
+* Before submitting a pull request, sync your fork repository with the upstream one to simplify your pull request. Details are as follows:
+```bash
git remote add upstream git@github.com:alibaba/nacos.git
git fetch upstream
git rebase upstream/master
@@ -61,42 +58,44 @@ git checkout -b your_awesome_patch
... add some work
git push origin your_awesome_patch
```
-* Submit a pull request to alibaba/nacos and wait for reply.
+* Submit a pull request to alibaba/nacos and await feedback. If replies are slow, kindly remind us.
+
+* For a detailed contribution process, refer to [Contribution Flow](./contributing-flow.md).
-* Detail contribution flow see [Contribution Flow](./contributing-flow.md)
+## Contributing Documentation
-Thanks for contributing!
+### Guidelines for Contributing Documentation
-## Contributing Document
+Before contributing documentation, please confirm and check the following:
-### Notice
+#### Confirm that the documentation indeed contains errors or is missing information.
-When contributing documents, please confirm and check the following:
+#### Familiarity with [Markdown](https://www.markdownguide.org/getting-started).
-#### Has been confirmed that the document is indeed wrong or missing.
+#### Familiarity with [docsite](https://github.com/txd-team/docsite), at least able to complete local debugging based on the [official documentation README.md](https://github.com/nacos-group/nacos-group.github.io).
-#### Familiar with [Markdown](https://www.markdownguide.org/getting-started)
+### Contribution Workflow
-#### Familiar with [docsite](https://github.com/txd-team/docsite), at least be able to complete local debugging according to the [document README.md](https://github.com/nacos-group/nacos-group.github.io).
+Refer to [Contribution Flow](./contributing-flow.md).
## Becoming a Committer
-We are always interested in adding new contributors. What we look for are series of contributions, good taste and ongoing interest in the project. If you are interested in becoming a committer, please let one of the existing committers know and they can help you walk through the process.
+We actively onboard new contributors. We prioritize a series of continuous contributions, good taste, and sustained interest in maintaining the project. If you wish to become a Committer, let an existing Committer know; they will assist you in joining through your contributions.
-Nowadays, we have several important contribution points:
+Currently, we have several key areas for contribution:
#### Wiki & JavaDoc
#### Nacos Console
-#### Nacos SDK(C++\.Net\PHP\Python\Go\Node.js)
+#### Nacos SDK (C++, .Net, PHP, Python, Go, Node.js)
#### Prerequisites
-If you want to contribute to the above listing points, you must abide by the prerequisites listed below:
+If you wish to contribute to these areas, you must adhere to some prerequisites:
-##### Readability - APIs as well as important methods must have Javadoc.
+##### Readability – An API must have JavaDoc, and some crucial methods must also have JavaDoc.
-##### Testability - Ensure over 80% unit test coverage for main processes.
+##### Testability – Unit test coverage for the testing process should be at least 80%.
-##### Maintainability - Comply with our [Code of Conduct](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md), with an update frequency at least once every 3 months.
+##### Maintainability – Must comply with our [Coding Convention](https://github.com/alibaba/nacos/blob/develop/style/codeStyle.md) and have an update frequency of at least once every three months.
-##### Deployability - We encourage you to deploy into [maven repository](http://search.maven.org/).
+##### Deployability – We encourage deployments to the [Maven Repository](http://search.maven.org/)
\ No newline at end of file
diff --git a/src/content/docs/latest/en/contribution/how-to-reporting-bugs.md b/src/content/docs/latest/en/contribution/how-to-reporting-bugs.md
index bfe00fd895f..a65ccd4a141 100644
--- a/src/content/docs/latest/en/contribution/how-to-reporting-bugs.md
+++ b/src/content/docs/latest/en/contribution/how-to-reporting-bugs.md
@@ -1,32 +1,28 @@
---
-title: How to report bugs
-keywords: [nacos,submit,reporting bugs]
-description: How to report bugs
-sidebar:
- order: 4
+title: How to Submit a Bug Report
+keywords: [Submit, Bug Report, Nacos, GitHub, Security Issue, ASRC]
+description: This article guides users on submitting bug reports for the Nacos project, detailing necessary steps and recommendations, as well as how to report security issues through ASRC for efficient handling.
---
-# Reporting bugs
+# How to Submit a Bug Report
-If any part of the Nacos project has bugs or documentation mistakes, please let us know by [opening an issue](https://github.com/alibaba/nacos/issues/new). We treat bugs and mistakes very seriously and believe no issue is too small, anyOne is implement. Before creating a bug report, please check that an issue reporting the same problem does not already exist.
+Should you encounter any issues or documentation problems within the Nacos project, please inform us by [opening an issue](https://github.com/alibaba/nacos/issues/new). We take errors and bugs very seriously; no problem is insignificant in front of our product. However, before creating a bug report, please verify if there's an existing issue reporting the same problem.
-To make the bug report accurate and easy to understand, please try to create bug reports that are:
+To ensure your bug report is accurate and easy to understand, attempt to include the following details:
-- Specific. Include as much details as possible: which version, what environment, what configuration, etc. If the bug is related to running the Nacos server, please attach the Nacos log (the starting log with Nacos configuration is especially important).
+- Be specific. Include as many details as possible: which version, what environment, what configurations, etc. If the error relates to running the Nacos server, attach the Nacos logs, with startup logs being particularly crucial.
-- Reproducible. Include the steps to reproduce the problem. We understand some issues might be hard to reproduce, please includes the steps that might lead to the problem. If possible, please attach the affected Nacos data dir and stack strace to the bug report.
+- Reproducible. Outline the steps to reproduce the issue. We understand some issues may be difficult to reproduce, but include any steps that might lead to the problem. If possible, append the affected Nacos data directory and stack strace to the bug report.
-- Unique. Do not duplicate existing bug report.
+- Avoid duplication. Do not replicate existing bug reports.
+Before filing a bug report, it's advisable to read Elika Etemad's article on [submitting good bug reports](http://fantasai.inkedblade.net/style/talks/filing-good-bugs/), which could be enlightening.
-It may be worthwhile to read [Elika Etemad’s article on filing good bug reports](http://fantasai.inkedblade.net/style/talks/filing-good-bugs/) before creating a bug report.
-
-We might ask for further information to locate a bug. A duplicated bug report will be closed.
+We may request additional information from you to pinpoint the bug. Duplicate reports will be closed.
[nacos-issue](https://github.com/alibaba/nacos/issues/new)
-
[filing-good-bugs](http://fantasai.inkedblade.net/style/talks/filing-good-bugs/)
-# Reporting security bugs
+# How to Report a Security Issue
-If you find any security problem in the Nacos project, please let us know through [ASRC (Alibaba Security Response Center)](https://security.alibaba.com).
+If you identify a security issue within the Nacos project, please notify us through the [ASRC (Alibaba Security Response Center)](https://security.alibaba.com).
\ No newline at end of file
diff --git a/src/content/docs/latest/en/contribution/pull-request.md b/src/content/docs/latest/en/contribution/pull-request.md
index b00b6ff00d2..56190812c9b 100644
--- a/src/content/docs/latest/en/contribution/pull-request.md
+++ b/src/content/docs/latest/en/contribution/pull-request.md
@@ -1,32 +1,30 @@
---
-title: Pull request template
-keywords: [pull request,template]
-description: Pull request template
-sidebar:
- order: 3
+title: Submitting Request Templates
+keywords: [pull request, template, changelog, unit test]
+description: This article outlines the guidelines for submitting request templates, emphasizing the need to create associated issues before submission. It clarifies the purpose of the change, provides a brief changelog, and details verification procedures. A comprehensive checklist is provided to guide contributors on effectively integrating their code, covering aspects such as using Github issues, formatting pull request titles, writing descriptive change summaries, conducting necessary unit tests, and executing essential Maven commands for checks. Contributors of substantial work are reminded to sign the Apache Individual Contributor License Agreement.
---
-# Pull request template
+# Submitting Request Templates
-Please do not create a Pull Request without creating an issue first.
+Do not submit requests without creating corresponding issues.
-## What is the purpose of the change
+## Purpose of the Change
XXXXX
-## Brief changelog
+## Brief Changelog
XX
-## Verifying this change
+## Verifying This Change
XXXX
-Follow this checklist to help us incorporate your contribution quickly and easily:
+Adhere to this checklist to facilitate the swift and effortless incorporation of your contribution:
-* [ ] Make sure there is a Github issue filed for the change (usually before you start working on it). Trivial changes like typos do not require a Github issue. Your pull request should address just this issue, without pulling in other changes - one PR resolves one issue.
-* [ ] Format the pull request title like `[ISSUE #123] Fix UnknownException when host config not exist`. Each commit in the pull request should have a meaningful subject line and body.
-* [ ] Write a pull request description that is detailed enough to understand what the pull request does, how, and why.
-* [ ] Write necessary unit-test to verify your logic correction, more mock a little better when cross module dependency exist. If the new feature or significant change is committed, please remember to add integration-test in [test module](https://github.com/apache/rocketmq/tree/master/test).
-* [ ] Run `mvn -B clean apache-rat:check findbugs:findbugs` to make sure basic checks pass. Run `mvn clean install -DskipITs` to make sure unit-test pass. Run `mvn clean test-compile failsafe:integration-test` to make sure integration-test pass.
-* [ ] If this contribution is large, please file an [Apache Individual Contributor License Agreement](http://www.apache.org/licenses/#clas).
\ No newline at end of file
+* [ ] Ensure a Github issue exists for the change (typically before starting work), except for trivial changes like typos. Your pull request should address solely this issue, avoiding inclusion of additional changes – one PR resolves one issue.
+* [ ] Format the pull request title as `[ISSUE #123] Fix UnknownException when host config not exist`. Each commit within the pull request should have a meaningful subject and body.
+* [ ] Compose a pull request description that sufficiently elucidates the change, its methodology, and rationale.
+* [ ] Develop necessary unit tests to validate your logic corrections, employing more mocks when dealing with cross-module dependencies. If introducing a new feature or significant modification, remember to include integration tests in the [test module](https://github.com/apache/rocketmq/tree/master/test).
+* [ ] Execute `mvn -B clean apache-rat:check findbugs:findbugs` to confirm the passage of fundamental checks. Run `mvn clean install -DskipITs` to ensure unit tests pass. Execute `mvn clean test-compile failsafe:integration-test` to verify the success of integration tests.
+* [ ] For substantial contributions, please submit an [Apache Individual Contributor License Agreement](http://www.apache.org/licenses/#clas).
\ No newline at end of file
diff --git a/src/content/docs/latest/en/ecology/use-nacos-with-k8s-sync.md b/src/content/docs/latest/en/ecology/use-nacos-with-k8s-sync.md
index e09006b216a..f7c1b57589d 100644
--- a/src/content/docs/latest/en/ecology/use-nacos-with-k8s-sync.md
+++ b/src/content/docs/latest/en/ecology/use-nacos-with-k8s-sync.md
@@ -1,37 +1,37 @@
---
-title: Nacos supports synchronizing metadata from K8S service discovery
-keywords: [Nacos,k8s,kubernetes]
-description: Nacos supports synchronizing metadata from K8S service discovery
+title: Nacos Supports Synchronizing Service Metadata from K8S Service Discovery
+keywords: [Nacos, k8s, Kubernetes, service metadata, synchronization]
+description: Nacos now integrates with Kubernetes (K8S) service discovery, enabling automatic synchronization of service metadata. This feature monitors dynamic changes in services and instances within K8S, ensuring timely updates to the Nacos service discovery module, compatible with K8S version 1.22 and above. It facilitates a two-way data flow with specific configurations, streamlining microservices architecture management.
---
-# Nacos supports synchronizing metadata from K8S service discovery
+# Nacos Supports Synchronization of Service Metadata from K8S Service Discovery
-## Data synchronization
-Nacos monitors the changes of services and instances in K8S, obtains its service metadata, and synchronizes the change information to Nacos' service discovery. Supports K8S version 1.22 (corresponding to K8S-Java-API version 14.0.0).The diagram is as follow:
-
+## Data Synchronization
+Nacos listens for changes in services and instances within K8S, acquiring their service metadata, and synchronizing update information to the Nacos service discovery module and instances. It supports K8S version 1.22 (corresponding to the K8S-Java-API version 14.0.0). An illustrative diagram is as follows:
+
-Mapping scheme of K8S resource synchronization to Nacos resource (single-direction, Nacos resource synchronization to K8S resource to be supplemented) :
+The mapping scheme for synchronizing K8S resources to Nacos resources (unidirectional, with supplementation for Nacos resource synchronization to K8S resources pending):
-K8S Data to be synchronized|Field in K8S|Field mapped to Nacos
----|---|---
-service name|service.metadata.name|service.name
-service targetPort(pod port)(multiple)|service.ports.targetPort|instance.port
-service name|service.metadata.name|instance.cluster
-service port(multiple)|service.ports.port|instance.extendData
-pod ip|pod.status.hostIP / service.ipFamilies|instance.ip
+|K8S Data to be Synchronized| K8S Field| Mapped to Nacos Field|
+|---|---|---|
+|Service Name| service.metadata.name| service.name|
+|Pod Target Port(s)| service.ports.targetPort| instance.port|
+|Service Name| service.metadata.name| instance.cluster|
+|Service Port(s)| service.ports.port| instance.extendData|
+|Pod IP| pod.status.hostIP or service.ipFamilies| instance.ip|
-## Configuration file
-Deploy the Nacos cluster according to [the deploy document](../guide/admin/deployment.md)
+## Configuration
+Follow the [Deployment Documentation](../guide/admin/deployment.md) to set up the Nacos cluster.
-Configure the application.properties file to enable K8S synchronization:
-```
+Modify the application.properties file to enable K8S synchronization:
+```properties
nacos.k8s.sync.enabled=true
```
-If you are using the Java API from an application outside the K8S cluster, you need to specify kubeConfig:
-```
+For applications outside the K8S cluster using the Java API, specify the kubeConfig:
+```properties
nacos.k8s.sync.outsideCluster=true
nacos.k8s.sync.kubeConfig=/.kube/config
```
-After configuration, services and instance changes in K8S are automatically synchronized to Nacos.
+Upon configuration, changes in services and instances within K8S will automatically synchronize to Nacos.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/guide/admin/cluster-mode-quick-start.md b/src/content/docs/latest/en/guide/admin/cluster-mode-quick-start.md
index dd50f34e954..dd34f98b66d 100644
--- a/src/content/docs/latest/en/guide/admin/cluster-mode-quick-start.md
+++ b/src/content/docs/latest/en/guide/admin/cluster-mode-quick-start.md
@@ -1,45 +1,50 @@
---
-title: Cluster deployment instructions
-keywords: [Cluster,deployment]
-description: Cluster deployment instructions
-sidebar:
- order: 2
+title: Cluster Deployment Guide
+keywords: [Cluster Deployment, Nacos, Cluster Mode, Port Configuration, Data Source Configuration, Service Registration and Discovery, Configuration Management, Authentication]
+description: This guide details the deployment process of Nacos in cluster mode, covering cluster architecture, environment setup, obtaining source code or packages, cluster configuration, data source setup, and comprehensive steps for service registration, discovery, and configuration management. It emphasizes port configurations for different deployment modes, usage of internal and external data sources, and provides examples for configuring authentication.
---
-
# Cluster deployment instructions
> Document optimizing...
-## Cluster Mode Deployment
+# Cluster Deployment Guide
-This Quick Start Manual is to help you quickly download, install and use Nacos on your computer to deploy the cluster mode for production use.
+This quick start manual assists you in rapidly downloading, installing, and using Nacos in a production-ready clustered mode on your computer.
-### Cluster Deployment Architecture
+## Cluster Deployment Architecture
-Therefore, when it is open source, it is recommended that users put all server lists under a vip and then hang under a domain name.
+Open-source recommendations include placing all service lists under a VIP and associating it with a domain name.
-Http://ip1:port/openAPI Directly connected to ip mode, the machine needs to be modified to use ip.
+- ``: Direct IP mode requires IP modification when a machine fails.
+- ``: SLB (intranet) mounting mode is not to be exposed to the public network due to security risks.
+- ``: Domain + SLB (intranet) mode is recommended for its readability and easy IP swapping.
-Http://SLB:port/openAPI Mount the SLB mode(Intranet, do not expose internet to avoid security risks), directly connect to SLB, the following server ip real ip, readability is not good.
+
-Http://nacos.com:port/openAPI Domain name + SLB mode(Intranet, do not expose internet to avoid security risks), good readability, and easy to change ip, recommended mode
+|Port| Offset from Main Port| Description|
+|--|--|--|
+|8848|0| Primary port used by clients, console, and OpenAPI for HTTP requests.|
+|9848|1000| gRPC client request to server port, for client-initiated connections and requests.|
+|9849|1001| Server-to-server gRPC request port for service synchronization, etc.|
+|7848|-1000| Jraft request to server port, for handling Raft-related requests among servers.|
-
+**When using VIP/nginx, configure TCP forwarding instead of http2 to prevent connection termination by nginx.**
+**Ports 9849 and 7848 are for inter-service communication; do not expose them to external networks or clients.**
-## 1. Preparing for the Environment
+## 1. Prerequisite Environment Preparation
-Make sure that it is installed and used in the environment:
+Ensure the following are installed and used:
-1. 64 bit OS Linux/Unix/Mac, recommended Linux system.
-2. 64 bit JDK 1.8+; [Download](http://www.oracle.com/technetwork/java/javase/downloads/jdk8-downloads-2133151.html). [Configuration](https://docs.oracle.com/cd/E19182-01/820-7851/inst_cli_jdk_javome_t/).
-3. Maven 3.2.x+; [Download](https://maven.apache.org/download.cgi). [Configuration](https://maven.apache.org/settings.html).
-4. 3 or more Nacos Nodes;
+- 64-bit OS: Linux/Unix/Mac, Linux is recommended.
+- 64-bit JDK 1.8+; [Download](http://www.oracle.com/technetwork/java/javase/downloads/jdk8-downloads-2133151.html). [Configuration](https://docs.oracle.com/cd/E19182-01/820-7851/inst_cli_jdk_javahome_t/).
+- Maven 3.2.x+; [Download](https://maven.apache.org/download.cgi). [Configuration](https://maven.apache.org/settings.html).
+- A minimum of 3 Nacos nodes to form a cluster.
