diff --git a/.coderabbit.yaml b/.coderabbit.yaml
index 08e1985ae7..acbadb976b 100644
--- a/.coderabbit.yaml
+++ b/.coderabbit.yaml
@@ -1,8 +1,8 @@
# yaml-language-server: $schema=https://coderabbit.ai/integrations/schema.v2.json
-language: "en-US"
+language: 'en-US'
early_access: false
reviews:
- profile: "chill"
+ profile: 'chill'
request_changes_workflow: true
high_level_summary: true
poem: true
diff --git a/.prettierrc b/.prettierrc
index 2c0fc022ce..3baced4112 100644
--- a/.prettierrc
+++ b/.prettierrc
@@ -1,4 +1,4 @@
{
- "singleQuote": true,
- "endOfLine": "auto"
-}
\ No newline at end of file
+ "singleQuote": true,
+ "endOfLine": "auto"
+}
diff --git a/CODE_STYLE.md b/CODE_STYLE.md
index df184b12a0..3d9dced6ac 100644
--- a/CODE_STYLE.md
+++ b/CODE_STYLE.md
@@ -25,7 +25,7 @@ code style should not be changed and must be followed.
- Typescript
-- React.js
+- React.js
- CSS module
@@ -43,10 +43,9 @@ code style should not be changed and must be followed.
- Should make use of React hooks where appropriate
-
## Code Style and Naming Conventions
-- All React components *must* be written in PascalCase, with their file names, and associated CSS modules being written in PascalCase
+- All React components _must_ be written in PascalCase, with their file names, and associated CSS modules being written in PascalCase
- All other files may follow the camelCase naming convention
@@ -55,13 +54,15 @@ code style should not be changed and must be followed.
- Use of custom classes directly are refrained, use of modular css is encouraged along with bootstrap classes
**Wrong way ❌**
+
```
...
...
// No using personal custom classes directly, here you should not use myCustomClass2
.container{...} // No changing the property of already existing classes reserved by boostrap directly in css files
```
-**Correct ways ✅**
+**Correct ways ✅**
+
```
...
// Use custom class defined in modular css file
...
// Use classes already defined in Bootstrap
@@ -74,7 +75,8 @@ code style should not be changed and must be followed.
**Wrong way ❌**
-Using plain Bootstrap classes and attributes without leveraging the React-Bootstrap library should be refrained. While it may work for basic functionality, it doesn't fully integrate with React and may cause issues when dealing with more complex state management or component interactions.
+Using plain Bootstrap classes and attributes without leveraging the React-Bootstrap library should be refrained. While it may work for basic functionality, it doesn't fully integrate with React and may cause issues when dealing with more complex state management or component interactions.
+
```
@@ -87,11 +89,11 @@ Using plain Bootstrap classes and attributes without leveraging the React-Bootst
```
-
**Correct way ✅**
It's recommended to use the React-Bootstrap library for seamless integration of Bootstrap components in a React application.
+
```
import Dropdown from 'react-bootstrap/Dropdown';
@@ -114,10 +116,9 @@ function BasicExample() {
export default BasicExample;
```
+## Test and Code Linting
-## Test and Code Linting
-
-Unit tests must be written for *all* code submissions to the repository,
+Unit tests must be written for _all_ code submissions to the repository,
the code submitted must also be linted ESLint and formatted with Prettier.
## Folder/Directory Structure
@@ -125,18 +126,19 @@ the code submitted must also be linted ESLint and formatted with Prettier.
### Sub Directories of `src`
`assets` - This houses all of the static assets used in the project
- - `css` - This houses all of the css files used in the project
- - `images` - This houses all of the images used in the project
- - `scss` - This houses all of the scss files used in the project
- - `components -` All Sass files for components
- - `content -` All Sass files for content
- - `forms -` All Sass files for forms
- - `_talawa.scss` - Partial Sass file for Talawa
- - `_utilities.scss` - Partial Sass file for utilities
- - `_variables.scss` - Partial Sass file for variables
- - `app.scss` - Main Sass file for the app, imports all other partial Sass files
-
-`components` - The directory for base components that will be used in the various views/screens
+
+- `css` - This houses all of the css files used in the project
+- `images` - This houses all of the images used in the project
+- `scss` - This houses all of the scss files used in the project
+ - `components -` All Sass files for components
+ - `content -` All Sass files for content
+ - `forms -` All Sass files for forms
+ - `_talawa.scss` - Partial Sass file for Talawa
+ - `_utilities.scss` - Partial Sass file for utilities
+ - `_variables.scss` - Partial Sass file for variables
+ - `app.scss` - Main Sass file for the app, imports all other partial Sass files
+
+`components` - The directory for base components that will be used in the various views/screens
`Constant` - This houses all of the constants used in the project
@@ -148,7 +150,6 @@ the code submitted must also be linted ESLint and formatted with Prettier.
`utils` - This holds the utility functions that do not fall into any of the other categories
-
## Imports
Absolute imports have been set up for the project, so imports may be done directly from `src`.
@@ -161,13 +162,12 @@ import Navbar from 'components/Navbar/Navbar';
Imports should be grouped in the following order:
- - React imports
- - Third party imports
- - Local imports
-
+- React imports
+- Third party imports
+- Local imports
If there is more than one import from a single library, they should be grouped together
-
+
Example - If there is single import from a library, both ways will work
```
@@ -190,38 +190,40 @@ Follow this [link](https://getbootstrap.com/docs/5.3/customize/sass/) to learn h
**File Structure**
- `src/assets/scss/components/{'{partialFile}'}.scss` - where the {'{partialFile}'} are the following files
- - **_accordion.scss**
- - **_alert.scss**
- - **_badge.scss**
- - **_breadcrumb.scss**
- - **_buttons.scss**
- - **_card.scss**
- - **_carousel.scss**
- - **_close.scss**
- - **_dropdown.scss**
- - **_list-group.scss**
- - **_modal.scss**
- - **_nav.scss**
- - **_navbar.scss**
- - **_offcanvas.scss**
- - **_pagination.scss**
- - **_placeholder.scss**
- - **_progress.scss**
- - **_spinners.scss**
+
+ - **\_accordion.scss**
+ - **\_alert.scss**
+ - **\_badge.scss**
+ - **\_breadcrumb.scss**
+ - **\_buttons.scss**
+ - **\_card.scss**
+ - **\_carousel.scss**
+ - **\_close.scss**
+ - **\_dropdown.scss**
+ - **\_list-group.scss**
+ - **\_modal.scss**
+ - **\_nav.scss**
+ - **\_navbar.scss**
+ - **\_offcanvas.scss**
+ - **\_pagination.scss**
+ - **\_placeholder.scss**
+ - **\_progress.scss**
+ - **\_spinners.scss**
- `src/assets/scss/content/{'{partialFile}'}.scss` - where the {'{partialFile}'} are the following files
- - **_table.scss**
- - **_typography.scss**
+ - **\_table.scss**
+ - **\_typography.scss**
- `src/assets/scss/forms/{'{partialFile}'}.scss` - where the {'{partialFile}'} are the following files
- - **_check-radios.scss**
- - **_floating-label.scss**
- - **_form-control.scss**
- - **_input-group.scss**
- - **_range.scss**
- - **_select.scss**
- - **_validation.scss**
+
+ - **\_check-radios.scss**
+ - **\_floating-label.scss**
+ - **\_form-control.scss**
+ - **\_input-group.scss**
+ - **\_range.scss**
+ - **\_select.scss**
+ - **\_validation.scss**
- `src/assets/scss/_utilities.scss` - The utility API is a Sass-based tool to generate utility classes.
- `src/assets/scss/_variables.scss` - This file contains all the Sass variables used in the project
@@ -245,9 +247,11 @@ To watch the Sass file for changes and compile it automatically, run the followi
```
npx sass src/assets/scss/app.scss src/assets/css/app.css --watch
```
+
The `src/assets/css/app.css.map` file associates the generated CSS code with the original SCSS code. It allows you to see your SCSS code in the browser's developer tools for debugging.
To skip generating the map file, run
+
```
npx sass --no-source-map src/assets/scss/app.scss src/assets/css/app.css
```
diff --git a/DOCUMENTATION.md b/DOCUMENTATION.md
index 7691b5d452..d961e5d132 100644
--- a/DOCUMENTATION.md
+++ b/DOCUMENTATION.md
@@ -1,4 +1,5 @@
# Documentation
+
Welcome to our documentation guide. Here are some useful tips you need to know!
# Table of Contents
@@ -15,18 +16,21 @@ Welcome to our documentation guide. Here are some useful tips you need to know!
Our documentation can be found in ONLY TWO PLACES:
-1. ***Inline within the repository's code files***: We have automated processes to extract this information and place it in our Talawa documentation site [docs.talawa.io](https://docs.talawa.io/).
-1. ***In our `talawa-docs` repository***: Our [Talawa-Docs](https://github.com/PalisadoesFoundation/talawa-docs) repository contains user edited markdown files that are automatically integrated into our Talawa documentation site [docs.talawa.io](https://docs.talawa.io/) using the [Docusaurus](https://docusaurus.io/) package.
+1. **_Inline within the repository's code files_**: We have automated processes to extract this information and place it in our Talawa documentation site [docs.talawa.io](https://docs.talawa.io/).
+1. **_In our `talawa-docs` repository_**: Our [Talawa-Docs](https://github.com/PalisadoesFoundation/talawa-docs) repository contains user edited markdown files that are automatically integrated into our Talawa documentation site [docs.talawa.io](https://docs.talawa.io/) using the [Docusaurus](https://docusaurus.io/) package.
## How to use Docusaurus
+
The process in easy:
+
1. Install `talawa-docs` on your system
-1. Launch docusaurus on your system according to the `talawa-docs`documentation.
- - A local version of `docs.talawa.io` should automatically launched in your browser at http://localhost:3000/
+1. Launch docusaurus on your system according to the `talawa-docs`documentation.
+ - A local version of `docs.talawa.io` should automatically launched in your browser at http://localhost:3000/
1. Add/modify the markdown documents to the `docs/` directory of the `talawa-docs` repository
1. If adding a file, then you will also need to edit the `sidebars.js` which is used to generate the [docs.talawa.io](https://docs.talawa.io/) menus.
-1. Always monitor the local website in your brower to make sure the changes are acceptable.
- - You'll be able to see errors that you can use for troubleshooting in the CLI window you used to launch the local website.
+1. Always monitor the local website in your brower to make sure the changes are acceptable.
+ - You'll be able to see errors that you can use for troubleshooting in the CLI window you used to launch the local website.
## Other information
-***PLEASE*** do not add markdown files in this repository. Add them to `talawa-docs`!
+
+**_PLEASE_** do not add markdown files in this repository. Add them to `talawa-docs`!
diff --git a/ISSUE_GUIDELINES.md b/ISSUE_GUIDELINES.md
index 5170de5839..73a58b2eb3 100644
--- a/ISSUE_GUIDELINES.md
+++ b/ISSUE_GUIDELINES.md
@@ -4,7 +4,8 @@
In order to give everyone a chance to submit a issues reports and contribute to the Talawa project, we have put restrictions in place. This section outlines the guidelines that should be imposed upon issue reports in the Talawa project.
-___
+---
+
## Table of Contents
@@ -18,15 +19,17 @@ ___
-___
+---
+
## Issue Management
In all cases please use the [GitHub open issue search](https://github.com/PalisadoesFoundation/talawa-admin/issues) to check whether the issue has already been reported.
### New Issues
+
To create new issues follow these steps:
-1. Your issue may have already been created. Search for duplicate open issues before submitting yours.for similar deficiencies in the code.duplicate issues are created.
+1. Your issue may have already been created. Search for duplicate open issues before submitting yours.for similar deficiencies in the code.duplicate issues are created.
1. Verify whether the issue has been fixed by trying to reproduce it using the latest master or development branch in the repository.
1. Click on the [`New Issue`](https://github.com/PalisadoesFoundation/talawa-admin/issues/new/choose) button
1. Use the templates to create a standardized report of what needs to be done and why.
@@ -49,11 +52,12 @@ Working on these types of existing issues is a good way of getting started with
Feature requests are welcome. But take a moment to find out whether your idea fits with the scope and aims of the project. It's up to you to make a strong case to convince the mentors of the merits of this feature. Please provide as much detail and context as possible.
-### Monitoring the Creation of New Issues
+### Monitoring the Creation of New Issues
+
1. Join our `#talawa-github` slack channel for automatic issue and pull request updates.
## General Guidelines
1. Discuss issues in our various slack channels when necessary
-2. Please do not derail or troll issues.
+2. Please do not derail or troll issues.
3. Keep the discussion on topic and respect the opinions of others.
diff --git a/PR_GUIDELINES.md b/PR_GUIDELINES.md
index 4c904c782d..46412edbc4 100644
--- a/PR_GUIDELINES.md
+++ b/PR_GUIDELINES.md
@@ -47,6 +47,7 @@ npm run format:check
1. Please read our [CONTRIBUTING.md](CONTRIBUTING.md) document for details on our testing policy.
## Pull Request Processing
+
These are key guidelines for the procedure:
### Only submit PRs against our `develop` branch, not the default `main` branch
diff --git a/README.md b/README.md
index 911ba11453..05d7277f04 100644
--- a/README.md
+++ b/README.md
@@ -44,13 +44,13 @@ Core features include:
1. You can install the software for this repository using the steps in our [INSTALLATION.md](INSTALLATION.md) file.
1. Do you want to contribute to our code base? Look at our [CONTRIBUTING.md](CONTRIBUTING.md) file to get started. There you'll also find links to:
- 1. Our code of conduct documentation in the [CODE_OF_CONDUCT.md](CODE_OF_CONDUCT.md) file.
- 1. How we handle the processing of new and existing issues in our [ISSUE_GUIDELINES.md](ISSUE_GUIDELINES.md) file.
- 1. The methodologies we use to manage our pull requests in our [PR_GUIDELINES.md](PR_GUIDELINES.md) file.
+ 1. Our code of conduct documentation in the [CODE_OF_CONDUCT.md](CODE_OF_CONDUCT.md) file.
+ 1. How we handle the processing of new and existing issues in our [ISSUE_GUIDELINES.md](ISSUE_GUIDELINES.md) file.
+ 1. The methodologies we use to manage our pull requests in our [PR_GUIDELINES.md](PR_GUIDELINES.md) file.
1. The `talawa` documentation can be found at our [docs.talawa.io](https://docs.talawa.io) site.
- 1. It is automatically generated from the markdown files stored in our [Talawa-Docs GitHub repository](https://github.com/PalisadoesFoundation/talawa-docs). This makes it easy for you to update our documenation.
+ 1. It is automatically generated from the markdown files stored in our [Talawa-Docs GitHub repository](https://github.com/PalisadoesFoundation/talawa-docs). This makes it easy for you to update our documenation.
-# Videos
+# Videos
1. Visit our [YouTube Channel playlists](https://www.youtube.com/@PalisadoesOrganization/playlists) for more insights
1. The "[Getting Started - Developers](https://www.youtube.com/watch?v=YpBUoHxEeyg&list=PLv50qHwThlJUIzscg9a80a9-HmAlmUdCF&index=1)" videos are extremely helpful for new open source contributors.
diff --git a/schema.graphql b/schema.graphql
index a0ff7bde8b..ef9c0fabcb 100644
--- a/schema.graphql
+++ b/schema.graphql
@@ -694,7 +694,12 @@ type Mutation {
revokeRefreshTokenForUser: Boolean!
saveFcmToken(token: String): Boolean!
sendMembershipRequest(organizationId: ID!): MembershipRequest!
- sendMessageToChat(chatId: ID!, messageContent: String!, type: String!, replyTo: ID): ChatMessage!
+ sendMessageToChat(
+ chatId: ID!
+ messageContent: String!
+ type: String!
+ replyTo: ID
+ ): ChatMessage!
signUp(data: UserInput!, file: String): AuthData!
togglePostPin(id: ID!, title: String): Post!
unassignUserTag(input: ToggleUserTagAssignInput!): User
@@ -1026,7 +1031,12 @@ type Query {
skip: Int
where: EventWhereInput
): [Event!]!
- advertisementsConnection(after: String, before: String, first: PositiveInt, last: PositiveInt): AdvertisementsConnection
+ advertisementsConnection(
+ after: String
+ before: String
+ first: PositiveInt
+ last: PositiveInt
+ ): AdvertisementsConnection
getDonationById(id: ID!): Donation!
getDonationByOrgId(orgId: ID!): [Donation]
getDonationByOrgIdConnection(
@@ -1084,7 +1094,7 @@ type Query {
skip: Int
where: UserWhereInput
): [UserData]!
- venue(id:ID!):[Venue]
+ venue(id: ID!): [Venue]
}
input RecaptchaVerification {
@@ -1388,7 +1398,7 @@ input UserInput {
firstName: String!
lastName: String!
password: String!
- selectedOrganization : ID!
+ selectedOrganization: ID!
}
enum UserOrderByInput {
@@ -1534,4 +1544,4 @@ input chatInput {
organizationId: ID
userIds: [ID!]!
name: String
-}
\ No newline at end of file
+}
diff --git a/scripts/githooks/check-localstorage-usage.js b/scripts/githooks/check-localstorage-usage.js
index 0a811df307..0a7e2adbfc 100755
--- a/scripts/githooks/check-localstorage-usage.js
+++ b/scripts/githooks/check-localstorage-usage.js
@@ -86,10 +86,10 @@ if (filesWithLocalStorage.length > 0) {
console.info(
'\x1b[34m%s\x1b[0m',
- '\nInfo: Consider using custom hook functions.'
+ '\nInfo: Consider using custom hook functions.',
);
console.info(
- 'Please use the getItem, setItem, and removeItem functions provided by the custom hook useLocalStorage.\n'
+ 'Please use the getItem, setItem, and removeItem functions provided by the custom hook useLocalStorage.\n',
);
process.exit(1);
diff --git a/scripts/githooks/update-toc.js b/scripts/githooks/update-toc.js
index 268becfd13..2b4e4f9b83 100644
--- a/scripts/githooks/update-toc.js
+++ b/scripts/githooks/update-toc.js
@@ -8,7 +8,6 @@ const markdownFiles = fs
markdownFiles.forEach((file) => {
const command = `markdown-toc -i "${file}" --bullets "-"`;
execSync(command, { stdio: 'inherit' });
-
});
console.log('Table of contents updated successfully.');
diff --git a/src/screens/PageNotFound/PageNotFound.tsx b/src/screens/PageNotFound/PageNotFound.tsx
index e50ede56a2..62ed90c423 100644
--- a/src/screens/PageNotFound/PageNotFound.tsx
+++ b/src/screens/PageNotFound/PageNotFound.tsx
@@ -3,7 +3,7 @@ import { Link } from 'react-router-dom';
import { useTranslation } from 'react-i18next';
import useLocalStorage from 'utils/useLocalstorage';
-import styles from '../../style/app.module.css'
+import styles from '../../style/app.module.css';
import Logo from 'assets/images/talawa-logo-600x600.png';
/**
diff --git a/talawa-admin-docs/README.md b/talawa-admin-docs/README.md
index 82dc89754c..c6d717a58e 100644
--- a/talawa-admin-docs/README.md
+++ b/talawa-admin-docs/README.md
@@ -1,6 +1,7 @@
talawa-admin / [Modules](modules.md)
# Talawa Admin
+
💬 Join the community on Slack. The link can be found in the `Talawa` [README.md](https://github.com/PalisadoesFoundation/talawa) file.
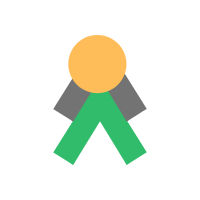
diff --git a/talawa-admin-docs/classes/components_AddOn_support_services_Plugin_helper.default.md b/talawa-admin-docs/classes/components_AddOn_support_services_Plugin_helper.default.md
index 31189c706c..f7c160f44b 100644
--- a/talawa-admin-docs/classes/components_AddOn_support_services_Plugin_helper.default.md
+++ b/talawa-admin-docs/classes/components_AddOn_support_services_Plugin_helper.default.md
@@ -40,7 +40,7 @@
[src/components/AddOn/support/services/Plugin.helper.ts:7](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/AddOn/support/services/Plugin.helper.ts#L7)
-___
+---
### fetchStore
@@ -54,21 +54,21 @@ ___
[src/components/AddOn/support/services/Plugin.helper.ts:2](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/AddOn/support/services/Plugin.helper.ts#L2)
-___
+---
### generateLinks
-▸ **generateLinks**(`plugins`): \{ `name`: `string` ; `url`: `string` \}[]
+▸ **generateLinks**(`plugins`): \{ `name`: `string` ; `url`: `string` \}[]
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :-------- | :------ |
| `plugins` | `any`[] |
#### Returns
-\{ `name`: `string` ; `url`: `string` \}[]
+\{ `name`: `string` ; `url`: `string` \}[]
#### Defined in
diff --git a/talawa-admin-docs/enums/components_EventCalendar_EventCalendar.ViewType.md b/talawa-admin-docs/enums/components_EventCalendar_EventCalendar.ViewType.md
index 29922c23d5..dad766e47b 100644
--- a/talawa-admin-docs/enums/components_EventCalendar_EventCalendar.ViewType.md
+++ b/talawa-admin-docs/enums/components_EventCalendar_EventCalendar.ViewType.md
@@ -15,17 +15,17 @@
### DAY
-• **DAY** = ``"Day"``
+• **DAY** = `"Day"`
#### Defined in
[src/components/EventCalendar/EventCalendar.tsx:46](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/EventCalendar/EventCalendar.tsx#L46)
-___
+---
### MONTH
-• **MONTH** = ``"Month"``
+• **MONTH** = `"Month"`
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeCheckIn.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeCheckIn.md
index eb47c5bd4e..c0a778b30b 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeCheckIn.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeCheckIn.md
@@ -22,17 +22,17 @@
[src/components/CheckIn/types.ts:8](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L8)
-___
+---
### checkIn
-• **checkIn**: ``null`` \| \{ `_id`: `string` ; `allotedRoom`: `string` ; `allotedSeat`: `string` ; `time`: `string` \}
+• **checkIn**: `null` \| \{ `_id`: `string` ; `allotedRoom`: `string` ; `allotedSeat`: `string` ; `time`: `string` \}
#### Defined in
[src/components/CheckIn/types.ts:10](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L10)
-___
+---
### user
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md
index 9ecfe473e1..ab31cda5f7 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md
@@ -18,9 +18,9 @@
#### Type declaration
-| Name | Type |
-| :------ | :------ |
-| `_id` | `string` |
+| Name | Type |
+| :----------------------- | :----------------------------------------------------------------------------------- |
+| `_id` | `string` |
| `attendeesCheckInStatus` | [`InterfaceAttendeeCheckIn`](components_CheckIn_types.InterfaceAttendeeCheckIn.md)[] |
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceModalProp.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceModalProp.md
index 2fa711670a..b3784d36f1 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceModalProp.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceModalProp.md
@@ -22,7 +22,7 @@
[src/components/CheckIn/types.ts:27](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L27)
-___
+---
### handleClose
@@ -40,7 +40,7 @@ ___
[src/components/CheckIn/types.ts:28](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L28)
-___
+---
### show
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableCheckIn.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableCheckIn.md
index e87af3ecc3..b34ed9dcfa 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableCheckIn.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableCheckIn.md
@@ -18,13 +18,13 @@
### checkIn
-• **checkIn**: ``null`` \| \{ `_id`: `string` ; `allotedRoom`: `string` ; `allotedSeat`: `string` ; `time`: `string` \}
+• **checkIn**: `null` \| \{ `_id`: `string` ; `allotedRoom`: `string` ; `allotedSeat`: `string` ; `time`: `string` \}
#### Defined in
[src/components/CheckIn/types.ts:35](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L35)
-___
+---
### eventId
@@ -34,7 +34,7 @@ ___
[src/components/CheckIn/types.ts:41](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L41)
-___
+---
### id
@@ -44,7 +44,7 @@ ___
[src/components/CheckIn/types.ts:32](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L32)
-___
+---
### name
@@ -54,7 +54,7 @@ ___
[src/components/CheckIn/types.ts:33](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L33)
-___
+---
### userId
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableData.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableData.md
index 390a79948f..4c0679df1d 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableData.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceTableData.md
@@ -22,7 +22,7 @@
[src/components/CheckIn/types.ts:47](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L47)
-___
+---
### id
@@ -32,7 +32,7 @@ ___
[src/components/CheckIn/types.ts:46](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L46)
-___
+---
### userName
diff --git a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceUser.md b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceUser.md
index 877cb3ff5a..78bde7ee8d 100644
--- a/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceUser.md
+++ b/talawa-admin-docs/interfaces/components_CheckIn_types.InterfaceUser.md
@@ -22,7 +22,7 @@
[src/components/CheckIn/types.ts:2](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L2)
-___
+---
### firstName
@@ -32,7 +32,7 @@ ___
[src/components/CheckIn/types.ts:3](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/types.ts#L3)
-___
+---
### lastName
diff --git a/talawa-admin-docs/interfaces/components_CollapsibleDropdown_CollapsibleDropdown.InterfaceCollapsibleDropdown.md b/talawa-admin-docs/interfaces/components_CollapsibleDropdown_CollapsibleDropdown.InterfaceCollapsibleDropdown.md
index a94bf4c9bf..7743512ded 100644
--- a/talawa-admin-docs/interfaces/components_CollapsibleDropdown_CollapsibleDropdown.InterfaceCollapsibleDropdown.md
+++ b/talawa-admin-docs/interfaces/components_CollapsibleDropdown_CollapsibleDropdown.InterfaceCollapsibleDropdown.md
@@ -21,7 +21,7 @@
[src/components/CollapsibleDropdown/CollapsibleDropdown.tsx:9](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CollapsibleDropdown/CollapsibleDropdown.tsx#L9)
-___
+---
### target
diff --git a/talawa-admin-docs/interfaces/components_IconComponent_IconComponent.InterfaceIconComponent.md b/talawa-admin-docs/interfaces/components_IconComponent_IconComponent.InterfaceIconComponent.md
index a8669a5913..50ea98493d 100644
--- a/talawa-admin-docs/interfaces/components_IconComponent_IconComponent.InterfaceIconComponent.md
+++ b/talawa-admin-docs/interfaces/components_IconComponent_IconComponent.InterfaceIconComponent.md
@@ -23,7 +23,7 @@
[src/components/IconComponent/IconComponent.tsx:17](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/IconComponent/IconComponent.tsx#L17)
-___
+---
### height
@@ -33,7 +33,7 @@ ___
[src/components/IconComponent/IconComponent.tsx:18](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/IconComponent/IconComponent.tsx#L18)
-___
+---
### name
@@ -43,7 +43,7 @@ ___
[src/components/IconComponent/IconComponent.tsx:16](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/IconComponent/IconComponent.tsx#L16)
-___
+---
### width
diff --git a/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEvent.InterfaceLeftDrawerProps.md b/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEvent.InterfaceLeftDrawerProps.md
index 2323d9e1b9..f26dce0b21 100644
--- a/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEvent.InterfaceLeftDrawerProps.md
+++ b/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEvent.InterfaceLeftDrawerProps.md
@@ -20,33 +20,33 @@
#### Type declaration
-| Name | Type |
-| :------ | :------ |
-| `_id` | `string` |
-| `description` | `string` |
-| `organization` | \{ `_id`: `string` \} |
-| `organization._id` | `string` |
-| `title` | `string` |
+| Name | Type |
+| :----------------- | :-------------------- |
+| `_id` | `string` |
+| `description` | `string` |
+| `organization` | \{ `_id`: `string` \} |
+| `organization._id` | `string` |
+| `title` | `string` |
#### Defined in
[src/components/LeftDrawerEvent/LeftDrawerEvent.tsx:17](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerEvent/LeftDrawerEvent.tsx#L17)
-___
+---
### hideDrawer
-• **hideDrawer**: ``null`` \| `boolean`
+• **hideDrawer**: `null` \| `boolean`
#### Defined in
[src/components/LeftDrawerEvent/LeftDrawerEvent.tsx:25](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerEvent/LeftDrawerEvent.tsx#L25)
-___
+---
### setHideDrawer
-• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<``null`` \| `boolean`\>\>
+• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<`null` \| `boolean`\>\>
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEventWrapper.InterfacePropType.md b/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEventWrapper.InterfacePropType.md
index 231bd29f60..358c9a355e 100644
--- a/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEventWrapper.InterfacePropType.md
+++ b/talawa-admin-docs/interfaces/components_LeftDrawerEvent_LeftDrawerEventWrapper.InterfacePropType.md
@@ -21,7 +21,7 @@
[src/components/LeftDrawerEvent/LeftDrawerEventWrapper.tsx:15](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerEvent/LeftDrawerEventWrapper.tsx#L15)
-___
+---
### event
@@ -29,13 +29,13 @@ ___
#### Type declaration
-| Name | Type |
-| :------ | :------ |
-| `_id` | `string` |
-| `description` | `string` |
-| `organization` | \{ `_id`: `string` \} |
-| `organization._id` | `string` |
-| `title` | `string` |
+| Name | Type |
+| :----------------- | :-------------------- |
+| `_id` | `string` |
+| `description` | `string` |
+| `organization` | \{ `_id`: `string` \} |
+| `organization._id` | `string` |
+| `title` | `string` |
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_LeftDrawerOrg_LeftDrawerOrg.InterfaceLeftDrawerProps.md b/talawa-admin-docs/interfaces/components_LeftDrawerOrg_LeftDrawerOrg.InterfaceLeftDrawerProps.md
index 4ba897ae0f..7ef5d75859 100644
--- a/talawa-admin-docs/interfaces/components_LeftDrawerOrg_LeftDrawerOrg.InterfaceLeftDrawerProps.md