-## 2. Download source code or installation package
+## 2. Obtain Source Code or Installation Package
-You can get Nacos in two ways.
+Nacos can be obtained through two methods.
-### Download source code from Github
+### Download Source Code from GitHub
```bash
unzip nacos-source.zip
@@ -48,20 +53,18 @@ mvn -Prelease-nacos clean install -U
cd nacos/distribution/target/nacos-server-1.3.0/nacos/bin
```
-### Download Compressed Packet after Compilation
-
-Download address
+### Download Compiled Package
-Select the latest stable version from [releases](https://github.com/alibaba/nacos/releases) and download `nacos-server-$version.zip` or `nacos-server-$version.tar.gz`
+Download from the [latest stable version](https://github.com/alibaba/nacos/releases) `nacos-server-$version.zip` or `nacos-server-$version.tar.gz`.
```bash
- unzip nacos-server-$version.zip OR tar -xvf nacos-server-$version.tar.gz
- cd nacos/bin
-```
+unzip nacos-server-$version.zip or tar -xvf nacos-server-$version.tar.gz
+cd nacos/bin
+```
-## 3. Configuration Cluster Profile
+## 3. Configure Cluster Configuration Files
-In the Nacos decompression directory Nacos / conf directory, there is a configuration file cluster. conf, please configure each line as ip: port.
+Under the `conf` directory in the extracted `nacos/`, edit `cluster.conf` with each line as `ip:port` (minimum 3 nodes).
```plain
# ip:port
@@ -70,61 +73,61 @@ In the Nacos decompression directory Nacos / conf directory, there is a configur
200.8.9.18:8848
```
-### 3.1 Open Default auth plugin (Optional)
+### 3.1 Enable Default Authentication Plugin (Optional)
-Then Setting configuration file `application.properties` under `conf`.
+Modify `application.properties` in the `conf` directory.
-Setting
+Set:
```properties
nacos.core.auth.enabled=true
nacos.core.auth.system.type=nacos
-nacos.core.auth.plugin.nacos.token.secret.key=${custom, make sure same in all nodes}
-nacos.core.auth.server.identity.key=${custom, make sure same in all nodes}
-nacos.core.auth.server.identity.value=${custom, make sure same in all nodes}
+nacos.core.auth.plugin.nacos.token.secret.key=${custom, ensure consistency across all nodes}
+nacos.core.auth.server.identity.key=${custom, ensure consistency across all nodes}
+nacos.core.auth.server.identity.value=${custom, ensure consistency across all nodes}
```
-Detail see [Authentication](../../plugin/auth-plugin.md).
-> Attention,Default value in Document `SecretKey012345678901234567890123456789012345678901234567890123456789` and `VGhpc0lzTXlDdXN0b21TZWNyZXRLZXkwMTIzNDU2Nzg=` is a public default, **only** should use in test temporary. Please **make sure** to replace it with another valid value when you actually deploy.
+For more details, refer to [Authentication](../../plugin/auth-plugin.md).
+
+> Note: The default values `SecretKey012345678901234567890123456789012345678901234567890123456789` and `VGhpc0lzTXlDdXN0b21TZWNyZXRLZXkwMTIzNDU2Nzg=` are public defaults, suitable for temporary testing. Replace with custom values for actual use.
-## 4. Determine The DataSource
+## 4. Determine Data Source
-### Using built-in data sources
+### Use Built-in Data Source
-No configuration is required
+No configuration required.
-### Use an external data source
+### Use External Data Source
-
-production and use recommendations at least backup mode, or high availability database.
+Recommended for production in at least master-slave mode or with a highly available database.
-#### Initializes the MySQL database
+#### Initialize MySQL Database
-[sql statement source file](https://github.com/alibaba/nacos/blob/master/distribution/conf/mysql-schema.sql)
+[SQL script source](https://github.com/alibaba/nacos/blob/master/distribution/conf/mysql-schema.sql)
-### application. properties configuration
+#### application.properties Configuration
-[application.properties configuration file](https://github.com/alibaba/nacos/blob/master/distribution/conf/application.properties)
+[application.properties file](https://github.com/alibaba/nacos/blob/master/distribution/conf/application.properties)
-## 5. start server
+## 5. Start Server
### Linux/Unix/Mac
-#### Standalone mode
+#### Standalone Mode
```bash
sh startup.sh -m standalone
```
-#### Cluster mode
+#### Cluster Mode
-> Using built-in data sources
+> Built-in Data Source
```bash
sh startup.sh -p embedded
```
-> Use an external data source
+> External Data Source
```bash
sh startup.sh
@@ -132,34 +135,31 @@ sh startup.sh
## 6. Service Registration & Discovery and Configuration Management
-### Service registration
-
-`curl -X POST 'http://127.0.0.1:8848/nacos/v1/ns/instance?serviceName=nacos.naming.serviceName&ip=20.18.7.10&port=8080'`
-
-> Attention: If open default auth plugin, please call with username and password in header.
-
-### Service discovery
-
-`curl -X GET 'http://127.0.0.1:8848/nacos/v1/ns/instance/list?serviceName=nacos.naming.serviceName'`
+### Service Registration
-> Attention: If open default auth plugin, please call with username and password in header.
+```bash
+curl -X POST 'http://127.0.0.1:8848/nacos/v1/ns/instance?serviceName=nacos.naming.serviceName&ip=20.18.7.10&port=8080'
+```
-### Publish configuration
+> If the default authentication plugin is enabled, include credentials in the Header.
-`curl -X POST "http://127.0.0.1:8848/nacos/v1/cs/configs?dataId=nacos.cfg.dataId&group=test&content=helloWorld"`
+### Service Discovery
-> Attention: If open default auth plugin, please call with username and password in header.
+```bash
+curl -X GET 'http://127.0.0.1:8848/nacos/v1/ns/instance/list?serviceName=nacos.naming.serviceName'
+```
-### get configuration
+> If the default authentication plugin is enabled, include credentials in the Header.
-`curl -X GET "http://127.0.0.1:8848/nacos/v1/cs/configs?dataId=nacos.cfg.dataId&group=test"`
+### Publish Configuration
-> Attention: If open default auth plugin, please call with username and password in header.
+```bash
+curl -X POST "http://127.0.0.1:8848/nacos/v1/cs/configs?dataId=nacos.cfg.dataId&group=test&content=helloWorld"
+```
-## 7. shut down server
+> If the default authentication plugin is enabled, include credentials in the Header.
-### Linux/Unix/Mac
+### Retrieve Configuration
```bash
-sh shutdown.sh
-```
+curl -X GET "http://127.0.0.1:8848/nacos/v1/cs/configs?dataId=nacos.cfg.dataId&group=test
\ No newline at end of file
diff --git a/src/content/docs/latest/en/guide/admin/console-guide.md b/src/content/docs/latest/en/guide/admin/console-guide.md
index 45a31d4a109..486bd204262 100644
--- a/src/content/docs/latest/en/guide/admin/console-guide.md
+++ b/src/content/docs/latest/en/guide/admin/console-guide.md
@@ -1,181 +1,86 @@
---
-title: Console Guide
-keywords: [console,guide]
-description: Nacos console aims to enhance the console for service list, health management, service management, a distributed configuration management control ability.
-sidebar:
- order: 5
+title: 控制台手册
+keywords: [Nacos Console, Service Management, Configuration Management, Namespace Management, Login Management, Microservices Governance, Distributed Configuration]
+description: The Nacos console is designed to enhance microservices management, encompassing service list monitoring, health status control, traffic weight adjustment, multi-format configuration editing with version control, namespace isolation, and secure login mechanisms, streamlining service governance and configuration management while reducing operational overhead.
---
-# Console Guide
+# Control Panel Manual
-[Nacos console](http://console.nacos.io/nacos/index.html) aims to enhance the console for service list, health management, service management, a distributed configuration management control ability, in order to help users reduce the cost of micro management service application architecture, will provide basic functions include the following:
+The [Nacos Control Panel](http://console.nacos.io/nacos/index.html) primarily focuses on augmenting control capabilities in areas such as service listings, health status management, service governance, and distributed configuration management, further assisting users in lowering the costs associated with managing microservice architectures. It offers fundamental functions including:
-* Service management
- * Service list and health status display
- * Service metadata storage and editing
- * Service flow weight adjustment
- * Service elegant line up and down
-* Configuration management
- * More configuration format editing
- * Edit DIFF
- * Sample code
- * Push status query
- * Configure version and rolled back
-* Namespace
-* Login management
+- **Service Management**
+ - Display of service lists and health statuses.
+ - Storage and editing of service metadata.
+ - Adjustment of service traffic weights.
+ - Graceful online/offline service handling.
+- **Configuration Management**
+ - Editing of various configuration formats.
+ - Edit DIFF review.
+ - Sample code provision.
+ - Push status query.
+ - Version control and one-click rollback for configurations.
+- **Namespace**
+- **Login Administration**
-## Features
+## Detailed Features
-### Service management
+### Service Management
-Developer or operations staff often require after service registry, through friendly interface to view the service registration situation, the current system, including the registration of all of the details of the services and each service.And in a case, with access control service of some of the configuration editor.Nacos in this version of open service found that part of the console, main is to provide users a basic operations page, to view, edit, the current registration services.
+Developers or operations personnel often require a user-friendly interface post-service registration to view service registration status, including all registered services and detailed information for each. With permission controls in place, Nacos's console service discovery section provides a basic operation page enabling users to view and edit registered services.
-#### Service list management
+#### Service List Management
-Service list to help users with a unified view management of all its service and health status.The overall layout is the upper left corner services and search box to search button, the page is the central service list.Service main display service name list, the cluster number, number of instances, health instance number and details button five columns.
+The service list facilitates unified management of all microservices and their health statuses through a layout featuring a search box and button in the upper left corner, with the service list displayed centrally. It showcases service names, cluster counts, instance counts, healthy instance numbers, and detail buttons.
-
+#### Traffic Weight Support and Protection
-In the service list page click details, you can see details of the service.Can look at the service, the basic information of the cluster and examples.
+Nacos equips users with traffic weighting controls and opens thresholds for service traffic protection, safeguarding service provider clusters from unexpected overload. As depicted, by clicking an instance's edit button, its weight can be adjusted; increasing the weight to boost traffic or setting it to zero to halt traffic reception.
-#### Service flow weighted support and protection
+#### Service Metadata Management
-Nacos flow provides the user with the ability of weight control, open the threshold of service flow protection at the same time, in order to help users better protection service cluster service providers are not accidentally break.The diagram below so, click the edit button instance, modify instance weights.If you want to increase the flow of instance, to turn up the weight, if you don't want to flow method receives the instance, the weight can be set to 0.
+Nacos exposes service metadata across multiple dimensions for storing custom information in a K-V structure. On the control panel, this data is presented and edited following a similar format, e.g., editing service metadata involves clicking the 'Edit Service' button on the top right corner of the service details page and inputting: `version=1.0,env=prod`.
-
+#### Graceful Service Online/Offline
-#### Service metadata management
+Nacos also supports the online/offline operations of service instances, accessible via the 'Online' or 'Offline' buttons on the service details page. Offline instances are excluded from the healthy instance list.
-Nacos provide multiple dimensions of service metadata exposed, help users to store the information of the custom.This information is based on data storage structure, K - V on the console, as to the k1 = v1, k2 = v2 show such format.Similarly, edit the metadata can be performed by the same format.Such as service metadata editing, first click on the service details in the top right corner of the page "edit service" button, and then in the metadata input: input box version = 1.0, env = prod.
+### Configuration Management
-
+Nacos supports configuration grouping management based on Namespaces and Groups, allowing users to manage numerous configurations flexibly per environment or application/module. Key management capabilities include configuration history, rollback, and subscriber querying.
-Click on the confirmation, you can in the service details page, see the service metadata has been updated.
+#### Multi-Format Configuration Editor
-
-
-#### Service elegant line up and down
-
-Nacos also offers the service instance line operation, up and down in the service details page, you can click on the instance of "on-line" or "off" button, the offline instance, cases of health will not be included in the list.
-
-
-
-### Configuration management
-
-Nacos support Group configuration based on the Namespace and Group management, so that users more flexible according to their own needs in accordance with the environment or application, module, such as grouping management services as well as the configuration of Spring, in the configuration management major provides configuration version history, rollback, subscriber query such as the core management abilities.
-
-
-
-#### More configuration format editor
-
-Nacos support YAML, Properties, TEXT, JSON, XML, HTML and other common configuration format online editing, syntax highlighting, format check, help users efficiently edit at the same time greatly reduced the risks of format error.
-
-Nacos support configuration tag ability, help users better and more flexible to the configuration of the classification and management based on the tag.Description of configuration and its change is support users at the same time, people or cross team collaboration management configuration.
-
-
+Supporting formats like YAML, Properties, TEXT, JSON, XML, HTML, Nacos provides online editing, syntax highlighting, and format validation, enhancing editing efficiency while minimizing formatting errors.
#### Edit DIFF
-Nacos supports editing a DIFF ability, help the user to check the changes, and reduce the risks of correction.
-
-
-
-#### Sample code
-
-Nacos provide sample code ability, can let a novice quickly using client-side programming consumption this configuration, novice slash barriers.
-
-
-
-
-
-#### Listener query
-
-Nacos provide configuration subscriber is the listener query ability, at the same time provide Client MD5 checksum value of the current configuration, in order to help users better check configuration changes pushed to the Client side.
-
-
-
-#### Configure version and rolled back
-
-Nacos by providing a key roll back configuration version management and its ability, help users can configure to quick recovery, reduce the micro service system in configuration management will meet the availability of the risk.
-
-
-
-
-
-## Namespace management
-
-Nacos based in Namespace helps users logic isolation based multiple namespaces, this can help users better management testing, service and configure the pretest, production environment, so that the same configuration environment (such as database data sources) can define different values.
-
-
-
-
-
-## Login management
-
-Nacos 0.8 version supports simple login function, the default username/password for: `nacos/nacos`.
-
-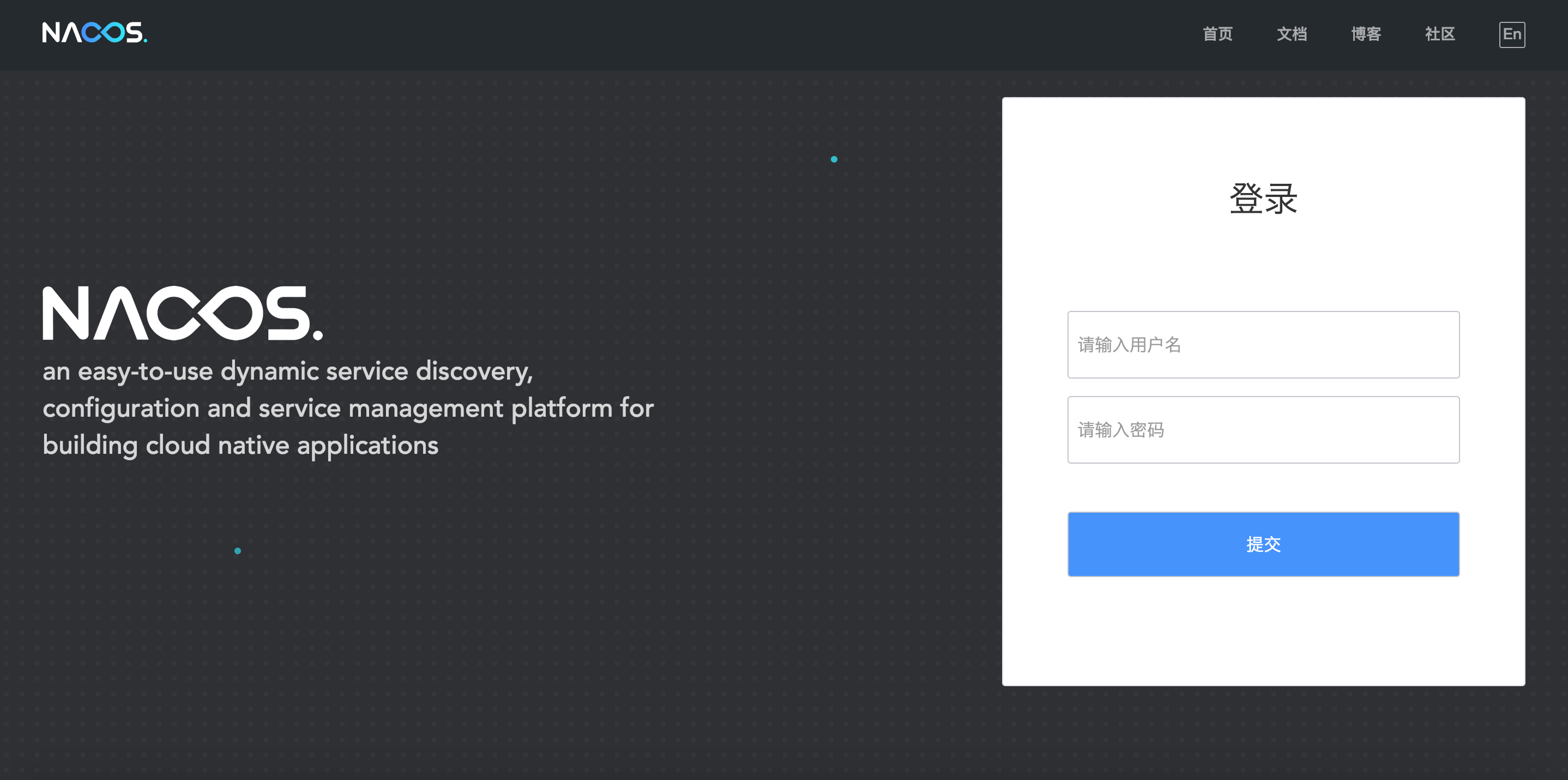
-
-### Change the default username/password method
-
-1. Generate encrypted password in `com.alibaba.nacos.console.utils.PasswordEncoderUtil.main` function, change nacos to you want to change the password, running with encryption algorithm.Note that salt is random, so the generated password every time may be different, please don't worry about it.
-
-```
-public class PasswordEncoderUtil {
-
- public static void main(String[] args) {
- System.out.println(new BCryptPasswordEncoder().encode("nacos"));
- }
-}
-```
-
-2. Create a user name or password, use specify a user name password.