+++ b/talawa-admin-docs/interfaces/components_LeftDrawerOrg_LeftDrawerOrg.InterfaceLeftDrawerProps.md
@@ -18,13 +18,13 @@
### hideDrawer
-• **hideDrawer**: ``null`` \| `boolean`
+• **hideDrawer**: `null` \| `boolean`
#### Defined in
[src/components/LeftDrawerOrg/LeftDrawerOrg.tsx:23](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerOrg/LeftDrawerOrg.tsx#L23)
-___
+---
### orgId
@@ -34,7 +34,7 @@ ___
[src/components/LeftDrawerOrg/LeftDrawerOrg.tsx:20](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerOrg/LeftDrawerOrg.tsx#L20)
-___
+---
### screenName
@@ -44,17 +44,17 @@ ___
[src/components/LeftDrawerOrg/LeftDrawerOrg.tsx:21](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerOrg/LeftDrawerOrg.tsx#L21)
-___
+---
### setHideDrawer
-• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<``null`` \| `boolean`\>\>
+• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<`null` \| `boolean`\>\>
#### Defined in
[src/components/LeftDrawerOrg/LeftDrawerOrg.tsx:24](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawerOrg/LeftDrawerOrg.tsx#L24)
-___
+---
### targets
diff --git a/talawa-admin-docs/interfaces/components_LeftDrawer_LeftDrawer.InterfaceLeftDrawerProps.md b/talawa-admin-docs/interfaces/components_LeftDrawer_LeftDrawer.InterfaceLeftDrawerProps.md
index d1fc895d2d..6694a5f350 100644
--- a/talawa-admin-docs/interfaces/components_LeftDrawer_LeftDrawer.InterfaceLeftDrawerProps.md
+++ b/talawa-admin-docs/interfaces/components_LeftDrawer_LeftDrawer.InterfaceLeftDrawerProps.md
@@ -16,13 +16,13 @@
### hideDrawer
-• **hideDrawer**: ``null`` \| `boolean`
+• **hideDrawer**: `null` \| `boolean`
#### Defined in
[src/components/LeftDrawer/LeftDrawer.tsx:16](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawer/LeftDrawer.tsx#L16)
-___
+---
### screenName
@@ -32,11 +32,11 @@ ___
[src/components/LeftDrawer/LeftDrawer.tsx:18](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/LeftDrawer/LeftDrawer.tsx#L18)
-___
+---
### setHideDrawer
-• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<``null`` \| `boolean`\>\>
+• **setHideDrawer**: `Dispatch`\<`SetStateAction`\<`null` \| `boolean`\>\>
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_OrgProfileFieldSettings_OrgProfileFieldSettings.InterfaceCustomFieldData.md b/talawa-admin-docs/interfaces/components_OrgProfileFieldSettings_OrgProfileFieldSettings.InterfaceCustomFieldData.md
index 7ccadce5c2..2b40cac4b1 100644
--- a/talawa-admin-docs/interfaces/components_OrgProfileFieldSettings_OrgProfileFieldSettings.InterfaceCustomFieldData.md
+++ b/talawa-admin-docs/interfaces/components_OrgProfileFieldSettings_OrgProfileFieldSettings.InterfaceCustomFieldData.md
@@ -21,7 +21,7 @@
[src/components/OrgProfileFieldSettings/OrgProfileFieldSettings.tsx:18](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrgProfileFieldSettings/OrgProfileFieldSettings.tsx#L18)
-___
+---
### type
diff --git a/talawa-admin-docs/interfaces/components_OrganizationDashCards_CardItem.InterfaceCardItem.md b/talawa-admin-docs/interfaces/components_OrganizationDashCards_CardItem.InterfaceCardItem.md
index 5efc787028..d2fbaeb495 100644
--- a/talawa-admin-docs/interfaces/components_OrganizationDashCards_CardItem.InterfaceCardItem.md
+++ b/talawa-admin-docs/interfaces/components_OrganizationDashCards_CardItem.InterfaceCardItem.md
@@ -26,7 +26,7 @@
[src/components/OrganizationDashCards/CardItem.tsx:17](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L17)
-___
+---
### enddate
@@ -36,7 +36,7 @@ ___
[src/components/OrganizationDashCards/CardItem.tsx:16](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L16)
-___
+---
### location
@@ -46,7 +46,7 @@ ___
[src/components/OrganizationDashCards/CardItem.tsx:18](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L18)
-___
+---
### startdate
@@ -56,7 +56,7 @@ ___
[src/components/OrganizationDashCards/CardItem.tsx:15](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L15)
-___
+---
### time
@@ -66,7 +66,7 @@ ___
[src/components/OrganizationDashCards/CardItem.tsx:14](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L14)
-___
+---
### title
@@ -76,11 +76,11 @@ ___
[src/components/OrganizationDashCards/CardItem.tsx:13](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationDashCards/CardItem.tsx#L13)
-___
+---
### type
-• **type**: ``"Event"`` \| ``"Post"`` \| ``"MembershipRequest"``
+• **type**: `"Event"` \| `"Post"` \| `"MembershipRequest"`
#### Defined in
diff --git a/talawa-admin-docs/interfaces/components_OrganizationScreen_OrganizationScreen.InterfaceOrganizationScreenProps.md b/talawa-admin-docs/interfaces/components_OrganizationScreen_OrganizationScreen.InterfaceOrganizationScreenProps.md
index 1b6a706c65..ed6313ed34 100644
--- a/talawa-admin-docs/interfaces/components_OrganizationScreen_OrganizationScreen.InterfaceOrganizationScreenProps.md
+++ b/talawa-admin-docs/interfaces/components_OrganizationScreen_OrganizationScreen.InterfaceOrganizationScreenProps.md
@@ -22,7 +22,7 @@
[src/components/OrganizationScreen/OrganizationScreen.tsx:12](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationScreen/OrganizationScreen.tsx#L12)
-___
+---
### screenName
@@ -32,7 +32,7 @@ ___
[src/components/OrganizationScreen/OrganizationScreen.tsx:11](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrganizationScreen/OrganizationScreen.tsx#L11)
-___
+---
### title
diff --git a/talawa-admin-docs/interfaces/components_SuperAdminScreen_SuperAdminScreen.InterfaceSuperAdminScreenProps.md b/talawa-admin-docs/interfaces/components_SuperAdminScreen_SuperAdminScreen.InterfaceSuperAdminScreenProps.md
index f6f3b33975..5951a7cd9b 100644
--- a/talawa-admin-docs/interfaces/components_SuperAdminScreen_SuperAdminScreen.InterfaceSuperAdminScreenProps.md
+++ b/talawa-admin-docs/interfaces/components_SuperAdminScreen_SuperAdminScreen.InterfaceSuperAdminScreenProps.md
@@ -22,7 +22,7 @@
[src/components/SuperAdminScreen/SuperAdminScreen.tsx:9](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/SuperAdminScreen/SuperAdminScreen.tsx#L9)
-___
+---
### screenName
@@ -32,7 +32,7 @@ ___
[src/components/SuperAdminScreen/SuperAdminScreen.tsx:8](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/SuperAdminScreen/SuperAdminScreen.tsx#L8)
-___
+---
### title
diff --git a/talawa-admin-docs/interfaces/components_TableLoader_TableLoader.InterfaceTableLoader.md b/talawa-admin-docs/interfaces/components_TableLoader_TableLoader.InterfaceTableLoader.md
index e545773409..cd75ea1e73 100644
--- a/talawa-admin-docs/interfaces/components_TableLoader_TableLoader.InterfaceTableLoader.md
+++ b/talawa-admin-docs/interfaces/components_TableLoader_TableLoader.InterfaceTableLoader.md
@@ -22,7 +22,7 @@
[src/components/TableLoader/TableLoader.tsx:7](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/TableLoader/TableLoader.tsx#L7)
-___
+---
### noOfCols
@@ -32,7 +32,7 @@ ___
[src/components/TableLoader/TableLoader.tsx:8](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/TableLoader/TableLoader.tsx#L8)
-___
+---
### noOfRows
diff --git a/talawa-admin-docs/modules/components_AddOn_AddOn.md b/talawa-admin-docs/modules/components_AddOn_AddOn.md
index 7708f3efc0..d28a6f8e61 100644
--- a/talawa-admin-docs/modules/components_AddOn_AddOn.md
+++ b/talawa-admin-docs/modules/components_AddOn_AddOn.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------------- |
| `«destructured»` | `InterfaceAddOnProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntry.md b/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntry.md
index d5d4096e0d..1209bfee4f 100644
--- a/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntry.md
+++ b/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntry.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------- |
| `«destructured»` | `InterfaceAddOnEntryProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntryMocks.md b/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntryMocks.md
index 2a0b34d8cf..247b387ead 100644
--- a/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntryMocks.md
+++ b/talawa-admin-docs/modules/components_AddOn_core_AddOnEntry_AddOnEntryMocks.md
@@ -6,13 +6,13 @@
### Variables
-- [ADD\_ON\_ENTRY\_MOCK](components_AddOn_core_AddOnEntry_AddOnEntryMocks.md#add_on_entry_mock)
+- [ADD_ON_ENTRY_MOCK](components_AddOn_core_AddOnEntry_AddOnEntryMocks.md#add_on_entry_mock)
## Variables
-### ADD\_ON\_ENTRY\_MOCK
+### ADD_ON_ENTRY_MOCK
-• `Const` **ADD\_ON\_ENTRY\_MOCK**: \{ `request`: \{ `query`: `DocumentNode` = UPDATE\_INSTALL\_STATUS\_PLUGIN\_MUTATION; `variables`: \{ `id`: `string` = '1'; `orgId`: `string` = 'undefined' \} \} ; `result`: \{ `data`: \{ `updatePluginStatus`: \{ `_id`: `string` = '123'; `pluginCreatedBy`: `string` = 'John Doe'; `pluginDesc`: `string` = 'This is a sample plugin description.'; `pluginName`: `string` = 'Sample Plugin'; `uninstalledOrgs`: `never`[] = [] \} = updatePluginStatus \} \} \}[]
+• `Const` **ADD_ON_ENTRY_MOCK**: \{ `request`: \{ `query`: `DocumentNode` = UPDATE_INSTALL_STATUS_PLUGIN_MUTATION; `variables`: \{ `id`: `string` = '1'; `orgId`: `string` = 'undefined' \} \} ; `result`: \{ `data`: \{ `updatePluginStatus`: \{ `_id`: `string` = '123'; `pluginCreatedBy`: `string` = 'John Doe'; `pluginDesc`: `string` = 'This is a sample plugin description.'; `pluginName`: `string` = 'Sample Plugin'; `uninstalledOrgs`: `never`[] = [] \} = updatePluginStatus \} \} \}[]
#### Defined in
diff --git a/talawa-admin-docs/modules/components_AddOn_core_AddOnRegister_AddOnRegister.md b/talawa-admin-docs/modules/components_AddOn_core_AddOnRegister_AddOnRegister.md
index d6f1d0ac38..ce219e40e5 100644
--- a/talawa-admin-docs/modules/components_AddOn_core_AddOnRegister_AddOnRegister.md
+++ b/talawa-admin-docs/modules/components_AddOn_core_AddOnRegister_AddOnRegister.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :---------------------------- |
| `«destructured»` | `InterfaceAddOnRegisterProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_AddOn_support_components_Action_Action.md b/talawa-admin-docs/modules/components_AddOn_support_components_Action_Action.md
index f93d3d6b94..c26133e4e0 100644
--- a/talawa-admin-docs/modules/components_AddOn_support_components_Action_Action.md
+++ b/talawa-admin-docs/modules/components_AddOn_support_components_Action_Action.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------- |
| `props` | `InterfaceActionProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_AddOn_support_components_MainContent_MainContent.md b/talawa-admin-docs/modules/components_AddOn_support_components_MainContent_MainContent.md
index af9faef144..df72886341 100644
--- a/talawa-admin-docs/modules/components_AddOn_support_components_MainContent_MainContent.md
+++ b/talawa-admin-docs/modules/components_AddOn_support_components_MainContent_MainContent.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------------------- |
| `«destructured»` | `InterfaceMainContentProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_AddOn_support_components_SidePanel_SidePanel.md b/talawa-admin-docs/modules/components_AddOn_support_components_SidePanel_SidePanel.md
index c9411bcff6..7a798f1ae3 100644
--- a/talawa-admin-docs/modules/components_AddOn_support_components_SidePanel_SidePanel.md
+++ b/talawa-admin-docs/modules/components_AddOn_support_components_SidePanel_SidePanel.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------ |
| `«destructured»` | `InterfaceSidePanelProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementEntry_AdvertisementEntry.md b/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementEntry_AdvertisementEntry.md
index 22f50c02b7..3de7548c5d 100644
--- a/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementEntry_AdvertisementEntry.md
+++ b/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementEntry_AdvertisementEntry.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------- |
| `«destructured»` | `InterfaceAddOnEntryProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementRegister_AdvertisementRegister.md b/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementRegister_AdvertisementRegister.md
index 5a91b6e729..82f3dabaa6 100644
--- a/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementRegister_AdvertisementRegister.md
+++ b/talawa-admin-docs/modules/components_Advertisements_core_AdvertisementRegister_AdvertisementRegister.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :---------------------------- |
| `«destructured»` | `InterfaceAddOnRegisterProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_ChangeLanguageDropdown_ChangeLanguageDropDown.md b/talawa-admin-docs/modules/components_ChangeLanguageDropdown_ChangeLanguageDropDown.md
index 44f300753c..0d75d2b576 100644
--- a/talawa-admin-docs/modules/components_ChangeLanguageDropdown_ChangeLanguageDropDown.md
+++ b/talawa-admin-docs/modules/components_ChangeLanguageDropdown_ChangeLanguageDropDown.md
@@ -17,8 +17,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------------- | :------- |
| `languageCode` | `string` |
#### Returns
@@ -29,7 +29,7 @@
[src/components/ChangeLanguageDropdown/ChangeLanguageDropDown.tsx:13](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/ChangeLanguageDropdown/ChangeLanguageDropDown.tsx#L13)
-___
+---
### default
@@ -37,8 +37,8 @@ ___
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------------- |
| `props` | `InterfaceChangeLanguageDropDownProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_CheckIn_CheckInModal.md b/talawa-admin-docs/modules/components_CheckIn_CheckInModal.md
index ccee8edbae..7fecbcecd2 100644
--- a/talawa-admin-docs/modules/components_CheckIn_CheckInModal.md
+++ b/talawa-admin-docs/modules/components_CheckIn_CheckInModal.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------------------------------------------------------------------- |
| `props` | [`InterfaceModalProp`](../interfaces/components_CheckIn_types.InterfaceModalProp.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_CheckIn_CheckInWrapper.md b/talawa-admin-docs/modules/components_CheckIn_CheckInWrapper.md
index 9bee87f600..8cd6c4ef99 100644
--- a/talawa-admin-docs/modules/components_CheckIn_CheckInWrapper.md
+++ b/talawa-admin-docs/modules/components_CheckIn_CheckInWrapper.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------- |
| `props` | `PropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_CheckIn_TableRow.md b/talawa-admin-docs/modules/components_CheckIn_TableRow.md
index aceaaf32f1..b20e312d53 100644
--- a/talawa-admin-docs/modules/components_CheckIn_TableRow.md
+++ b/talawa-admin-docs/modules/components_CheckIn_TableRow.md
@@ -16,11 +16,11 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `«destructured»` | `Object` |
-| › `data` | [`InterfaceTableCheckIn`](../interfaces/components_CheckIn_types.InterfaceTableCheckIn.md) |