-```
-INSERT INTO users (username, password, enabled) VALUES ('nacos', '$2a$10$EuWPZHzz32dJN7jexM34MOeYirDdFAZm2kuWj7VEOJhhZkDrxfvUu', TRUE);
-INSERT INTO roles (username, role) VALUES ('nacos', 'ROLE_ADMIN');
-```
-
-### Close the login function
-
-As part of its own development console, do not want to be nacos security filter interceptor.Therefore nacos support custom close the login functionFind the configuration file `${nacoshome}/conf/application.properties`. The properties, replace the following content.
-
-```
-## spring security config
-### turn off security
-spring.security.enabled=false
-management.security=false
-security.basic.enabled=false
-nacos.security.ignore.urls=/**
-
-#nacos.security.ignore.urls=/,/**/*.css,/**/*.js,/**/*.html,/**/*.map,/**/*.svg,/**/*.png,/**/*.ico,/console-fe/public/**,/v1/auth/login,/v1/console/health,/v1/cs/**,/v1/ns/**,/v1/cmdb/**,/actuator/**
-
-```
+Nacos's edit DIFF capability aids in verifying changes, mitigating risks from erroneous modifications.
-### Session time
+#### Sample Code
-The default session to keep time for 30 minutes.After 30 minutes need to login authentication.Temporarily does not support to modify the default time.
+Nacos furnishes sample code capabilities, enabling novices to swiftly consume configurations with client-side programming, significantly reducing the learning curve.
-## Community participation in the front end of the building
+#### Subscriber Query
-In Nacos front style, the layout of the discussion, the community vote, finally choose the style of the classic black and white and blue skin, and through our UED Yao Cheng design, layout, make interaction is very natural.
+Nacos enables querying of configuration subscribers along with providing the MD5 checksum of the current client configuration, assisting in verifying whether configuration changes have been pushed to clients.
-In the development of the console, we recruited through community many front students to participate in the development of the front-end code, in this especially thank Chen Li, Qing Wang, Yanmin Wang Nacos front-end development process in the strong support!
+#### Configuration Versioning and One-Click Rollback
-## Adhere to the community development, welcome to join and contribute to the community
+With version management and one-click rollback features, Nacos ensures rapid recovery from misconfigurations, reducing availability risks inherent in configuration management.
-> DISS is cheap, show me your hand!
+### Additional Features
-To join Nacos WeChat community discussion Nacos the evolution of the product, you can sweep through **xuechaos** WeChat QRcode, let "xuechaos" help you pull in "Nacos community communication group".
+ namespaces for logical isolation across environments, login management with default credentials, session timeouts, and community-driven frontend enhancements. Users can also disable the default console or customize login behavior with authentication plugins.
-
+Nacos continues to evolve through community participation, welcoming contributions to its development and fostering a vibrant ecosystem around microservices and distributed systems management.
-More Nacos related open source project information:
+For more information on Nacos-related open-source projects, visit:
-* [Nacos](https://github.com/alibaba/nacos)
-* [Nacos Spring Project](https://github.com/nacos-group/nacos-spring-project)
-* [Nacos Spring Boot](https://github.com/nacos-group/nacos-spring-boot-project)
-* [Spring Cloud Alibaba](https://github.com/spring-cloud-incubator/spring-cloud-alibaba)
-* [Dubbo](https://github.com/apache/dubbo)
-* [Sentinel](https://github.com/alibaba/Sentinel)
-* [Spring Cloud](https://projects.spring.io/spring-cloud/)
-* [Nepxion Discovery](https://github.com/Nepxion/Discovery)
+- [Nacos](https://github.com/alibaba/nacos)
+- [Nacos Spring Project](https://github.com/nacos-group/nacos-spring-project)
+- [Nacos Spring Boot](https://github.com/nacos-group/nacos-spring-boot-project)
+- [Spring Cloud Alibaba](https://github.com/spring-cloud-incubator/spring-cloud-alibaba)
+- [Dubbo](https://github.com/apache/dubbo)
+- [Sentinel](https://github.com/alibaba/Sentinel)
+- [Spring Cloud](https://projects.spring.io/spring-cloud/)
+- [Nepxion Discovery](https://github.com/Nepxion/Discovery)
\ No newline at end of file
diff --git a/src/content/docs/latest/en/guide/admin/management-api.md b/src/content/docs/latest/en/guide/admin/management-api.md
index 7672c8dc1fb..5a83c1750fd 100644
--- a/src/content/docs/latest/en/guide/admin/management-api.md
+++ b/src/content/docs/latest/en/guide/admin/management-api.md
@@ -1,11 +1,9 @@
---
title: Management API
-keywords: [Management,API]
-description: In plan with Nacos 1.x.x
-sidebar:
- order: 4
+keywords: [Management API, Nacos 1.x.x, Documentation Optimization]
+description: This document pertains to the introduction of Management API within Nacos 1.x.x version, currently undergoing documentation optimization and is pending completion.
---
-> Document optimizing...
+> Documentation under optimization...
-TODO
+TODO
\ No newline at end of file
diff --git a/src/content/docs/latest/en/guide/user/failover.md b/src/content/docs/latest/en/guide/user/failover.md
index 2379a2abf1f..d5e1edd7b4b 100644
--- a/src/content/docs/latest/en/guide/user/failover.md
+++ b/src/content/docs/latest/en/guide/user/failover.md
@@ -1,146 +1,97 @@
---
-title: Java Client Failover
-keywords: [Failover]
-description: Java client failover user guide
-sidebar:
- order: 7
- hidden: true
+title: Java Client Disaster Recovery
+keywords: [Java Client Disaster Recovery, Data Source for Disaster Recovery, Disk Disaster Recovery, SPI Extension, Nacos Server Issues]
+description: This article delves into the disaster recovery mechanisms for Java clients, explaining how to maintain client stability when facing Nacos server issues by启用 local disaster recovery and leveraging cached data. It elaborates on two usage scenarios, the disaster recovery process, specifics of disk disaster recovery implementation, and the method for customizing and extending disaster recovery data sources.
---
-# Java Client Failover
+# Java Client Disaster Recovery
-We can turn on the local data failover feature to handle the situation when Nacos server side is unstable or has problematic data.
+We can enable local disaster recovery on the client side to ensure data and API stability when the Nacos server encounters issues.
-There are two typical scenarios:
+There are two application scenarios:
-1. When Nacos server is in deployment, we can switch on the failover so the clients use local data only. The data anomaly or oscillation at Nacos server won't affect the clients. After the deployment and the data verification are done, we can switch off the failover feature.
-2. When there is a sudden data anomaly at Nacos server at runtime, we can turn on the failover feature to prevent Nacos clients using wrong data.
+1. During Nacos server deployment, we proactively activate disaster recovery, allowing clients to solely use local disaster recovery data. This ensures that fluctuations or errors in Nacos server data do not affect clients. We then disable disaster recovery after the upgrade is complete and data validation is successful.
+2. If Nacos services unexpectedly become unavailable or exhibit data anomalies during operation, we can swiftly enable disaster recovery, enabling clients to utilize this backup data. This minimizes the impact window on services until the Nacos server is restored and disaster recovery can be deactivated.
-The full detailed solution description can be found in https://github.com/alibaba/nacos/issues/11053
+Refer to https://github.com/alibaba/nacos/issues/11053 for a detailed solution.
-## Procedures
+## Brief Process Overview
-
+[Image Description: Illustrates the flow where client queries first pass through the FailoverReactor. If data is available, it is used directly, bypassing data from the Nacos Server; otherwise, the normal process of fetching data from ServiceInfoHolder proceeds.]
-As shown above, the query requests to Nacos client would first be checked by FailoverReactor, and only if FailoverReactor has no related data, can the requests move on to query ServiceInfoHolder.
+## Disk Disaster Recovery
-## Disk based Failover
+The data within the FailoverReactor can leverage different data sources, with the default being disk storage.
-FailoverReactor can select different data sources. Disk is the default option.
+### Disk Disaster Recovery Directory
-### Disk Failover File Path
-
-The default path of disk failover files are:
+The default directory for disk disaster recovery files is:
```
{user.home}/nacos/naming/{namespace}/failover
```
-This path can be customised via -D argument:
-
-```
--DJM.SNAPSHOT.PATH=/mypath
-```
-
-So the path becomes:
+This directory can be customized using the `-DJM.SNAPSHOT.PATH=/mypath` parameter, changing the disaster recovery disk file directory to:
```
/mypath/nacos/naming/{namespace}/failover
```
-### Disk Failover Switch
+### Disaster Recovery Switch on Disk
-The disk failover switch is stored in a file with name:
+The disaster recovery switch resides in a file within the disk disaster recovery directory, named:
```
00-00---000-VIPSRV_FAILOVER_SWITCH-000---00-00
```
-The content of this file is just a number 0 or 1, where 0 represents failover is off, 1 is on.
-
-### Disk Failover Data
+The file contains a digit, 0 for disabling or 1 for enabling disaster recovery.
-The disk failover data is stored in multiple files under the failover path. Each file stores the failover data for a single service.
+### Disk Disaster Recovery Data
-The file name is in the following format:
+Disaster recovery data is divided across multiple files stored in the disk disaster recovery directory. Each file contains the disaster recovery data for a single service, with filenames formatted as:
```
{group.name}%40%40{service.name}
```
-The content in the file is the JSON string of one ServiceInfo object, for instance:
-```
-{
- "name":"DEFAULT_GROUP@@test.2",
- "groupName":"DEFAULT_GROUP",
- "clusters":"",
- "cacheMillis":10000,
- "hosts":[
- {
- "instanceId":"1.1.2.1#8888#DEFAULT#DEFAULT_GROUP@@test.2",
- "ip":"1.1.2.1",
- "port":8888,
- "weight":1,
- "healthy":true,
- "enabled":true,
- "ephemeral":true,
- "clusterName":"DEFAULT",
- "serviceName":"DEFAULT_GROUP@@test.2",
- "metadata":{
- "k1":"v1"
- },
- "instanceHeartBeatInterval":5000,
- "instanceHeartBeatTimeOut":15000,
- "ipDeleteTimeout":30000
- }
- ],
- "lastRefTime":1689835375819,
- "checksum":"",
- "allIPs":false,
- "reachProtectionThreshold":false,
- "valid":true
-}
-```
+Content includes a JSON serialized string of the ServiceInfo class.
-## Extent Failover Data Source
+## Expanding Disaster Recovery Data Sources
-Disk failover is simple and requires no extra remote components. But sometimes we may want to use another kind of data source, such as Redis, Mysql, etc.
+While disk-based disaster recovery is dependency-free and simple, managing it can be cumbersome. Therefore, we also support SPI extensions for using storage beyond disk. Below are the steps for such an extension.
-Now we support extending the failover data source with SPI mechanism. Here are the steps:
+### Developing a Custom Disaster Recovery Data Source Class
-### Develop Your Own Failover Data Source
+Implement the interface `com.alibaba.nacos.client.naming.backups.FailoverDataSource`:
-Write a class and implement the interface com.alibaba.nacos.client.naming.backups.FailoverDataSource:
-
-```
+```java
public class MyFailoverDataSource implements FailoverDataSource {
-
@Override
public FailoverSwitch getSwitch() {
- // TODO write your own implementation.
+ // Implement your logic here.
return null;
}
-
+
@Override
public Map getFailoverData() {
- // TODO write your own implementation. For naming module, the map
- // should contain failover data with service name as key and ServiceInfo as value
+ // Provide your implementation. For the naming module, the map should associate service names with ServiceInfo instances.
return null;
}
}
```
-### Configure Failover Data Source Class
+### Configuring the Disaster Recovery Data Source Class
-Create a file under the resource root path:
+Create a file under the resource directory:
```
{resource.root}/META-INF/services/com.alibaba.nacos.client.naming.backups.FailoverDataSource
```
-One example of `{resource.root}` is src/main/resources.
+An example of {resource.root} is `src/main/resources`.
-The file content is:
+File content should be:
```
your.package.MyFailoverDataSource
diff --git a/src/content/docs/latest/en/plugin/address-plugin.md b/src/content/docs/latest/en/plugin/address-plugin.md
index 47db604d127..78a57962bce 100644
--- a/src/content/docs/latest/en/plugin/address-plugin.md
+++ b/src/content/docs/latest/en/plugin/address-plugin.md
@@ -1,26 +1,22 @@
---
title: Addressing Plugin
-keywords: [Addressing, Plugin]
-description: This article describes how to develop and use Nacos' addressing plugin.
-sidebar:
- order: 2
- hidden: true
+keywords: [Nacos, Addressing Plugin, SPI, Server-side, Client-side, Custom Plugin]
+description: This article elaborates on the support for cluster addressing plugins in Nacos starting from version 2.3.0, including steps to develop server and client-side addressing plugins, implementation details of interfaces, and how to configure and enable these plugins. It also alerts developers that the addressing plugin is currently in Beta, with potential API changes in future updates.
---
-
# Addressing Plugin
-Since version 2.3.0, Nacos support to inject addressing plugins through [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html), and select a plugin implementation in the configuration file `application.properties ` as the actual addressing service. This document will describe how to implement an addressing plugin and how to make it work.
+Starting with version 2.3.0, Nacos supports injecting cluster addressing-related plugins through the [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html) mechanism and selecting one of the plugin implementations in the `application.properties` configuration file for actual addressing services. This document will provide a comprehensive guide on implementing an addressing plugin and making it effective.
-> Attention:
-> At present, the addressing plugin is still in the beta stage, and its API and interface definitions maybe modified with version upgrades. Please pay attention to the applicable version of your plugin.
+> Note:
+> The addressing plugin is currently in Beta testing, and its APIs and interface definitions may change with subsequent version upgrades. Please ensure your plugin compatibility accordingly.
-## Overview Of Addressing Plugin
+## Overview of Addressing Plugins
-At present, there are three addressing modes for Nacos cluster addressing: stand-alone addressing, profile addressing and address server addressing. Through the addressing plugin, users can write their own addressing logic.
+Currently, Nacos offers three addressing methods: single-machine addressing, configuration file addressing, and address server addressing. With addressing plugins, users can write their own addressing logic.
-## Develop Nacos Server Addressing Plugin
+## Developing Nacos Server-Side Addressing Plugin
-To develop a Nacos server-side addressing plugin, developer first need to depend on the relevant API of the address plugin.
+To develop a Nacos server-side addressing plugin, first, depend on the relevant API for addressing plugins:
```xml
@@ -30,57 +26,61 @@ To develop a Nacos server-side addressing plugin, developer first need to depend
```
-`${project.version}` is the version of Nacos for your development plugin.
+Where `${project.version}` corresponds to the Nacos version you are developing the plugin against.
-Then implement interface`com.alibaba.nacos.plugin.address.spi.AddressPlugin`, and put your implementation into services of SPI.
+Then, implement the `com.alibaba.nacos.plugin.address.spi.AddressPlugin` interface and add your implementation to the SPI's services.
-The methods of interface in following:
+Methods to implement within the interface include:
-|method name|parameters|returns|description|
+|Method Name|Input|Return|Description|
|-----|-----|-----|---|
-|start|void|String|Start the addressing function of the plugin.|
-|getServerList|void|List<String>|Returns the addresses of all Nacos cluster nodes. The address format is`IP: Port`.|
-|getPluginName|void|String|The name of the plugin. When the name is the same, the plugin loaded later will overwrite the plugin loaded first.|
-|registerListener|Consumer<List<String>>|AddressPlugin|Register the listener and call the listener when the cluster address changes|
-|shutdown|void|void|Shutdown plugin|
-
-This interface is defined by `com.alibaba.nacos.plugin.address.spi.AbstractAddressPlugin`.The abstract class implements`getServerList`, `registerListener` and `shutdown` methods by default, Users can inherit AbstractAddressPlugin to implement other methods when actually writing plugins. AbstractAddressPlugin has a List<String>member variable named serverList, that is, the cluster address collection. The user needs to maintain this variable.
-When users need to configure plugin related parameters in the configuration file, they need to configure keys starting with `address.plugin` in the property configuration file. In this case, the corresponding parameters can be obtained through the `com.alibaba.nacos.plugin.address.common.AddressProperties` singleton class
+|start|void|void|Initiates the addressing function of the plugin.|
+|getServerList|void|List|Returns all Nacos cluster node addresses in the format `IP:Port`.|
+|getPluginName|void|String|The name of the plugin; when names clash, the later-loaded plugin overrides the earlier one.|
+|registerListener|Consumer>|AddressPlugin|Registers a listener called when cluster addresses change.|
+|shutdown|void|void|Disables the plugin.|
+
+The `com.alibaba.nacos.plugin.address.spi.AbstractAddressPlugin` abstract class provides default implementations for `getServerList`, `registerListener`, and `shutdown`. Users extending `AbstractAddressPlugin` only need to implement the remaining methods. The `AbstractAddressPlugin` has a `serverList` member variable (List) representing the cluster address collection, which users must maintain after calling the start method.
+
+For configuring plugin-related parameters in the property file, use keys prefixed with `address.plugin`, accessible via the `com.alibaba.nacos.plugin.address.common.AddressProperties` singleton:
+
```properties
-address.plugin.$ {key} = ${val}
+address.plugin.${key} = ${val}
```
-After configuration, users can write plugins through the
+
+Post-configuration, developers can retrieve parameters through:
+
```java
AddressProperties.getProperty(${key})
```
-To get the parameters.
-### Use Server Plugin
+### Using Server-Side Plugins
-After the plugin finished, it needs to be packaged into jar/zip and places in the classpath of the nacos server. If you don't know how to add plugins into the classpath, please place plugins under `${nacos-server.path}/plugins` directly.
+After development, package the plugin as a jar/zip and place it in the Nacos server's classpath or directly into `${nacos-server.path}/plugins`.
-After Adding plugins into classpath, also need to modify some configuration in `${nacos-server.path}/conf/application.properties`.
+Modify `${nacos-server.path}/conf/application.properties`:
```properties
-### The plugin name nacos using,should be same as the return value of `com.alibaba.nacos.plugin.address.spi.AddressPlugin#getPluginName`
+### Enabled Nacos addressing plugin name, corresponding to `com.alibaba.nacos.plugin.address.spi.AddressService#getPlugin` return value
nacos.core.member.lookup.type=${addressPluginName}
```
-Restart nacos cluster, and after any request, some logs can be saw in `${nacos-server.path}/logs/nacos-cluster.log`:
+Restart Nacos cluster, and upon receiving requests, observe logs in `${nacos-server.path}/logs/nacos-cluster.log`:
```text
[AddressPluginManager] Load AddressPlugin(xxxx) PluginName(xxx) successfully.
```
-### Use the default Nacos addressing plugin
+### Leveraging Built-in Nacos Addressing Plugins
+
+For backward compatibility, without custom plugins, configurations remain unchanged with `nacos.core.member.lookup.type=[file,address-server]`.
-In order to be compatible with the addressing of the old version, when the user does not use the custom plug-in, the configuration is the same as the original, or the configuration item `nacos.core.member.lookup.type=[file, address server]`.
+## Client-Side Plugins
-## Client Plugin
+### Implementing Custom Plugins
+
+Nacos client-side users follow similar steps as the server-side for custom addressing plugin implementation. After packaging the developed client plugin into a jar/zip and placing it in the application's classpath, it automatically takes effect. When initializing `NacosConfigService` or `NacosNamingService`, include a property in the passed `Properties` object with key `addressPluginName` and value from `getPluginName` method of the plugin.
-### Use Custom Plugins
-The implementation of custom plugins is the same as that of the server. When users need to use custom plugins, they inherit `com.alibaba.nacos.plugin.address.spi.AbstractAddressPlugin` or implement `com.alibaba.nacos.plugin.address.spi.AddressPlugin`, package the developed client plug-in into jar/zip, and put it into your application's classpath to automatically take effect. When initializing `NacosConfigService` or `NacosNamingService`, the key passed in the `Properties` object is `addressPluginName`, and val is the parameter returned by the plugin `getPluginName`.
-for example:
```java
Properties properties = new Properties();
properties.put("addressPluginName", ${addressPluginName});
@@ -88,9 +88,10 @@ for example:
String content = configService.getConfig(dataId, group, 5000);
```
-### Use the default Nacos addressing plugin
-The Java client plug-in of Nacos is adapted to the old version. If the customized plug-in is not applicable, the use of the client is the same as before.
+### Using Built-in Nacos Client-Side Plugins
+
+For Nacos Java client, built-in addressing plugin adaptations ensure compatibility with previous versions. Without custom plugins, client usage remains unchanged.
-### Plugin for other programming language
+### Addressing Plugins for Other Language Clients
-TODO
+Awaiting community contributions.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/plugin/config-change-plugin.md b/src/content/docs/latest/en/plugin/config-change-plugin.md
index 18452f0c615..0f62abf6796 100644
--- a/src/content/docs/latest/en/plugin/config-change-plugin.md
+++ b/src/content/docs/latest/en/plugin/config-change-plugin.md
@@ -1,51 +1,46 @@
---
-title: Config Change Plugin
-keywords: [Config Change,Config audit,Config format check,webhook]
-description: Nacos support Config Change Plugin.
-sidebar:
- order: 8
+title: Configuration Change Plugins
+keywords: [Nacos, Configuration Change, Configuration Auditing, Configuration Format Validation, Webhook, SPI, AOP, Plugin Development]
+description: Nacos introduces configuration change plugins in version 2.3.0, enabling configuration auditing, format validation, and webhook callbacks. Leveraging SPI injection, developers can customize pre- and post-change logic, enhancing configuration management flexibility. This article delves into plugin concepts, development流程, loading procedures, custom parameter passing, and provides a demo implementation.
---
-> Translated by AI.
-# Config Change Plugin
+# Configuration Change Plugins
-Community has long been hoping for Nacos Configuration Center to provide notifications to specific systems when configurations change. These notifications are used for recording, warning, and auditing purposes. Before version 2.3.0, the only way to achieve this was by simulating Nacos client subscription to the configurations. This approach involved subscribing to changes in core configurations and executing functionalities such as sending records and warnings upon receiving change notifications.
+The community has long desired for Nacos Configuration Center to notify specific systems upon configuration changes, facilitating logging, alerts, and other audit functions. Prior to version 2.3.0, the only approach was to mimic Nacos client subscription to configurations, allowing for subscription to core configuration change operations. Upon receiving change notifications, actions such as logging and alerts were executed.
-However, this implementation had a few significant issues. Firstly, individual configurations needed to be added one by one, making it difficult to capture all configuration changes. Secondly, functionalities could only be executed after configuration changes, and there was no capability for performing pre-change operations such as format validation or whitelist validation.
+This implementation had several major drawbacks: first, monitored configurations had to be added individually, making it difficult to capture all configuration changes; second, only post-change actions could be performed, lacking pre-change operations like format validation and whitelist checks.
-To address these limitations, starting from Nacos version 2.3.0, Nacos introduced support for injecting configuration change plugins through the [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html). This allows users to define custom plugins to execute specific logic before and after configuration changes. Examples of such logic include format validation, whitelist validation, and webhook integrations.
+Consequently, starting from version 2.3.0, Nacos supports injecting configuration change plugins via [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html), enabling users to implement custom logic before and after configuration changes through plugins, including format validation, whitelist checks, and webhooks.
-These enhancements provide more flexibility and control for users to implement their own custom logic before and after configuration changes in Nacos Configuration Center.
+## Concepts in Configuration Change Plugins
-## Concepts in Config Change Plugin
+Adopting the design philosophy of Aspect-Oriented Programming (AOP), Nacos configures change operations (such as creation, update, deletion) as `join points (PointCut)` and weaves plugins `before` and `after` these join points.
-Nacos's configuration change plugin design takes inspiration from the aspect-oriented programming (AOP) paradigm. It treats configuration change operations, such as adding, updating, and deleting, as the `pointcuts` and weaves the plugins `before` and `after` these pointcuts.
+### Configuration Change Join Points (ConfigChangePointCutTypes)
-### ConfigChangePointCutTypes
+Based on the behavior and source of configuration changes, Nacos defines several `Configuration Change Join Points (ConfigChangePointCutTypes)` in `com.alibaba.nacos.plugin.config.constants.ConfigChangePointCutTypes`. Details include:
-Nacos has categorized the configuration change operations based on their behaviors and sources. These configuration change operations are defined as several `ConfigChangePointCutTypes` in `com.alibaba.nacos.plugin.config.constants.ConfigChangePointCutTypes`. The specific details are as follows:
-
-|PointCut Name|Description|Start version|
+|Join Point Name| Description| Starting Version|
|-----|-----|-----|
-|PUBLISH_BY_HTTP|Configuration is published through the HTTP interface, including creating and modifying configurations.|2.3.0|
-|PUBLISH_BY_RPC|Configuration is published through the gRPC interface, including creating and modifying configurations.|2.3.0|
-|REMOVE_BY_HTTP|Configuration is removed through the HTTP interface.|2.3.0|
-|REMOVE_BY_RPC|Configuration is removed through the gRPC interface.|2.3.0|
-|IMPORT_BY_HTTP|Configuration is imported through the HTTP interface.|2.3.0|
-|REMOVE_BATCH_HTTP|Configurations are batch removed through the HTTP interface.|2.3.0|
+|PUBLISH_BY_HTTP| Configurations published through HTTP interfaces, including creation and modification.| 2.3.0|
+|PUBLISH_BY_RPC| Configurations published through GRPC interfaces, including creation and modification.| 2.3.0|
+|REMOVE_BY_HTTP| Configurations deleted through HTTP interfaces.| 2.3.0|
+|REMOVE_BY_RPC| Configurations deleted through GRPC interfaces.| 2.3.0|
+|IMPORT_BY_HTTP| Configurations imported through HTTP interfaces.| 2.3.0|
+|REMOVE_BATCH_HTTP| Batch deletion of configurations through HTTP interfaces.| 2.3.0|
-### ConfigChangeExecuteTypes
+### Configuration Change Weaving Types (ConfigChangeExecuteTypes)
-In Nacos, the configuration change plugins need to be executed before or after the `ConfigChangePointCutTypes` by selecting the `ConfigChangeExecuteTypes`. These execution types are defined in `com.alibaba.nacos.plugin.config.constants.ConfigChangeExecuteTypes`. The specific details are as follows:
+Nacos configuration change plugins need to execute before or after `Configuration Change Join Points`, choosing `Configuration Change Weaving Types (ConfigChangeExecuteTypes)` defined in `com.alibaba.nacos.plugin.config.constants.ConfigChangeExecuteTypes`. Details are:
-|Execute Type|Description|Start version|
+|Weaving Type| Description| Starting Version|
|-----|-----|-----|
-|EXECUTE_BEFORE_TYPE|Plugin execute **Before** `ConfigChangePointCutTypes`|2.3.0|
-|EXECUTE_AFTER_TYPE|Plugin execute **After** `ConfigChangePointCutTypes`|2.3.0|
+|EXECUTE_BEFORE_TYPE| Plugin implementation executes **before** the Configuration Change Join Point.| 2.3.0|
+|EXECUTE_AFTER_TYPE| Plugin implementation executes **after** the Configuration Change Join Point.| 2.3.0|
## Plugin Development
-To develop a config change plugin for the Nacos server, you first need to depend on the relevant API of the config change plugin.
+To develop Nacos server-side configuration change plugins, start by depending on the relevant API for configuration change plugins.
```xml
@@ -55,87 +50,46 @@ To develop a config change plugin for the Nacos server, you first need to depend
```
-`${project.version}` is the Nacos version, at least `2.3.0`.
+`${project.version}` should match the Nacos version you are developing the plugin for, starting from `2.3.0`.
-Then implement `com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService` interface, which include following method:
+Then, implement the `com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService` interface, with methods to implement:
-|Method name|Parameters|Return|Description|
+|Method Name| Input Content| Return Content| Description|
|-----|-----|-----|-----|
-|getServiceType|void|String|Returns the name of the plugin, which is used to differentiate between different types of plugin implementations.|
-|getOrder|void|int|Returns the execution order of the plugin. The configuration change plugin is designed with a chain plugin pattern, where multiple plugins are executed in order. The smaller the return value of getOrder, the earlier the plugin is executed.|
-|executeType|void|ConfigChangeExecuteTypes|Returns `ConfigChangeExecuteTypes` implemented by the plugin.|
-|pointcutMethodNames|void|ConfigChangePointCutTypes[]|Returns `ConfigChangePointCutTypes` where the plugin is implemented.|
-|execute|ConfigChangeRequest,ConfigChangeResponse|void|Executes the actual logic of the plugin.|
-
-`ConfigChangeRequest` and `ConfigChangeResponse` refer to the contents passed in during the execution of logic and the resulting execution outcome, respectively.
+|getServiceType| void| String| The name of the plugin, distinguishing different types of plugin implementations.|
+|getOrder| void| int| Execution order of the plugin; in a chain-like plugin design, multiple plugins execute in order, with smaller getOrder values preceding.|
+|executeType| void| ConfigChangeExecuteTypes| The `Configuration Change Weaving Type` implemented by the plugin.|
+|pointcutMethodNames| void| ConfigChangePointCutTypes[]| The `Configuration Change Join Points` woven by the plugin.|
+|execute| ConfigChangeRequest, ConfigChangeResponse| void| The actual execution logic of the plugin.|
-`ConfigChangeRequest` mainly include follow contents:
-
-|Field name|Type|Description|
-|-----|-----|-----|
-|requestType|ConfigChangePointCutTypes|The pointcut types of this configuration change|
-|requestArgs|HashMap|The actual parameters of this configuration change, mainly including `namespace`, `group`, `dataId`, `content`, etc., with different parameters for different pointcut types|
-
-`ConfigChangeResponse` mainly include follow contents:
-
-|Field name|Type|Description|
-|-----|-----|-----|
-|responseType|ConfigChangePointCutTypes|The pointcut types of this configuration change|
-|isSuccess|boolean|Indicates whether the execution is successful. When the return value is `false`, the configuration change will be intercepted and the failure result will be returned directly|
-|retVal|Object|Return content, a reserved field that is currently not used|
-|msg|String|Execution result information, obtained when `isSuccess` is `false`, used to return information to the client|
-|args|Object[]|The execution parameters of the configuration change, effective for the `EXECUTE_BEFORE_TYPE` plugin type. It can be used to modify the content of the actual executed configuration change, such as changing certain values in content to other values|
+The `ConfigChangeRequest` and `ConfigChangeResponse` detail the logic execution contents and results.
-### Load Plugin
+### Loading Plugins
-After the plugin finished, it needs to be packaged into jar/zip and places in the classpath of the nacos server. If you don't know how to add plugins into the classpath, please place plugins under `${nacos-server.path}/plugins` directly.
-
-After Adding plugins into classpath, also need to modify some configuration in `${nacos-server.path}/conf/application.properties`.
+After plugin development, package into jar/zip and place in Nacos server's classpath, or directly under `${nacos-server.path}/plugins`. Modify `${nacos-server.path}/conf/application.properties`:
```properties
-### The name of the config change plugin enabled in Nacos, corresponding to the return value of com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService's getServiceType method.
+### Enabled Nacos configuration change plugin name, corresponding to the getServiceType return value of com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService
nacos.core.config.plugin.${configChangePluginName}.enabled=true
```
-After restarting the Nacos cluster and completing the startup, you can see the following logs in `${nacos-server.path}/logs/plugin-control.log`:
+Restart the Nacos cluster. Upon completion, logs will appear in `${nacos-server.path}/logs/nacos.log`:
```text
[ConfigChangePluginManager] Load ${className}(${classFullName}) ConfigChangeServiceName(${configChangePluginName}) successfully.
```
-### Custom Plugin properties
+### Custom Parameter Passing for Plugins
-Some plugins may want to set certain parameters through a configuration file. Custom plugins can modify the following configurations in `${nacos-server.path}/conf/application.properties` to achieve this:
+Some plugins may require configuring parameters via a configuration file. This can be achieved by modifying `${nacos-server.path}/conf/application.properties`:
```properties
-### The name of the config change plugin enabled in Nacos, corresponding to the return value of com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService's getServiceType method.
+### Enabled Nacos configuration change plugin name, corresponding to the getServiceType return value of com.alibaba.nacos.plugin.config.spi.ConfigChangePluginService
nacos.core.config.plugin.${configChangePluginName}.${propertyKey}=${propertyValue}
```
-In `ConfigChangeRequest`, getting properties by following way:
+Parameters can then be retrieved in `ConfigChangeRequest`:
```Java
final Properties properties = (Properties) configChangeRequest.getArg(ConfigChangeConstants.PLUGIN_PROPERTIES);
-final String ${propertyKey} = properties.getProperty("${propertyKey}");
-```
-
-## Plugin Demo
-
-In the [nacos-group/nacos-plugin](https://github.com/nacos-group/nacos-plugin) repository, there is a demo implementation of a configuration change plugin. This demo plugin implements validation of the configuration content format, validation of the whitelist for importing configuration names, and a webhook callback after the change. To use this plugin, you need to package it as a JAR/ZIP file and place it in the classpath of the Nacos server. Additionally, add the following configuration in `${nacos-server.path}/conf/application.properties`:
-
-```properties
-# webhook
-#nacos.core.config.plugin.webhook.enabled=true
-# It is recommended to use EB https://help.aliyun.com/document_detail/413974.html
-#nacos.core.config.plugin.webhook.url=http://${webhookIp}:${webhookPort}/${webhookUri}?token=***
-# The content push max capacity ,byte
-#nacos.core.config.plugin.webhook.contentMaxCapacity=102400
-
-# whitelist
-#nacos.core.config.plugin.whitelist.enabled=true
-# The import file suffixs
-#nacos.core.config.plugin.whitelist.suffixs=xml,text,properties,yaml,html
-
-# fileformatcheck,which validate the import file of type and content
-#nacos.core.config.plugin.fileformatcheck.enabled=true
-```
\ No newline at end of file
+final String ${propertyKey} = properties.getProperty("${propertyKey}
\ No newline at end of file
diff --git a/src/content/docs/latest/en/plugin/control-plugin.md b/src/content/docs/latest/en/plugin/control-plugin.md
new file mode 100644
index 00000000000..36b8c24f860
--- /dev/null
+++ b/src/content/docs/latest/en/plugin/control-plugin.md
@@ -0,0 +1,47 @@
+---
+title: Anti-Fragility
+keywords: [Anti-Fragility, Rate Limiting, Connection Limit, TPS]
+description: Nacos supports anti-fragility plugins from version 2.3.0, avoiding cluster capacity issues under high pressure.
+---
+# Anti-Fragility Plugin
+
+Starting with Nacos 2.3.0, it supports injecting anti-fragility related plugins via [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html) and selecting one of these plugin implementations in the `application.properties` configuration file for actual anti-fragility capabilities. This document comprehensively explains how to implement an anti-fragility plugin and make it effective.
+
+## Concepts in Anti-Fragility Plugins
+
+Anti-fragility refers to strategies that restrict access when the **frequency and number** of requests to a service endpoint reach a certain level, protecting the server from extensive unavailability due to resource exhaustion under high pressure. Nacos' anti-fragility plugins abstract information into 'Control Points' and 'Control Rules.'
+
+### Control Points (ControlPoint)
+
+A Control Point maps to the resources consumed by service requests, primarily focusing on 'Connections' and 'Transactions Per Second (TPS).'
+
+- The Connection Control Point monitors the number of long connections from Nacos 2.x clients and the configuration long polling from Nacos 1.x clients independently.
+- The Transactions Per Second (TPS) Control Point tracks the frequency of core interface accesses on the Nacos server, treating similar operation interfaces as the same Control Point. Specific TPS Control Points are detailed later in this document under [Control Point Names](#1.1).
+
+### Control Rules (ControlRule)
+
+Control Rules enforce different limiting strategies for each Control Point, specifically 'Connection Control Rule' and 'TPS Control Rule.'
+
+The Connection Control Rule includes details such as count limits and monitored IP lists for detailed operation tracking.
+
+The TPS Control Rule involves specifying the rule's corresponding Control Point name and detailed rule content (`RuleDetail`) including max transaction limits, period of rule effectivity, and monitoring mode (monitor or intercept).
+
+## Plugin Development
+
+To develop an Nacos server-side anti-fragility plugin, start by depending on the relevant API for anti-fragility plugins.
+
+Subclass the abstract classes `com.alibaba.nacos.plugin.control.connection.ConnectionControlManager` and `com.alibaba.nacos.plugin.control.tps.TpsControlManager`, implementing the missing methods. Then, implement the `com.alibaba.nacos.plugin.control.spi.ControlManagerBuilder` interface to create instances of these abstract classes. Finally, add your implementation to SPI services.