-| › `refetch` | () =\> `void` |
+| Name | Type |
+| :--------------- | :----------------------------------------------------------------------------------------- |
+| `«destructured»` | `Object` |
+| › `data` | [`InterfaceTableCheckIn`](../interfaces/components_CheckIn_types.InterfaceTableCheckIn.md) |
+| › `refetch` | () =\> `void` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_CheckIn_mocks.md b/talawa-admin-docs/modules/components_CheckIn_mocks.md
index 209120d33e..2544697f4a 100644
--- a/talawa-admin-docs/modules/components_CheckIn_mocks.md
+++ b/talawa-admin-docs/modules/components_CheckIn_mocks.md
@@ -14,27 +14,27 @@
### checkInMutationSuccess
-• `Const` **checkInMutationSuccess**: \{ `request`: \{ `query`: `DocumentNode` = MARK\_CHECKIN; `variables`: \{ `allotedRoom`: `string` = ''; `allotedSeat`: `string` = ''; `eventId`: `string` = 'event123'; `userId`: `string` = 'user123' \} \} ; `result`: \{ `data`: \{ `checkIn`: \{ `_id`: `string` = '123' \} \} \} \}[]
+• `Const` **checkInMutationSuccess**: \{ `request`: \{ `query`: `DocumentNode` = MARK_CHECKIN; `variables`: \{ `allotedRoom`: `string` = ''; `allotedSeat`: `string` = ''; `eventId`: `string` = 'event123'; `userId`: `string` = 'user123' \} \} ; `result`: \{ `data`: \{ `checkIn`: \{ `_id`: `string` = '123' \} \} \} \}[]
#### Defined in
[src/components/CheckIn/mocks.ts:48](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/mocks.ts#L48)
-___
+---
### checkInMutationUnsuccess
-• `Const` **checkInMutationUnsuccess**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = MARK\_CHECKIN; `variables`: \{ `allotedRoom`: `string` = ''; `allotedSeat`: `string` = ''; `eventId`: `string` = 'event123'; `userId`: `string` = 'user123' \} \} \}[]
+• `Const` **checkInMutationUnsuccess**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = MARK_CHECKIN; `variables`: \{ `allotedRoom`: `string` = ''; `allotedSeat`: `string` = ''; `eventId`: `string` = 'event123'; `userId`: `string` = 'user123' \} \} \}[]
#### Defined in
[src/components/CheckIn/mocks.ts:69](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/CheckIn/mocks.ts#L69)
-___
+---
### checkInQueryMock
-• `Const` **checkInQueryMock**: \{ `request`: \{ `query`: `DocumentNode` = EVENT\_CHECKINS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: [`InterfaceAttendeeQueryResponse`](../interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md) = checkInQueryData \} \}[]
+• `Const` **checkInQueryMock**: \{ `request`: \{ `query`: `DocumentNode` = EVENT_CHECKINS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: [`InterfaceAttendeeQueryResponse`](../interfaces/components_CheckIn_types.InterfaceAttendeeQueryResponse.md) = checkInQueryData \} \}[]
#### Defined in
diff --git a/talawa-admin-docs/modules/components_CollapsibleDropdown_CollapsibleDropdown.md b/talawa-admin-docs/modules/components_CollapsibleDropdown_CollapsibleDropdown.md
index 0811628058..26b56c6199 100644
--- a/talawa-admin-docs/modules/components_CollapsibleDropdown_CollapsibleDropdown.md
+++ b/talawa-admin-docs/modules/components_CollapsibleDropdown_CollapsibleDropdown.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :--------------------------------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceCollapsibleDropdown`](../interfaces/components_CollapsibleDropdown_CollapsibleDropdown.InterfaceCollapsibleDropdown.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_ContriStats_ContriStats.md b/talawa-admin-docs/modules/components_ContriStats_ContriStats.md
index 07484f6961..1a1e5bc548 100644
--- a/talawa-admin-docs/modules/components_ContriStats_ContriStats.md
+++ b/talawa-admin-docs/modules/components_ContriStats_ContriStats.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------- |
| `props` | `InterfaceContriStatsProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EditCustomFieldDropDown_EditCustomFieldDropDown.md b/talawa-admin-docs/modules/components_EditCustomFieldDropDown_EditCustomFieldDropDown.md
index 740b3130a9..ed378a221a 100644
--- a/talawa-admin-docs/modules/components_EditCustomFieldDropDown_EditCustomFieldDropDown.md
+++ b/talawa-admin-docs/modules/components_EditCustomFieldDropDown_EditCustomFieldDropDown.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------------------- |
| `props` | `InterfaceEditCustomFieldDropDownProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventCalendar_EventCalendar.md b/talawa-admin-docs/modules/components_EventCalendar_EventCalendar.md
index be0467b5cb..d1b6930319 100644
--- a/talawa-admin-docs/modules/components_EventCalendar_EventCalendar.md
+++ b/talawa-admin-docs/modules/components_EventCalendar_EventCalendar.md
@@ -16,18 +16,18 @@
### default
-▸ **default**(`props`, `context?`): ``null`` \| `ReactElement`\<`any`, `any`\>
+▸ **default**(`props`, `context?`): `null` \| `ReactElement`\<`any`, `any`\>
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `PropsWithChildren`\<`InterfaceCalendarProps`\> |
-| `context?` | `any` |
+| Name | Type |
+| :--------- | :---------------------------------------------- |
+| `props` | `PropsWithChildren`\<`InterfaceCalendarProps`\> |
+| `context?` | `any` |
#### Returns
-``null`` \| `ReactElement`\<`any`, `any`\>
+`null` \| `ReactElement`\<`any`, `any`\>
#### Defined in
diff --git a/talawa-admin-docs/modules/components_EventListCard_EventListCard.md b/talawa-admin-docs/modules/components_EventListCard_EventListCard.md
index d2df808a4e..ed32343bd5 100644
--- a/talawa-admin-docs/modules/components_EventListCard_EventListCard.md
+++ b/talawa-admin-docs/modules/components_EventListCard_EventListCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :---------------------------- |
| `props` | `InterfaceEventListCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsModal.md b/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsModal.md
index a8458ded95..9605137c04 100644
--- a/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsModal.md
+++ b/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsModal.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------- |
| `props` | `ModalPropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsWrapper.md b/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsWrapper.md
index 32d3c032d5..1ecb3d1908 100644
--- a/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsWrapper.md
+++ b/talawa-admin-docs/modules/components_EventRegistrantsModal_EventRegistrantsWrapper.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------- |
| `props` | `PropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventStats_EventStats.md b/talawa-admin-docs/modules/components_EventStats_EventStats.md
index 2278652afb..404c0b2470 100644
--- a/talawa-admin-docs/modules/components_EventStats_EventStats.md
+++ b/talawa-admin-docs/modules/components_EventStats_EventStats.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------- |
| `«destructured»` | `ModalPropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventStats_EventStatsWrapper.md b/talawa-admin-docs/modules/components_EventStats_EventStatsWrapper.md
index d8f65115de..56e56a0aaa 100644
--- a/talawa-admin-docs/modules/components_EventStats_EventStatsWrapper.md
+++ b/talawa-admin-docs/modules/components_EventStats_EventStatsWrapper.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------- |
| `props` | `PropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventStats_Statistics_AverageRating.md b/talawa-admin-docs/modules/components_EventStats_Statistics_AverageRating.md
index 2970784a82..9c87d9cb95 100644
--- a/talawa-admin-docs/modules/components_EventStats_Statistics_AverageRating.md
+++ b/talawa-admin-docs/modules/components_EventStats_Statistics_AverageRating.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------- |
| `«destructured»` | `ModalPropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventStats_Statistics_Feedback.md b/talawa-admin-docs/modules/components_EventStats_Statistics_Feedback.md
index d6c62473e2..0c938037b7 100644
--- a/talawa-admin-docs/modules/components_EventStats_Statistics_Feedback.md
+++ b/talawa-admin-docs/modules/components_EventStats_Statistics_Feedback.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------- |
| `«destructured»` | `ModalPropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_EventStats_Statistics_Review.md b/talawa-admin-docs/modules/components_EventStats_Statistics_Review.md
index 2260ddfa76..91e3766b28 100644
--- a/talawa-admin-docs/modules/components_EventStats_Statistics_Review.md
+++ b/talawa-admin-docs/modules/components_EventStats_Statistics_Review.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :-------------- |
| `«destructured»` | `ModalPropType` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_IconComponent_IconComponent.md b/talawa-admin-docs/modules/components_IconComponent_IconComponent.md
index bc862524a0..e5e902024d 100644
--- a/talawa-admin-docs/modules/components_IconComponent_IconComponent.md
+++ b/talawa-admin-docs/modules/components_IconComponent_IconComponent.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------------------------------------------------------------------------------------------- |
| `props` | [`InterfaceIconComponent`](../interfaces/components_IconComponent_IconComponent.InterfaceIconComponent.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEvent.md b/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEvent.md
index 2305d3dbad..72fab37499 100644
--- a/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEvent.md
+++ b/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEvent.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :----------------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceLeftDrawerProps`](../interfaces/components_LeftDrawerEvent_LeftDrawerEvent.InterfaceLeftDrawerProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEventWrapper.md b/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEventWrapper.md
index e2b5d4d9a6..8cbba37540 100644
--- a/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEventWrapper.md
+++ b/talawa-admin-docs/modules/components_LeftDrawerEvent_LeftDrawerEventWrapper.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :---------------------------------------------------------------------------------------------------------- |
| `props` | [`InterfacePropType`](../interfaces/components_LeftDrawerEvent_LeftDrawerEventWrapper.InterfacePropType.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_LeftDrawerOrg_LeftDrawerOrg.md b/talawa-admin-docs/modules/components_LeftDrawerOrg_LeftDrawerOrg.md
index 6d0b7b40de..49bcb45312 100644
--- a/talawa-admin-docs/modules/components_LeftDrawerOrg_LeftDrawerOrg.md
+++ b/talawa-admin-docs/modules/components_LeftDrawerOrg_LeftDrawerOrg.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceLeftDrawerProps`](../interfaces/components_LeftDrawerOrg_LeftDrawerOrg.InterfaceLeftDrawerProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_LeftDrawer_LeftDrawer.md b/talawa-admin-docs/modules/components_LeftDrawer_LeftDrawer.md
index ae7da72814..cef34ea075 100644
--- a/talawa-admin-docs/modules/components_LeftDrawer_LeftDrawer.md
+++ b/talawa-admin-docs/modules/components_LeftDrawer_LeftDrawer.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceLeftDrawerProps`](../interfaces/components_LeftDrawer_LeftDrawer.InterfaceLeftDrawerProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_Loader_Loader.md b/talawa-admin-docs/modules/components_Loader_Loader.md
index dd36c8b540..7b19bcd94c 100644
--- a/talawa-admin-docs/modules/components_Loader_Loader.md
+++ b/talawa-admin-docs/modules/components_Loader_Loader.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------- |
| `props` | `InterfaceLoaderProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_MemberRequestCard_MemberRequestCard.md b/talawa-admin-docs/modules/components_MemberRequestCard_MemberRequestCard.md
index 62629fd66c..3b4fc4dc36 100644
--- a/talawa-admin-docs/modules/components_MemberRequestCard_MemberRequestCard.md
+++ b/talawa-admin-docs/modules/components_MemberRequestCard_MemberRequestCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------------- |
| `props` | `InterfaceMemberRequestCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_NotFound_NotFound.md b/talawa-admin-docs/modules/components_NotFound_NotFound.md
index 3c27d00110..8e3230358b 100644
--- a/talawa-admin-docs/modules/components_NotFound_NotFound.md
+++ b/talawa-admin-docs/modules/components_NotFound_NotFound.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------- |
| `props` | `InterfaceNotFoundProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgAdminListCard_OrgAdminListCard.md b/talawa-admin-docs/modules/components_OrgAdminListCard_OrgAdminListCard.md
index a502f77abd..0ea36fcda6 100644
--- a/talawa-admin-docs/modules/components_OrgAdminListCard_OrgAdminListCard.md
+++ b/talawa-admin-docs/modules/components_OrgAdminListCard_OrgAdminListCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------------- |
| `props` | `InterfaceOrgPeopleListCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgContriCards_OrgContriCards.md b/talawa-admin-docs/modules/components_OrgContriCards_OrgContriCards.md
index 7793777b0d..c4372a107a 100644
--- a/talawa-admin-docs/modules/components_OrgContriCards_OrgContriCards.md
+++ b/talawa-admin-docs/modules/components_OrgContriCards_OrgContriCards.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------------- |
| `props` | `InterfaceOrgContriCardsProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgListCard_OrgListCard.md b/talawa-admin-docs/modules/components_OrgListCard_OrgListCard.md
index 18bfd4460b..fd37d5ace4 100644
--- a/talawa-admin-docs/modules/components_OrgListCard_OrgListCard.md
+++ b/talawa-admin-docs/modules/components_OrgListCard_OrgListCard.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------------------------------------------------------------------------------------------- |
| `props` | [`InterfaceOrgListCardProps`](../interfaces/components_OrgListCard_OrgListCard.InterfaceOrgListCardProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgPeopleListCard_OrgPeopleListCard.md b/talawa-admin-docs/modules/components_OrgPeopleListCard_OrgPeopleListCard.md
index 3bfe921cb6..a2845e1868 100644
--- a/talawa-admin-docs/modules/components_OrgPeopleListCard_OrgPeopleListCard.md
+++ b/talawa-admin-docs/modules/components_OrgPeopleListCard_OrgPeopleListCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------------- |
| `props` | `InterfaceOrgPeopleListCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgPostCard_OrgPostCard.md b/talawa-admin-docs/modules/components_OrgPostCard_OrgPostCard.md
index abd4ea5663..039691bec3 100644