+
+For plugin loading, package the plugin as a jar/zip and place it in the Nacos server's classpath, or directly in `${nacos-server.path}/plugins`. Modify the `application.properties` to specify the plugin type and restart the Nacos cluster.
+
+## Using Nacos' Built-in Anti-Fragility Plugin
+
+Nacos 2.3.0 onwards includes a simple anti-fragility plugin for connection and specific interface TPS restrictions. Configuration adjustments and file-based rule settings are outlined.
+
+## Advanced Plugin Development
+
+Nacos anti-fragility plugins also support advanced extensions for external rule storage, dynamic rule loading, custom rule format parsing, and custom TPS time window definitions to cater to diverse operational requirements.
+
+---
+
+The document further elaborates on supported Control Point names, rule storage locations, rule formats, and provides guidance on enhancing TPS statistics through custom time window algorithms, enabling developers to tailor anti-fragility measures precisely to their system's needs.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/plugin/datasource-plugin.md b/src/content/docs/latest/en/plugin/datasource-plugin.md
index 3b69bdd0790..f229286dba7 100644
--- a/src/content/docs/latest/en/plugin/datasource-plugin.md
+++ b/src/content/docs/latest/en/plugin/datasource-plugin.md
@@ -1,9 +1,62 @@
---
-title: Multiple data sources
-keywords: [MySQL,Derby,Multiple data sources]
-description: Multiple data sources
+title: Multiple Data Sources
+keywords: [Nacos, Multi-data Source Plugin, SPI Mechanism, MySQL, Derby, Data Source Plugin Development]
+description: Starting from version 2.2.0, Nacos supports injecting multi-data source plugins through the SPI mechanism, enabling users to easily switch between data sources like MySQL or Derby via configuration. This document details how to implement a multi-data source plugin, its usage, and development guide, encompassing dependency introduction, Mapper interface implementation, and SPI configuration writing, thereby simplifying data source extension and management. Note that this feature is currently in Beta testing and may undergo significant changes in future versions.
sidebar:
order: 4
---
+# Multi-Data Source Plugin
+Since version 2.2.0, Nacos has enabled the injection of multiple data source plugins through the SPI mechanism. Upon introducing the corresponding data source implementation, Nacos can select and load the appropriate multi-data source plugin at startup based on the `spring.datasource.platform` configuration item in the `application.properties` file. This document comprehensively explains how to realize such a plugin and make it effective.
-To be determined
\ No newline at end of file
+> **Note:**
+> The multi-data source plugin is currently in Beta testing. Its APIs and interface definitions may undergo substantial modifications in subsequent version upgrades. Please ensure compatibility with your plugin version.
+
+# Plugin Implementation
+In the original Config module, all SQL operations were executed by directly using JdbcTemplate with hardcoded SQL statements, leading to high coupling between SQL statements and business logic and supporting only Derby and MySQL data sources. The original architecture of the Config module is depicted as follows.
+
+
+
+The new multi-data source plugin leverages the SPI mechanism to abstract SQL operations by database table into several Mapper interfaces. Implementations of these Mapper interfaces require writing SQL dialect-specific implementations for different data sources. By default, Derby and MySQL Mapper implementations are provided and can be used directly; other data sources necessitate users to load data source plugins. The modified architecture diagram is shown below.
+
+
+
+# Usage
+1. Users should check if their required data source is supported by the current Nacos. Nacos provides default implementations for Derby and MySQL. If not supported, refer to the plugin development steps below to develop a plugin for personal use or contribution.
+2. Modify `spring.datasource.platform` in the `application.properties` to the corresponding data source name and configure related parameters.
+3. Compile and run to support this data source.
+
+# How Plugin Developers Can Develop
+1. Include the `nacos-datasource-plugin` dependency.
+2. Implement special SQL methods in the Mapper interfaces under `com.alibaba.nacos.plugin.datasource.mapper` that pertain to specific database tables, mainly focusing on pagination differences and other dialect peculiarities. Refer to the Derby and MySQL implementations in `com.alibaba.nacos.plugin.datasource.impl`. The mapping between database tables and Mappers is as follows:
+
+|Database Table| Mapper|
+|---|---|
+|config_info_aggr| ConfigInfoAggrMapper|
+|config_info_beta| ConfigInfoBetaMapper|
+|config_info|ConfigInfoMapper|
+|config_info_tag|ConfigInfoTagMapper|
+|config_tags_relation|ConfigTagsRelationMapper|
+|his_config_info|HistoryConfigInfoMapper|
+
+3. Write an SPI configuration file named `com.alibaba.nacos.plugin.datasource.mapper.Mapper`, which specifies the classes implementing the Mapper interfaces. See the Derby and MySQL configurations in the config module for reference.
+4. Plugin users can then leverage this plugin to achieve operations for the targeted data source by compiling and running it.
+
+# Compilation
+Before compiling the plugin, you need to first compile Nacos and install it in your local repository.
+1. `git clone git@github.com:alibaba/nacos.git`
+2. `cd nacos && mvn -B clean package install -Dmaven.test.skip=true`
+
+> If the `revision` variable cannot be resolved, update Maven to the latest version.
+
+3. `git clone #{Git address of the corresponding data source plugin implementation}`
+4. `mvn install`
+
+It's recommended to upload to your company's Maven repository.
+
+# Future Plans
+Upcoming version updates include:
+- [ ] Further SQL segmentation to reduce SQL statements while enhancing dynamic SQL implementation friendliness.
+- [ ] Extraction of differences among various data sources into a list, replaceable via configuration files or classes, facilitating plugin developers in writing plugins.
+
+# Implementations for Other Data Sources
+To be added.
\ No newline at end of file
diff --git a/src/content/docs/latest/en/plugin/trace-plugin.md b/src/content/docs/latest/en/plugin/trace-plugin.md
index 4d8e25adfae..05d50fc5045 100644
--- a/src/content/docs/latest/en/plugin/trace-plugin.md
+++ b/src/content/docs/latest/en/plugin/trace-plugin.md
@@ -1,54 +1,49 @@
---
-title: Tracing
-keywords: [Track tracing, push tracing, modifying tracing]
-description: Nacos supports Track tracing plugin, can help developers and maintainers find out problems quickly by extending plugin like push tracing.
-sidebar:
- order: 5
+title: Trace Tracking
+keywords: [Nacos, Trace Tracking, Push Tracking, Change Tracking, SPI mechanism, Plugin Development, Service Registration, Deregistration, Distributed Tracing, Beta Testing]
+description: Nacos supports custom trace tracking plugins via the SPI mechanism, monitoring operations like service registration and deregistration to facilitate rapid issue localization by operations personnel. Plugins are based on an event model, including types like RegisterInstanceTraceEvent, making them easily extensible and customizable. Currently in Beta, users should be aware of potential API changes.
---
+# Trace Tracking Plugin
-# Track tracing plugin
+Starting from version 2.2.0, Nacos allows injection of trace tracking implementation plugins through the [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html) mechanism. Plugins can subscribe and process tracing events, handling them according to desired methods (e.g., logging, storage). This document provides a comprehensive guide on implementing a trace tracking plugin and enabling its functionality.
-Since version 2.2.0, Nacos support to inject track tracing plugins through [SPI](https://docs.oracle.com/javase/tutorial/sound/SPI-intro.html), to subscribe and process trace events in the plugin with the way you want (such as logging, writing to storage, etc.).
-This document will describe how to implement a track tracing plugin and how to make it work.
+> Note:
+> The trace tracking plugin is currently in Beta testing; its APIs and interface definitions may undergo significant modifications in future updates. Please ensure compatibility with your plugin's target version.
+>
+> Unlike conventional distributed tracing, Nacos's trace tracking focuses on monitoring and recording operations related to Nacos, such as service registration, de-registration, pushes, and status changes, not inter-service communication paths. For monitoring service interactions, use dedicated distributed tracing solutions.
-> Attention:
-> At present, the track tracing plugin is still in the beta stage, and its API and interface definitions maybe modified with version upgrades. Please pay attention to the applicable version of your plugin.
->
-> The track tracing of Nacos is different from the tracking in general sense. It is mainly used to trace and record some Nacos related operations, such as service registration, de-registration, push, status change, etc.
-> It is not used to trace the access and request between micro-services. If you need to monitor the access and request between services, please use the corresponding tracing projects.
+## Concepts in Trace Tracking Plugins
-## Concepts in Track tracing Plugin
+### Trace Event
-### TraceEvent
+Nacos embeds tracing points at critical operational stages, defining a series of trace events (`TraceEvent`). Linking multiple events targeting the same resource (e.g., services, configurations) forms a trace for that resource.
-Nacos embeds points in the important operations, and defines a series of trace events named 'TraceEvent'. After combined multiple 'TraceEvent's for the same resource (such as services, configurations, etc.), the trace of the resource will be gotten.
+A `TraceEvent` includes:
-The TraceEvent will include following:
+|Field Name| Description|
+|----------|------------|
+|type| The type of event, defined by specific events|
+|eventTime| Time of the event occurrence|
+|namespaceId| Namespace ID of the event's corresponding resource|
+|group| Group name of the event's corresponding resource|
+|name| Resource name, such as a service name or configuration dataId|
-|Field Name|Description|
-|-----|---|
-|type|Type of event, defined by sub-events|
-|eventTime|Time of the event occurs|
-|namespaceId|Corresponding resource namespace ID of the event|
-|group| Corresponding resource group name of the event|
-|name | Corresponding resource name of the event,such as service name or dataId for config|
-
-Currently, the sub event types defined in Nacos include:
-
-|Event Name|Description|Details|
-|-----|---|---|
-|RegisterInstanceTraceEvent|The event for service instance registration, mainly occurs when the service provider is registered|[Detail](#1.1)|
-|DeregisterInstanceTraceEvent|The event for service instance de-registration, mainly occurs when the service provider is de-registered|[Detail](#1.2)|
-|RegisterServiceTraceEvent|The event for service registration, different from `RegisterInstanceTraceEvent`, mainly occurs when create empty services|[Detail](#1.3)|
-|DeregisterServiceTraceEvent|The event for service de-registration, different from `DeregisterInstanceTraceEvent`, mainly occurs when remove empty services|[Detail](#1.4)|
-|SubscribeServiceTraceEvent|The event for service subscription, mainly occurs when the service is subscribed|[Detail](#1.5)|
-|UnsubscribeServiceTraceEvent|The event for service unsubscription, mainly occurs when the service is unsubscribed|[Detail](#1.6)|
-|PushServiceTraceEvent|The event for service pushing, mainly occurs when the service is pushed to subscribed|[Detail](#1.7)|
-|HealthStateChangeTraceEvent|The event for service instance health state changing, mainly occurs when an instance's health state changes due to a heartbeat/health check|[Detail](#1.8)|
+Nacos has predefined sub-event types:
+
+|Event Name| Description| Details|
+|----------|------------|--------|
+|RegisterInstanceTraceEvent| Service instance registration event, triggered when registering a service provider||
+|DeregisterInstanceTraceEvent| Service instance deregistration event, triggered upon service provider deregistration||
+|RegisterServiceTraceEvent| Service registration event, distinct from instance registration, occurs during service creation||
+|DeregisterServiceTraceEvent| Service deregistration event, different from instance deregistration, happens when deleting a service||
+|SubscribeServiceTraceEvent| Service subscription event, activated when subscribing to a service||
+|UnsubscribeServiceTraceEvent| Service unsubscription event, triggered upon unsubscribing from a service||
+|PushServiceTraceEvent| Service push event, occurs during service push||
+|HealthStateChangeTraceEvent| Service instance health state change event, triggered when instance health changes due to heartbeats or health checks||
## Plugin Development
-To develop a Nacos track tracing plugin, developer first need to depend on the relevant API of the track tracing plugin.
+To develop a Nacos server-side trace tracking plugin, first, depend on the trace tracking plugin's API:
```xml
@@ -58,33 +53,31 @@ To develop a Nacos track tracing plugin, developer first need to depend on the r
```
-`${project.version}` is the version of Nacos for your development plugin.
-
-Then implement interface `com.alibaba.nacos.plugin.trace.spi.NacosTraceSubscriber`, and put your implementation into services of SPI.
+Replace `${project.version}` with the Nacos version for which you're developing the plugin.
-The methods of interface in following:
+Next, implement the `com.alibaba.nacos.plugin.trace.spi.NacosTraceSubscriber` interface and register your implementation into SPI services.
-|method name|parameters|returns|description|
-|-----|-----|-----|---|
-|getName|void|String|he name of the plugin. When the name is the same, the plugin loaded later will overwrite the plugin loaded first.|
-|subscribeTypes|void|List>|The expected the event type of the subscription for this plugin. If returns an empty list, plugin will not subscribe any event.|
-|onEvent|TraceEvent|void|The logic for handle events. The type of events will defined by `subscribeTypes`.|
-|executor|void|Executor|When return not `null`, Nacos will use the `Executor` to call `onEvent`, otherwise use event distribution thread to call `onEvent`.|
+The required methods include:
-> Attention:
-> It is recommend that you use a dependent Executor for plugin implementations, such as blocked IO operations in plugin implementations, which will block onEvent called to other events when there are IO exceptions, causing backlogs.
+|Method Name| Input Content| Return Content| Description|
+|-----------|--------------|---------------|------------|
+|getName| void| String| The plugin's name; if names conflict, the latter loaded plugin will overwrite the former.|
+|subscribeTypes| void| List>| The types of events this plugin subscribes to; returns an empty list for no subscriptions.|
+|onEvent| TraceEvent| void| The logic for event handling; input event types are defined by `subscribeTypes`.|
+|executor| void| Executor| If not `null`, the `onEvent` call uses this Executor; otherwise, it uses the event distribution thread.|
-In [nacos-group/nacos-plugin](https://github.com/nacos-group/nacos-plugin),providing a demo implementation for track tracing plugin. This demo subscribes `RegisterInstanceTraceEvent` and `DeregisterInstanceTraceEvent` and print result information into logs.
+> Note:
+> It is advised to use a dedicated Executor in plugin implementations to prevent blocking I/O operations in one plugin from delaying other events' processing.
-## Degradation of Track Tracking Plugin
+A demo trace tracking plugin implementation is available in the [nacos-group/nacos-plugin](https://github.com/nacos-group/nacos-plugin) repository, subscribing to instance registration and deregistration events and logging them.
-Because the Track Tracking Plugin is for the monitoring category, and will not affect Nacos data. So when the Track Tracking Plugin has problems, it should not affect the Nacos primary works.
+## Plugin Degradation Strategy
-It is recommend that you use a dependent Executor for plugin implementations, such as blocked IO operations in plugin implementations, which will block onEvent called to other events when there are IO exceptions, causing backlogs.
+As a monitoring enhancement, trace tracking plugins do not impact Nacos data. Thus, when issues arise, the primary workflow should remain unaffected.
-If the backlog occurs unfortunately, subsequent events will be automatically discarded when the event queue of the Track Tracking Plugin reaches the upper limit to ensure overall system stability.
+Hence, using a dedicated Executor is recommended. If there are blocking I/O operations in the plugin, I/O exceptions could stall other event `onEvent` calls, causing a backlog.
-You can see the words `Trace Event Publish failed, event : {}, publish queue size : {}` in `nacos.log` when the discard occurred
+In case of a backlog, the trace tracking plugin's event queue automatically discards subsequent events once its capacity is reached, ensuring overall system stability. Log entries indicating dropped events will appear in `nacos.log`.
## Appendix: Sub-trace Event Details
diff --git a/src/content/docs/latest/en/what-is-nacos.md b/src/content/docs/latest/en/what-is-nacos.md
index 6048c5f7bc0..adf1609c6d8 100644
--- a/src/content/docs/latest/en/what-is-nacos.md
+++ b/src/content/docs/latest/en/what-is-nacos.md
@@ -1,96 +1,78 @@
---
-title: Nacos Configuration Center profile
-keywords: [nacos]
-description: What is Nacos
+title: Nacos Configuration Center Introduction, What is Nacos
+keywords: [Nacos, service discovery, configuration management, microservices, cloud-native, dynamic DNS, ecosystem]
+description: An introduction to the Nacos Configuration Center, explaining what Nacos is and its role in microservices architecture.
---
+# What is Nacos?
-# What is Nacos
-
-> Document optimizing...
+> Documentation under optimization...
## Overview
-Welcome to Nacos!
-
-Nacos `/nɑ:kəʊs/` is the acronym for 'Dynamic Naming and Configuration Service',an easy-to-use dynamic service discovery, configuration and service management platform for building cloud native applications。
-
-Nacos is committed to help you discover, configure, and manage your microservices. It provides a set of simple and useful features enabling you to realize dynamic service discovery, service configuration, service metadata and traffic management.
+Welcome to the world of Nacos!
-Nacos makes it easier and faster to construct, deliver and manage your microservices platform. It is the infrastructure that supports a service-centered modern application architecture with a microservices or cloud-native approach.
+Nacos, pronounced `/nɑ:kəʊs/`, stands for Dynamic Naming and Configuration Service, a platform simplifying the construction of cloud-native applications with dynamic service discovery, configuration management, and service governance capabilities.
-## What is Nacos?
+Nacos aims to facilitate the discovery, configuration, and management of microservices. It offers a set of easy-to-use features that enable rapid implementation of dynamic service discovery, service configuration, metadata management, and traffic control.
-Service is a first-class citizen in Nacos. Nacos supports discovering, configuring, and managing almost all types of services:
+Nacos empowers you to build, deliver, and manage microservice platforms more agilely and effortlessly. It serves as a service infrastructure for modern application architectures centered around "services," such as microservices and cloud-native paradigms.
-[Kubernetes Service](https://kubernetes.io/docs/concepts/services-networking/service/)
+## What is Nacos?
+In the Nacos realm, services are first-class citizens. Nacos supports the discovery, configuration, and management of virtually all mainstream service types:
-[gRPC](https://grpc.io/docs/guides/concepts.html#service-definition) & [Dubbo RPC Service](https://dubbo.apache.org)
+- [Kubernetes Service](https://kubernetes.io/docs/concepts/services-networking/service/)
+- [gRPC](https://grpc.io/docs/guides/concepts.html#service-definition) & [Dubbo RPC Service](https://dubbo.apache.org)
+- [Spring Cloud RESTful Service](https://spring.io/projects/spring-cloud)
-[Spring Cloud RESTful Service](https://spring.io/projects/spring-cloud)
+Key Nacos features include:
-Key features of Nacos:
+- **Service Discovery and Health Monitoring**
-* **Service Discovery And Service Health Check**
+ Nacos supports service discovery via DNS and RPC. After providers register services using the native SDK, OpenAPI, or an independent Agent, consumers can locate and discover services through DNS or HTTP & API.
- Nacos supports both DNS-based and RPC-based (Dubbo/gRPC) service discovery. After a service provider registers a service with [native](./guide/user/sdk.md), [OpenAPI](./guide/user/open-api.md), or [a dedicated agent](./guide/user/other-language.md), a consumer can discover the service with either [DNS_TODO](./what-is-nacos.md) or [HTTP](./guide/user/open-api.md).
+ Real-time health checks by Nacos prevent requests from being sent to unhealthy hosts or service instances. It supports transport layer (PING or TCP) and application layer (e.g., HTTP, MySQL, user-defined) health checks. For complex cloud environments and network topologies like VPCs and edge networks, Nacos provides agent reporting and server-side active detection modes for health checks. A unified dashboard for health status helps manage service availability and traffic.
- Nacos provides real-time health check to prevent services from sending requests to unhealthy hosts or service instances. Nacos supports both transport layer (PING or TCP) health check and application layer (such as HTTP, Redis, MySQL, and user-defined protocol) health check. For the health check of complex clouds and network topologies(such as VPC, Edge Service etc), Nacos provides both agent mode and server mode health check. Nacos also provide a unity service health dashboard to help you manage the availability and traffic of services.
+- **Dynamic Configuration Service**
-* **Dynamic configuration management**
+ This allows centralized, externalized, and dynamic management of all environment application and service configurations.
- Dynamic configuration service allows you to manage the configuration of all applications and services in a centralized, externalized and dynamic manner across all environments.
+ Dynamic configurations eliminate the need to redeploy applications and services upon configuration changes, enhancing configuration management efficiency and agility.
- Dynamic configuration eliminates the need to redeploy applications and services when configurations are updated.
+ Centralized configuration management simplifies stateless service implementation and facilitates elastic scaling.
- Centralized management of configuration makes it more convenient for you to achieve stateless services and elastic expansion of service instances on-demand.
+ Nacos provides a straightforward UI ([Control Panel Demo](http://console.nacos.io/nacos/index.html)) for managing all service and application configurations. It also includes out-of-the-box features such as version tracking, Canary releases, one-click rollback, and client update status tracking for safer production environment configuration changes.
- Nacos provides an easy-to-use UI ([DEMO](http://console.nacos.io/nacos/index.html)) to help you manage all of your application or services's configurations. It provides some out-of-box features including configuration version tracking, canary/beta release, configuration rollback, and client configuration update status tracking to ensure the safety and control the risk of configuration change.
+- **Dynamic DNS Service**
-* **Dynamic DNS service**
+ Supports weighted routing, facilitating middle-tier load balancing, flexible routing strategies, traffic control, and simple DNS resolution within data centers. It also eases DNS-based service discovery to mitigate vendor lock-in risks.
- Dynamic DNS service which supports weighted routing makes it easier for you to implement mid-tier load balancing, flexible routing policies, traffic control, and simple DNS resolution services in your production environment within your data center. Dynamic DNS service makes it easier for you to implement DNS-based Service discovery.
+ Nacos offers simple [DNS APIs](./ecology/use-nacos-with-coredns.md) for managing service-associated domains and available IP:PORT lists.
- Nacos provides some simple [DNS APIs TODO](./what-is-nacos.md) for you to manage your DNS domain names and IPs.
+- **Service and Metadata Management**
-* **Service governance and metadata management**
-
- Nacos allows you to manage all of your services and metadata from the perspective of a microservices platform builder. This includes managing service description, life cycle, service static dependencies analysis, service health status, service traffic management,routing and security rules, service SLA, and first line metrics.
+ Nacos lets you manage all services and metadata in your data center from a microservices platform perspective, including descriptions, lifecycles, static dependency analysis, health statuses, traffic management, routing policies, security strategies, SLAs, and crucial metrics statistics.
## Nacos Map
-A picture to understand Nacos, the following structure will be described in detail.
-
-// TODO this picture need to translate.
-
-
-
-- Large picture of characteristics: To introduce the characteristics of the problem domain we want to solve from the functional characteristics and non-functional characteristics.
-- Larger architecture: Get a quick entry into the Nacos world with a clear architecture
-- Business picture: Business scenarios that can be supported with current features, and best practices
-- Big picture of the ecology: systematically sorting out the relationship between Nacos and mainstream technology ecology
-- Big picture of advantage: showcase Nacos core competitiveness
-- Strategic picture: Nacos's macro advantage from strategic to tactical level
-
-
-## Nacos landscape
-
-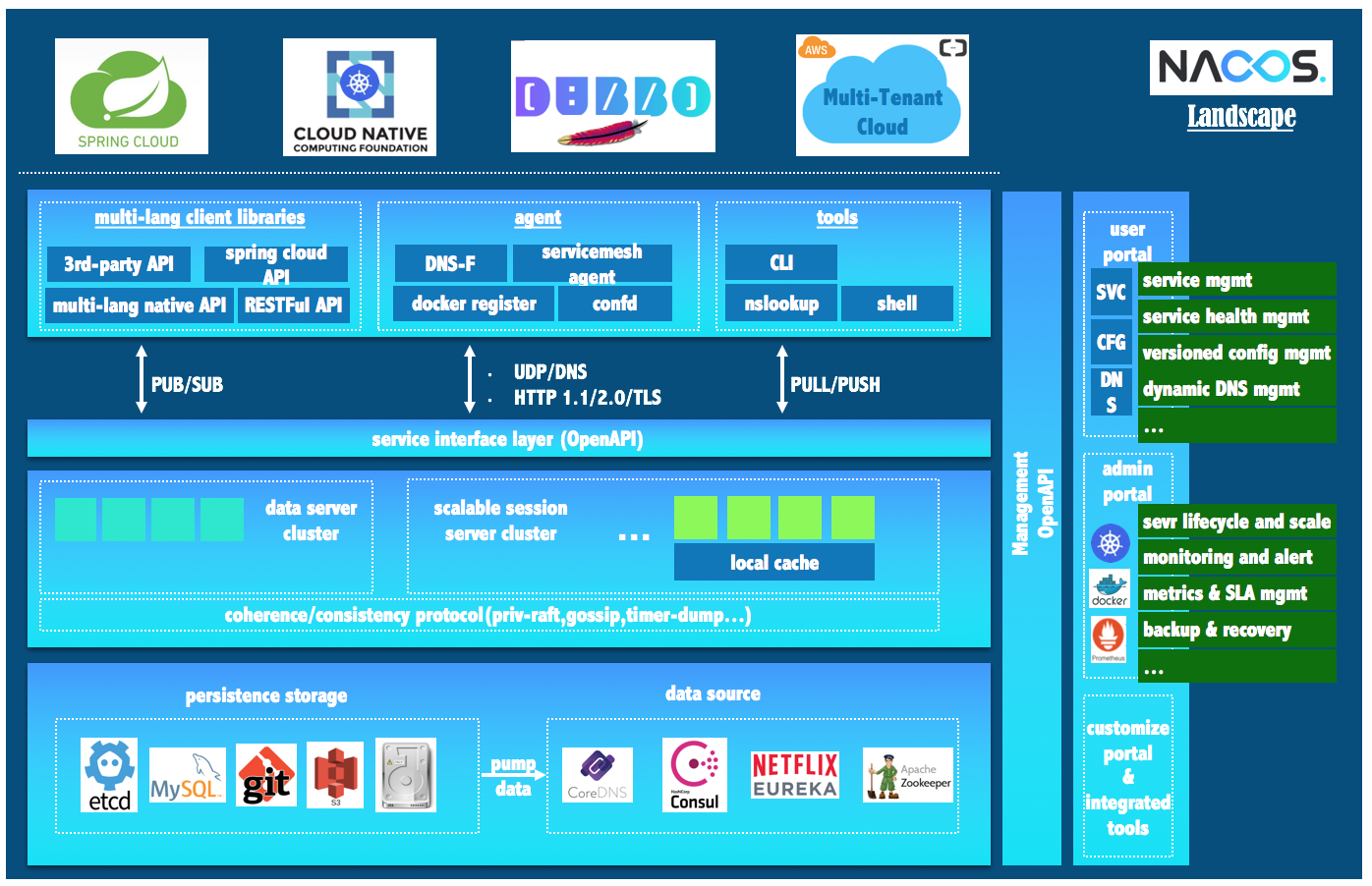
+A comprehensive visual guide to Nacos, with detailed architecture, business scenarios, ecosystem integration, advantages, and strategic benefits outlined below.
-As the figure above shows, Nacos seamlessly supports open source ecologies including
+## Nacos Ecosystem Map
-* [Spring Cloud](./ecology/use-nacos-with-spring-cloud.md)
-* [Apache Dubbo and Dubbo Mesh](./ecology/use-nacos-with-dubbo.md)
-* [Kubernetes and CNCF](./quickstart/quick-start-kubernetes.md)
+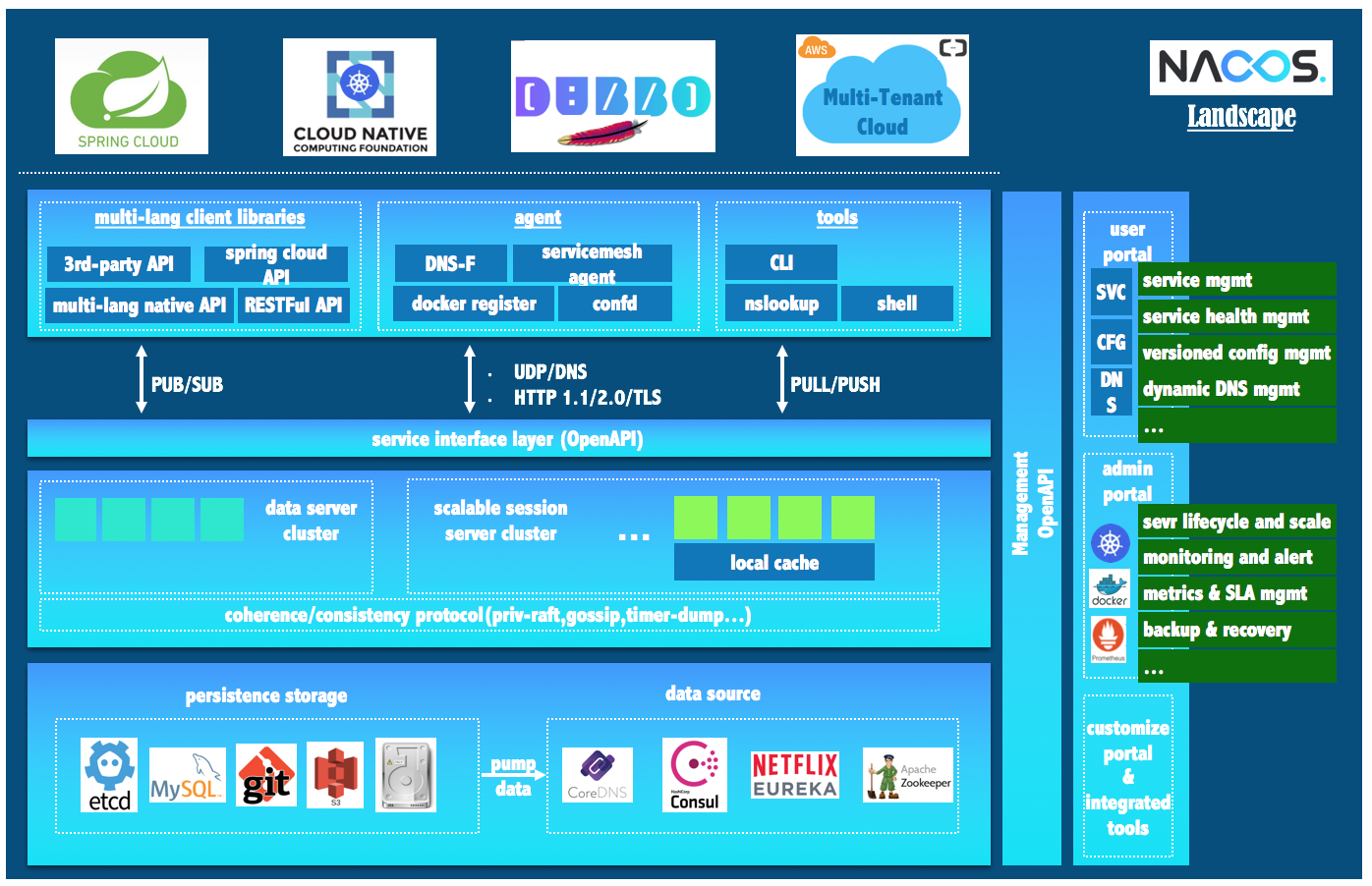
-Use Nacos to simplify your solutions in service discovery, configuration management, and service governance and management. With Nacos, microservices management in open source system is easy.
+As depicted in the Nacos全景图, Nacos seamlessly integrates with leading open-source ecosystems, including:
-For more information about how to use Nacos with other open source projects, see the following:
+- [Spring Cloud](./ecology/use-nacos-with-spring-cloud.md)
+- [Apache Dubbo and Dubbo Mesh](./ecology/use-nacos-with-dubbo.md)
+- [Kubernetes and CNCF](./quickstart/quick-start-kubernetes.md)
-[Use Nacos with Spring Cloud](./ecology/use-nacos-with-spring-cloud.md)
+Nacos streamlines service discovery, configuration management, service governance, and administration, making microservice discovery, management, sharing, and composition more accessible.
-[Use Nacos with Kubernetes](./quickstart/quick-start-kubernetes.md)
+For guides on using Nacos within these ecosystems, refer to:
-[Use Nacos with Dubbo](./ecology/use-nacos-with-dubbo.md)
+- [Using Nacos with Spring Cloud](./ecology/use-nacos-with-spring-cloud.md)
+- [Using Nacos with Kubernetes](./quickstart/quick-start-kubernetes.md)
+- [Using Nacos with Dubbo](./ecology/use-nacos-with-dubbo.md)
-## What’s next
+## Next Steps
-Continue with [quick start](./quickstart/quick-start.md) to get started with Nacos.
+Continue reading the [Quick Start](./quickstart/quick-start.md) guide to get started with Nacos swiftly.