--- a/talawa-admin-docs/modules/components_OrgPostCard_OrgPostCard.md
+++ b/talawa-admin-docs/modules/components_OrgPostCard_OrgPostCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------- |
| `props` | `InterfaceOrgPostCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdate.md b/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdate.md
index f3671db7f1..6ffa2b243b 100644
--- a/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdate.md
+++ b/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdate.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------ |
| `props` | `InterfaceOrgUpdateProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdateMocks.md b/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdateMocks.md
index 356df928c1..59143e464e 100644
--- a/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdateMocks.md
+++ b/talawa-admin-docs/modules/components_OrgUpdate_OrgUpdateMocks.md
@@ -7,34 +7,34 @@
### Variables
- [MOCKS](components_OrgUpdate_OrgUpdateMocks.md#mocks)
-- [MOCKS\_ERROR\_ORGLIST](components_OrgUpdate_OrgUpdateMocks.md#mocks_error_orglist)
-- [MOCKS\_ERROR\_UPDATE\_ORGLIST](components_OrgUpdate_OrgUpdateMocks.md#mocks_error_update_orglist)
+- [MOCKS_ERROR_ORGLIST](components_OrgUpdate_OrgUpdateMocks.md#mocks_error_orglist)
+- [MOCKS_ERROR_UPDATE_ORGLIST](components_OrgUpdate_OrgUpdateMocks.md#mocks_error_update_orglist)
## Variables
### MOCKS
-• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `organizations`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: `never`[] = []; `creator`: \{ `email`: `string` = 'johndoe@example.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'Equitable Access to STEM Education Jobs'; `image`: ``null`` = null; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'samsmith@gmail.com'; `firstName`: `string` = 'Sam'; `lastName`: `string` = 'Smith' \} \} ; `name`: `string` = 'Palisadoes'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `updateOrganization?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = UPDATE\_ORGANIZATION\_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `id`: `string` = '123'; `image`: `File` ; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} ; `result`: \{ `data`: \{ `organizations?`: `undefined` ; `updateOrganization`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} \} \})[]
+• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `organizations`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: `never`[] = []; `creator`: \{ `email`: `string` = 'johndoe@example.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'Equitable Access to STEM Education Jobs'; `image`: `null` = null; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'samsmith@gmail.com'; `firstName`: `string` = 'Sam'; `lastName`: `string` = 'Smith' \} \} ; `name`: `string` = 'Palisadoes'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `updateOrganization?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = UPDATE_ORGANIZATION_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `id`: `string` = '123'; `image`: `File` ; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} ; `result`: \{ `data`: \{ `organizations?`: `undefined` ; `updateOrganization`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} \} \})[]
#### Defined in
[src/components/OrgUpdate/OrgUpdateMocks.ts:4](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrgUpdate/OrgUpdateMocks.ts#L4)
-___
+---
-### MOCKS\_ERROR\_ORGLIST
+### MOCKS_ERROR_ORGLIST
-• `Const` **MOCKS\_ERROR\_ORGLIST**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST; `variables`: \{ `id`: `string` = '123' \} \} \}[]
+• `Const` **MOCKS_ERROR_ORGLIST**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST; `variables`: \{ `id`: `string` = '123' \} \} \}[]
#### Defined in
[src/components/OrgUpdate/OrgUpdateMocks.ts:109](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/components/OrgUpdate/OrgUpdateMocks.ts#L109)
-___
+---
-### MOCKS\_ERROR\_UPDATE\_ORGLIST
+### MOCKS_ERROR_UPDATE_ORGLIST
-• `Const` **MOCKS\_ERROR\_UPDATE\_ORGLIST**: (\{ `erorr?`: `undefined` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `organizations`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: `never`[] = []; `creator`: \{ `email`: `string` = 'johndoe@example.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'Equitable Access to STEM Education Jobs'; `image`: ``null`` = null; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'samsmith@gmail.com'; `firstName`: `string` = 'Sam'; `lastName`: `string` = 'Smith' \} \} ; `name`: `string` = 'Palisadoes'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] \} \} \} \| \{ `erorr`: `Error` ; `request`: \{ `query`: `DocumentNode` = UPDATE\_ORGANIZATION\_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `id`: `string` = '123'; `image`: `File` ; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} ; `result?`: `undefined` \})[]
+• `Const` **MOCKS_ERROR_UPDATE_ORGLIST**: (\{ `erorr?`: `undefined` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `organizations`: \{ `_id`: `string` = '123'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: `never`[] = []; `creator`: \{ `email`: `string` = 'johndoe@example.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'Equitable Access to STEM Education Jobs'; `image`: `null` = null; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'samsmith@gmail.com'; `firstName`: `string` = 'Sam'; `lastName`: `string` = 'Smith' \} \} ; `name`: `string` = 'Palisadoes'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] \} \} \} \| \{ `erorr`: `Error` ; `request`: \{ `query`: `DocumentNode` = UPDATE_ORGANIZATION_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is an updated test organization'; `id`: `string` = '123'; `image`: `File` ; `name`: `string` = 'Updated Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \} \} ; `result?`: `undefined` \})[]
#### Defined in
diff --git a/talawa-admin-docs/modules/components_OrganizationCardStart_OrganizationCardStart.md b/talawa-admin-docs/modules/components_OrganizationCardStart_OrganizationCardStart.md
index 1f3d0f7f15..d1d8e73992 100644
--- a/talawa-admin-docs/modules/components_OrganizationCardStart_OrganizationCardStart.md
+++ b/talawa-admin-docs/modules/components_OrganizationCardStart_OrganizationCardStart.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------------ |
| `props` | `InterfaceOrganizationCardStartProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrganizationCard_OrganizationCard.md b/talawa-admin-docs/modules/components_OrganizationCard_OrganizationCard.md
index e72bc4b169..1c22ab5b6d 100644
--- a/talawa-admin-docs/modules/components_OrganizationCard_OrganizationCard.md
+++ b/talawa-admin-docs/modules/components_OrganizationCard_OrganizationCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------- |
| `props` | `InterfaceOrganizationCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrganizationDashCards_CardItem.md b/talawa-admin-docs/modules/components_OrganizationDashCards_CardItem.md
index 149ddf73b5..c18bbc3fef 100644
--- a/talawa-admin-docs/modules/components_OrganizationDashCards_CardItem.md
+++ b/talawa-admin-docs/modules/components_OrganizationDashCards_CardItem.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------------------------------------------------------------------------------- |
| `props` | [`InterfaceCardItem`](../interfaces/components_OrganizationDashCards_CardItem.InterfaceCardItem.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrganizationDashCards_DashboardCard.md b/talawa-admin-docs/modules/components_OrganizationDashCards_DashboardCard.md
index 4874366105..047d74117b 100644
--- a/talawa-admin-docs/modules/components_OrganizationDashCards_DashboardCard.md
+++ b/talawa-admin-docs/modules/components_OrganizationDashCards_DashboardCard.md
@@ -16,12 +16,12 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `Object` |
-| `props.count?` | `number` |
-| `props.icon` | `ReactNode` |
-| `props.title` | `string` |
+| Name | Type |
+| :------------- | :---------- |
+| `props` | `Object` |
+| `props.count?` | `number` |
+| `props.icon` | `ReactNode` |
+| `props.title` | `string` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_OrganizationScreen_OrganizationScreen.md b/talawa-admin-docs/modules/components_OrganizationScreen_OrganizationScreen.md
index 8abc38e92f..30fc7c98d4 100644
--- a/talawa-admin-docs/modules/components_OrganizationScreen_OrganizationScreen.md
+++ b/talawa-admin-docs/modules/components_OrganizationScreen_OrganizationScreen.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :--------------------------------------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceOrganizationScreenProps`](../interfaces/components_OrganizationScreen_OrganizationScreen.InterfaceOrganizationScreenProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_PaginationList_PaginationList.md b/talawa-admin-docs/modules/components_PaginationList_PaginationList.md
index 2b35c78487..be43d62761 100644
--- a/talawa-admin-docs/modules/components_PaginationList_PaginationList.md
+++ b/talawa-admin-docs/modules/components_PaginationList_PaginationList.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------ |
| `props` | `InterfacePropsInterface` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_Pagination_Pagination.md b/talawa-admin-docs/modules/components_Pagination_Pagination.md
index d4d4be6cd8..93d7eb4bec 100644
--- a/talawa-admin-docs/modules/components_Pagination_Pagination.md
+++ b/talawa-admin-docs/modules/components_Pagination_Pagination.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------------- |
| `props` | `InterfaceTablePaginationActionsProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_SecuredRoute_SecuredRoute.md b/talawa-admin-docs/modules/components_SecuredRoute_SecuredRoute.md
index 1f48f3cb87..811b366346 100644
--- a/talawa-admin-docs/modules/components_SecuredRoute_SecuredRoute.md
+++ b/talawa-admin-docs/modules/components_SecuredRoute_SecuredRoute.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :---- |
| `props` | `any` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_SuperAdminScreen_SuperAdminScreen.md b/talawa-admin-docs/modules/components_SuperAdminScreen_SuperAdminScreen.md
index 11c00f6131..cce639104a 100644
--- a/talawa-admin-docs/modules/components_SuperAdminScreen_SuperAdminScreen.md
+++ b/talawa-admin-docs/modules/components_SuperAdminScreen_SuperAdminScreen.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :--------------- | :------------------------------------------------------------------------------------------------------------------------------- |
| `«destructured»` | [`InterfaceSuperAdminScreenProps`](../interfaces/components_SuperAdminScreen_SuperAdminScreen.InterfaceSuperAdminScreenProps.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_TableLoader_TableLoader.md b/talawa-admin-docs/modules/components_TableLoader_TableLoader.md
index 9874a5c25e..7681dc0263 100644
--- a/talawa-admin-docs/modules/components_TableLoader_TableLoader.md
+++ b/talawa-admin-docs/modules/components_TableLoader_TableLoader.md
@@ -20,8 +20,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------------------------------------------------------------------------- |
| `props` | [`InterfaceTableLoader`](../interfaces/components_TableLoader_TableLoader.InterfaceTableLoader.md) |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserListCard_UserListCard.md b/talawa-admin-docs/modules/components_UserListCard_UserListCard.md
index f8f040f88e..f6a564ba38 100644
--- a/talawa-admin-docs/modules/components_UserListCard_UserListCard.md
+++ b/talawa-admin-docs/modules/components_UserListCard_UserListCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------------- |
| `props` | `InterfaceUserListCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPasswordUpdate_UserPasswordUpdate.md b/talawa-admin-docs/modules/components_UserPasswordUpdate_UserPasswordUpdate.md
index 517a6d692f..b7743fed04 100644
--- a/talawa-admin-docs/modules/components_UserPasswordUpdate_UserPasswordUpdate.md
+++ b/talawa-admin-docs/modules/components_UserPasswordUpdate_UserPasswordUpdate.md
@@ -12,18 +12,18 @@
### default
-▸ **default**(`props`, `context?`): ``null`` \| `ReactElement`\<`any`, `any`\>
+▸ **default**(`props`, `context?`): `null` \| `ReactElement`\<`any`, `any`\>
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `PropsWithChildren`\<`InterfaceUserPasswordUpdateProps`\> |
-| `context?` | `any` |
+| Name | Type |
+| :--------- | :-------------------------------------------------------- |
+| `props` | `PropsWithChildren`\<`InterfaceUserPasswordUpdateProps`\> |
+| `context?` | `any` |
#### Returns
-``null`` \| `ReactElement`\<`any`, `any`\>
+`null` \| `ReactElement`\<`any`, `any`\>
#### Defined in
diff --git a/talawa-admin-docs/modules/components_UserPortal_ChatRoom_ChatRoom.md b/talawa-admin-docs/modules/components_UserPortal_ChatRoom_ChatRoom.md
index 84480b9f02..18840f6280 100644
--- a/talawa-admin-docs/modules/components_UserPortal_ChatRoom_ChatRoom.md
+++ b/talawa-admin-docs/modules/components_UserPortal_ChatRoom_ChatRoom.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------- |
| `props` | `InterfaceChatRoomProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_CommentCard_CommentCard.md b/talawa-admin-docs/modules/components_UserPortal_CommentCard_CommentCard.md
index 9d36774a58..981aff15a2 100644
--- a/talawa-admin-docs/modules/components_UserPortal_CommentCard_CommentCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_CommentCard_CommentCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------- |
| `props` | `InterfaceCommentCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_ContactCard_ContactCard.md b/talawa-admin-docs/modules/components_UserPortal_ContactCard_ContactCard.md
index f40934d0f8..a611ce7fde 100644
--- a/talawa-admin-docs/modules/components_UserPortal_ContactCard_ContactCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_ContactCard_ContactCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------------- |
| `props` | `InterfaceContactCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_DonationCard_DonationCard.md b/talawa-admin-docs/modules/components_UserPortal_DonationCard_DonationCard.md
index c40b5fd806..c341b29f49 100644
--- a/talawa-admin-docs/modules/components_UserPortal_DonationCard_DonationCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_DonationCard_DonationCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------------- |
| `props` | `InterfaceDonationCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_EventCard_EventCard.md b/talawa-admin-docs/modules/components_UserPortal_EventCard_EventCard.md
index 564eb9afa7..0c53e65e52 100644
--- a/talawa-admin-docs/modules/components_UserPortal_EventCard_EventCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_EventCard_EventCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------ |
| `props` | `InterfaceEventCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_Login_Login.md b/talawa-admin-docs/modules/components_UserPortal_Login_Login.md
index 4b80b3969f..685641f4b4 100644
--- a/talawa-admin-docs/modules/components_UserPortal_Login_Login.md
+++ b/talawa-admin-docs/modules/components_UserPortal_Login_Login.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :-------------------- |
| `props` | `InterfaceLoginProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_OrganizationCard_OrganizationCard.md b/talawa-admin-docs/modules/components_UserPortal_OrganizationCard_OrganizationCard.md
index c7b1e8643e..f064f7376a 100644
--- a/talawa-admin-docs/modules/components_UserPortal_OrganizationCard_OrganizationCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_OrganizationCard_OrganizationCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------- |
| `props` | `InterfaceOrganizationCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_OrganizationNavbar_OrganizationNavbar.md b/talawa-admin-docs/modules/components_UserPortal_OrganizationNavbar_OrganizationNavbar.md
index 492e7a44c7..bf846a1b41 100644
--- a/talawa-admin-docs/modules/components_UserPortal_OrganizationNavbar_OrganizationNavbar.md
+++ b/talawa-admin-docs/modules/components_UserPortal_OrganizationNavbar_OrganizationNavbar.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :--------------------- |
| `props` | `InterfaceNavbarProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_PeopleCard_PeopleCard.md b/talawa-admin-docs/modules/components_UserPortal_PeopleCard_PeopleCard.md
index a3497d6bca..d09695bbb6 100644
--- a/talawa-admin-docs/modules/components_UserPortal_PeopleCard_PeopleCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_PeopleCard_PeopleCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------------------------------- |
| `props` | `InterfaceOrganizationCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_PostCard_PostCard.md b/talawa-admin-docs/modules/components_UserPortal_PostCard_PostCard.md
index 533ef95663..e11e7a295b 100644
--- a/talawa-admin-docs/modules/components_UserPortal_PostCard_PostCard.md
+++ b/talawa-admin-docs/modules/components_UserPortal_PostCard_PostCard.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------- |
| `props` | `InterfacePostCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_PromotedPost_PromotedPost.md b/talawa-admin-docs/modules/components_UserPortal_PromotedPost_PromotedPost.md
index 8ce7350f58..f3b92a9289 100644
--- a/talawa-admin-docs/modules/components_UserPortal_PromotedPost_PromotedPost.md
+++ b/talawa-admin-docs/modules/components_UserPortal_PromotedPost_PromotedPost.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------- |
| `props` | `InterfacePostCardProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_Register_Register.md b/talawa-admin-docs/modules/components_UserPortal_Register_Register.md
index 7865759c03..d9ce59e738 100644
--- a/talawa-admin-docs/modules/components_UserPortal_Register_Register.md
+++ b/talawa-admin-docs/modules/components_UserPortal_Register_Register.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :----------------------- |
| `props` | `InterfaceRegisterProps` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserPortal_SecuredRouteForUser_SecuredRouteForUser.md b/talawa-admin-docs/modules/components_UserPortal_SecuredRouteForUser_SecuredRouteForUser.md
index b834c74894..bc22357b68 100644
--- a/talawa-admin-docs/modules/components_UserPortal_SecuredRouteForUser_SecuredRouteForUser.md
+++ b/talawa-admin-docs/modules/components_UserPortal_SecuredRouteForUser_SecuredRouteForUser.md
@@ -16,8 +16,8 @@
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :---- |
| `props` | `any` |
#### Returns
diff --git a/talawa-admin-docs/modules/components_UserUpdate_UserUpdate.md b/talawa-admin-docs/modules/components_UserUpdate_UserUpdate.md
index 6e9f01258e..06c0d030b8 100644
--- a/talawa-admin-docs/modules/components_UserUpdate_UserUpdate.md
+++ b/talawa-admin-docs/modules/components_UserUpdate_UserUpdate.md
@@ -12,18 +12,18 @@
### default
-▸ **default**(`props`, `context?`): ``null`` \| `ReactElement`\<`any`, `any`\>
+▸ **default**(`props`, `context?`): `null` \| `ReactElement`\<`any`, `any`\>
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `PropsWithChildren`\<`InterfaceUserUpdateProps`\> |
-| `context?` | `any` |
+| Name | Type |
+| :--------- | :------------------------------------------------ |
+| `props` | `PropsWithChildren`\<`InterfaceUserUpdateProps`\> |
+| `context?` | `any` |
#### Returns
-``null`` \| `ReactElement`\<`any`, `any`\>
+`null` \| `ReactElement`\<`any`, `any`\>
#### Defined in
diff --git a/talawa-admin-docs/modules/components_UsersTableItem_UserTableItemMocks.md b/talawa-admin-docs/modules/components_UsersTableItem_UserTableItemMocks.md
index 85305b69e0..17e530c1c0 100644
--- a/talawa-admin-docs/modules/components_UsersTableItem_UserTableItemMocks.md
+++ b/talawa-admin-docs/modules/components_UsersTableItem_UserTableItemMocks.md
@@ -12,7 +12,7 @@
### MOCKS
-• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = UPDATE\_USERTYPE\_MUTATION; `variables`: \{ `id`: `string` = '123'; `organizationId?`: `undefined` = 'abc'; `orgid?`: `undefined` = 'abc'; `role?`: `undefined` = 'ADMIN'; `userId?`: `undefined` = '123'; `userType`: `string` = 'ADMIN'; `userid?`: `undefined` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember?`: `undefined` ; `updateUserRoleInOrganization?`: `undefined` ; `updateUserType`: \{ `data`: \{ `id`: `string` = '123' \} \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = REMOVE\_MEMBER\_MUTATION; `variables`: \{ `id?`: `undefined` = '456'; `organizationId?`: `undefined` = 'abc'; `orgid`: `string` = 'abc'; `role?`: `undefined` = 'ADMIN'; `userId?`: `undefined` = '123'; `userType?`: `undefined` = 'ADMIN'; `userid`: `string` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember`: \{ `_id`: `string` = '123' \} ; `updateUserRoleInOrganization?`: `undefined` ; `updateUserType?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = UPDATE\_USER\_ROLE\_IN\_ORG\_MUTATION; `variables`: \{ `id?`: `undefined` = '456'; `organizationId`: `string` = 'abc'; `orgid?`: `undefined` = 'abc'; `role`: `string` = 'ADMIN'; `userId`: `string` = '123'; `userType?`: `undefined` = 'ADMIN'; `userid?`: `undefined` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember?`: `undefined` ; `updateUserRoleInOrganization`: \{ `_id`: `string` = '123' \} ; `updateUserType?`: `undefined` \} \} \})[]
+• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = UPDATE_USERTYPE_MUTATION; `variables`: \{ `id`: `string` = '123'; `organizationId?`: `undefined` = 'abc'; `orgid?`: `undefined` = 'abc'; `role?`: `undefined` = 'ADMIN'; `userId?`: `undefined` = '123'; `userType`: `string` = 'ADMIN'; `userid?`: `undefined` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember?`: `undefined` ; `updateUserRoleInOrganization?`: `undefined` ; `updateUserType`: \{ `data`: \{ `id`: `string` = '123' \} \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = REMOVE_MEMBER_MUTATION; `variables`: \{ `id?`: `undefined` = '456'; `organizationId?`: `undefined` = 'abc'; `orgid`: `string` = 'abc'; `role?`: `undefined` = 'ADMIN'; `userId?`: `undefined` = '123'; `userType?`: `undefined` = 'ADMIN'; `userid`: `string` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember`: \{ `_id`: `string` = '123' \} ; `updateUserRoleInOrganization?`: `undefined` ; `updateUserType?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = UPDATE_USER_ROLE_IN_ORG_MUTATION; `variables`: \{ `id?`: `undefined` = '456'; `organizationId`: `string` = 'abc'; `orgid?`: `undefined` = 'abc'; `role`: `string` = 'ADMIN'; `userId`: `string` = '123'; `userType?`: `undefined` = 'ADMIN'; `userid?`: `undefined` = '123' \} \} ; `result`: \{ `data`: \{ `removeMember?`: `undefined` ; `updateUserRoleInOrganization`: \{ `_id`: `string` = '123' \} ; `updateUserType?`: `undefined` \} \} \})[]
#### Defined in
diff --git a/talawa-admin-docs/modules/components_UsersTableItem_UsersTableItem.md b/talawa-admin-docs/modules/components_UsersTableItem_UsersTableItem.md
index c1e49109f4..8d9f1d9cdb 100644
--- a/talawa-admin-docs/modules/components_UsersTableItem_UsersTableItem.md
+++ b/talawa-admin-docs/modules/components_UsersTableItem_UsersTableItem.md
@@ -16,7 +16,7 @@
#### Parameters
-| Name | Type |
+| Name | Type |
| :------ | :------ |
| `props` | `Props` |
diff --git a/talawa-admin-docs/modules/components_plugins.md b/talawa-admin-docs/modules/components_plugins.md
index a72385a04b..0f393c96ea 100644
--- a/talawa-admin-docs/modules/components_plugins.md
+++ b/talawa-admin-docs/modules/components_plugins.md
@@ -15,7 +15,7 @@
Renames and re-exports [default](components_plugins_DummyPlugin_DummyPlugin.md#default)
-___
+---
### DummyPlugin2
diff --git a/talawa-admin-docs/modules/screens_EventDashboard_EventDashboard_mocks.md b/talawa-admin-docs/modules/screens_EventDashboard_EventDashboard_mocks.md
index 55d32ca1f5..a759f3d8f2 100644
--- a/talawa-admin-docs/modules/screens_EventDashboard_EventDashboard_mocks.md
+++ b/talawa-admin-docs/modules/screens_EventDashboard_EventDashboard_mocks.md
@@ -13,17 +13,17 @@
### queryMockWithTime
-• `Const` **queryMockWithTime**: (\{ `request`: \{ `query`: `DocumentNode` = EVENT\_FEEDBACKS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay?`: `undefined` = false; `attendees?`: `undefined` = []; `averageFeedbackScore`: `number` = 0; `description?`: `undefined` = 'This is a new update'; `endDate?`: `undefined` = '2/2/23'; `endTime?`: `undefined` = '07:00'; `feedback`: `never`[] = []; `location?`: `undefined` = 'New Delhi'; `organization?`: `undefined` ; `startDate?`: `undefined` = '1/1/23'; `startTime?`: `undefined` = '02:00'; `title?`: `undefined` = 'Updated title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT\_DETAILS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay`: `boolean` = false; `attendees`: \{ `_id`: `string` = 'user1' \}[] ; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: `string` = '09:00:00'; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = 'org1'; `members`: \{ `_id`: `string` = 'user1'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] \} ; `startDate`: `string` = '1/1/23'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Event Title' \} \} \} \})[]
+• `Const` **queryMockWithTime**: (\{ `request`: \{ `query`: `DocumentNode` = EVENT_FEEDBACKS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay?`: `undefined` = false; `attendees?`: `undefined` = []; `averageFeedbackScore`: `number` = 0; `description?`: `undefined` = 'This is a new update'; `endDate?`: `undefined` = '2/2/23'; `endTime?`: `undefined` = '07:00'; `feedback`: `never`[] = []; `location?`: `undefined` = 'New Delhi'; `organization?`: `undefined` ; `startDate?`: `undefined` = '1/1/23'; `startTime?`: `undefined` = '02:00'; `title?`: `undefined` = 'Updated title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT_DETAILS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay`: `boolean` = false; `attendees`: \{ `_id`: `string` = 'user1' \}[] ; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: `string` = '09:00:00'; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = 'org1'; `members`: \{ `_id`: `string` = 'user1'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] \} ; `startDate`: `string` = '1/1/23'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Event Title' \} \} \} \})[]
#### Defined in
[src/screens/EventDashboard/EventDashboard.mocks.ts:69](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/EventDashboard/EventDashboard.mocks.ts#L69)
-___
+---
### queryMockWithoutTime
-• `Const` **queryMockWithoutTime**: (\{ `request`: \{ `query`: `DocumentNode` = EVENT\_FEEDBACKS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay?`: `undefined` = false; `attendees?`: `undefined` = []; `averageFeedbackScore`: `number` = 0; `description?`: `undefined` = 'This is a new update'; `endDate?`: `undefined` = '2/2/23'; `endTime?`: `undefined` = '07:00'; `feedback`: `never`[] = []; `location?`: `undefined` = 'New Delhi'; `organization?`: `undefined` ; `startDate?`: `undefined` = '1/1/23'; `startTime?`: `undefined` = '02:00'; `title?`: `undefined` = 'Updated title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT\_DETAILS; `variables`: \{ `id`: `string` = '' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = ''; `allDay`: `boolean` = false; `attendees`: `never`[] = []; `averageFeedbackScore?`: `undefined` = 0; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: `string` = '09:00:00'; `feedback?`: `undefined` = []; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = ''; `members`: `never`[] = [] \} ; `startDate`: `string` = '1/1/23'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Event Title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT\_DETAILS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay`: `boolean` = false; `attendees`: \{ `_id`: `string` = 'user1' \}[] ; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: ``null`` = null; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = 'org1'; `members`: \{ `_id`: `string` = 'user1'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] \} ; `startDate`: `string` = '1/1/23'; `startTime`: ``null`` = null; `title`: `string` = 'Event Title' \} \} \} \})[]
+• `Const` **queryMockWithoutTime**: (\{ `request`: \{ `query`: `DocumentNode` = EVENT_FEEDBACKS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay?`: `undefined` = false; `attendees?`: `undefined` = []; `averageFeedbackScore`: `number` = 0; `description?`: `undefined` = 'This is a new update'; `endDate?`: `undefined` = '2/2/23'; `endTime?`: `undefined` = '07:00'; `feedback`: `never`[] = []; `location?`: `undefined` = 'New Delhi'; `organization?`: `undefined` ; `startDate?`: `undefined` = '1/1/23'; `startTime?`: `undefined` = '02:00'; `title?`: `undefined` = 'Updated title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT_DETAILS; `variables`: \{ `id`: `string` = '' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = ''; `allDay`: `boolean` = false; `attendees`: `never`[] = []; `averageFeedbackScore?`: `undefined` = 0; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: `string` = '09:00:00'; `feedback?`: `undefined` = []; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = ''; `members`: `never`[] = [] \} ; `startDate`: `string` = '1/1/23'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Event Title' \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = EVENT_DETAILS; `variables`: \{ `id`: `string` = 'event123' \} \} ; `result`: \{ `data`: \{ `event`: \{ `_id`: `string` = 'event123'; `allDay`: `boolean` = false; `attendees`: \{ `_id`: `string` = 'user1' \}[] ; `description`: `string` = 'Event Description'; `endDate`: `string` = '2/2/23'; `endTime`: `null` = null; `location`: `string` = 'India'; `organization`: \{ `_id`: `string` = 'org1'; `members`: \{ `_id`: `string` = 'user1'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] \} ; `startDate`: `string` = '1/1/23'; `startTime`: `null` = null; `title`: `string` = 'Event Title' \} \} \} \})[]