\ No newline at end of file
diff --git a/src/content/docs/latest/zh-cn/architecture.md b/src/content/docs/latest/zh-cn/architecture.md
index ae8a3737a4b..c1a9ddf06b8 100644
--- a/src/content/docs/latest/zh-cn/architecture.md
+++ b/src/content/docs/latest/zh-cn/architecture.md
@@ -1,7 +1,7 @@
---
title: Nacos 架构
-keywords: [Nacos,架构]
-description: Nacos 架构
+keywords: [Nacos, 架构, 服务注册中心, 服务元数据, 服务提供方, 服务消费方, 配置管理, 名字服务, 配置服务]
+description: Nacos作为服务与配置管理平台,支持服务注册发现、配置与元数据管理,兼容Kubernetes、gRPC、Dubbo及Spring Cloud。架构含服务注册中心与配置管理模块,通过精细元数据区分服务角色,配置管理强调动态配置与版本控制,提升灵活性。逻辑架构涉及服务、配置、元数据管理组件及高级特性如插件机制、事件驱动。Nacos具备丰富领域模型、类视图与多种部署启动模式,适应公有云集成,满足多元化需求。
---
# Nacos 架构
diff --git a/src/content/docs/latest/zh-cn/archive/activity.md b/src/content/docs/latest/zh-cn/archive/activity.md
index 03b086225ae..4ef4cdd975d 100644
--- a/src/content/docs/latest/zh-cn/archive/activity.md
+++ b/src/content/docs/latest/zh-cn/archive/activity.md
@@ -1,7 +1,7 @@
---
title: Nacos 有奖活动介绍
-keywords: [Nacos,活动]
-description: Nacos 有奖活动介绍
+keywords: [Nacos, 有奖活动, 社区贡献, GitHub Issue, 定制礼品]
+description: Nacos举办有奖活动激励用户改进产品,涉及官网文档优化、启动流程简化、集群模式测试、Java SDK及OpenAPI建议等。参与者加入微信群,通过GitHub提交Issue,前40名可获定制礼品,强调邮件列表和Issue沟通以记录分享。
---
# Nacos 有奖活动介绍
diff --git a/src/content/docs/latest/zh-cn/archive/cli-guide.md b/src/content/docs/latest/zh-cn/archive/cli-guide.md
index b9609816ef5..9b20665a618 100644
--- a/src/content/docs/latest/zh-cn/archive/cli-guide.md
+++ b/src/content/docs/latest/zh-cn/archive/cli-guide.md
@@ -1,7 +1,7 @@
---
title: CLI Guide
-keywords: [CLI,Guide]
-description: In plan with Nacos 1.x.x
+keywords: [CLI, Guide, Nacos, 1.x.x, 计划中]
+description: 本文概述Nacos 1.x.x版本命令行界面(CLI)指南,着重介绍计划实施的新特性和改进点。
---
**IN PLAN** with Nacos 1.x.x
diff --git a/src/content/docs/latest/zh-cn/archive/contributing-dev.md b/src/content/docs/latest/zh-cn/archive/contributing-dev.md
index 28130fd7a0f..bcb9c67dca5 100644
--- a/src/content/docs/latest/zh-cn/archive/contributing-dev.md
+++ b/src/content/docs/latest/zh-cn/archive/contributing-dev.md
@@ -1,6 +1,6 @@
---
title: 为 Nacos 做贡献
-keywords: [Nacos,贡献]
+keywords: [Nacos, 贡献, 行为守则, GitHub, Pull Request, 社区角色, Committer]
description: 欢迎来到Nacos!本文档是关于如何为Nacos做出贡献的指南。
---
diff --git a/src/content/docs/latest/zh-cn/archive/feature-list.md b/src/content/docs/latest/zh-cn/archive/feature-list.md
index da01c218d2b..61c32046fcb 100644
--- a/src/content/docs/latest/zh-cn/archive/feature-list.md
+++ b/src/content/docs/latest/zh-cn/archive/feature-list.md
@@ -1,7 +1,7 @@
---
title: Nacos功能和需求列表
-keywords: [Nacos,功能]
-description: Nacos功能和需求列表
+keywords: [Nacos, 功能, 需求列表, 服务发现, 配置管理, 元数据管理, 地址服务器, 内核优化, 安全稳定性, 云原生, 客户端支持, Docker部署, Kubernetes集成, Nacos-Sync, 官网建设, 共建参与]
+description: 本文详细罗列了开源微服务项目Nacos的各项功能与未来需求规划,覆盖服务发现、配置管理、元数据管理等多个维度。通过表格形式展示了各功能的开发状态、负责人及预期发布时间,同时介绍了如何参与Nacos的开发与贡献,鼓励开发者认领任务,共同推进项目发展。
---
# Nacos功能和需求列表
diff --git a/src/content/docs/latest/zh-cn/archive/roadmap.md b/src/content/docs/latest/zh-cn/archive/roadmap.md
index 17d13a7b55a..fd56028e23f 100644
--- a/src/content/docs/latest/zh-cn/archive/roadmap.md
+++ b/src/content/docs/latest/zh-cn/archive/roadmap.md
@@ -1,7 +1,7 @@
---
title: Nacos 规划
-keywords: [Nacos,规划]
-description: Nacos 规划
+keywords: [Nacos, 规划, 生产可用, 服务发现, 配置管理, 开源生态, Spring Cloud, Kubernetes, Dubbo, 服务治理体系]
+description: Nacos计划在0.8.0版后达到生产环境使用标准,预计6至8个月内完成进化,不超过1年。主要路线图覆盖Nacos 1.0与2.0,聚焦于构建服务相关工具集、生态融合支持、易用性提升、大规模性能调优及统一服务管理平台建设。
---
# Nacos 规划
diff --git a/src/content/docs/latest/zh-cn/archive/sdk-properties.md b/src/content/docs/latest/zh-cn/archive/sdk-properties.md
index dd03fe72f90..28b03140506 100644
--- a/src/content/docs/latest/zh-cn/archive/sdk-properties.md
+++ b/src/content/docs/latest/zh-cn/archive/sdk-properties.md
@@ -1,7 +1,7 @@
---
title: Nacos 客户端初始化说明
-keywords: [Nacos,客户端,初始化]
-description: Nacos 客户端初始化说明
+keywords: [Nacos,客户端,初始化,配置,集群]
+description: 本文详细介绍了Nacos客户端初始化的两种方式,包括直接配置服务器信息与通过接入点接入,以及非必传参数如链接路径、区域隔离与鉴权设置。
---
Nacos 客户端初始化说明
diff --git a/src/content/docs/latest/zh-cn/archive/use-nacos-with-istio.md b/src/content/docs/latest/zh-cn/archive/use-nacos-with-istio.md
index 36a05c3dd3a..982bd0101d1 100644
--- a/src/content/docs/latest/zh-cn/archive/use-nacos-with-istio.md
+++ b/src/content/docs/latest/zh-cn/archive/use-nacos-with-istio.md
@@ -1,7 +1,7 @@
---
title: Nacos Istio
-keywords: [Nacos,Istio]
-description: In plan with Nacos 1.x.x
+keywords: [Nacos,Istio,计划,集成,服务发现]
+description: 本文讨论了与Nacos 1.x.x版本的集成计划,着重于如何在Istio环境中利用Nacos进行高效的服务发现与管理。
---
**IN PLAN** with Nacos 1.x.x
\ No newline at end of file
diff --git a/src/content/docs/latest/zh-cn/archive/use-nacos-with-springcloud.md b/src/content/docs/latest/zh-cn/archive/use-nacos-with-springcloud.md
index 6b0a6b1f9c0..ebf429edf18 100644
--- a/src/content/docs/latest/zh-cn/archive/use-nacos-with-springcloud.md
+++ b/src/content/docs/latest/zh-cn/archive/use-nacos-with-springcloud.md
@@ -1,7 +1,7 @@
---
title: Nacos Spring Cloud
-keywords: [nacos,Spring Cloud]
-description: Nacos Spring Cloud
+keywords: [Nacos,Spring Cloud,微服务配置,服务发现,阿里巴巴云]
+description: Nacos Spring Cloud是阿里巴巴开源的微服务管理工具,它无缝集成了Spring Cloud框架,提供了强大而灵活的服务发现、配置管理功能,通过Nacos Config Example和Nacos Discovery Example学习其应用,相关项目包括Nacos、Nacos Spring等,是构建云原生应用的理想选择。
---
# Nacos Spring Cloud
diff --git a/src/content/docs/latest/zh-cn/community/community.md b/src/content/docs/latest/zh-cn/community/community.md
index 515e9bb11a7..a3612e396d6 100644
--- a/src/content/docs/latest/zh-cn/community/community.md
+++ b/src/content/docs/latest/zh-cn/community/community.md
@@ -1,7 +1,7 @@
---
title: 社区
-keywords: [社区,联系方式]
-description: 社区
+keywords: [社区,联系方式,邮件列表,Nacos,Gitter,微博,segmentfault,钉钉群]
+description: 介绍Nacos社区的联系方式,包括Gitter聊天室、微博账号、segmentfault板块以及邮件列表的详细信息,同时邀请加入Nacos钉钉群以便更直接的交流。
sidebar:
order: 1
---
diff --git a/src/content/docs/latest/zh-cn/community/nacos-dev.md b/src/content/docs/latest/zh-cn/community/nacos-dev.md
index 93d7dd0ebb4..23bc0be8cc1 100644
--- a/src/content/docs/latest/zh-cn/community/nacos-dev.md
+++ b/src/content/docs/latest/zh-cn/community/nacos-dev.md
@@ -1,7 +1,7 @@
---
title: 开发者
-keywords: [nacos,开发者,committer]
-description: Nacos 开发者页
+keywords: [Nacos, 开发者, Maintainer, Committer, Contributor, 开源项目, 贡献者角色]
+description: 本文详细介绍了Nacos项目的开发者体系,包括Maintainer、Committer、Contributor三种角色的定义、权利与义务,以及展示了部分核心开发团队成员信息,体现了开源项目中不同贡献者的职责与贡献认可。
sidebar:
order: 2
---
diff --git a/src/content/docs/latest/zh-cn/concepts.md b/src/content/docs/latest/zh-cn/concepts.md
index fa82b3d9349..0517907b648 100644
--- a/src/content/docs/latest/zh-cn/concepts.md
+++ b/src/content/docs/latest/zh-cn/concepts.md
@@ -1,7 +1,7 @@
---
title: Nacos 概念
-keywords: [Nacos,概念]
-description: Nacos 概念
+keywords: [Nacos, 概念, 配置管理, 服务发现, 健康检查]
+description: Nacos作为动态配置与服务发现平台,阐述了地域、可用区、配置等核心概念,强调配置管理在多环境中的隔离策略,以及服务注册与健康监测机制,提升系统灵活性与稳定性。
---
# Nacos 概念
diff --git a/src/content/docs/latest/zh-cn/contribution/contributing-flow.md b/src/content/docs/latest/zh-cn/contribution/contributing-flow.md
index 0d7f28b0d9f..1d07962ed01 100644
--- a/src/content/docs/latest/zh-cn/contribution/contributing-flow.md
+++ b/src/content/docs/latest/zh-cn/contribution/contributing-flow.md
@@ -1,7 +1,7 @@
---
title: 贡献流程
-keywords: [贡献,源码]
-description: 此贡献流程适用于所有的Nacos社区内容,包括但不限于`Nacos`、`Nacos wiki/doc`、`Nacos SDK`。
+keywords: [Nacos, 贡献流程, 源码, GitHub, 社区贡献, Pull Request, Rebase]
+description: 本文详细介绍了向Nacos社区贡献代码的标准化流程,包括如何Fork项目、克隆代码、设置上游仓库、创建开发分支、遵循代码规范、Rebase解决冲突、推送更改及创建Pull Request,旨在帮助新贡献者顺利参与Nacos开源项目。
sidebar:
order: 2
---
diff --git a/src/content/docs/latest/zh-cn/contribution/contributing.md b/src/content/docs/latest/zh-cn/contribution/contributing.md
index c286342063d..3370e8ea444 100644
--- a/src/content/docs/latest/zh-cn/contribution/contributing.md
+++ b/src/content/docs/latest/zh-cn/contribution/contributing.md
@@ -1,7 +1,7 @@
---
title: 如何贡献
-keywords: [贡献,源码]
-description: 我们非常欢迎您的贡献和加入,无论是微不足道的清理或大的新功能。
+keywords: [贡献, 源码, Nacos, 代码规约, 文档, 提交者, 拉取请求]
+description: 本文详细介绍了如何向Nacos项目贡献代码和文档,包括联系项目团队、遵循代码规范、贡献流程、文档编辑要求以及成为提交者的路径,强调了持续贡献、代码质量与项目参与的重要性。
sidebar:
order: 1
---
diff --git a/src/content/docs/latest/zh-cn/contribution/how-to-reporting-bugs.md b/src/content/docs/latest/zh-cn/contribution/how-to-reporting-bugs.md
index 39e0dccfc15..d21977c1256 100644
--- a/src/content/docs/latest/zh-cn/contribution/how-to-reporting-bugs.md
+++ b/src/content/docs/latest/zh-cn/contribution/how-to-reporting-bugs.md
@@ -1,7 +1,7 @@
---
title: 如何提交问题报告
-keywords: [提交,问题报告]
-description: 如何提交问题报告
+keywords: [提交问题报告, Nacos, GitHub, 错误报告, 安全问题, ASRC]
+description: 本文指导用户如何向Nacos项目提交问题报告,包括详细步骤和建议,以及如何通过ASRC报告安全问题,确保问题得到有效处理。
sidebar:
order: 4
---
diff --git a/src/content/docs/latest/zh-cn/contribution/pull-request.md b/src/content/docs/latest/zh-cn/contribution/pull-request.md
index 08c8864b121..0c7381b4e69 100644
--- a/src/content/docs/latest/zh-cn/contribution/pull-request.md
+++ b/src/content/docs/latest/zh-cn/contribution/pull-request.md
@@ -1,7 +1,7 @@
---
title: 提交需求模板
-keywords: [pull request,模板]
-description: 提交需求模板
+keywords: [提交需求模板,pull request,模板,changelog,单元测试]
+description: 本文介绍了提交需求模板的规范,强调了提交前应创建相关问题,明确了修改的目的、简要变更日志以及验证方法。提供了检查清单指导贡献者快速有效地整合代码,包括使用Github issue跟踪、格式化提交标题、详尽描述更改、编写单元测试及运行必要的Maven命令进行检查。对于大型贡献,需签署Apache个人贡献者许可协议。
sidebar:
order: 3
---
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-sync.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-sync.md
index c28f8e3c2fd..1a6c1b6a051 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-sync.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-sync.md
@@ -1,6 +1,6 @@
---
title: NacosSync 用户手册
-keywords: [NacosSync,迁移,用户手册]
+keywords: [NacosSync,迁移,用户手册,多注册中心同步,服务迁移,Zookeeper,Nacos,Dubbo]
description: NacosSync 迁移用户手册
sidebar:
order: 5
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-coredns.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-coredns.md
index c2cb8814e49..a19db7dd4fa 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-coredns.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-coredns.md
@@ -1,7 +1,7 @@
---
title: Nacos 融合CoreDNS 下发DNS域名
-keywords: [CoreDNS, DNS-F]
-description: Nacos 融合CoreDNS 下发DNS域名
+keywords: [Nacos, CoreDNS, DNS域名, 服务发现, golang]
+description: Nacos融合CoreDNS插件可将Nacos注册服务导出为DNS域名,作为side car代理进程实现服务发现。支持Linux和Mac系统,需nacos 2.2+和golang 1.17+版本。通过配置nacos_server_host与namespaceId,利用build脚本构建并运行,未注册域名自动转发至upstream。
sidebar:
order: 6
---
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-dubbo.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-dubbo.md
index 0d3bc912db9..41a15371af8 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-dubbo.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-dubbo.md
@@ -1,7 +1,7 @@
---
title: Dubbo 融合 Nacos 成为注册中心
-keywords: [Dubbo,Nacos,注册中心]
-description: Dubbo 融合 Nacos 成为注册中心
+keywords: [Dubbo,Nacos,注册中心,微服务,Spring框架,Maven依赖,Spring Boot,外部化配置,服务提供者,服务消费者]
+description: Dubbo 融合 Nacos 成为注册中心
sidebar:
order: 1
---
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-istio.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-istio.md
index 05d22ddb01a..07e0d0079cb 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-istio.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-istio.md
@@ -1,7 +1,7 @@
---
title: Nacos 融合Istio 下发xDS协议
-keywords: [Istio,xDs,Envoy]
-description: Nacos 融合Istio 下发xDS协议
+keywords: [Nacos, Istio融合, xDS协议, 服务发现, Envoy]
+description: 本文介绍了如何在Nacos中集成Istio以支持xDS协议,包括CDS与EDS服务,实现了对Envoy及其他xDS协议客户端的兼容。通过配置Nacos服务端与Envoy客户端,详细指导了如何启用增量推送及全量推送功能,以实现高效的服务发现与管理。
---
# Istio 指南
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-k8s-sync.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-k8s-sync.md
index b6a48b326c8..af2cbd94c91 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-k8s-sync.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-k8s-sync.md
@@ -1,7 +1,7 @@
---
title: Nacos支持从K8S服务发现中同步服务元数据
-keywords: [Nacos,k8s,kubernetes]
-description: Nacos支持从K8S服务发现中同步服务元数据
+keywords: [Nacos, k8s, Kubernetes, 服务元数据, 同步]
+description: Nacos现支持与Kubernetes(K8S)服务发现集成,实现服务元数据的自动同步。该功能监控K8S内服务与实例的动态,确保Nacos服务发现模块及时更新,兼容K8S 1.22版本及以上,通过特定配置开启双向数据流动,简化微服务架构管理。
---
# Nacos支持从K8S服务发现中同步服务元数据
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-boot.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-boot.md
index cb46db6f7bb..8882bc718f9 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-boot.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-boot.md
@@ -1,7 +1,7 @@
---
title: Nacos 融合 Spring Boot,成为注册配置中心
-keywords: [Nacos,Spring Boot]
-description: 本文主要面向 Spring Boot 的使用者,通过示例来介绍如何使用 Nacos 来实现分布式环境下的配置管理和服务发现。
+keywords: [Nacos, Spring Boot, 配置管理, 服务发现, 动态变更, 注册中心]
+description: 本文主要面向 Spring Boot 的使用者,通过示例来介绍如何使用 Nacos 来实现分布式环境下的配置管理和服务发现。
sidebar:
order: 3
---
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-cloud.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-cloud.md
index 64a8682ee68..e01c31d301a 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-cloud.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring-cloud.md
@@ -1,7 +1,7 @@
---
title: Nacos 融合 Spring Cloud,成为注册配置中心
-keywords: [Nacos,Spring Cloud]
-description: 本文主要面向 Spring Cloud 的使用者,通过示例来介绍如何使用 Nacos 来实现分布式环境下的配置管理和服务发现
+keywords: [Nacos, Spring Cloud, 配置管理, 服务发现, 注册中心, 分布式环境, 动态配置]
+description: 文章介绍了如何将Nacos与Spring Cloud结合,构建分布式系统中的配置管理和服务发现功能。通过实战示例,展示了使用Nacos Server与Spring Cloud starter实现配置动态变更和服务注册的具体步骤,包括配置Nacos server地址、在Spring Boot应用中添加依赖、配置文件设置以及如何利用Open API动态更新配置。同时,也演示了服务发现功能的启用过程,让读者能够快速上手在Spring Cloud项目中集成Nacos。
sidebar:
order: 4
---
diff --git a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring.md b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring.md