#### Defined in
diff --git a/talawa-admin-docs/modules/screens_MemberDetail_MemberDetail.md b/talawa-admin-docs/modules/screens_MemberDetail_MemberDetail.md
index 3775c53c7c..c3f093014a 100644
--- a/talawa-admin-docs/modules/screens_MemberDetail_MemberDetail.md
+++ b/talawa-admin-docs/modules/screens_MemberDetail_MemberDetail.md
@@ -14,24 +14,24 @@
### default
-▸ **default**(`props`, `context?`): ``null`` \| `ReactElement`\<`any`, `any`\>
+▸ **default**(`props`, `context?`): `null` \| `ReactElement`\<`any`, `any`\>
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `PropsWithChildren`\<`MemberDetailProps`\> |
-| `context?` | `any` |
+| Name | Type |
+| :--------- | :----------------------------------------- |
+| `props` | `PropsWithChildren`\<`MemberDetailProps`\> |
+| `context?` | `any` |
#### Returns
-``null`` \| `ReactElement`\<`any`, `any`\>
+`null` \| `ReactElement`\<`any`, `any`\>
#### Defined in
[src/screens/MemberDetail/MemberDetail.tsx:28](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/MemberDetail/MemberDetail.tsx#L28)
-___
+---
### getLanguageName
@@ -39,8 +39,8 @@ ___
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :----- | :------- |
| `code` | `string` |
#### Returns
@@ -51,7 +51,7 @@ ___
[src/screens/MemberDetail/MemberDetail.tsx:328](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/MemberDetail/MemberDetail.tsx#L328)
-___
+---
### prettyDate
@@ -59,8 +59,8 @@ ___
#### Parameters
-| Name | Type |
-| :------ | :------ |
+| Name | Type |
+| :------ | :------- |
| `param` | `string` |
#### Returns
diff --git a/talawa-admin-docs/modules/screens_OrgList_OrgListMocks.md b/talawa-admin-docs/modules/screens_OrgList_OrgListMocks.md
index 95d493570c..86e07fd1c9 100644
--- a/talawa-admin-docs/modules/screens_OrgList_OrgListMocks.md
+++ b/talawa-admin-docs/modules/screens_OrgList_OrgListMocks.md
@@ -7,45 +7,45 @@
### Variables
- [MOCKS](screens_OrgList_OrgListMocks.md#mocks)
-- [MOCKS\_ADMIN](screens_OrgList_OrgListMocks.md#mocks_admin)
-- [MOCKS\_EMPTY](screens_OrgList_OrgListMocks.md#mocks_empty)
-- [MOCKS\_WITH\_ERROR](screens_OrgList_OrgListMocks.md#mocks_with_error)
+- [MOCKS_ADMIN](screens_OrgList_OrgListMocks.md#mocks_admin)
+- [MOCKS_EMPTY](screens_OrgList_OrgListMocks.md#mocks_empty)
+- [MOCKS_WITH_ERROR](screens_OrgList_OrgListMocks.md#mocks_with_error)
## Variables
### MOCKS
-• `Const` **MOCKS**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `image?`: `undefined` ; `name?`: `undefined` = ''; `orderBy`: `string` = 'createdAt\_ASC'; `skip`: `number` = 0; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `createOrganization?`: `undefined` ; `createSampleOrganization?`: `undefined` ; `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `orderBy?`: `undefined` = 'createdAt\_ASC'; `skip?`: `undefined` = 0; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE\_SAMPLE\_ORGANIZATION\_MUTATION; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `createOrganization?`: `undefined` ; `createSampleOrganization`: \{ `id`: `string` = '1'; `name`: `string` = 'Sample Organization' \} ; `organizationsConnection?`: `undefined` = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE\_ORGANIZATION\_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is a dummy organization'; `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id?`: `undefined` = '456'; `image`: `string` = ''; `name`: `string` = 'Dummy Organization'; `orderBy?`: `undefined` = 'createdAt\_ASC'; `skip?`: `undefined` = 0; `userRegistrationRequired`: `boolean` = false; `visibleInSearch`: `boolean` = true \} \} ; `result`: \{ `data`: \{ `createOrganization`: \{ `_id`: `string` = '1' \} ; `createSampleOrganization?`: `undefined` ; `organizationsConnection?`: `undefined` = organizations \} \} \})[]
+• `Const` **MOCKS**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `image?`: `undefined` ; `name?`: `undefined` = ''; `orderBy`: `string` = 'createdAt_ASC'; `skip`: `number` = 0; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: \{ `createOrganization?`: `undefined` ; `createSampleOrganization?`: `undefined` ; `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `address?`: `undefined` ; `description?`: `undefined` = 'This is a new update'; `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `image?`: `undefined` ; `name?`: `undefined` = ''; `orderBy?`: `undefined` = 'createdAt_ASC'; `skip?`: `undefined` = 0; `userRegistrationRequired?`: `undefined` = true; `visibleInSearch?`: `undefined` = false \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE_SAMPLE_ORGANIZATION_MUTATION; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `createOrganization?`: `undefined` ; `createSampleOrganization`: \{ `id`: `string` = '1'; `name`: `string` = 'Sample Organization' \} ; `organizationsConnection?`: `undefined` = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE_ORGANIZATION_MUTATION; `variables`: \{ `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `description`: `string` = 'This is a dummy organization'; `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id?`: `undefined` = '456'; `image`: `string` = ''; `name`: `string` = 'Dummy Organization'; `orderBy?`: `undefined` = 'createdAt_ASC'; `skip?`: `undefined` = 0; `userRegistrationRequired`: `boolean` = false; `visibleInSearch`: `boolean` = true \} \} ; `result`: \{ `data`: \{ `createOrganization`: \{ `_id`: `string` = '1' \} ; `createSampleOrganization?`: `undefined` ; `organizationsConnection?`: `undefined` = organizations \} \} \})[]
#### Defined in
[src/screens/OrgList/OrgListMocks.ts:101](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/OrgList/OrgListMocks.ts#L101)
-___
+---
-### MOCKS\_ADMIN
+### MOCKS_ADMIN
-• `Const` **MOCKS\_ADMIN**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt\_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt\_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = adminUser \} \})[]
+• `Const` **MOCKS_ADMIN**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = adminUser \} \})[]
#### Defined in
[src/screens/OrgList/OrgListMocks.ts:235](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/OrgList/OrgListMocks.ts#L235)
-___
+---
-### MOCKS\_EMPTY
+### MOCKS_EMPTY
-• `Const` **MOCKS\_EMPTY**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt\_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `never`[] = [] \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt\_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \})[]
+• `Const` **MOCKS_EMPTY**: (\{ `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `never`[] = [] \} \} \} \| \{ `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \})[]
#### Defined in
[src/screens/OrgList/OrgListMocks.ts:171](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/OrgList/OrgListMocks.ts#L171)
-___
+---
-### MOCKS\_WITH\_ERROR
+### MOCKS_WITH_ERROR
-• `Const` **MOCKS\_WITH\_ERROR**: (\{ `error?`: `undefined` ; `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt\_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `error?`: `undefined` ; `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt\_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \} \| \{ `error`: `Error` ; `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE\_SAMPLE\_ORGANIZATION\_MUTATION; `variables?`: `undefined` \} ; `result?`: `undefined` \})[]
+• `Const` **MOCKS_WITH_ERROR**: (\{ `error?`: `undefined` ; `request`: \{ `notifyOnNetworkStatusChange`: `boolean` = true; `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables`: \{ `filter`: `string` = ''; `first`: `number` = 8; `id?`: `undefined` = '456'; `orderBy`: `string` = 'createdAt_ASC'; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection`: `InterfaceOrgConnectionInfoType`[] = organizations \} \} \} \| \{ `error?`: `undefined` ; `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `filter?`: `undefined` = ''; `first?`: `undefined` = 8; `id`: `string` = '123'; `orderBy?`: `undefined` = 'createdAt_ASC'; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: `InterfaceUserType` = superAdminUser \} \} \| \{ `error`: `Error` ; `request`: \{ `notifyOnNetworkStatusChange?`: `undefined` = true; `query`: `DocumentNode` = CREATE_SAMPLE_ORGANIZATION_MUTATION; `variables?`: `undefined` \} ; `result?`: `undefined` \})[]
#### Defined in
diff --git a/talawa-admin-docs/modules/screens_OrgList_OrganizationModal.md b/talawa-admin-docs/modules/screens_OrgList_OrganizationModal.md
index 70df93939d..baad73e936 100644
--- a/talawa-admin-docs/modules/screens_OrgList_OrganizationModal.md
+++ b/talawa-admin-docs/modules/screens_OrgList_OrganizationModal.md
@@ -12,20 +12,20 @@
### default
-▸ **default**(`props`, `context?`): ``null`` \| `ReactElement`\<`any`, `any`\>
+▸ **default**(`props`, `context?`): `null` \| `ReactElement`\<`any`, `any`\>
Represents the organization modal component.
#### Parameters
-| Name | Type |
-| :------ | :------ |
-| `props` | `PropsWithChildren`\<`InterfaceOrganizationModalProps`\> |
-| `context?` | `any` |
+| Name | Type |
+| :--------- | :------------------------------------------------------- |
+| `props` | `PropsWithChildren`\<`InterfaceOrganizationModalProps`\> |
+| `context?` | `any` |
#### Returns
-``null`` \| `ReactElement`\<`any`, `any`\>
+`null` \| `ReactElement`\<`any`, `any`\>
#### Defined in
diff --git a/talawa-admin-docs/modules/screens_OrganizationDashboard_OrganizationDashboardMocks.md b/talawa-admin-docs/modules/screens_OrganizationDashboard_OrganizationDashboardMocks.md
index 58a98c70e1..d48e597818 100644
--- a/talawa-admin-docs/modules/screens_OrganizationDashboard_OrganizationDashboardMocks.md
+++ b/talawa-admin-docs/modules/screens_OrganizationDashboard_OrganizationDashboardMocks.md
@@ -6,35 +6,35 @@
### Variables
-- [EMPTY\_MOCKS](screens_OrganizationDashboard_OrganizationDashboardMocks.md#empty_mocks)
-- [ERROR\_MOCKS](screens_OrganizationDashboard_OrganizationDashboardMocks.md#error_mocks)
+- [EMPTY_MOCKS](screens_OrganizationDashboard_OrganizationDashboardMocks.md#empty_mocks)
+- [ERROR_MOCKS](screens_OrganizationDashboard_OrganizationDashboardMocks.md#error_mocks)
- [MOCKS](screens_OrganizationDashboard_OrganizationDashboardMocks.md#mocks)
## Variables
-### EMPTY\_MOCKS
+### EMPTY_MOCKS
-• `Const` **EMPTY\_MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Delhi'; `countryCode`: `string` = 'IN'; `dependentLocality`: `string` = 'Some Dependent Locality'; `line1`: `string` = '123 Random Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = '110001'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Delhi' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: \{ `_id`: `string` = '789'; `email`: `string` = 'stevesmith@gmail.com'; `firstName`: `string` = 'Steve'; `lastName`: `string` = 'Smith' \}[] ; `creator`: \{ `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'This is a Dummy Organization'; `image`: `string` = ''; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `membershipRequests`: `never`[] = []; `name`: `string` = 'Dummy Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `postsByOrganizationConnection?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_POST\_CONNECTION\_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations?`: `undefined` ; `postsByOrganizationConnection`: \{ `edges`: `never`[] = [] \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_EVENT\_CONNECTION\_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection`: `never`[] = []; `organizations?`: `undefined` ; `postsByOrganizationConnection?`: `undefined` \} \} \})[]
+• `Const` **EMPTY_MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Delhi'; `countryCode`: `string` = 'IN'; `dependentLocality`: `string` = 'Some Dependent Locality'; `line1`: `string` = '123 Random Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = '110001'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Delhi' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: \{ `_id`: `string` = '789'; `email`: `string` = 'stevesmith@gmail.com'; `firstName`: `string` = 'Steve'; `lastName`: `string` = 'Smith' \}[] ; `creator`: \{ `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `description`: `string` = 'This is a Dummy Organization'; `image`: `string` = ''; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `membershipRequests`: `never`[] = []; `name`: `string` = 'Dummy Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `postsByOrganizationConnection?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_POST_CONNECTION_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations?`: `undefined` ; `postsByOrganizationConnection`: \{ `edges`: `never`[] = [] \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_EVENT_CONNECTION_LIST \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection`: `never`[] = []; `organizations?`: `undefined` ; `postsByOrganizationConnection?`: `undefined` \} \} \})[]
#### Defined in
[src/screens/OrganizationDashboard/OrganizationDashboardMocks.ts:197](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/OrganizationDashboard/OrganizationDashboardMocks.ts#L197)
-___
+---
-### ERROR\_MOCKS
+### ERROR_MOCKS
-• `Const` **ERROR\_MOCKS**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST \} \}[]
+• `Const` **ERROR_MOCKS**: \{ `error`: `Error` ; `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST \} \}[]
#### Defined in
[src/screens/OrganizationDashboard/OrganizationDashboardMocks.ts:281](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/OrganizationDashboard/OrganizationDashboardMocks.ts#L281)
-___
+---