index 59cca0d5165..2776306a3eb 100644
--- a/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring.md
+++ b/src/content/docs/latest/zh-cn/ecology/use-nacos-with-spring.md
@@ -1,6 +1,6 @@
---
title: Nacos 融合 Spring,成为注册配置中心
-keywords: [Nacos,Spring,快速开始]
+keywords: [Nacos, Spring, 注解驱动, 依赖注入, 外部化配置, 事件驱动, 配置管理, 服务发现]
description: 本文主要面向 Spring 的使用者,通过两个示例来介绍如何使用 Nacos 来实现分布式环境下的配置管理和服务发现。
sidebar:
order: 2
diff --git a/src/content/docs/latest/zh-cn/guide/admin/cluster-mode-quick-start.md b/src/content/docs/latest/zh-cn/guide/admin/cluster-mode-quick-start.md
index e185d15979b..53c81089927 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/cluster-mode-quick-start.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/cluster-mode-quick-start.md
@@ -1,7 +1,7 @@
---
title: 集群部署说明
-keywords: [集群,部署]
-description: 集群部署说明
+keywords: [集群部署, Nacos, 集群模式, 端口配置, 数据源配置, 服务注册与发现, 配置管理, 权限认证]
+description: 该文档指导如何进行Nacos集群模式的部署,包括集群架构介绍、环境准备、源码或安装包获取、集群配置、数据源设置以及服务注册、发现与配置管理的详细步骤。强调了不同部署模式下的端口配置细节、内外置数据源的使用方法,并提供了权限认证的配置示例。
sidebar:
order: 2
---
diff --git a/src/content/docs/latest/zh-cn/guide/admin/console-guide.md b/src/content/docs/latest/zh-cn/guide/admin/console-guide.md
index 6aa7bf39d0c..c18bb73a7de 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/console-guide.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/console-guide.md
@@ -1,7 +1,7 @@
---
title: 控制台手册
-keywords: [控制台,手册]
-description: Nacos 控制台主要旨在于增强对于服务列表,健康状态管理,服务治理,分布式配置管理等方面的管控能力。
+keywords: [Nacos控制台,服务管理,配置管理,命名空间管理,登录管理,微服务治理,分布式配置]
+description: Nacos控制台旨在强化微服务管理,包括服务列表监控、健康状态控制、流量权重调整、配置多格式编辑与版本控制、命名空间隔离及登录安全机制,提升服务治理与配置管理效率,简化运维成本。
sidebar:
order: 5
---
diff --git a/src/content/docs/latest/zh-cn/guide/admin/deployment.md b/src/content/docs/latest/zh-cn/guide/admin/deployment.md
index 90dca2646bc..055cbf1f45e 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/deployment.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/deployment.md
@@ -1,7 +1,7 @@
---
title: Nacos支持三种部署模式
-keywords: [Nacos,部署模式]
-description: Nacos支持三种部署模式
+keywords: [Nacos,部署模式,单机模式,集群模式,多集群模式,JDK,MySQL]
+description: Nacos支持单机、集群及多集群三种部署模式,适用于不同场景需求。推荐在内部网络环境下部署,确保安全与稳定性。单机模式适用于测试,集群模式保证生产环境的高可用性,多集群模式满足多数据中心部署。环境准备包括JDK 1.8+、建议硬件配置及MySQL数据库支持。
sidebar:
order: 1
---
diff --git a/src/content/docs/latest/zh-cn/guide/admin/management-api.md b/src/content/docs/latest/zh-cn/guide/admin/management-api.md
index d8880b015f7..711b7a42b06 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/management-api.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/management-api.md
@@ -1,7 +1,7 @@
---
title: Management API
-keywords: [Management,API]
-description: In plan with Nacos 1.x.x
+keywords: [管理API, Nacos 1.x.x, 文档优化]
+description: 本文档涉及Nacos 1.x.x版本中的管理API介绍,目前正处于文档优化阶段,待完善。
sidebar:
order: 4
---
diff --git a/src/content/docs/latest/zh-cn/guide/admin/monitor-guide.md b/src/content/docs/latest/zh-cn/guide/admin/monitor-guide.md
index e3292107017..ffc0620cc16 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/monitor-guide.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/monitor-guide.md
@@ -1,6 +1,6 @@
---
title: Nacos 监控手册
-keywords: [Nacos,监控手册]
+keywords: [Nacos, 监控手册, Prometheus, Grafana, metrics, 集群, 告警, 指标]
description: Nacos 监控手册
sidebar:
order: 6
diff --git a/src/content/docs/latest/zh-cn/guide/admin/nacos2-naming-benchmark.md b/src/content/docs/latest/zh-cn/guide/admin/nacos2-naming-benchmark.md
index d6149b2c00b..8a3e3cfc189 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/nacos2-naming-benchmark.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/nacos2-naming-benchmark.md
@@ -1,7 +1,7 @@
---
title: Nacos2.0服务发现性能测试报告
-keywords: [Nacos,服务,发现,性能]
-description: Nacos2.0服务发现性能测试报告
+keywords: [Nacos, 服务发现, 性能测试, TPS, RT, 注册实例, 查询实例, 注销实例, Nacos2.0, Nacos1.X, 服务端性能]
+description: 报告详细介绍了Nacos2.0.0-ALPHA2服务发现性能的测试情况,包括注册、查询、注销实例三大关键接口的性能负载和容量提升。通过与Nacos1.X版本对比,展示了在服务端机能减半的条件下,Nacos2.0实现了注册和注销性能至少2倍的提升,查询性能提升至3倍,达到百万级服务及实例的稳定处理能力。测试使用自研PAS平台,对集群规模、启动参数及测试场景进行了详尽配置。
sidebar:
order: 8
---
diff --git a/src/content/docs/latest/zh-cn/guide/admin/system-configurations.md b/src/content/docs/latest/zh-cn/guide/admin/system-configurations.md
index b3dc11ec5c5..95d4eb6f6da 100644
--- a/src/content/docs/latest/zh-cn/guide/admin/system-configurations.md
+++ b/src/content/docs/latest/zh-cn/guide/admin/system-configurations.md
@@ -1,6 +1,6 @@
---
title: Nacos系统参数介绍
-keywords: [Nacos,系统参数]
+keywords: [Nacos, 系统参数, 服务器配置, Java客户端, 数据库配置, 连接池, 日志级别, 配置模块, 命名空间]
description: Nacos系统参数介绍
sidebar:
order: 3
diff --git a/src/content/docs/latest/zh-cn/guide/user/auth.md b/src/content/docs/latest/zh-cn/guide/user/auth.md
index 56c4ec4d5da..0f708a56310 100644
--- a/src/content/docs/latest/zh-cn/guide/user/auth.md
+++ b/src/content/docs/latest/zh-cn/guide/user/auth.md
@@ -1,6 +1,6 @@
---
title: Authorization
-keywords: [Authorization]
+keywords: [Nacos, 鉴权, 微服务, 安全风险, 插件开发, JWT, Token, 缓存, 服务端, 客户端, SDK, Open-API, Docker, 身份识别]
description: Authorization
sidebar:
order: 5
diff --git a/src/content/docs/latest/zh-cn/guide/user/failover.md b/src/content/docs/latest/zh-cn/guide/user/failover.md
index 13613788f23..ad22b3d882d 100644
--- a/src/content/docs/latest/zh-cn/guide/user/failover.md
+++ b/src/content/docs/latest/zh-cn/guide/user/failover.md
@@ -1,7 +1,7 @@
---
title: Java客户端容灾
-keywords: [容灾]
-description: Java客户端容灾用户指南
+keywords: [Java客户端容灾, 容灾数据源, 磁盘容灾, SPI扩展, Nacos服务端]
+description: 本文介绍了Java客户端容灾机制,包括如何在Nacos服务端问题时维持客户端稳定,通过开启本地容灾使用缓存数据。详细说明了两种应用场景、容灾流程、磁盘容灾的实现细节及自定义扩展容灾数据源的方法。
sidebar:
order: 7
hidden: true
diff --git a/src/content/docs/latest/zh-cn/guide/user/faq.md b/src/content/docs/latest/zh-cn/guide/user/faq.md
index 46652402c74..e1a25a621b5 100644
--- a/src/content/docs/latest/zh-cn/guide/user/faq.md
+++ b/src/content/docs/latest/zh-cn/guide/user/faq.md
@@ -1,7 +1,7 @@
---
title: FAQ
-keywords: [Nacos,FAQ]
-description: Nacos FAQ
+keywords: [Nacos, FAQ, 常规问题, 运维问题, 使用问题, 原理问题, 微服务, 配置管理, 服务发现, Docker, Kubernetes, Spring Cloud, Dubbo, 多环境, 生产可用, 监控, 扩缩容, 权重, 加密, 错误代码401, 日志, 集群部署]
+description: 本文档整理了Nacos常见问题解答(FAQ),涵盖Nacos的常规理解、运维部署、使用技巧以及深入原理等多个维度。针对微服务配置管理与服务发现的需求,详细解答了如何在不同环境部署Nacos、如何处理生产环境中遇到的问题、与Spring Cloud、Dubbo等框架的集成方法、如何进行监控与日志管理、服务扩缩容操作、配置加密等高级话题。此外,还涉及Nacos在Docker、Kubernetes等容器化环境下的部署策略,以及解决启动、运行时常见错误的方法。
sidebar:
order: 6
---
diff --git a/src/content/docs/latest/zh-cn/plugin/address-plugin.md b/src/content/docs/latest/zh-cn/plugin/address-plugin.md
index 8baec700bbf..90251b48142 100644
--- a/src/content/docs/latest/zh-cn/plugin/address-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/address-plugin.md
@@ -1,6 +1,6 @@
---
title: 寻址插件
-keywords: [寻址, 插件]
+keywords: [Nacos, 寻址插件, SPI, 服务端, 客户端, 自定义插件]
description: 本文描述如何开发及使用Nacos的寻址插件
sidebar:
order: 2
diff --git a/src/content/docs/latest/zh-cn/plugin/auth-plugin.md b/src/content/docs/latest/zh-cn/plugin/auth-plugin.md
index 8e8db2857bf..60342d92683 100644
--- a/src/content/docs/latest/zh-cn/plugin/auth-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/auth-plugin.md
@@ -1,6 +1,6 @@
---
title: 鉴权插件
-keywords: [鉴权, 插件]
+keywords: [鉴权插件, Nacos, SPI, API, 服务端插件, 客户端插件, 自定义插件, 身份信息, 资源, 操作类型, Beta测试]
description: 本文描述如何开发及使用Nacos的鉴权插件
sidebar:
order: 1
diff --git a/src/content/docs/latest/zh-cn/plugin/config-change-plugin.md b/src/content/docs/latest/zh-cn/plugin/config-change-plugin.md
index ed10f6ae29a..5537ccc5dd0 100644
--- a/src/content/docs/latest/zh-cn/plugin/config-change-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/config-change-plugin.md
@@ -1,7 +1,7 @@
---
title: 配置变更
-keywords: [配置变更,配置审计,配置格式校验,webhook]
-description: Nacos 支持配置变更插件,可支持配置审计、配置格式校验、及变更后webhook回调
+keywords: [Nacos, 配置变更, 配置审计, 配置格式校验, webhook, SPI, AOP, 插件开发]
+description: Nacos 2.3.0引入配置变更插件支持,实现配置审计、格式校验与webhook回调。通过SPI注入,开发者能定制变更前后逻辑,增强配置管理灵活性。文章详述了插件概念、开发流程、加载与自定义参数传递,及DEMO实现。
sidebar:
order: 8
---
diff --git a/src/content/docs/latest/zh-cn/plugin/config-encryption-plugin.md b/src/content/docs/latest/zh-cn/plugin/config-encryption-plugin.md
index f328e4cc473..deafd373275 100644
--- a/src/content/docs/latest/zh-cn/plugin/config-encryption-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/config-encryption-plugin.md
@@ -1,7 +1,7 @@
---
title: 配置加密
-keywords: [AES,encryption,配置加密]
-description: 配置加密
+keywords: [Nacos, 配置加密, AES, 数据安全, SPI, 加密算法, MySQL, 内嵌数据库]
+description: Nacos新特性支持配置加密,确保用户敏感数据安全,适用于2.x版本以上,通过SPI机制实现AES加密等插件化加解密操作,无需单独加密配置。用户可自定义加解密方式,服务端与客户端均需引入特定依赖,如nacos-aes-encryption-plugin。加密配置通过控制台便捷创建,以cipher-前缀标识自动加密处理。
sidebar:
order: 3
---
diff --git a/src/content/docs/latest/zh-cn/plugin/control-plugin.md b/src/content/docs/latest/zh-cn/plugin/control-plugin.md
index 50ef82af6b4..967d3efef28 100644
--- a/src/content/docs/latest/zh-cn/plugin/control-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/control-plugin.md
@@ -1,6 +1,6 @@
---
title: 反脆弱
-keywords: [反脆弱,限流,连接数限制,TPS]
+keywords: [反脆弱, Nacos, SPI, 连接数限制, TPS, 插件开发, 反脆弱规则, 监控点, ControlPoint, ControlRule]
description: Nacos 支持反脆弱插件,避免高压下的集群容量问题。
sidebar:
order: 7
diff --git a/src/content/docs/latest/zh-cn/plugin/custom-environment-plugin.md b/src/content/docs/latest/zh-cn/plugin/custom-environment-plugin.md
index 1ab79dccfd0..d9cecd7b202 100644
--- a/src/content/docs/latest/zh-cn/plugin/custom-environment-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/custom-environment-plugin.md
@@ -1,7 +1,7 @@
---
title: 自定义环境变量
keywords: [自定义环境变量,自定义配置,数据库密码加密]
-description: Nacos 自定义环境变量插件,可自定义扩展服务端配置等功能,例如数据库密码加密。
+description: Nacos自定义环境变量插件支持通过SPI机制扩展服务端配置,实现如数据库密码加密等功能,适用于2.2.0及以上版本,目前处于Beta阶段,需留意API变动。
sidebar:
order: 6
---
diff --git a/src/content/docs/latest/zh-cn/plugin/datasource-plugin.md b/src/content/docs/latest/zh-cn/plugin/datasource-plugin.md
index 84f65b6bca6..64e374a5b64 100644
--- a/src/content/docs/latest/zh-cn/plugin/datasource-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/datasource-plugin.md
@@ -1,7 +1,7 @@
---
title: 多数据源
-keywords: [MySQL,Derby,多数据源]
-description: 多数据源
+keywords: [Nacos,多数据源插件,SPI机制,MySQL,Derby,数据源插件开发]
+description: Nacos从2.2.0版起支持SPI机制注入多数据源插件,允许用户通过配置轻松切换MySQL或Derby等数据源。本文介绍如何实现多数据源插件、使用方法及开发指南,包括引入依赖、实现Mapper接口和编写SPI配置,简化数据源扩展和管理。注意该功能目前为Beta阶段,可能随版本更新变动。
sidebar:
order: 4
---
diff --git a/src/content/docs/latest/zh-cn/plugin/trace-plugin.md b/src/content/docs/latest/zh-cn/plugin/trace-plugin.md
index e290bc8604f..be9b2df1624 100644
--- a/src/content/docs/latest/zh-cn/plugin/trace-plugin.md
+++ b/src/content/docs/latest/zh-cn/plugin/trace-plugin.md
@@ -1,7 +1,7 @@
---
title: 轨迹追踪
-keywords: [轨迹追踪,推送轨迹,变更轨迹]
-description: Nacos 支持轨迹追踪插件,可自定义扩展制作推送轨迹等功能,帮助运维人员快速定位问题。
+keywords: [Nacos, 轨迹追踪, 推送轨迹, 变更轨迹, SPI机制, 插件开发, 服务注册, 注销, 链路追踪, Beta测试]
+description: Nacos通过SPI机制支持自定义轨迹追踪插件,用于服务注册、注销等操作的监控,帮助运维快速定位问题。插件基于事件模型,如RegisterInstanceTraceEvent等,便于扩展和定制化处理。目前处于Beta阶段,需留意API变动。
sidebar:
order: 5
---
diff --git a/src/content/docs/latest/zh-cn/quickstart/quick-start-docker.md b/src/content/docs/latest/zh-cn/quickstart/quick-start-docker.md
index aa9dff93906..fe292a63671 100644
--- a/src/content/docs/latest/zh-cn/quickstart/quick-start-docker.md
+++ b/src/content/docs/latest/zh-cn/quickstart/quick-start-docker.md
@@ -1,7 +1,7 @@
---
title: Nacos Docker 快速开始
-keywords: [Nacos,Docker]
-description: Nacos Docker 快速开始
+keywords: [Nacos, Docker, 快速开始, 服务注册, 服务发现, 配置管理, 集群模式, 单机模式, MySQL, Prometheus, Grafana]
+description: 本文介绍了如何快速使用Docker部署Nacos服务,包括单机与集群模式的配置,以及如何利用Docker Compose启动不同存储模式下的Nacos实例。详细步骤覆盖了Derby与MySQL数据库的集成,服务的注册与发现,配置发布与获取,还提供了Nacos控制台访问方式。此外,文章提及了Nacos与其他工具如Grafana和Prometheus的集成监控方案,并列出了Nacos的常用属性配置项及其说明。
sidebar:
order: 2
---
diff --git a/src/content/docs/latest/zh-cn/quickstart/quick-start-kubernetes.md b/src/content/docs/latest/zh-cn/quickstart/quick-start-kubernetes.md
index 9ff3dfbb091..316c0b60c7a 100644
--- a/src/content/docs/latest/zh-cn/quickstart/quick-start-kubernetes.md
+++ b/src/content/docs/latest/zh-cn/quickstart/quick-start-kubernetes.md
@@ -1,7 +1,7 @@
---
title: Kubernetes Nacos
-keywords: [nacos,kubernetes]
-description: 本项目包含一个可构建的Nacos Docker Image,旨在利用 StatefulSets 在 Kubernetes上部署 Nacos。
+keywords: [Kubernetes, Nacos, Docker Image, StatefulSets, 部署, 持久卷, 自动扩容缩容, NFS, MySQL, 集群配置]
+description: 该文档介绍了如何在Kubernetes上部署Nacos服务,通过定制化的Docker镜像与StatefulSets实现稳定部署。提供了从快速启动到高级配置的全面指南,包括使用NFS进行持久化存储、MySQL数据库集成、以及如何进行服务的注册与发现等操作。还展示了如何利用Nacos Operator进行更简便的部署管理,以及如何实施动态扩容以满足不同场景需求。
sidebar:
order: 3
---
diff --git a/src/content/docs/latest/zh-cn/upgrading/200-compatibility.md b/src/content/docs/latest/zh-cn/upgrading/200-compatibility.md
index df04e61aa72..f770013a412 100644
--- a/src/content/docs/latest/zh-cn/upgrading/200-compatibility.md
+++ b/src/content/docs/latest/zh-cn/upgrading/200-compatibility.md
@@ -1,7 +1,7 @@
---
title: Nacos 2.0.0 兼容性文档
-keywords: [Nacos,2.0.0]
-description: Nacos 2.0.0 兼容性文档
+keywords: [Nacos, 2.0.0, 兼容性, gRPC, 部署, 生态兼容, FAQ]
+description: 本文详细介绍了Nacos 2.0.0版本的兼容性特性,包括服务端与1.X客户端的平滑升降级能力,新引入的gRPC通信方式及其端口配置,各功能模块的完成度与旧版本客户端的适配情况,生态系统的兼容性改进,如Spring Cloud Alibaba、Dubbo的适配进展,以及新版本的部署注意事项和常见问题解答。
sidebar:
order: 1
---
diff --git a/src/content/docs/latest/zh-cn/what-is-nacos.md b/src/content/docs/latest/zh-cn/what-is-nacos.md
index d52b9c13925..d2ed03000ed 100644
--- a/src/content/docs/latest/zh-cn/what-is-nacos.md
+++ b/src/content/docs/latest/zh-cn/what-is-nacos.md
@@ -1,7 +1,7 @@
---
title: Nacos 配置中心简介, Nacos 是什么
-keywords: [nacos]
-description: Nacos 配置中心简介,Nacos 是什么
+keywords: [Nacos, 服务发现, 配置管理, 微服务, 云原生, 动态DNS, 生态系统]
+description: Nacos是一个面向云原生应用的动态服务发现、配置管理及服务管理平台,支持多种服务类型,简化微服务构建、部署和管理,提供服务发现、健康监测、动态配置、DNS服务及元数据管理等功能。它无缝融入Spring Cloud、Apache Dubbo等主流开源生态,加速微服务架构的实施。
---
# 什么是 Nacos