### MOCKS
-• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS\_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Delhi'; `countryCode`: `string` = 'IN'; `dependentLocality`: `string` = 'Some Dependent Locality'; `line1`: `string` = '123 Random Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = '110001'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Delhi' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: \{ `_id`: `string` = '789'; `email`: `string` = 'stevesmith@gmail.com'; `firstName`: `string` = 'Steve'; `lastName`: `string` = 'Smith' \}[] ; `creator`: \{ `email`: `string` = ''; `firstName`: `string` = ''; `lastName`: `string` = '' \} ; `description`: `string` = 'This is a Dummy Organization'; `image`: `string` = ''; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'janedoe@gmail.com'; `firstName`: `string` = 'Jane'; `lastName`: `string` = 'Doe' \} \}[] ; `name`: `string` = 'Dummy Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `postsByOrganizationConnection?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_POST\_CONNECTION\_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations?`: `undefined` ; `postsByOrganizationConnection`: \{ `edges`: \{ `_id`: `string` = '6411e54835d7ba2344a78e29'; `commentCount`: `number` = 2; `comments`: \{ `__typename`: `string` = 'Comment'; `_id`: `string` = '64eb13beca85de60ebe0ed0e'; `creator`: \{ `__typename`: `string` = 'User'; `_id`: `string` = '63d6064458fce20ee25c3bf7'; `email`: `string` = 'test@gmail.com'; `firstName`: `string` = 'Noble'; `lastName`: `string` = 'Mittal' \} ; `likeCount`: `number` = 1; `likedBy`: \{ `_id`: `number` = 1 \}[] ; `text`: `string` = 'Yes, that is $50' \}[] ; `createdAt`: `Dayjs` ; `creator`: \{ `_id`: `string` = '640d98d9eb6a743d75341067'; `email`: `string` = 'adidacreator1@gmail.com'; `firstName`: `string` = 'Aditya'; `lastName`: `string` = 'Shelke' \} ; `imageUrl`: ``null`` = null; `likeCount`: `number` = 0; `likedBy`: \{ `_id`: `string` = '63d6064458fce20ee25c3bf7'; `firstName`: `string` = 'Comment'; `lastName`: `string` = 'Likkert' \}[] ; `pinned`: `boolean` = false; `text`: `string` = 'Hey, anyone saw my watch that I left at the office?'; `title`: `string` = 'Post 2'; `videoUrl`: ``null`` = null \}[] \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_EVENT\_CONNECTION\_LIST; `variables`: \{ `organization_id`: `string` = '123' \} \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection`: \{ `_id`: `string` = '1'; `allDay`: `boolean` = false; `description`: `string` = 'Sample Description'; `endDate`: `string` = '2023-10-29T23:59:59.000Z'; `endTime`: `string` = '17:00:00'; `isPublic`: `boolean` = true; `isRegisterable`: `boolean` = true; `location`: `string` = 'Sample Location'; `recurring`: `boolean` = false; `startDate`: `string` = '2023-10-29T00:00:00.000Z'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Sample Event' \}[] ; `organizations?`: `undefined` ; `postsByOrganizationConnection?`: `undefined` \} \} \})[]
+• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = ORGANIZATIONS_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Delhi'; `countryCode`: `string` = 'IN'; `dependentLocality`: `string` = 'Some Dependent Locality'; `line1`: `string` = '123 Random Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = '110001'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Delhi' \} ; `admins`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `blockedUsers`: \{ `_id`: `string` = '789'; `email`: `string` = 'stevesmith@gmail.com'; `firstName`: `string` = 'Steve'; `lastName`: `string` = 'Smith' \}[] ; `creator`: \{ `email`: `string` = ''; `firstName`: `string` = ''; `lastName`: `string` = '' \} ; `description`: `string` = 'This is a Dummy Organization'; `image`: `string` = ''; `members`: \{ `_id`: `string` = '123'; `email`: `string` = 'johndoe@gmail.com'; `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \}[] ; `membershipRequests`: \{ `_id`: `string` = '456'; `user`: \{ `email`: `string` = 'janedoe@gmail.com'; `firstName`: `string` = 'Jane'; `lastName`: `string` = 'Doe' \} \}[] ; `name`: `string` = 'Dummy Organization'; `userRegistrationRequired`: `boolean` = true; `visibleInSearch`: `boolean` = false \}[] ; `postsByOrganizationConnection?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_POST_CONNECTION_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection?`: `undefined` ; `organizations?`: `undefined` ; `postsByOrganizationConnection`: \{ `edges`: \{ `_id`: `string` = '6411e54835d7ba2344a78e29'; `commentCount`: `number` = 2; `comments`: \{ `__typename`: `string` = 'Comment'; `_id`: `string` = '64eb13beca85de60ebe0ed0e'; `creator`: \{ `__typename`: `string` = 'User'; `_id`: `string` = '63d6064458fce20ee25c3bf7'; `email`: `string` = 'test@gmail.com'; `firstName`: `string` = 'Noble'; `lastName`: `string` = 'Mittal' \} ; `likeCount`: `number` = 1; `likedBy`: \{ `_id`: `number` = 1 \}[] ; `text`: `string` = 'Yes, that is $50' \}[] ; `createdAt`: `Dayjs` ; `creator`: \{ `_id`: `string` = '640d98d9eb6a743d75341067'; `email`: `string` = 'adidacreator1@gmail.com'; `firstName`: `string` = 'Aditya'; `lastName`: `string` = 'Shelke' \} ; `imageUrl`: `null` = null; `likeCount`: `number` = 0; `likedBy`: \{ `_id`: `string` = '63d6064458fce20ee25c3bf7'; `firstName`: `string` = 'Comment'; `lastName`: `string` = 'Likkert' \}[] ; `pinned`: `boolean` = false; `text`: `string` = 'Hey, anyone saw my watch that I left at the office?'; `title`: `string` = 'Post 2'; `videoUrl`: `null` = null \}[] \} \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_EVENT_CONNECTION_LIST; `variables`: \{ `organization_id`: `string` = '123' \} \} ; `result`: \{ `data`: \{ `eventsByOrganizationConnection`: \{ `_id`: `string` = '1'; `allDay`: `boolean` = false; `description`: `string` = 'Sample Description'; `endDate`: `string` = '2023-10-29T23:59:59.000Z'; `endTime`: `string` = '17:00:00'; `isPublic`: `boolean` = true; `isRegisterable`: `boolean` = true; `location`: `string` = 'Sample Location'; `recurring`: `boolean` = false; `startDate`: `string` = '2023-10-29T00:00:00.000Z'; `startTime`: `string` = '08:00:00'; `title`: `string` = 'Sample Event' \}[] ; `organizations?`: `undefined` ; `postsByOrganizationConnection?`: `undefined` \} \} \})[]
#### Defined in
diff --git a/talawa-admin-docs/modules/screens_Users_UsersMocks.md b/talawa-admin-docs/modules/screens_Users_UsersMocks.md
index 31b72fc622..dca7939a7e 100644
--- a/talawa-admin-docs/modules/screens_Users_UsersMocks.md
+++ b/talawa-admin-docs/modules/screens_Users_UsersMocks.md
@@ -6,35 +6,35 @@
### Variables
-- [EMPTY\_MOCKS](screens_Users_UsersMocks.md#empty_mocks)
+- [EMPTY_MOCKS](screens_Users_UsersMocks.md#empty_mocks)
- [MOCKS](screens_Users_UsersMocks.md#mocks)
- [MOCKS2](screens_Users_UsersMocks.md#mocks2)
## Variables
-### EMPTY\_MOCKS
+### EMPTY_MOCKS
-• `Const` **EMPTY\_MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = USER\_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `users`: `never`[] = [] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: `never`[] = []; `users?`: `undefined` \} \} \})[]
+• `Const` **EMPTY_MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = USER_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `users`: `never`[] = [] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: `never`[] = []; `users?`: `undefined` \} \} \})[]
#### Defined in
[src/screens/Users/UsersMocks.ts:392](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/Users/UsersMocks.ts#L392)
-___
+---
### MOCKS
-• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `first?`: `undefined` = 8; `firstName_contains?`: `undefined` = 'john'; `id`: `string` = 'user1'; `lastName_contains?`: `undefined` = ''; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user`: \{ `_id`: `string` = 'user1'; `adminFor`: \{ `_id`: `number` = 1; `image`: `string` = ''; `name`: `string` = 'Palisadoes' \}[] ; `email`: `string` = 'John\_Does\_Palasidoes@gmail.com'; `firstName`: `string` = 'John'; `image`: `string` = ''; `lastName`: `string` = 'Doe'; `userType`: `string` = 'SUPERADMIN' \} ; `users?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = USER\_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `id?`: `undefined` = '456'; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user?`: `undefined` ; `users`: \{ `_id`: `string` = 'user1'; `adminApproved`: `boolean` = true; `adminFor`: \{ `_id`: `string` = '123' \}[] ; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `joinedOrganizations`: \{ `_id`: `string` = 'abc'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `name`: `string` = 'Joined Organization 1' \}[] ; `lastName`: `string` = 'Doe'; `organizationsBlockedBy`: \{ `_id`: `string` = 'xyz'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `name`: `string` = 'ABC' \}[] ; `userType`: `string` = 'SUPERADMIN' \}[] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = 'user1' \}[] ; `createdAt`: `string` = '09/11/2001'; `creator`: \{ `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `members`: \{ `_id`: `string` = 'user1' \}[] ; `name`: `string` = 'Palisadoes' \}[] ; `user?`: `undefined` ; `users?`: `undefined` \} \} \})[]
+• `Const` **MOCKS**: (\{ `request`: \{ `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `first?`: `undefined` = 8; `firstName_contains?`: `undefined` = 'john'; `id`: `string` = 'user1'; `lastName_contains?`: `undefined` = ''; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user`: \{ `_id`: `string` = 'user1'; `adminFor`: \{ `_id`: `number` = 1; `image`: `string` = ''; `name`: `string` = 'Palisadoes' \}[] ; `email`: `string` = 'John_Does\_Palasidoes@gmail.com'; `firstName`: `string` = 'John'; `image`: `string` = ''; `lastName`: `string` = 'Doe'; `userType`: `string` = 'SUPERADMIN' \} ; `users?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = USER_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `id?`: `undefined` = '456'; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user?`: `undefined` ; `users`: \{ `_id`: `string` = 'user1'; `adminApproved`: `boolean` = true; `adminFor`: \{ `_id`: `string` = '123' \}[] ; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `joinedOrganizations`: \{ `_id`: `string` = 'abc'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `name`: `string` = 'Joined Organization 1' \}[] ; `lastName`: `string` = 'Doe'; `organizationsBlockedBy`: \{ `_id`: `string` = 'xyz'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `name`: `string` = 'ABC' \}[] ; `userType`: `string` = 'SUPERADMIN' \}[] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = 'user1' \}[] ; `createdAt`: `string` = '09/11/2001'; `creator`: \{ `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `members`: \{ `_id`: `string` = 'user1' \}[] ; `name`: `string` = 'Palisadoes' \}[] ; `user?`: `undefined` ; `users?`: `undefined` \} \} \})[]
#### Defined in
[src/screens/Users/UsersMocks.ts:7](https://github.com/PalisadoesFoundation/talawa-admin/blob/12d9229/src/screens/Users/UsersMocks.ts#L7)
-___
+---
### MOCKS2
-• `Const` **MOCKS2**: (\{ `request`: \{ `query`: `DocumentNode` = USER\_ORGANIZATION\_LIST; `variables`: \{ `first?`: `undefined` = 8; `firstName_contains?`: `undefined` = 'john'; `id`: `string` = 'user1'; `lastName_contains?`: `undefined` = ''; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user`: \{ `_id`: `string` = 'user1'; `adminFor`: \{ `_id`: `number` = 1; `image`: `string` = ''; `name`: `string` = 'Palisadoes' \}[] ; `email`: `string` = 'John\_Does\_Palasidoes@gmail.com'; `firstName`: `string` = 'John'; `image`: `string` = ''; `lastName`: `string` = 'Doe'; `userType`: `string` = 'SUPERADMIN' \} ; `users?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = USER\_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `id?`: `undefined` = '456'; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user?`: `undefined` ; `users`: \{ `_id`: `string` = 'user1'; `adminApproved`: `boolean` = true; `adminFor`: \{ `_id`: `string` = '123' \}[] ; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `joinedOrganizations`: \{ `_id`: `string` = 'abc'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `name`: `string` = 'Joined Organization 1' \}[] ; `lastName`: `string` = 'Doe'; `organizationsBlockedBy`: \{ `_id`: `string` = 'xyz'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: ``null`` = null; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `name`: `string` = 'ABC' \}[] ; `userType`: `string` = 'SUPERADMIN' \}[] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION\_CONNECTION\_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = 'user1' \}[] ; `createdAt`: `string` = '09/11/2001'; `creator`: \{ `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `image`: ``null`` = null; `members`: \{ `_id`: `string` = 'user1' \}[] ; `name`: `string` = 'Palisadoes' \}[] ; `user?`: `undefined` ; `users?`: `undefined` \} \} \})[]
+• `Const` **MOCKS2**: (\{ `request`: \{ `query`: `DocumentNode` = USER_ORGANIZATION_LIST; `variables`: \{ `first?`: `undefined` = 8; `firstName_contains?`: `undefined` = 'john'; `id`: `string` = 'user1'; `lastName_contains?`: `undefined` = ''; `skip?`: `undefined` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user`: \{ `_id`: `string` = 'user1'; `adminFor`: \{ `_id`: `number` = 1; `image`: `string` = ''; `name`: `string` = 'Palisadoes' \}[] ; `email`: `string` = 'John_Does\_Palasidoes@gmail.com'; `firstName`: `string` = 'John'; `image`: `string` = ''; `lastName`: `string` = 'Doe'; `userType`: `string` = 'SUPERADMIN' \} ; `users?`: `undefined` \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = USER_LIST; `variables`: \{ `first`: `number` = 12; `firstName_contains`: `string` = ''; `id?`: `undefined` = '456'; `lastName_contains`: `string` = ''; `skip`: `number` = 0 \} \} ; `result`: \{ `data`: \{ `organizationsConnection?`: `undefined` = organizations; `user?`: `undefined` ; `users`: \{ `_id`: `string` = 'user1'; `adminApproved`: `boolean` = true; `adminFor`: \{ `_id`: `string` = '123' \}[] ; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `joinedOrganizations`: \{ `_id`: `string` = 'abc'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `name`: `string` = 'Joined Organization 1' \}[] ; `lastName`: `string` = 'Doe'; `organizationsBlockedBy`: \{ `_id`: `string` = 'xyz'; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `createdAt`: `string` = '20/06/2022'; `creator`: \{ `_id`: `string` = '123'; `createdAt`: `string` = '20/06/2022'; `email`: `string` = 'john@example.com'; `firstName`: `string` = 'John'; `image`: `null` = null; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `name`: `string` = 'ABC' \}[] ; `userType`: `string` = 'SUPERADMIN' \}[] \} \} \} \| \{ `request`: \{ `query`: `DocumentNode` = ORGANIZATION_CONNECTION_LIST; `variables?`: `undefined` \} ; `result`: \{ `data`: \{ `organizationsConnection`: \{ `_id`: `number` = 123; `address`: \{ `city`: `string` = 'Kingston'; `countryCode`: `string` = 'JM'; `dependentLocality`: `string` = 'Sample Dependent Locality'; `line1`: `string` = '123 Jamaica Street'; `line2`: `string` = 'Apartment 456'; `postalCode`: `string` = 'JM12345'; `sortingCode`: `string` = 'ABC-123'; `state`: `string` = 'Kingston Parish' \} ; `admins`: \{ `_id`: `string` = 'user1' \}[] ; `createdAt`: `string` = '09/11/2001'; `creator`: \{ `firstName`: `string` = 'John'; `lastName`: `string` = 'Doe' \} ; `image`: `null` = null; `members`: \{ `_id`: `string` = 'user1' \}[] ; `name`: `string` = 'Palisadoes' \}[] ; `user?`: `undefined` ; `users?`: `undefined` \} \} \})[]
#### Defined in